"Inheritance" is not from classes
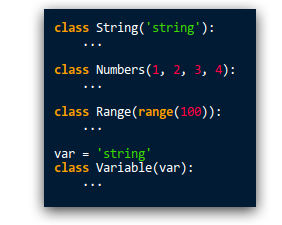
In Python, it doesn't really matter what you put in the class definition. It can be strings, numbers, objects, variables. In Python 3, you can even pass named arguments.
Implementation
First you need to disappoint: in Python you cannot inherit from classes, i.e. You cannot pass a
type
parameter to a class constructor bases
that has nonclasses . However, it’s not specified anywhere what you will include in the class definition. There is nothing complicated in the implementation of the code above, for this, you just need to create a metaclass in which nonclasses from will be filtered out
bases
(which can be used further at your discretion). I think the example will explain everything, copy & run :Copy Source | Copy HTML- # coding: utf-8
-
- class Metaclass(type):
-
- '''<br/> Metaclass печатает все объекты, от которых наследует класс<br/> и которые не являеются классами.<br/> '''
-
- def __new__(cls, name, bases, dict):
- import inspect
-
- new_bases = []
- for base in bases:
- # отфильтруем классы,
- # остальное просто напечатаем
- if inspect.isclass(base):
- new_bases.append(base)
- else:
- print base
-
- return type.__new__(cls, name, tuple(new_bases), dict)
-
-
- class Print(object):
-
- __metaclass__ = Metaclass
-
-
- class String(Print, 'Programming', 'is', 'all', 'about', 'architecture.', ''):
- pass
-
- class Numbers(Print, 0, 1, 2.718, 3.1459, ''):
- pass
-
- class More(Print, None, True, 'or', False, ['to be', 'or', 'not to be'], ''):
- pass
-
- the_end = 'The end.'
- class End(Print, the_end):
- pass
Using named arguments in class definition in third Python, copy & run :
Copy Source | Copy HTML- # Python 3+
-
- def metaclass(name, bases, dict, private=True):
- print('Private:', private)
- return type(name, bases, dict)
-
-
- class MyClass(object, metaclass=metaclass, private=False):
-
- pass
Practical expediency
In most cases, this feature is not required at all. Moreover, the code becomes complex and “magical”, which is not at all welcome. However, in some cases, it is indispensable, as are metaclasses in general. In fact, two advantages can be distinguished:
- Metaclass Management and
- Simplification of the API.
Management of the construction of classes (and generally metaclasses)
Passing parameters for the metaclass directly in the class definition. Of course, you can pass the same parameters through the attributes of a regular class, but it clogs the class and does not always look readable. For example, let it be necessary to inherit either a full glass or an empty one (the example is dragged by the ears, I didn’t come up with a simple and understandable one, but I couldn’t get it from the finished code). Copy & run :
Copy Source | Copy HTML- #coding: utf-8
-
- class EmptyGlass: pass
- class FullGlass: pass
-
-
- class GlassMetaclass(type):
-
- '''<br/> GlassMetaclass создает либо класс EmptyGlass, либо FullGlass,<br/> в зависимости от того, было ли указано True или False в определении<br/> класса.<br/> '''
-
- empty_glass = EmptyGlass
- full_glass = FullGlass
-
- def __new__(cls, name, bases, dict):
- if bases[-1] is True: # is True, потому что нам надо именно объект True
- # полный бокал
- bases = [cls.full_glass] + list(bases[:-1])
- elif bases[-1] is False:
- # пустой
- bases = [cls.empty_glass] + list(bases[:-1])
-
- return super(GlassMetaclass, cls).__new__(cls, name, tuple(bases), dict)
-
-
- class Glass(object):
-
- __metaclass__ = GlassMetaclass
-
-
- if __name__ == '__main__':
- class MyGlass(Glass, True): pass # полный стакан
- class YourGlass(Glass, False): pass # пустой стакан
-
- print issubclass(MyGlass, FullGlass) # True
- print issubclass(YourGlass, EmptyGlass) # True
API simplification
Suppose we need to create a tree from classes (not a data structure, but just a hierarchy). Let it be hierarchical regular expressions. In this case, each class needs to pass a string that will be compiled into a regular expression. In the classic form, it will look something like this:
Copy Source | Copy HTML- class Music(MyRe):
-
- pattern = '/music'
-
- class Artist(MyRe):
-
- pattern = '/artist/\d+'
-
- class Song(MyRe):
-
- pattern = '/song/\d+'
-
- class Album(MyRe):
-
- pattern = '/album/\d+'
Including strings directly in the class definition allows you to get more beautiful and understandable code:
Copy Source | Copy HTML- class Music(MyRe, '/music'):
-
- class Artist(MyRe, '/artist/\d+'): pass
- class Song(MyRe, '/song/\d+'): pass
- class Album(MyRe, '/album/\d+'): pass
Conclusion
Passing parameters to metaclasses in class definitions, on the one hand, complicates the implementation of the program code, on the other hand, allows you to build a more humane interface and continue to focus on solving problems. In other words, be one step closer to DSL.
Further Reading on Metaclasses
- White papers, Customizing class creation. docs.python.org/3.1/reference/datamodel.html#customizing-class-creation
- Unifying types and classes in Python 2.2 #Metaclasses, Guido van Rossum. www.python.org/download/releases/2.2/descrintro/#metaclasses
- PEP-3115, Metaclasses in Python 3000. www.python.org/dev/peps/pep-3115
- Python Metaclasess: Who? Why? When ?, Mike C. Fletcher at PyCon 2004, presentation. www.vrplumber.com/programming/metaclasses-pycon.pdf