Writing a telegram bot in python using the telebot library part 1
Attention, the second part of the article has been published , I recommend reading it
Lyrical digression
Bots today is a multifunctional tool, both for business and for entertainment. Personally, I believe that the future lies with them. Of course, after reading this article, you will not be able to create large projects, but to get interested and start is quite.
Installation and setup
To get started, let's download python itself. You can do this on the official website . Do not forget to check add to PATH during installation! After installing python, we need a good code editor. JetBrains comes to the rescue with its free PyCharm . We are close, it remains to download the telebot library. To do this, go to the command line and write:
pip install pytelegrambotapi
If everything went well, we can continue!
VPN
I think everyone knows about telegram blocking in Russia and the only solution, as always, is vpn. Personally, I recommend Windscribe , as they give you 2 GB. traffic is completely free!
Bot father
In the search for telegram we find Bot Farher and create our bot using the / newbot command. Then enter the name and username. Please note that the username must end in bot!

As you see, we were given a special api token with which you can control your bot (in my case, it is: 776550937: AAELEr0c3H6dM-9QnlDD-0Q0Fcd65pPyAiM ). You can remember your token, but I recommend writing it down.
The code
The moment has come that everyone has been waiting for. Open PyCharm and create a new project.

Here I recommend putting everything as mine (the name, of course, can be changed). After creating the project, let's create a file in which our code will be. Right-click on the folder with your project, then New → Python File. Ok, let's start writing code. Import the telebot library using:
import telebot
Now we need to create the bot variable. Actually, the variable name can be anything, but I'm used to writing bot.
bot = telebot.TeleBot('ваш токен')
We will write the bot .message_handler () decorator , with which our bot will respond to the / start command. To do this, we write commands = ['start'] in parentheses. As a result, we should get this:
@bot.message_handler(commands=['start'])
If you try to launch your bot (RMB-> Run), then nothing will work for you. First, at the end of the code, we need to write bot.polling (). This is necessary so that the bot does not turn off immediately, but works and checks to see if there is a new message on the server. And secondly, our bot, even if it checks for messages, will still not be able to respond. It's time to fix it! After our decorator, we create the start_message function, which will accept the message parameter (the name of the function can be anything). Next, let's implement sending messages from the bot itself. In the function, we write bot.send_message (message.chat.id, 'Hello, you wrote me / start'). See what you should get:
import telebot
bot = telebot.TeleBot('776550937:AAELEr0c3H6dM-9QnlDD-0Q0Fcd65pPyAiM')
@bot.message_handler(commands=['start'])
def start_message(message):
bot.send_message(message.chat.id, 'Привет, ты написал мне /start')
bot.polling()
Let's check ...
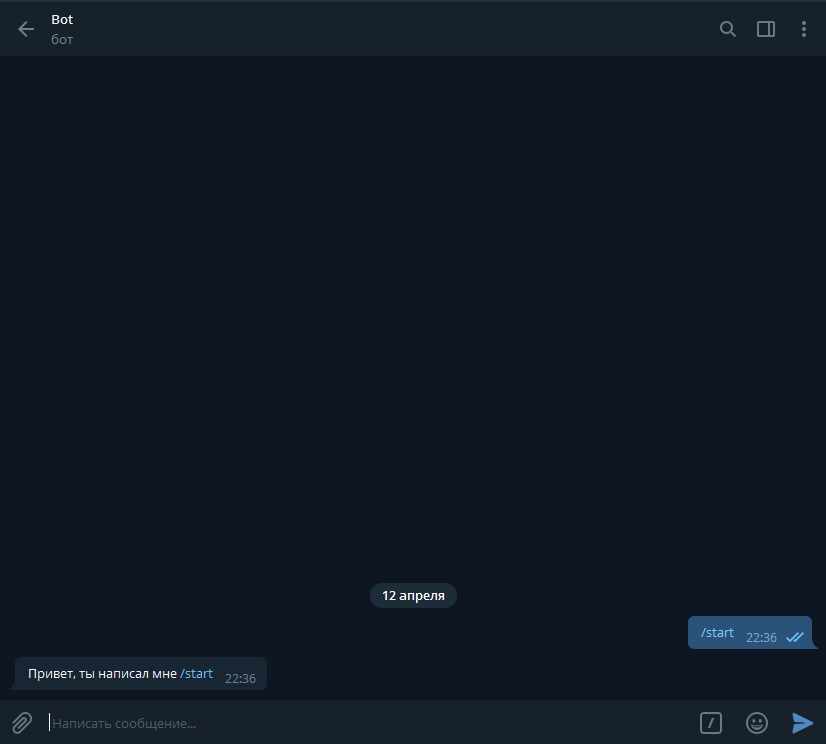
Great, our bot works! To make it respond not only to commands, but also to messages, create a new decorator bot .message_handler (), and write content_types = ['text'] in parentheses. In general, there are many types of content, for example location, photo, audio, sticker, etc. But we need to respond to the text, right? Therefore, we create a send_text function that takes a message parameter. In the function, we write the condition:
@bot.message_handler(content_types=['text'])
def send_text(message):
if message.text == 'Привет':
bot.send_message(message.chat.id, 'Привет, мой создатель')
elif message.text == 'Пока':
bot.send_message(message.chat.id, 'Прощай, создатель')
If the message text is “Hello”, then the bot answers “Hello, my creator”, and if the message text is “Bye”, the bot will answer “Goodbye, creator”. Here I think everything is clear. But you most likely wondered, and if the user writes “hello”, or “Hi”, what should be done in this situation? Everything is quite simple! In the condition, after the message.text write the function .lower (), and in the text replace all uppercase letters with lowercase. Now our bot responds not only to “hello”, but also to “PrivT”, and even “PRIVET”.
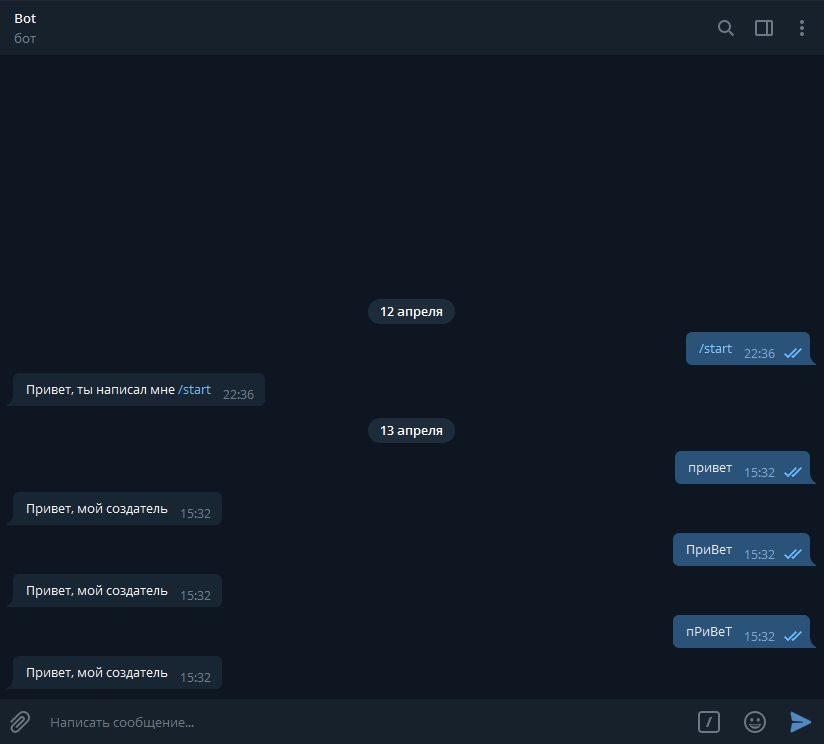
Here is what you should get:
import telebot
bot = telebot.TeleBot('776550937:AAELEr0c3H6dM-9QnlDD-0Q0Fcd65pPyAiM')
@bot.message_handler(commands=['start'])
def start_message(message):
bot.send_message(message.chat.id, 'Привет, ты написал мне /start')
@bot.message_handler(content_types=['text'])
def send_text(message):
if message.text.lower() == 'привет':
bot.send_message(message.chat.id, 'Привет, мой создатель')
elif message.text.lower() == 'пока':
bot.send_message(message.chat.id, 'Прощай, создатель')
bot.polling()
Well, we figured out the text, but how to send a sticker for example? Everything is simple! Each sticker has its own id; accordingly, knowing the id, we can send it. There are two ways to get the id of a sticker. The first (simple) - through a special bot “What's the sticker id?”

Well, the second way, for those who are not looking for easy ways. We create a new decorator bot .message_handler (), only in parentheses we write content_types = ['sticker']. Then everything is as usual. We create a function that takes the message parameter, but here we write print (message). We launch the bot.

Look, as soon as I sent the sticker, it immediately displayed information in the console, and at the very end will be our sticker id (file_id). Let's make sure that when the user sends the bot “I love you”, the bot responds with a sticker. There is no need to create a new decorator, we just add the condition that was before that. But instead of bot.send_message () we write bot.send_sticker (), and instead of the text we write the id of the sticker.
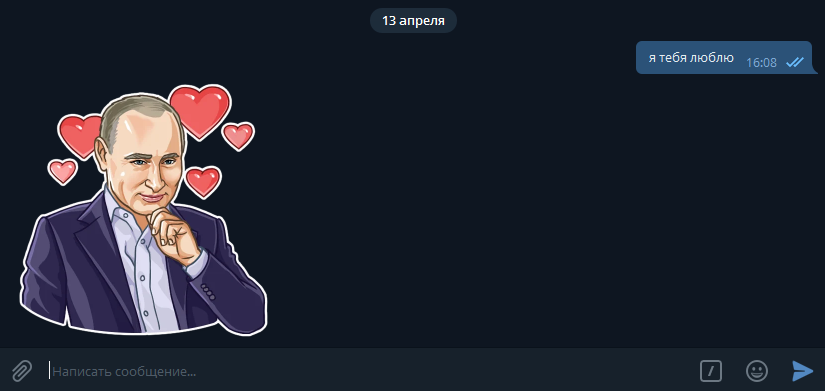
Congratulations, everything worked out! I’m thinking how to send audio, photos, and geolocation, you will figure it out for yourself. I want to show you how to make a keyboard that the bot will show you at startup. This will make it harder. We create the keyboard1 variable, in which we write telebot.types.ReplyKeyboardMarkup (). This function calls up the keyboard. Next, create the rows, but remember that there can be no more than 12 rows! In order to create them, write keyboard1.row (). In parentheses, write down whatever you want; personally, I will write “Hello” and “Bye.” Now, to call the keyboard, let's add reply_markup = keyboard1 to the function of sending a message at startup. Here is what you should get:
keyboard1 = telebot.types.ReplyKeyboardMarkup()
keyboard1.row('Привет', 'Пока')
@bot.message_handler(commands=['start'])
def start_message(message):
bot.send_message(message.chat.id, 'Привет, ты написал мне /start', reply_markup=keyboard1)
We launch the bot ...
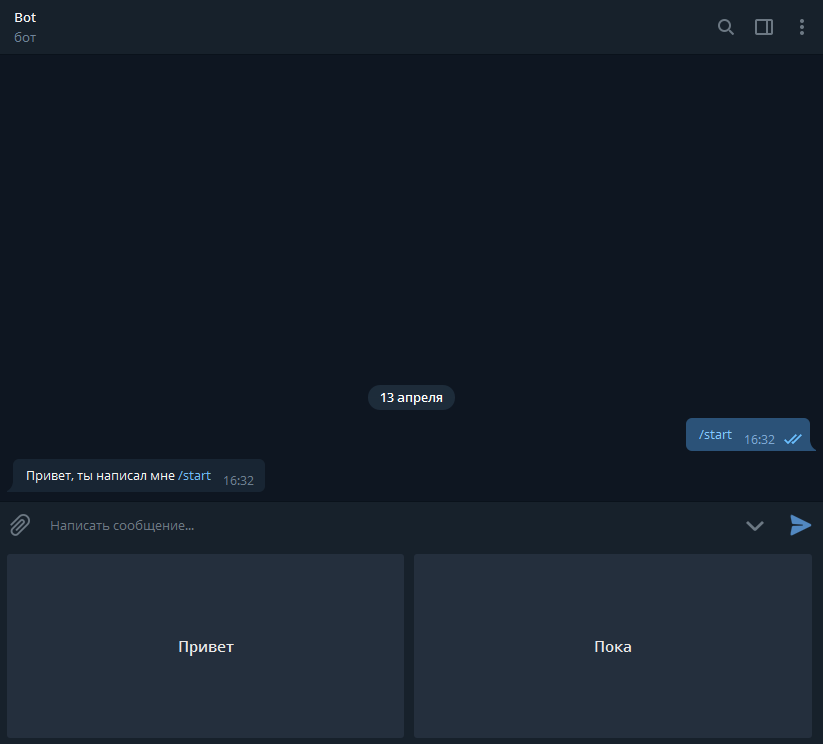
You see that the keyboard is somehow big. To fix this, you just need to register True in ReplyKeyboardMarkup (). Well, if you want the keyboard to hide as soon as the user clicks on it, then write another True. You can read more about what these True mean in the official documentation .
keyboard1 = telebot.types.ReplyKeyboardMarkup(True, True)
Well, that’s all! Of course, this is not all possible of bots in telegram, but I showed you the main features. Thanks for attention.
Source:
import telebot
bot = telebot.TeleBot('<ваш токен>')
keyboard1 = telebot.types.ReplyKeyboardMarkup()
keyboard1.row('Привет', 'Пока')
@bot.message_handler(commands=['start'])
def start_message(message):
bot.send_message(message.chat.id, 'Привет, ты написал мне /start', reply_markup=keyboard1)
@bot.message_handler(content_types=['text'])
def send_text(message):
if message.text.lower() == 'привет':
bot.send_message(message.chat.id, 'Привет, мой создатель')
elif message.text.lower() == 'пока':
bot.send_message(message.chat.id, 'Прощай, создатель')
elif message.text.lower() == 'я тебя люблю':
bot.send_sticker(message.chat.id, 'CAADAgADZgkAAnlc4gmfCor5YbYYRAI')
@bot.message_handler(content_types=['sticker'])
def sticker_id(message):
print(message)
bot.polling()
If you have any questions - you can write to telegram dimagorovtsov