React Tutorial, Part 6: Some Course, JSX, and JavaScript Features
- Transfer
- Tutorial
Today we publish the continuation of the training course on React. Here we will talk about some features of the course, concerning, in particular, the style of the code. Here we will talk more about the relationship between JSX and JavaScript.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: About some features of the course, JSX and JavaScript
→ Part 7: Inline Styles
→
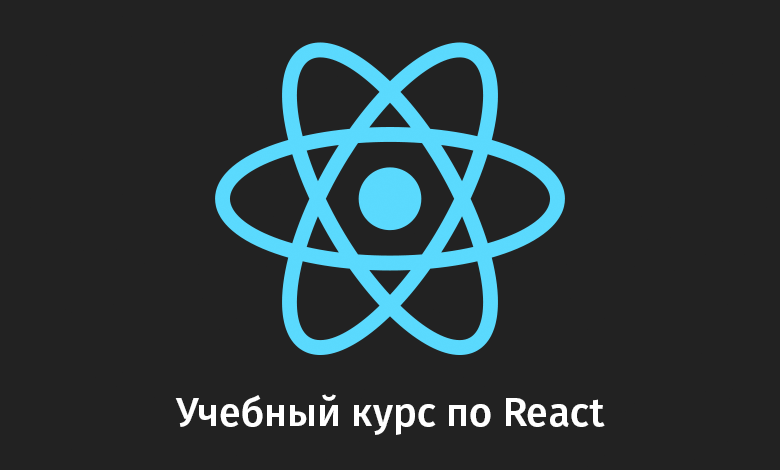
Part 8: continued work on a TODO application, familiarity with the properties of components
→ Part 9: properties of components
→ Part 10: a workshop on working with properties of components and styling
→ Part 11: dynamic formation of markup and the array method map
→ Part 12: workshop, third stage towards TODO-application
→ Part 13: components based on classes
→ Part 14: workshop on the components, based on classes state components
→ Part 15: workshops to work with components state
→ Part 16: fourth stage towards TODO-attached eat, event handling
→Part 17: fifth stage of work on the TODO application, modification of the state of the components
→ Part 18: sixth stage of work on the TODO application
→ Part 19: methods of the component life cycle
→ Part 20: first lesson on conditional rendering
→ Part 21: second lesson and workshop on conditional rendering
→ Part 22: the seventh stage of working on a TODO application, loading data from external sources
→ Part 23: the first session on working with forms
→ Part 24: the second session on working with forms
→ Part 25: a workshop on working with forms
→ Part 26: application architecture, n Container / Component
→Part 27: course project
→ Original
Before we continue classes, I would like to talk a little about some of the features of the code that I demonstrate in this course. You might have noticed that the code does not usually use semicolons. For example, as you can see, in examples like the following, they are not:
Perhaps you are used to putting semicolons wherever possible. Then, for example, the first two lines of the previous code snippet would look like this:
I recently decided that I would do without them, as a result I get the code that you see in the examples. Of course, in JavaScript there are constructions in which you cannot do without semicolons. Say, when describing a for loop , the syntax of which looks like this:
But in most cases you can do without semicolons at the end of lines. And their absence in the code will not disrupt its work. In fact, the question of using semicolons in code is a matter of personal preference for the programmer.
Another feature of the code that I write is that although ES6 technically allows the use of switch functions in cases where functions are declared using a keyword
For example, the code above can be rewritten as:
But I'm not used to this. I believe that the arrow functions are extremely useful in certain cases in which the features of these functions do not interfere with the correct operation of the code. For example, when anonymous functions are usually used, or when class methods are written. But I prefer to use traditional features. Many, when describing functional components, use the arrow functions. I agree that this approach has advantages over the use of traditional structures. However, I do not seek to impose any particular way of declaring functional components.
→ Original
In the following classes we will talk about the built-in styles. Before turning to these topics, we need to clarify some features of the interaction between JavaScript and JSX. You already know that, using the capabilities of React, we can, from regular JavaScript code, return constructs that resemble ordinary HTML markup, but are JSX code. This happens, for example, in the code of functional components.
What if there is some variable whose value needs to be substituted into the JSX-code returned by the functional component?
Suppose we have this code:
Add to the functional component a pair of variables containing the user's first and last name.
Now we want that what the functional component returns is not a first-level heading with text
Let's try to rewrite what the component returns, like this:
If you look at what appears on the page after processing such a code, it turns out that it does not look like we need. Namely, the page will get the text
Let us ask ourselves how to take advantage of JavaScript features in JSX code. In fact, it's pretty easy to do. In our case, it suffices to conclude what should be interpreted as JavaScript code, in curly braces. As a result, what the component returns will look like this:
At the same time the text gets to the page

The page, the markup of which is formed by JSX and JavaScript.
Since, when working with strings in modern conditions, ES6 capabilities are mainly used, we will rewrite the code with their use. Namely, we are talking about the template literals , issued using backward quotation marks (
Notice that the first and last names are separated by a space, which is interpreted here as an ordinary character. The result of the execution of this code will be the same as shown above. In general, the most important thing you need to understand now is that what is enclosed in braces that are in the JSX code is a regular JS.
Consider another example. Namely, we will rewrite our code so that, if it is called in the morning, it would output the text
A new instance of the object has been created here
There is a variable
As usual, it is recommended to experiment with what we have learned today.
In this lesson, we talked about some of the features of the code style used in this course, as well as the interaction between JSX and JavaScript. Using JavaScript-code in JSX opens up great opportunities, the practical usefulness of which we will feel in the next lesson, when we will deal with the built-in styles.
Dear readers! Do you use semicolons in your JavaScript code?

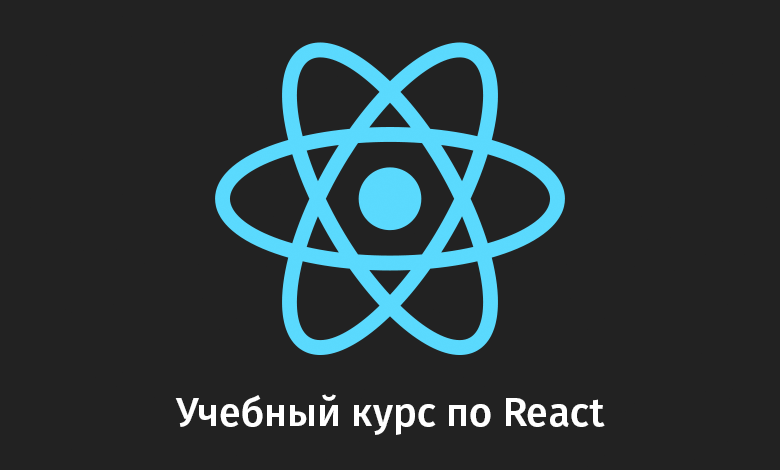
Part 8: continued work on a TODO application, familiarity with the properties of components
→ Part 9: properties of components
→ Part 10: a workshop on working with properties of components and styling
→ Part 11: dynamic formation of markup and the array method map
→ Part 12: workshop, third stage towards TODO-application
→ Part 13: components based on classes
→ Part 14: workshop on the components, based on classes state components
→ Part 15: workshops to work with components state
→ Part 16: fourth stage towards TODO-attached eat, event handling
→Part 17: fifth stage of work on the TODO application, modification of the state of the components
→ Part 18: sixth stage of work on the TODO application
→ Part 19: methods of the component life cycle
→ Part 20: first lesson on conditional rendering
→ Part 21: second lesson and workshop on conditional rendering
→ Part 22: the seventh stage of working on a TODO application, loading data from external sources
→ Part 23: the first session on working with forms
→ Part 24: the second session on working with forms
→ Part 25: a workshop on working with forms
→ Part 26: application architecture, n Container / Component
→Part 27: course project
Session 13. About some features of the course.
→ Original
Before we continue classes, I would like to talk a little about some of the features of the code that I demonstrate in this course. You might have noticed that the code does not usually use semicolons. For example, as you can see, in examples like the following, they are not:
import React from"react"import ReactDOM from"react-dom"functionApp() {
return (
<h1>Hello world!</h1>
)
}
ReactDOM.render(<App />, document.getElementById("root"))
Perhaps you are used to putting semicolons wherever possible. Then, for example, the first two lines of the previous code snippet would look like this:
import React from"react";
import ReactDOM from"react-dom";
I recently decided that I would do without them, as a result I get the code that you see in the examples. Of course, in JavaScript there are constructions in which you cannot do without semicolons. Say, when describing a for loop , the syntax of which looks like this:
for ([инициализация]; [условие]; [финальное выражение])выражение
But in most cases you can do without semicolons at the end of lines. And their absence in the code will not disrupt its work. In fact, the question of using semicolons in code is a matter of personal preference for the programmer.
Another feature of the code that I write is that although ES6 technically allows the use of switch functions in cases where functions are declared using a keyword
function
, I do not use it. For example, the code above can be rewritten as:
import React from"react"import ReactDOM from"react-dom"const App = () => <h1>Hello world!</h1>
ReactDOM.render(<App />, document.getElementById("root"))
But I'm not used to this. I believe that the arrow functions are extremely useful in certain cases in which the features of these functions do not interfere with the correct operation of the code. For example, when anonymous functions are usually used, or when class methods are written. But I prefer to use traditional features. Many, when describing functional components, use the arrow functions. I agree that this approach has advantages over the use of traditional structures. However, I do not seek to impose any particular way of declaring functional components.
Lesson 14: JSX and JavaScript
→ Original
In the following classes we will talk about the built-in styles. Before turning to these topics, we need to clarify some features of the interaction between JavaScript and JSX. You already know that, using the capabilities of React, we can, from regular JavaScript code, return constructs that resemble ordinary HTML markup, but are JSX code. This happens, for example, in the code of functional components.
What if there is some variable whose value needs to be substituted into the JSX-code returned by the functional component?
Suppose we have this code:
import React from"react"import ReactDOM from"react-dom"functionApp() {
return (
<h1>Hello world!</h1>
)
}
ReactDOM.render(<App />, document.getElementById("root"))
Add to the functional component a pair of variables containing the user's first and last name.
functionApp() {
const firstName = "Bob"
const lastName = "Ziroll"
return (
<h1>Hello world!</h1>
)
}
Now we want that what the functional component returns is not a first-level heading with text
Hello world!
, but a heading containing a type greeting Hello Bob Ziroll!
that is formed using the variables in the component. Let's try to rewrite what the component returns, like this:
<h1>Hello firstName + " " + lastName!</h1>
If you look at what appears on the page after processing such a code, it turns out that it does not look like we need. Namely, the page will get the text
Hello firstName + " " + lastName!
. In this case, if for example, run a standard project created tools create-react-app
, we were warned that the constants firstName
and lastName
values are assigned, which are not used anywhere. However, this does not prevent the appearance on the page of the text, which is exactly what was returned by the functional component, without substitution instead of what seemed to us the names of variables, their values. Names of variables in this form, the system considers plain text.Let us ask ourselves how to take advantage of JavaScript features in JSX code. In fact, it's pretty easy to do. In our case, it suffices to conclude what should be interpreted as JavaScript code, in curly braces. As a result, what the component returns will look like this:
<h1>Hello {firstName + " " + lastName}!</h1>
At the same time the text gets to the page
Hello Bob Ziroll!
. In these fragments of JSX-code, marked with curly brackets, you can use regular JavaScript-constructions. Here’s what the code displays in the browser:
The page, the markup of which is formed by JSX and JavaScript.
Since, when working with strings in modern conditions, ES6 capabilities are mainly used, we will rewrite the code with their use. Namely, we are talking about the template literals , issued using backward quotation marks (
` `
). Such strings may contain view constructs${выражение}
. The standard behavior of patterned literals involves evaluating the expressions contained in curly braces and converting what is produced into a string. In our case, it will look like this:<h1>Hello {`${firstName}${lastName}`}!</h1>
Notice that the first and last names are separated by a space, which is interpreted here as an ordinary character. The result of the execution of this code will be the same as shown above. In general, the most important thing you need to understand now is that what is enclosed in braces that are in the JSX code is a regular JS.
Consider another example. Namely, we will rewrite our code so that, if it is called in the morning, it would output the text
Good morning
, if in the afternoon - Good afternoon
, and if in the evening - Good night
. To begin, we will write a program that tells you what time it is. Here is the code of the functional component App
that solves this problem:functionApp() {
const date = newDate()
return (
<h1>It is currently about {date.getHours() % 12} o'clock!</h1>
)
}
A new instance of the object has been created here
Date
. JSX uses JavaScript-code, thanks to which we learn by calling the method date.getHours()
, which is now an hour, then, calculating the remainder of dividing this number by 12
, we reduce the time to a 12-hour format. Similarly, you can check the time, form the line we need. For example, it might look like this:functionApp() {
const date = newDate()
const hours = date.getHours()
let timeOfDay
if (hours < 12) {
timeOfDay = "morning"
} elseif (hours >= 12 && hours < 17) {
timeOfDay = "afternoon"
} else {
timeOfDay = "night"
}
return (
<h1>Good {timeOfDay}!</h1>
)
}
There is a variable
timeOfDay
, and analyzing the current time with the help of the construction if
, we find out the time of day and write it into this variable. After that, we use a variable in the JSX code returned by the component. As usual, it is recommended to experiment with what we have learned today.
Results
In this lesson, we talked about some of the features of the code style used in this course, as well as the interaction between JSX and JavaScript. Using JavaScript-code in JSX opens up great opportunities, the practical usefulness of which we will feel in the next lesson, when we will deal with the built-in styles.
Dear readers! Do you use semicolons in your JavaScript code?
