
Exchange data between React components using the RxJS library
- Transfer
Here is a translation of an article by Chidume Nnamdi published on blog.bitsrc.io. The translation is published with permission of the author.

The advent of the RxJS library has opened up a ton of new possibilities in the JS world. The goal of RxJS is to achieve a lot with a small amount of code. After reading this article, you will learn how to exchange data between application components on React, using the capabilities of RxJS.
Tip : Use Bit to organize and share React components. This will allow your team to develop their applications faster. Just give it a try.

React Components Collection
The exchange of data between unrelated React components is what the state libraries were created for. There are many state management templates, but two are best known: Flux and Redux.
Redux is popular because of its simplicity and its use of pure features. Indeed, thanks to them, there is no doubt that the use of reducers will not lead to any side effects.
When working with Redux, the first thing we do is create a centralized data warehouse:

Next, we associate the components with this repository and, if desired, update or delete the state. Any changes made to the vault will be reflected in the components associated with it. Thus, the data flow extends to all components, regardless of their degree of nesting. A component located at the nth level of the hierarchical structure is capable of transmitting data to a component of the highest level. The latter, in turn, can transmit data to the component 21 level.
With the advent of RxJS, using state management libraries has become much easier. Many liked the Observer pattern provided by RxJS.
We simply create a stream
We create the application on React, using
Next, we generate the project in React:
Go to the directory:
Install the rxjs library:
We should have a file that creates a new instance
Thus

The src folder contains the file
Suppose we have the following components:
In a hierarchical structure, they look like this:

The application component sends a message to ProducerA and ConsumerB. ProducerA sends the data to ConsumerA, and the message from ConsumerB gets to ProducerB.

The ConsumerA and ConsumerB components have an individual status counter. In their method,
ProducerA and ProducerB have
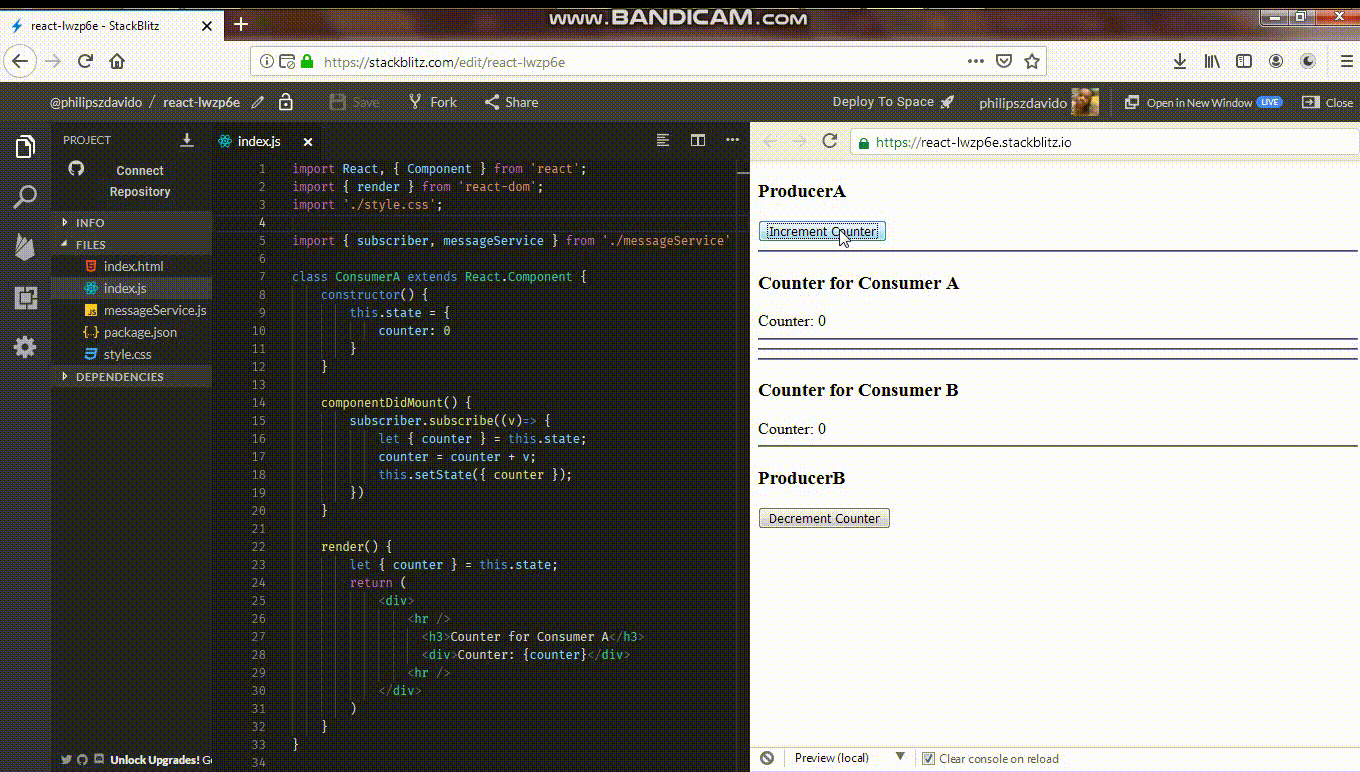
Let's look at the hierarchical structure again:

ProducerB transmits ConsumerA data, although it is completely unrelated. ProducerA transfers data to ConsumerB, not being its parent component. That's the whole point of RxJS: we just created a central event flow node and let the components listen on it. When any component generates events, listening components immediately pick them up.
You can play with the application on stackblitz: https://react-lwzp6e.stackblitz.io
So, we saw how you can exchange data between React components using RxJS. We used
If you have questions regarding this topic or you want me to add, correct or delete something, write about it in the comments, in the email or in a personal message.
Thanks for attention!

The advent of the RxJS library has opened up a ton of new possibilities in the JS world. The goal of RxJS is to achieve a lot with a small amount of code. After reading this article, you will learn how to exchange data between application components on React, using the capabilities of RxJS.
Tip : Use Bit to organize and share React components. This will allow your team to develop their applications faster. Just give it a try.

React Components Collection
Redux
The exchange of data between unrelated React components is what the state libraries were created for. There are many state management templates, but two are best known: Flux and Redux.
Redux is popular because of its simplicity and its use of pure features. Indeed, thanks to them, there is no doubt that the use of reducers will not lead to any side effects.
When working with Redux, the first thing we do is create a centralized data warehouse:

Next, we associate the components with this repository and, if desired, update or delete the state. Any changes made to the vault will be reflected in the components associated with it. Thus, the data flow extends to all components, regardless of their degree of nesting. A component located at the nth level of the hierarchical structure is capable of transmitting data to a component of the highest level. The latter, in turn, can transmit data to the component 21 level.
Rxjs
With the advent of RxJS, using state management libraries has become much easier. Many liked the Observer pattern provided by RxJS.
We simply create a stream
Observable
and enable all components to listen to it. If some component is added to the stream, listener (or “signed”) components respond to the DOM update.Installation
We create the application on React, using
create-react-app
. If you don’t have one create-react-app
, then install it globally first:npm i create-react-app -g
Next, we generate the project in React:
create-react-app react-prj
Go to the directory:
cd react-prj
Install the rxjs library:
npm i rxjs
We should have a file that creates a new instance
BehaviourSubject
.Why do we use BehaviorSubject?
BehaviorSubject
Is one of the Subject in the RxJS library. Being a child component of Subject, BehaviorSubject
it allows many observers to listen to the stream, and also makes mass broadcasting of events to these observers. BehaviorSubject
saves the last value and passes it to all new signed components. Thus
BehaviorSubject
:- Allows bulk mailing.
- It stores the latest values published by subscribers, and mass-mails these values.

The src folder contains the file
messageService.js
that exports the instance BehaviorSubject
and object to the subscriber messageService
. The subscriber object is created at the beginning of the file - so it is available for any importing component. The object messageService
has a send function that takes a parameter msg
: it contains the data that is needed for transmission to all listening components. In the body of the function, we call the method emit
. It bulk-mails data to the signed components in the subscribing object. Suppose we have the following components:
- ConsumerA;
- ConsumerB;
- ProducerA;
- ProducerB.
In a hierarchical structure, they look like this:

The application component sends a message to ProducerA and ConsumerB. ProducerA sends the data to ConsumerA, and the message from ConsumerB gets to ProducerB.

The ConsumerA and ConsumerB components have an individual status counter. In their method,
componentDidMount
they are subscribed to the same thread subscriber
. As soon as an event is published, the counter is updated for both components. ProducerA and ProducerB have
Increment Counter
and buttons Decrement Counter
that, when pressed, display 1
or -1
. The signed components ConsumerA and ConsumerB pick up the event and launch their callback functions, updating the value of the status counter and DOM. 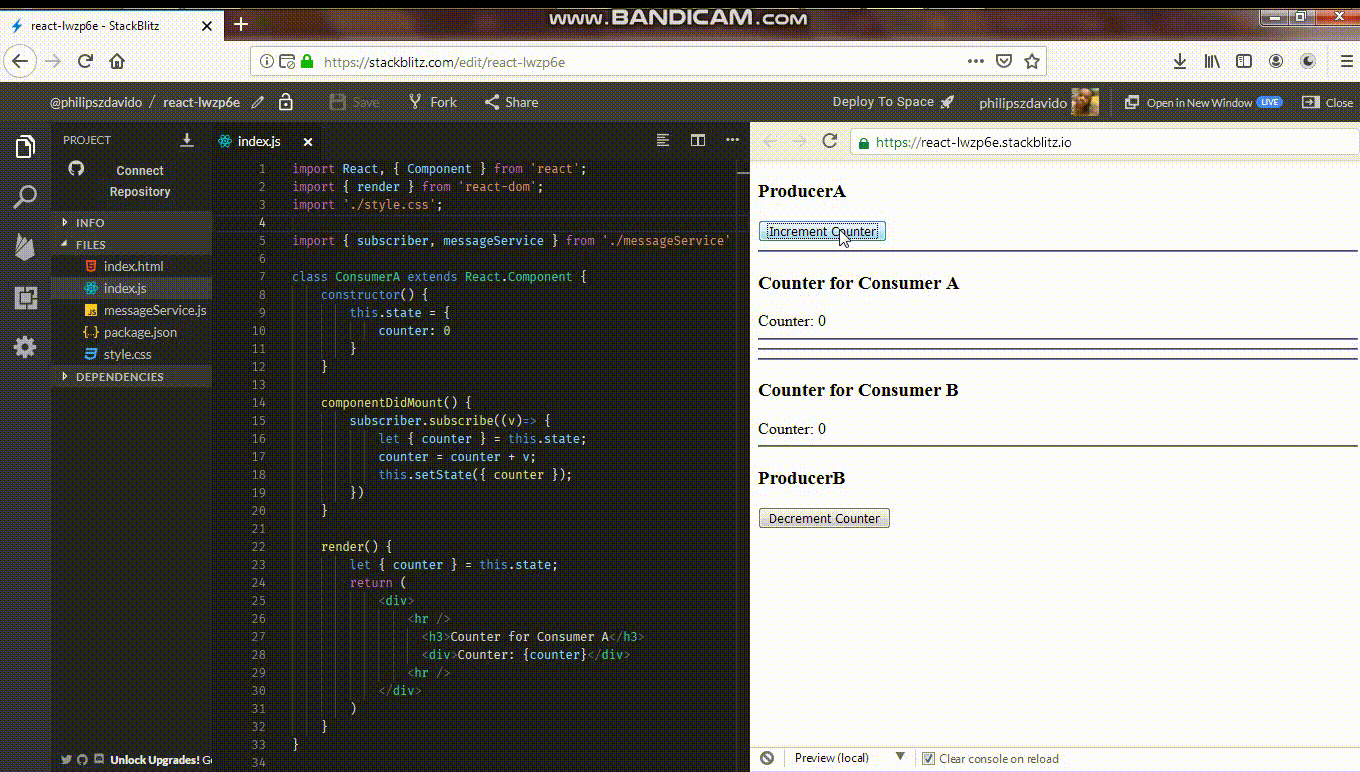
Let's look at the hierarchical structure again:

ProducerB transmits ConsumerA data, although it is completely unrelated. ProducerA transfers data to ConsumerB, not being its parent component. That's the whole point of RxJS: we just created a central event flow node and let the components listen on it. When any component generates events, listening components immediately pick them up.
You can play with the application on stackblitz: https://react-lwzp6e.stackblitz.io
Conclusion
So, we saw how you can exchange data between React components using RxJS. We used
BehaviourSubject
to create a centralized data stream, and then allowed the rest of the components to subscribe to this stream. Now, when one of the components generates data, the other components also receive them. The level of components in the hierarchical structure is unimportant. If you have questions regarding this topic or you want me to add, correct or delete something, write about it in the comments, in the email or in a personal message.
Thanks for attention!