
Commenting on the code: good, bad, evil
- Transfer
- Tutorial

You've probably heard that: “Good code is self-documenting . ”
I have been earning money for more than 20 years by writing code, and I heard this phrase most often. This is a cliche .
And as in many other cliches, there is a grain of truth. But this truth has already been abused so much that most of those who utter this phrase do not understand what it really means.
Is she true? Yes .
Does it mean you should never comment on the code? No .
In this article we will look at various aspects of commenting on code.
For beginners: There are two different kinds of comments. I call them documentary comments andexplanatory comments .
Documentation Comments
Documentation comments are intended for those who would rather use your code rather than read it. If you are creating a library or framework for other developers, then you will need something like API documentation.
The farther the API documentation is from your source code, the more likely it will become obsolete or incorrect over time. It’s best to embed the documentation directly into the code, and then extract it using some kind of tool.
Here is an example of a documentary comment from the popular Lodash JS library :
/**
* Creates an object composed of keys generated from the results of running
* each element of `collection` thru `iteratee`. The corresponding value of
* each key is the number of times the key was returned by `iteratee`. The
* iteratee is invoked with one argument: (value).
*
* @static
* @memberOf _
* @since 0.5.0
* @category Collection
* @param {Array|Object} collection The collection to iterate over.
* @param {Function} [iteratee=_.identity] The iteratee to transform keys.
* @returns {Object} Returns the composed aggregate object.
* @example
*
* _.countBy([6.1, 4.2, 6.3], Math.floor);
* // => { '4': 1, '6': 2 }
*
* // The `_.property` iteratee shorthand.
* _.countBy(['one', 'two', 'three'], 'length');
* // => { '3': 2, '5': 1 }
*/
var countBy = createAggregator(function(result, value, key) {
if (hasOwnProperty.call(result, key)) {
++result[key];
} else {
baseAssignValue(result, key, 1);
}
});
If you compare these comments with the online documentation of the library , you will see that everything is the same there.
When writing documentary comments, make sure that you do this in accordance with the appropriate standard, and that the comments can be easily distinguished from any inline explanatory comments that you can add as well. Some popular and well-supported standards and tools are: JSDoc for JavaScript, DocFx for .NET, JavaDoc for Java.
The disadvantages of documentary comments include the fact that they are capable of making a lot of noise, and it is more difficult for programmers who are actively involved in maintaining the code to read them. But on the other hand, most editors support "folding code blocks" (code folding), which allows you to hide comments and pay all attention only to the code.
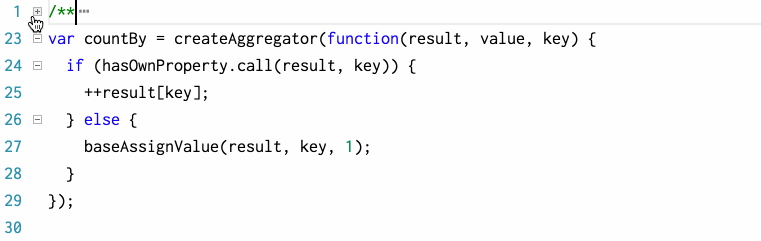
Collapse comments in Visual Studio code.
Explanatory Comments
Explanatory comments are intended for everyone (including yourself in the future) who will accompany, refactor, or extend the code.
Explanatory comments are often a sign of bad code. Their presence indicates the excessive complexity of the code base. Therefore, try to remove explanatory comments and simplify the code because “good code is self-documenting”.
Here's an example of a bad - albeit very funny - explanatory comment:
/*
* Replaces with spaces
* the braces in cases
* where braces in places
* cause stasis.
**/
$str = str_replace(array("\{","\}")," ",$str);
Instead of decorating the confusing expression with a clever rhyme - written by double-foot amphibrachium , no less - the author should spend time on a function that makes the code more readable and easier to understand. For example, you can make a function
removeCurlyBraces
called from a function sanitizeInput
. Do not get it wrong, there are situations - especially when working on a very difficult task - when the soul asks for a little humor. But if you write a funny comment balancing bad code, then hardly anyone will want to refactor or fix it later.
Do you really want to deprive other programmers of the pleasure of reading your witty little poem? Most of them will laugh and go about their business, ignoring the flaws of the code.
But there are situations when you come across an excessive comment. If the code is really simple and obvious, no need to add comments.
For example, do not do this:
/*
set the value of the age integer to 32
*/
int age = 32;
But it also happens: no matter what you do with the code, an explanatory comment is justified. This usually happens when you need to add some context to a non-obvious solution. Here is a good example from Lodash:
function addSetEntry(set, value) {
/*
Don't return `set.add` because it's not chainable in IE 11.
*/
set.add(value);
return set;
}
Or there are such situations: after much thought and experimentation, you realize that the solution, which seemed naive, is actually the best. In the future, other programmers will almost inevitably decide that they are smarter than you, and begin to remodel the code so that later they realize that your method turned out to be the best.
Sometimes you can be such a programmer yourself.
In such situations, it is better to save someone else's time and write a comment.
This stub comment perfectly illustrates what is described:
/**
Dear maintainer:
Once you are done trying to 'optimize' this routine,
and have realized what a terrible mistake that was,
please increment the following counter as a warning
to the next guy:
total_hours_wasted_here = 42
**/
Of course, this is more likely to entertain than help. But you SHOULD leave comments that caution others against looking for a seemingly “better solution” if you have already tried and rejected other options. In this case, the comment should describe what you tried to do and why you refused such decisions.
A simple example in JavaScript:
/*
don't use the global isFinite() because it returns true for null values
*/
Number.isFinite(value)
Evil
So, you read about the good and the bad, but what about the evil?
Unfortunately, in any profession you can feel disappointed, and when you write a code for the sake of earning, you may be tempted to express this disappointment in the comments.
If you work with a sufficient number of code bases, then you will come across comments from cynical and depressive to gloomy and vicious.
From seemingly harmless ...
/*
This code sucks, you know it and I know it.
Move on and call me an idiot later.
*/
... deliberately offensive
/*
Class used to workaround Richard being a f***ing idiot
*/
Such comments may seem funny, or temporarily help to reduce disappointment, but if they get into production, they discredit the professionalism of the author and his employer, put them in a bad light.
Do not do this.