
Advanced Python Content
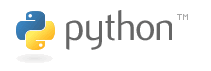
After reading Dive into Python or similar and reading the documentation, the question arises, but what to read next? You can refer to the list of books at python.org. There is a section of Advanced Books , but there are only 6 books in it (the seventh did not appear), and only one I would call truly worthwhile.
Fortunately, Python has very detailed and high-quality documentation. But even in it, many topics are either only superficially touched, or it is very difficult to find (because the documentation is large, and if you don’t know where to look, you won’t find it).
Below are collected complex materials about Python, its device and capabilities. Everything is in English (sin, not knowing technical English). About Dive into Python I cunning. Most of the above materials require a good knowledge of Python and the presence of programming experience on it.
Articles from official documentation
Data model A
basic article about objects in Python. It must be known by heart (truth, truth). Without it, further understanding of how Python works is almost impossible. I would also like to focus on:
- Implementing Descriptors . In my opinion, descriptors are Python’s best, unmatched ability, but the most underestimated one. Now they are, for the most part, used only in frameworks. It will be necessary, somehow, to write how descriptors simplify development and improve the quality of the code.
- Customizing attribute access . And the first thing to read about is the difference between
__getattribute__
and__getattr__
. - Special method lookup for new-style classes . Important: access to magic methods is bypassed
__getattribute__
unless you directly call them (len(obj)
andobj.__len__()
).
Exceptions Exceptions
deal with inheritance ( PEP 352: Exceptions as New-Style Classes ). In Python, 2.4
Exception
is a classic class, not a subclass object
. In Python 2.5, it Exception
already inherits BaseException(object)
, i.e. this is a new class. Also note that in version 2.4 KeyboardInterrupt
they SystemExit
inherit Exception
, and in 2.5 - BaseException
. I also recommend directly taking and comparing (putting two windows side by side) the hierarchy of exceptions in Python 2.4 and the hierarchy in Python 2.6 (compared to 2.5, only a few classes were added).
Articles about classes, attributes, and methods
Unifying types and classes in Python 2.2 , Guido van Rossum
An article from the creator of the language about what new-style classes are. I think it’s not necessary to write why it should be read (better, several times).
The Python 2.3 Method Resolution Order , Michele Simionato
How the Method of Access to Methods (MRO) and Attributes are Calculated for Multiple Inheritance. New classes can be obtained through the attribute
__mro__
. Shalabh Chaturvedi:
Very nice and great article books + beautifully laid out.
Python Types and Objects
How
type
andobject
. Python Attributes and Methods
How attributes are accessed in new classes, the difference between functions and methods, description of descriptors and MRO.
Be Pythonic
A short, not so important article, how to write code in the style of Python (added only to list all the articles of the author).
Metaclasses
I would like to give one [very famous] quote about metaclasses:
English Русский [Metaclasses] are deeper magic Metaclasses are complex magic, than 99% of users should ever about which 99% of developers even worry about. If you wonder do not worry. If you whether you need them, you ask if you need don't (the people who actually metaclasses, know they don’t need them know with certainty need (people to whom they that they need them, and don't really need, absolutely need an explanation about why) are sure of this, and they don’t need Tim Peters (clp post 2002-12-22) explanation of why). (it’s very bad that you don’t know English in this article links only in English materials)
It’s easier to insert as monospaced than to try to draw a table with cells without the width attribute, not to mention the styles. I honestly tried for about 20 minutes.
Now, this is not so . If you do not know metaclasses, you do not know Python. Now, after motivation, you can return to the articles.
Customizing class creation White
papers.
Python Metaclasses: Who? Why? When? , Mike Fletcher , PDF
Many practical examples of how and why it is possible [but often not necessary , ed.] To use metaclasses.
Metaclass programming in Python , Part 2 , Part 3 , David Mertz, Michele Simionato
Very detailed articles, understands everything by bones. The only BUT: it is very boring and academic written. Although it is entirely possible that this is just my bias towards articles on IBM DeveloperWorks.
Metaclasses in Python 3000, PEP 3115
Metaclasses in Third Python. The main differences are the ability to pass kwargs directly in the class definition
class Foo(*bases, **kwargs)
and support for a function __prepare__
that should return mapping for class attributes, such as an ordered dictionary. In general, about metaclasses: to know, to know, and to know again. But! If you decide to use them, think, then think again and, nevertheless, try to do without them ;-D.
Articles on other topics
Python 401: Some Advanced Topics , PDF The
best and most detailed explanation of string interpolation (
'Hello, %s.' % username
). Plus iterators, generators, descriptors, metaclasses, why methods are slower than functions (because an object is created with every call), etc. How-To Guide for Descriptors , Raymond Hettinger
How Descriptors Work, How to Call and Use Them.
David Beazley
writes very good, and most importantly, practical articles. Only he can read how to use the generators to parse the Apache log or how to write an “operating system” on coroutines.
Inside the Python GIL
How Global Interpreter Lock works and why using threads in Python can slow down a program.
Inside the New GIL (Python 3.2)
Description and tests of the new GIL, which will be in Python 3.2 (and maybe will be ported in 2.7).
Generator Tricks for Systems Programmers , Version 2 The
practical use of generators and their advantages (speed + low memory consumption).
A Curious Course on Coroutines and Concurrency
What are coroutines and why it is necessary to clearly distinguish them from ordinary generators (they have different functions: some produce data, others process them). In the end, he will write an analog of the operating system on them.
My recommendations
See the result
dir(method)
anddir(func)
. Read the FAQ on the modulesnew
,itertools
,functools
. Be sure, if you still do not know, get acquainted with the functionsmap
, reduce
, zip
And filter
(map can take just a lot of iterators). Read about Abstract Base Classes and collections. And not only in Python, but also in Java . ABC is in many ways following in its wake.
Visit the website of PyCon , the annual developer conference. The materials of past conferences are also available there (although they are often not available). You need to watch in Conference / Schedule .
I almost forgot, be sure to read the Python Cookbook. This practical book is simply excellent. How to quickly sort using the decorate-sort-redecorate method, which sorting algorithm Python uses (insertion sort for a small number of elements and timsort for a large one), how to work with XML, dynamically create modules and much more.
Conclusion
I wrote that I remembered or found in bookmarks. Hope it helps a lot. Also don't forget ipython and it? and ?? .. (for those who don’t know, this is a very powerful interactive shell for Python, and questions show information about objects, for example,
import sys; sys?
). May strength come in you.