Powering your Raspberry Pi using Arduino
Many probably know that supplying power to the Arduino from the Raspberry Pi is not difficult, you just need a USB cable for this. The inverse problem looks more complicated, since most Arduino controllers do not have a USB output (Due is an exception). However, this can be done using the GPIO pins, and I want to talk about a specific example for the Arduino Nano V3.0 and Raspberry Pi B rev.2. In addition to the power supply itself, I will also tell you how to control this power using the button and the MOSFET transistor.
Most Arduino-compatible controllers use 5V pins. The only exception is the Arduino Due and 3.3V output from the Arduino, but this is not the case now. It is also known that one of the ways to power the Raspberry Pi is to use 5V and GND pins on the 26-pin P1 connector:

It would seem that the solution is obvious - you need to connect the Raspberry Pi to any of the Arduino pins, and everything will work. My attempt to do this caused the Raspberry Pi to light up with the PWR LED, but the ACT LED did not light up. The reason is the very small current strength from the Arduino pins (about 40-50 mA). But Arduino has a separate 5V pin, which (according to the link ) can produce about 400-500 mA. Now you need to check if there is enough current to power the Raspberry.
Raspberry Pi needs about 700 mA for normal power with two USB devices connected. Each USB device can consume up to 140 mA ( source ). Raspberries can consume even more current if it is dispersed (mine is not). Thus, if you use non-accelerated RPi without USB devices, then the current from the Arduino 5V pin should be enough.
In order to control the power supply, you need a few more ingredients: a power button and something that can control high currents. I used a MOSFET transistor for these purposes. We proceed directly to the used parts.
I used the following "iron" parts:
For the Arduino firmware, you need an IDE, I used version 1.5.8 of BETA, but stable 1.0.6 is also suitable. You will also need my little library for PowerButton (link at the end of the article in the section on utilities).
The connection diagram looks like this:

Schematic diagram like this:
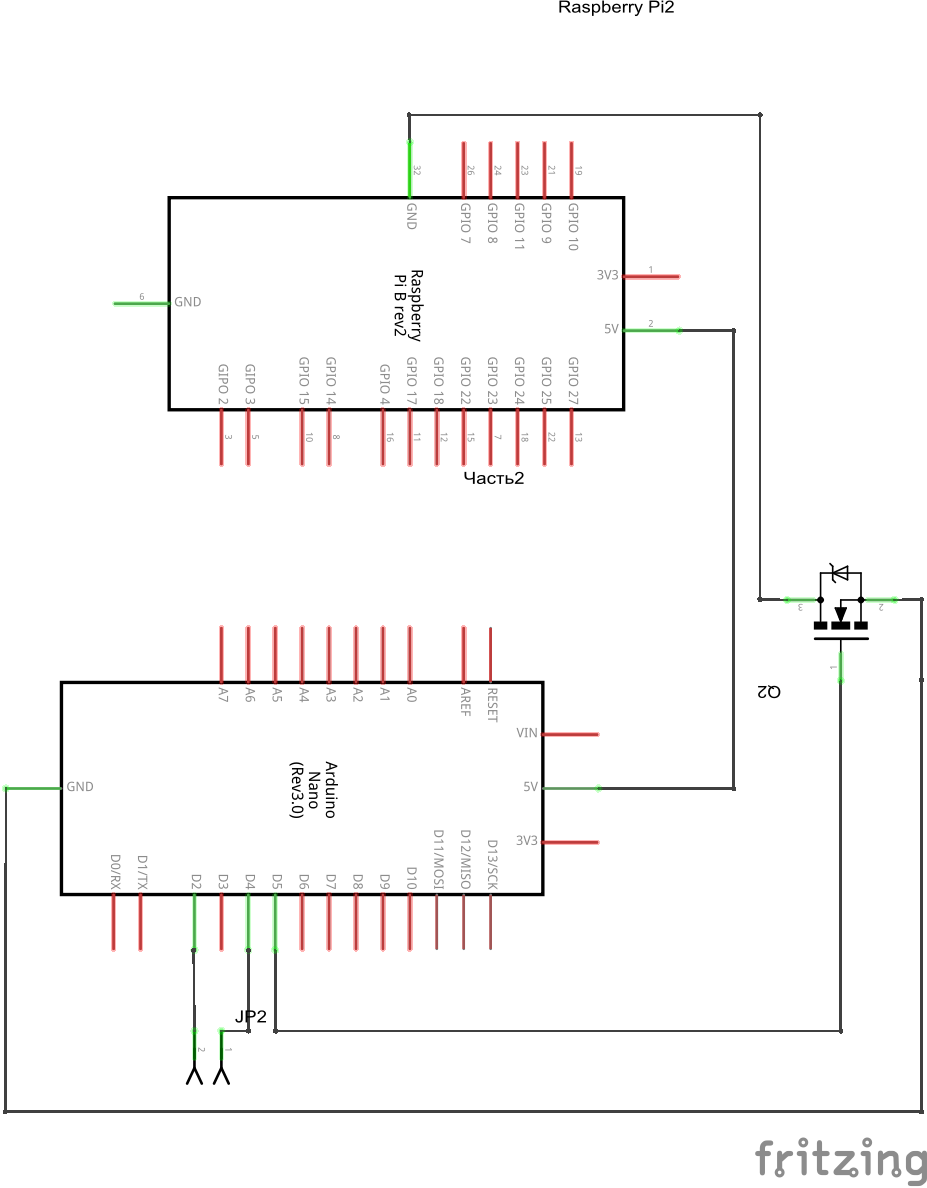
Explanations for the schemes:
Connecting to a D2 pin is not accidental: the library for the button uses interrupts, and for Arduino Nano, only D2 / D3 pins are designed for these purposes (you can check which pins on your Arduino support interrupts here ).
The library takes most of the actions, so the code is very simple.
Short video with testing:
As you can see, visually everything works. But still, you need to check the voltage between the pins TP1 / TP2 (the technique is here ). I got a value of ~ 4.6V, the recommended value is more than 4.75V.
Despite the fact that everything works, there is still a suspicion that when connecting a peripheral current from 5V, the Arduino pin will not be enough. MOSFET and the button work perfectly in pairs, such a bundle may come in handy for future projects.
Utilities and libraries used for writing:
Since this is my first post, reviews and comments will be very useful.
Theoretical opportunity
Most Arduino-compatible controllers use 5V pins. The only exception is the Arduino Due and 3.3V output from the Arduino, but this is not the case now. It is also known that one of the ways to power the Raspberry Pi is to use 5V and GND pins on the 26-pin P1 connector:

It would seem that the solution is obvious - you need to connect the Raspberry Pi to any of the Arduino pins, and everything will work. My attempt to do this caused the Raspberry Pi to light up with the PWR LED, but the ACT LED did not light up. The reason is the very small current strength from the Arduino pins (about 40-50 mA). But Arduino has a separate 5V pin, which (according to the link ) can produce about 400-500 mA. Now you need to check if there is enough current to power the Raspberry.
Raspberry Pi needs about 700 mA for normal power with two USB devices connected. Each USB device can consume up to 140 mA ( source ). Raspberries can consume even more current if it is dispersed (mine is not). Thus, if you use non-accelerated RPi without USB devices, then the current from the Arduino 5V pin should be enough.
In order to control the power supply, you need a few more ingredients: a power button and something that can control high currents. I used a MOSFET transistor for these purposes. We proceed directly to the used parts.
Necessary hardware and software
I used the following "iron" parts:
- Raspberry Pi B rev. 2;
- Arduino Nano V3.0;
- button for power control (I used a button with a latch and a signal wire);
- MOSFET transistor (I had an IRF530N);
- Breadboard and some wires.
For the Arduino firmware, you need an IDE, I used version 1.5.8 of BETA, but stable 1.0.6 is also suitable. You will also need my little library for PowerButton (link at the end of the article in the section on utilities).
Schemes
The connection diagram looks like this:

Schematic diagram like this:
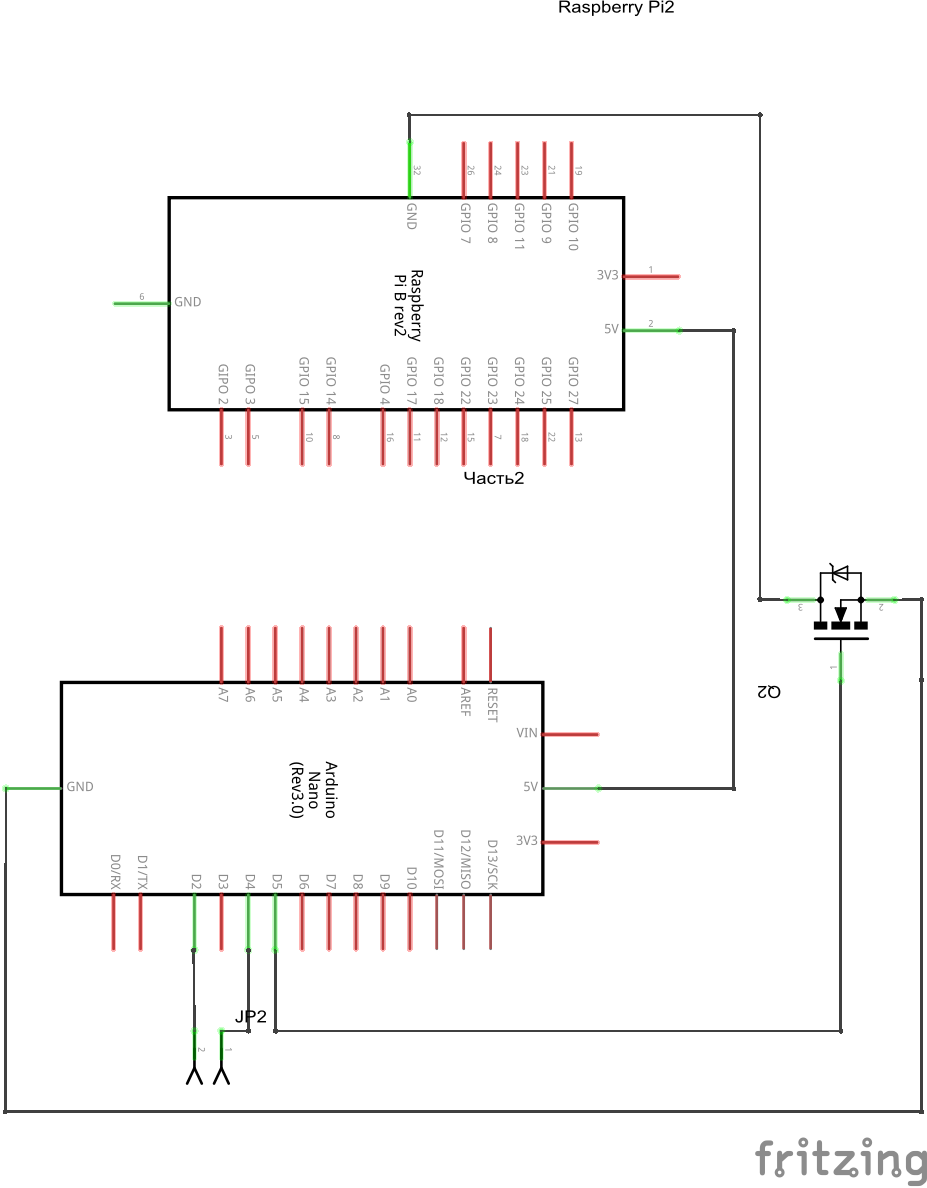
Explanations for the schemes:
- D2 is connected to the SIG pin at the button.
- D4 is connected to the VCC pin of the button.
- D5 is connected to the MOSFET shutter.
Connecting to a D2 pin is not accidental: the library for the button uses interrupts, and for Arduino Nano, only D2 / D3 pins are designed for these purposes (you can check which pins on your Arduino support interrupts here ).
Source code of the program for Arduino
#include
#define POWER_PIN_SIG 2
#define POWER_PIN_VCC 4
#define POWER_FET_GATE 5
#define POWER_PIN_INT 0
PowerButtonSwitch pbs;
void onPowerOn () {
Serial.println ("Power On");
digitalWrite (POWER_FET_GATE, 1); // Open the shutter (gate)
}
void onPowerOff () {
Serial.println ("Power Off");
digitalWrite (POWER_FET_GATE, 0); // Close the gate (gate)
}
void setup () {
Serial.begin (9600);
// Signal output from Arduino to the gate MOSFET (gate)
pinMode (POWER_FET_GATE, OUTPUT);
digitalWrite (POWER_FET_GATE, 0);
// Initial setting of the power button
pbs.setupPowerButton (POWER_PIN_SIG, POWER_PIN_VCC, POWER_PIN_INT);
// Read the current value
// If there is a signal from the button,
// turn on the Raspberry Pi
int st = pbs.getSwitchStatus ();
if (st == POWER_ON) {
onPowerOn ();
}
// Add event handlers
pbs.onPowerOn (onPowerOn);
pbs.onPowerOff (onPowerOff);
}
void loop () {
// Empty loop
delay (1000);
Serial.println ("No actions");
}
The library takes most of the actions, so the code is very simple.
Solution testing
Short video with testing:
As you can see, visually everything works. But still, you need to check the voltage between the pins TP1 / TP2 (the technique is here ). I got a value of ~ 4.6V, the recommended value is more than 4.75V.
Conclusion
Despite the fact that everything works, there is still a suspicion that when connecting a peripheral current from 5V, the Arduino pin will not be enough. MOSFET and the button work perfectly in pairs, such a bundle may come in handy for future projects.
Utilities and libraries used for writing:
- Fritzing : used to draw diagrams, available here .
- the actual library for PowerButton : you can get it from GitHub here .
Since this is my first post, reviews and comments will be very useful.