Organization of automated GUI testing
During development, we try to cover our code not only with obscenities, but also with unit tests. However, covering everything with tests does not always work. In addition, there remains a GUI for which writing tests is a rather laborious job. For all these problems, the requirement remains unchanged that each subsequent Kamit to the repository should not spoil the existing functionality.
In this article I want to tell what tools we use to test our desktop applications written in Qt.
Half a year ago, while researching GUI testing tools, froglogic 's Squish came into my view . Of the advantages of this solution, the following can be noted:
On the other side of the scale lay the cost of the license 93,000 rubles. (this is at the August exchange rate of 2650 euros).
Since none of the competitors offered such goodies for the money, and I did not find similar free solutions, reluctantly I bought a license.
So, with the background finished, let's move on to creating tests. For demonstration, I chose one of our public projects - MDC . The task is simple, check whether MDC can connect to ICQ, Gtalk, mail.ru. The topic is especially relevant in the light of the latest surprises of AOL :). The appearance of the contact sheet window will be considered a sign of connection.
Thus, we got the text of the test, which we will use as a basis.
Using this text without changes is certainly possible and for a wide range of tasks this is quite enough, which makes it possible to work with Squish not only to developers, but also to less qualified employees, but this code is not suitable for our task.
Squish supports 2 types of object naming: symbolic name and real Name. When automatically creating test text, Squish uses a symbolic name, which is not always convenient. So, for example, the contact sheet window is automatically defined as
symbolic name: MDC v1.0.3.1.nightly_CContactListWidget
where MDC v1.0.3.1.nightly is the title of the window, and CContactListWidget is the type of this widget. There is a version in the window title that will quite obviously change and the object set in this way will never be found. In this case, use real name: {name = 'contactList' type = 'CContactListWidget' visible = '1'}. Where name is set as a constant in MDC code via setObjectName
Thus, regardless of the window title, we can always find the widget we need and the final code of our test will look like this:
It is easy to make a test script for connecting to jabber and icq from it, replacing the login, password and click coordinates. The video below shows the launch of a test suit, consisting of three test cases (icq, mail.ru, jabber)
After that, this test suit can be made to run after unit tests have been completed with each successive cam so that you always have such a beautiful picture on hand: But this is another story, which, if it is interesting, I will write with pleasure. In this article, I only briefly touched on the functionality of Squish deliberately without touching on issues such as synchronization primitives, working with Qt objects through the API. I plan to write about these things in the following posts.
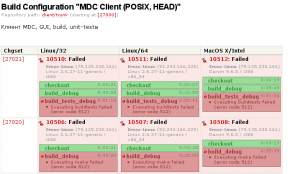
In this article I want to tell what tools we use to test our desktop applications written in Qt.
Half a year ago, while researching GUI testing tools, froglogic 's Squish came into my view . Of the advantages of this solution, the following can be noted:
- Squish's close friendship with Qt classes (including items in QGraphiscScene);
- cross-platform;
- support for scripting languages (JavaScript, Python);
- automated test text generation;
- convenient system for running tests from the console.
On the other side of the scale lay the cost of the license 93,000 rubles. (this is at the August exchange rate of 2650 euros).
Since none of the competitors offered such goodies for the money, and I did not find similar free solutions, reluctantly I bought a license.
So, with the background finished, let's move on to creating tests. For demonstration, I chose one of our public projects - MDC . The task is simple, check whether MDC can connect to ICQ, Gtalk, mail.ru. The topic is especially relevant in the light of the latest surprises of AOL :). The appearance of the contact sheet window will be considered a sign of connection.
- We collect Squish from sources with the same Qt version with which MDC will be compiled.
- We collect MDC debug mode (c release Squish cannot work).
- Launch Squish.
- Create a Test Suit for MDC.
- Choose the language in which we will write the tests.
- We configure application launch parameters (command line parameters, environment variables, etc.).
- We create a new test case in record mode (Squish starts MDC and remembers in a script all the actions that you will do with the application).
- We check his work.
Thus, we got the text of the test, which we will use as a basis.
- def main():
- waitForObject(":_QWidget")
- sendEvent("QResizeEvent", ":_QWidget", 22, 22, 769, 474)
- waitForObject(":_QGraphicsItem")
- mouseClick(":_QGraphicsItem", 221, 193, 1, Qt.LeftButton)
- waitForObject(":_QLineEdit")
- dragItemBy(":_QLineEdit", 153, -191, 26, 198, 1, Qt.LeftButton)
- waitForObject(":_QLineEdit")
- sendEvent("QMouseEvent", ":_QLineEdit", QEvent.MouseButtonRelease, 179, 7, Qt.LeftButton, 1)
- waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "
") - waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "squish@mail.ru")
- waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "
") - waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "1234")
- waitForObject(":MDC: Авторизация_CStartUpWidget")
- sendEvent("QMoveEvent", ":MDC: Авторизация_CStartUpWidget", 577, 70, 734, 62)
- mouseClick(":MDC: Авторизация_QWidget", 66, 372, 1, Qt.LeftButton)
- waitForObject(":MDC v1.0.3.1.nightly_CContactListWidget")
* This source code was highlighted with Source Code Highlighter.
Using this text without changes is certainly possible and for a wide range of tasks this is quite enough, which makes it possible to work with Squish not only to developers, but also to less qualified employees, but this code is not suitable for our task.
Squish supports 2 types of object naming: symbolic name and real Name. When automatically creating test text, Squish uses a symbolic name, which is not always convenient. So, for example, the contact sheet window is automatically defined as
symbolic name: MDC v1.0.3.1.nightly_CContactListWidget
where MDC v1.0.3.1.nightly is the title of the window, and CContactListWidget is the type of this widget. There is a version in the window title that will quite obviously change and the object set in this way will never be found. In this case, use real name: {name = 'contactList' type = 'CContactListWidget' visible = '1'}. Where name is set as a constant in MDC code via setObjectName
- m_widget = new CContactListWidget(this_ptr);
- m_widget->setObjectName("contactList");
* This source code was highlighted with Source Code Highlighter.
Thus, regardless of the window title, we can always find the widget we need and the final code of our test will look like this:
- def main():
- waitForObject(":_QWidget")
- sendEvent("QResizeEvent", ":_QWidget", 22, 22, 629, 418)
- waitForObject(":_QLineEdit")
- dragItemBy(":_QLineEdit", 140, -183, 26, 198, 1, Qt.LeftButton)
- waitForObject(":_QLineEdit")
- sendEvent("QMouseEvent", ":_QLineEdit", QEvent.MouseButtonRelease, 166, 15, Qt.LeftButton, 1)
- waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "
") - waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "squish@mail.ru")
- waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "
") - waitForObject(":MDC: Авторизация_QGraphicsView")
- type(":MDC: Авторизация_QGraphicsView", "1234")
- mouseClick(":MDC: Авторизация_QWidget", 154, 369, 1, Qt.LeftButton)
- waitForObject(":{name='contactList' type='CContactListWidget' visible='1'}")
* This source code was highlighted with Source Code Highlighter.
It is easy to make a test script for connecting to jabber and icq from it, replacing the login, password and click coordinates. The video below shows the launch of a test suit, consisting of three test cases (icq, mail.ru, jabber)
After that, this test suit can be made to run after unit tests have been completed with each successive cam so that you always have such a beautiful picture on hand: But this is another story, which, if it is interesting, I will write with pleasure. In this article, I only briefly touched on the functionality of Squish deliberately without touching on issues such as synchronization primitives, working with Qt objects through the API. I plan to write about these things in the following posts.
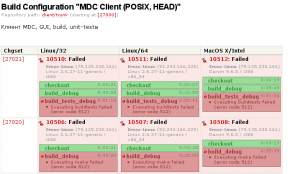