
In-App Updates: Speeding Up Android Application Updates

Among the variety of tools announced on Android Dev Summit , I would like to pay special attention to the In-App Updates (IAUs) application update mechanism, which helps developers speed up the addition of new features, bug fixes and performance improvements. Since this functionality was published after Google I / O 2019, in this article I will talk in detail about IAUs, describe the recommended implementation schemes and give some code examples. I will also talk about our experience integrating IAUs into Pandao , an application for ordering goods from China.
The new API allows developers to initiate an application update to the latest version available on Google Play. In this way, IAUs complement the existing Google Play automatic update mechanism. IAUs contain several implementation schemes that are fundamentally different in terms of user interaction.
- Flexible Flow offers users to download the update in the background and install it at a time convenient for the user. It is intended for cases when users can still use the old version, but a new one is already available.
- Immediate Flow requires users to download and install the update before continuing to use the application. It is intended for cases where it is critical for developers to update the application.
Since the second option is not so important and less suitable for the Pandao application, we will analyze the Flexible Flow scenario in more detail.
IAUs Flexible Flow Integration
Use cases
The upgrade process using IAUs consists of several steps.
- An application using the Play Core library, which checks on Google Play whether there are any updates available.
- If they are, then the application asks Google Play to show IAUs dialogue. Google Play shows the user a dialog with a proposal to update.
- If the user agrees, Google Play in the background downloads the update, showing the user in the status bar the download progress.
- If the download is completed when the application is running in the background, Google Play will automatically complete the installation. If the application is active at this moment, then for such cases it is necessary to determine your own logic of installation completion. Consider the following scenarios.
- The application starts the installation process by showing the user a Google Play dialog with a progress indicator. After installation is complete, an updated version of the application is launched. In this case, it is recommended to display an additional dialog that allows the user to confirm that he is ready to restart the application now. This is the recommended implementation scheme .
- The application waits until it is in the background, and then completes the update. On the one hand, this is a less intrusive behavior from the point of view of UX, since user interaction with the application is not interrupted. But on the other hand, it requires the developer to implement the logic to determine if the application is in the background.
- The application starts the installation process by showing the user a Google Play dialog with a progress indicator. After installation is complete, an updated version of the application is launched. In this case, it is recommended to display an additional dialog that allows the user to confirm that he is ready to restart the application now. This is the recommended implementation scheme .
If the installation of the downloaded update was not completed, then Google Play may complete the installation in the background. This option is better not to use explicitly, because it does not guarantee the installation of the update.
Basic Testing Requirements
To manually complete the entire update process on a test device, you must have at least two versions of the application with different assembly numbers: source and target .
- The original version with a higher number must be published on Google Play, it will be identified by Google Play as an available update. The target version with a lower build number and integrated IAUs must be installed on the device, we will update it. The bottom line is that when the application asks Google Play to check for an update, it will compare the assembly numbers of the installed and available version. So IAUs will only be launched if the build number on Google Play is higher than the current version on the device.
- The source and target versions must have the same package name and must be signed with the same release certificate .
- Android 5.0 (API level 21) or higher.
- Play Core library 1.5.0 or higher.
Code example
Here we look at a sample code for using IAUs Flexible Flow, which can also be found in the official documentation . First you need to add the Play Core library to the build.gradle file at the module level.
dependencies {
...
implementation "com.google.android.play:core:1.5.0"
}
Then we create an instance
AppUpdateManager
and add a callback function to AppUpdateInfo
, in which information about the availability of the update, the object to start the update (if available) and the current download progress, if it has already begun, will be returned.// Create instance of the IAUs manager.
val appUpdateManager = AppUpdateManagerFactory.create(context)
// Add state listener to app update info task.
appUpdateManager.appUpdateInfo.addOnSuccessListener { appUpdateInfo ->
// If there is an update available, prepare to promote it.
if (appUpdateInfo.updateAvailability() == UpdateAvailability.UPDATE_AVAILABLE) {
// ...
}
// If the process of downloading is finished, start the completion flow.
if (appUpdateInfo.installStatus() == InstallStatus.DOWNLOADED) {
// ...
}
}
.addOnFailureListener { e ->
// Handle the error.
}
To show the dialog for requesting updates from Google Play, you must pass the received object
AppUpdateInfo
to the method startIntentSenderForResult
. appUpdateManager.startUpdateFlowForResult(
// Pass the intent that is returned by 'getAppUpdateInfo()'.
appUpdateInfo,
// Or 'AppUpdateType.IMMEDIATE for immediate updates.
AppUpdateType.FLEXIBLE,
// The current activity.
activity,
REQUEST_CODE
)
You can add an event listener to the IAUs manager to track update status
InstallStateUpdatedListener
.// Create a listener to track downloading state updates.
val listener = InstallStateUpdatedListener { state ->
// Update progress indicator, request user to approve app reload, etc.
}
// At some point before starting an update, register a listener for updates.
appUpdateManager.registerListener(listener)
// ...
// At some point when status updates are no longer needed, unregister the listener.
appUpdateManager.unregisterListener(listener)
Once the update is downloaded (status
DOWNLOADED
), you need to restart the application to complete the update. You can initiate a restart with a call appUpdateManager.completeUpdate()
, but before that it is recommended to show a dialog box so that the user clearly confirms his readiness to restart the application.Snackbar.make(
rootView,
"An update has just been downloaded from Google Play",
Snackbar.LENGTH_INDEFINITE
).apply {
setAction("RELOAD") { appUpdateManager.completeUpdate() }
show()
}
Error "Update is Not Available"
First, recheck compliance with the requirements listed in the Basic Implementation Requirements section. If you have done everything, but the update according to the call
onSuccess
is still not available, then the problem may be in caching. It is likely that the Google Play application is not aware of the available update due to the internal caching mechanism. To avoid this during manual testing, you can force a cache reset by going to the "My apps and games" page on Google Play. Or you can just clear the cache in the settings of the Google Play application. Please note that this problem only occurs during testing, it should not affect end users, since their cache is still updated daily.IAUs Flexible Flow in Pandao app
We participated in an early access program and integrated IAUs Flexible Flow (recommended implementation) into the Pandao application, a platform on which manufacturers and vendors can trade Chinese goods. The IAUs dialog was displayed on the main screen so that the maximum number of users could interact with it. Initially, we wanted to show the dialogue no more than once a day, so as not to distract people from interacting with the application.
Since A / B testing plays a key role in the life cycle of any new feature, we decided to evaluate the effect of IAUs in our application. We randomly divided users into two disjoint groups. The first was a control one, without using IAUs, and the second group was a test one, we showed IAUs dialogue to these users.

IAUs Flexible Flow A / B test in Pandao app.
Over the past few releases, we have measured the percentage of active users of each version of the application. It turned out that among the active users with the latest version available at that time, the main part was made up of participants from group B, that is, with the IAU function. The purple line in the graph shows that in the first days after the publication of version 1.29.1, the number of active users with IAUs exceeded the number of users without this function. Therefore, it can be argued that users with IAUs update the application faster.
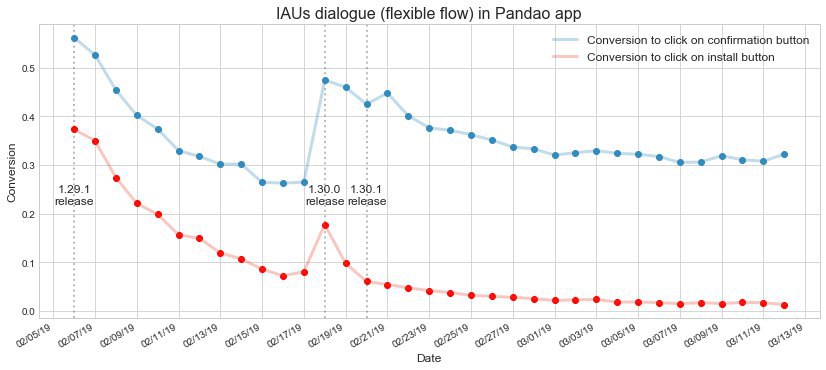
The IAUs Flexible Flow dialog in the Pandao app.
According to our data (see the graph above), users most often click on the confirmation button in the IAUs dialog in the first days after the release, and then the conversion is constantly reduced until the publication of the next version of the application. The same thing is observed with the install button in the dialog box, which initiates the installation of the downloaded update. Therefore, we can say that the average conversion value in both cases is directly proportional to the frequency of releases. In Pandao, the average conversion within one month reaches 35% for a click on the confirmation button and 7% for a click on the install button.
We assume that a decrease in the percentage of confirmations over time is only a user experience problem, because people who are interested in the new version will update quite quickly, and those who are not interested in updating will not be interested. Based on this assumption, we decided not to disturb those who are not interested in updating, and not to ask them every day. It’s good practice to use a different query logic, which is based on “obsolescence,” that is, in order not to bother users, we evaluate how old versions they have and how often we have already suggested that they update.
In general, IAUs showed good results during A / B testing, so we rolled out IAUs for all users.
Acknowledgments
Thank you for your help in writing this article Marina Pleshkova Maryna_Pliashkova , Alexander Cherny alexchernyy , Ilya Nazarov RolaRko , Gleb Bodyachevsky, Daniil Polozov jokerdab , Anastasia Kulik, Vladislav Breus and Vladislav Goldin Vladiskus .