
F3: a small PHP framework with great features
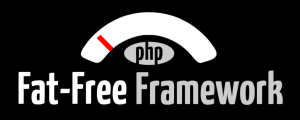
I want to bring to your attention a lightweight PHP framework that I recently came across and which I immediately fell in love with.
Fat-Free is somewhat similar to the famous Sinatra Ruby framework. The author of Fat-Free is obsessed with minimalism and code cleanliness, which positively affected this simple framework for developing a wide variety of applications.
Fat-Free consists of a single file and weighs only 55KB. At the same time, the framework has such functionality: a specific and fairly convenient template engine, flexible caching, automatic spam protection, integrated tools for unit tests, and code profiler.
It is so small and fast that it can even be used to control Web server traffic.
It is also the only framework that protects your application from hotlinking and DoS attacks.
It doesn't matter if you are a beginner or a professional PHP-programmer, Fat-Free is easy to use and powerful at the same time. It does not require installation or a clear directory structure. This framework allows you to write applications in both object-oriented and procedural styles. The Fat-Free philosophy and its approach to MVC architecture strive to minimize structural components, avoid complexity and balance between code elegance, application performance and programmer productivity.
Fat-Free comes with plugins that will help expand the capabilities of the framework:
- Auto-mapping ORM called Axon, which automatically determines the table fields in the database and creates the corresponding object variables for them;
- M2 for working with MongoDB;
- Form handler;
- CAPTCHA generator;
- JavaScript and CSS compressor;
- Dynamic XML sitemap generator
- Thumbnail and pseudo-image generator;
- Identicons
- Authorization
- Flexible logging system;
- Means for communication with other servers;
- Processing ZIP archives;
- Functions for working with the Google API, Twitter, Yahoo! and Akismet;
- Support for multilingualism.
Here is the file that displays the greeting on the main page:
- require_once 'path/to/F3.php';
- F3::route('GET /','home');
- function home() {
- echo 'Hello, world!';
- }
- F3::run();
-
Getting to work
Attention: for the framework to work, it needs a PHP version of at least 5.3 and an installed and configured mod_rewrite!
In order not to make this guide too cumbersome, I decided to avoid writing a design and some obvious features for our simple guestbook application.
To begin, create a database in any way convenient for you. Both SQLite / MySQL and MongoDB can act as databases. Since the application is exponential and simple, I choose SQLite.
There is only one table in my guestbook.sqlite database - Comments , which contains the fields id , author and comment .
Create the index.php file in the root of your application and rename the htaccess file to .htaccess (with a dot in front).
Next, connect the main and only framework file in index.php and determine the path to the database:
- require_once ('F3 / F3.php'); // connect the framework
- F3 :: set ('DB', array ('dsn' => 'sqlite: guestbook.sqlite')); / * Initialization of connection to the database.
- Attention: if you have version sqlite = 2, the DSN should look like this:
- 'dsn' => 'sqlite2: guestbook.sqlite'
- * /
In the first example, where the Hello World message is displayed, you saw one of the methods for processing routes (paths). In fact, there are several of them and you are free to choose:
1. Route handler function - as in the first example. The second parameter of the F3 :: rote () method is the function that we describe in the same file, anywhere
2. Anonymous function in the second parameter of the F3 :: rote () method : 3. The static class method in the same second parameter:
- require_once 'path/to/F3.php';
- F3::route('GET /',function () {
- echo 'Hello, world!';
- });
- F3::run();
- require_once 'path / to / F3.php';
- class MyClass {
- public static function hello () {
- echo 'Hello, world!';
- }
- }
- F3 :: route ('GET /', 'MyClass :: hello');
- F3 :: run ();
4. The usual class method:
- require_once 'path / to / F3.php';
- class MyClass {
- public function hello () {
- echo 'Hello, world!';
- }
- }
- $ test = new MyClass;
- F3 :: route ('GET /', array ($ test, 'hello'));
- F3 :: run ();
5. Finally, the handler may be a separate file located in an arbitrary directory with other such files. The directory with the handler files must be declared at the beginning of the index.php file, after which you can call the route handler file:
- //index.php:
- require_once 'path / to / F3.php';
- F3 :: set ('IMPORTS', 'yourpath');
- F3 :: route ('GET /', (: yourfile));
- F3 :: run ();
- //yourpath/yourfile.php
We continue the development of the guest book. Add the path to the plugin files in the index.php file - we need this to use the ORM called Axon, which comes with Fat-Free: Add the processing of the main page request in one of the above ways. I choose the first as the most visual. Here you go. Displaying comments is already working. You can proceed to the addition. But first, I want to talk a little about templates. The path to the templates is set in the same way as the others: You cannot pass a variable to a specific template. Variables in Fat-Free are set for the entire application. This is done using the already familiar static method:
- require_once('F3/F3.php');
- F3::set('AUTOLOAD','autoload/'); /*установка пути к плагинам.
- По умолчанию папка с плагинами называется autoload.
- Вы можете переименовать её и вынести за пределы корня сайта для большей безопасности.*/
- F3::set('DB',array('dsn'=>'sqlite:guestbook.sqlite'));
- require_once('F3/F3.php');
- F3::set('AUTOLOAD','autoload/');
- F3::set('DB',array('dsn'=>'sqlite:guestbook.sqlite'));
- F3::route('GET /','home');
- function home() {
- $comments = new Axon('comments'); //подключение к таблице comments БД
- $all_comments = $comments->find(); // Извлечение всех комментариев
- foreach ($all_comments as $comment) { /* Обход всех результатов в массиве
- и последуюющее их отображение. Напомню, что в данном примере
- я пишу минимальный код без проверок на ошибки. */
- echo 'Автор: '.$comment['author'].'
'; - echo 'Комментарий: '.$comment['comment'].'
'; - }
- }
- F3::set('GUI','путь к шаблонам');
- F3::set('переменная','значение');
Now this variable is available everywhere. In HTML code and in string form, its value is called as {@ variable}, in PHP code as:
- F3 :: get ('variable');
To call the template, you should use the serve method: Thus, my application now looks like: In this example, we see how the Fat-Free template engine works: the loop processes a variable that contains all the comments. The next step is to add a comment form to the page. To do this, first describe the form itself in the form.html file located in the templates folder. In my case, the form looks like this. Next, you need to download this form inside the existing main page template in order to display the form under all comments. To do this, use the template tag inside the template.html template.
- F3::serve('template.html');
- //index.php:
-
- require_once('F3/F3.php');
- F3::set('AUTOLOAD','autoload/');
- F3::set('DB',array('dsn'=>'sqlite:guestbook.sqlite'));
-
- F3::route('GET /','home');
- function home() {
- $comments = new Axon('comments'); //подключение к таблице comments БД
- $all_comments = $comments->find(); // Извлечение всех комментариев
- F3::set('comments',$all_comments);
- F3::serve('template.html');
- }
-
- ?>
-
-
-
{@comment.id}
-
Now you should have a question - how to process the data sent? Everything is simple. Declare a handler in the index.php file for the POST method of the main page: Well, that’s it. The minimum guest book is ready. It took about 30 lines of code! Framework site Download the latest version of Fat-Free IRC support on irc.freenode.net: #fatfree The author of the framework is Bong Cosca. Here is his blog.
- require_once('F3/F3.php');
- F3::set('AUTOLOAD','autoload/');
- F3::set('DB',array('dsn'=>'sqlite:guestbook.sqlite'));
-
- F3::route('GET /','home');
- function home() {
- $comments = new Axon('comments');
- $all_comments = $comments->find();
- F3::set('comments',$all_comments);
- F3::serve('template.html');
- }
-
- F3::route('POST /','savecomment');
- function savecomment() {
- $comments = new Axon('comment');
- $comments->copyFrom('REQUEST'); /* Если названия полей формы
- соответствуют названиям полей таблицы,
- нет необходимости присваивать каждое значение отдельно.
- Можно воспользоваться удобной функцией copyFrom
- и получить данные из переменной $_REQUEST */
-
- /* ...
- * тут добавьте обработку полученных данных
- */
-
- $comments->save(); // добавление в базу данных
- $F3::reroute('/'); // переадресация на главную страницу
- }
- ?>