
Methods of simulation of probability distributions in the Python programming language
In this article, we consider methods of simulation based on the theory of queuing systems, which widely use elements of mathematical statistics and probability theory. The purpose of this article is to develop methods that can be used to create models based on probability distributions and mass serving elements.
Before proceeding to the development of methods, we define the key approaches that we will use in the process: the generation of random variables, probability distributions, as well as some elements of probability theory and mathematical statistics. In the results of the experiments, we will see an experimental demonstration of the behavior of probability distributions and simulations of random processes. All this will give us an instrumental basis for creating various simulation models in which probability distributions appear.
All modeling methods for this study are presented in the programming language Python. This language is a common tool in research and education.
In the further stages of the study, which will be presented in future articles, we will move on to more complex experiments: consider probabilistic distributions that are not included in the scope of this article, consider simulation modeling of queuing systems and networks, and also demonstrate the programming of such models using parallel computing.
Consider simple models of tossing one or more dice. We begin the simulation by generating random numbers. The task of generating numbers and questions about true and quasi-randomness are not included in our research, but you can find these discussions in other sources. Our models use the Python pseudo-random variable generator. At the initial stage, for clarity of study, you can increase the number of tests by observing the simulation result.
Let's start by generating random variables. The methods for generating them boil down to using standard Python libraries.
module randomfrom the standard library provides the ability to get random real numbers in the range from 0 to 1, random integers in a given range, randomly select elements of a sequence and much more: the
random module can be used, for example, to mix a deck of cards in a game, randomly select an image in a program slide shows, in statistical modeling programs and so on. See the Python Standard Library Guide for more information. NumPy
extension containing the implementation of mathematical calculations for Python by combining compiled and optimized extension libraries with the Python NumPy languageturns Python into a powerful, efficient, and convenient math tool.
A detailed description of the NumPy module can be found in the official documentation for this package.
Let's start modeling: we will toss a dice. The following is a listing of the programming of the model of a toss of one die (Fig. 1). A cube can take one of six values.
Fig. 1. Programming listing for the model of tossing one cube
In the presented program, we set the number of tests: how many times the cube is thrown, and the array: what value falls out in each test. The result is displayed as a histogram (Fig. 2).
Considering the result, we can assume that the distribution is uniform. Increasing the number of tests to 10,000 times, you can see that the histogram has a view similar to a histogram of uniform distribution.
The next test will consider the model of tossing two dice. The code of the program will change slightly (Fig. 3).

Fig. 2. Simulation results of one die roll
Fig. 3. Part of the modified listing for programming the model of tossing two dice
In the presented listing, a significant change is the assignment of a value to an array in which we write out the dropped values of two dice. The second change, less significant, is the histogram presentation of the corresponding value options. The result of the program is shown in Fig. 4.
Having received the simulation result of the tossing of two cubes, we consider the problem of modeling the normal (Gauss) distribution and how much it correlates with the problem of modeling the tossing of several cubes. The program code also changes slightly: only one line of assigning values to the array changes (Fig. 5).
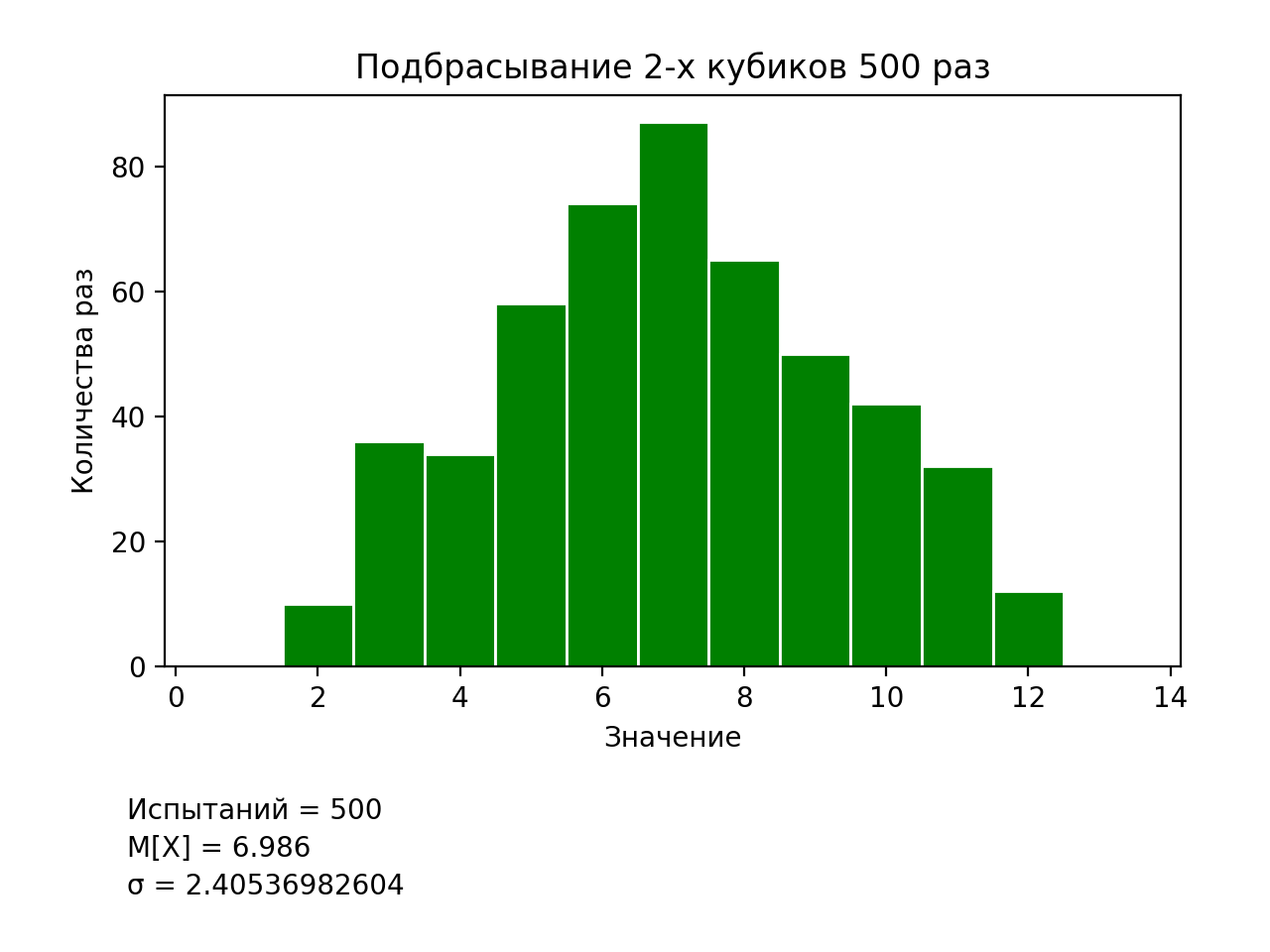
Fig. 4. The simulation results of the toss of two dice
Fig. 5. Part of the modified listing for programming the normal distribution model.
The listing used uses the normalvariate method of the random library, which generates values with a normal probability distribution with the parameters mu and sigma, which are the average value and standard deviation of the distribution, respectively. The result of the program is shown in Fig. 6.

Fig. 6. Normal distribution simulation results
The final step is modeling the exponential distribution. The exponential distribution is often used in modeling the distribution (duration) of intervals between the moments of receipt of applications in queuing systems of various types. The listing also differs slightly from the rest of the program listings presented in our study (Fig. 7).
The simulation result is shown in Fig. 8.
Fig. 7. Part of the modified listing for programming the exponential distribution model
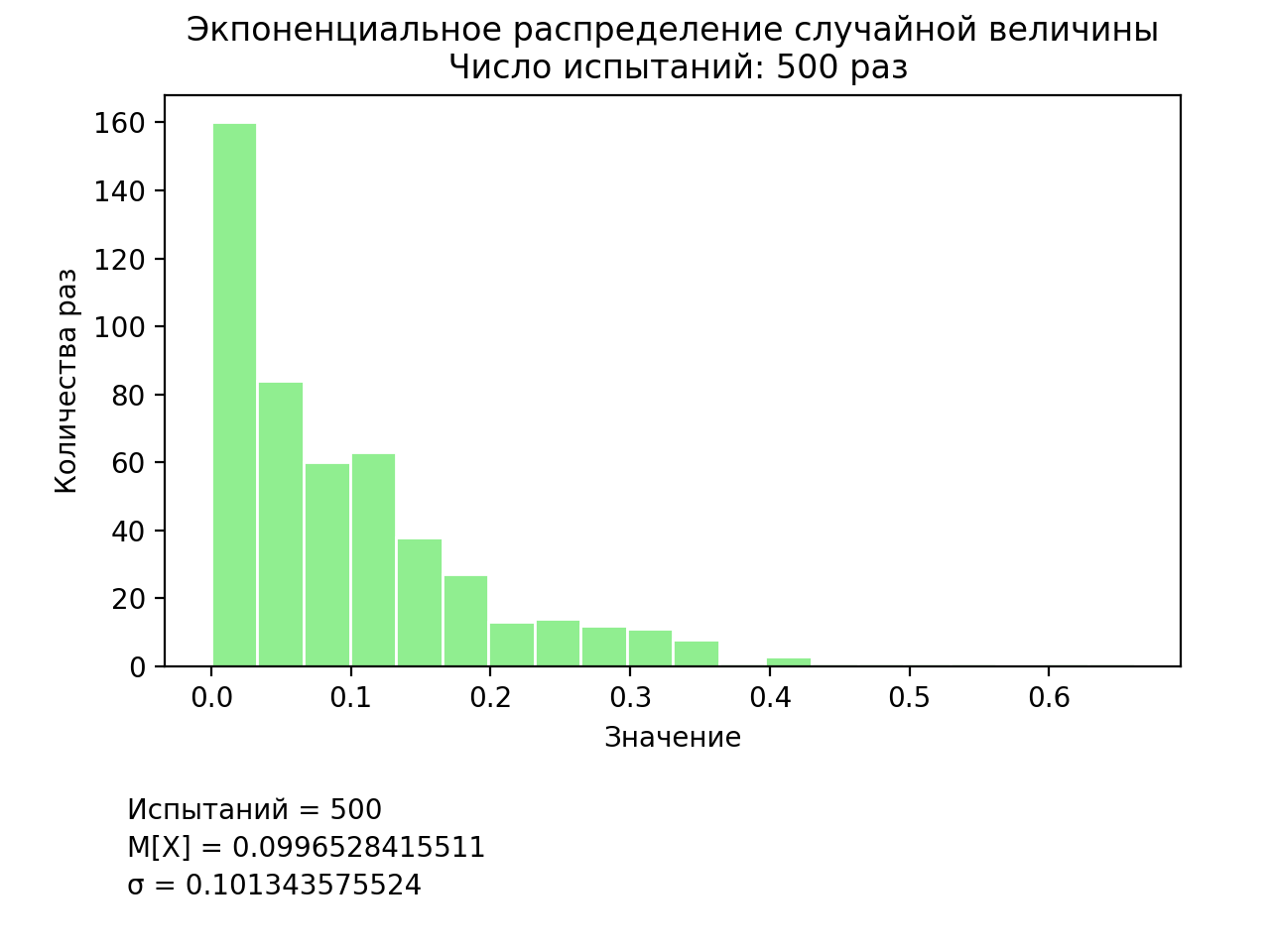
Fig. 8. Exponential Distribution Modeling Results
This article examined several software implementations of probability distribution models in Python. These models provide the basis for conducting your own experiments and research.
At this stage, only some methods and approaches are presented, and in the future, the author will present additional methods for modeling using probability distributions, the Python programming language, and queuing theory.
Before proceeding to the development of methods, we define the key approaches that we will use in the process: the generation of random variables, probability distributions, as well as some elements of probability theory and mathematical statistics. In the results of the experiments, we will see an experimental demonstration of the behavior of probability distributions and simulations of random processes. All this will give us an instrumental basis for creating various simulation models in which probability distributions appear.
All modeling methods for this study are presented in the programming language Python. This language is a common tool in research and education.
In the further stages of the study, which will be presented in future articles, we will move on to more complex experiments: consider probabilistic distributions that are not included in the scope of this article, consider simulation modeling of queuing systems and networks, and also demonstrate the programming of such models using parallel computing.
Python random number modeling methods and random number generators
Consider simple models of tossing one or more dice. We begin the simulation by generating random numbers. The task of generating numbers and questions about true and quasi-randomness are not included in our research, but you can find these discussions in other sources. Our models use the Python pseudo-random variable generator. At the initial stage, for clarity of study, you can increase the number of tests by observing the simulation result.
Let's start by generating random variables. The methods for generating them boil down to using standard Python libraries.
module randomfrom the standard library provides the ability to get random real numbers in the range from 0 to 1, random integers in a given range, randomly select elements of a sequence and much more: the
random module can be used, for example, to mix a deck of cards in a game, randomly select an image in a program slide shows, in statistical modeling programs and so on. See the Python Standard Library Guide for more information. NumPy
extension containing the implementation of mathematical calculations for Python by combining compiled and optimized extension libraries with the Python NumPy languageturns Python into a powerful, efficient, and convenient math tool.
A detailed description of the NumPy module can be found in the official documentation for this package.
Methods for modeling probability distributions using python
Let's start modeling: we will toss a dice. The following is a listing of the programming of the model of a toss of one die (Fig. 1). A cube can take one of six values.
import pylab
import numpy
# Количество испытаний
trials = 500
# Массив значений
values = numpy.random.randint(1, 7, size=trials)
pylab.hist(values, bins=[0.5, 1.5, 2.5, 3.5, 4.5, 5.5, 6.5], edgecolor='white')
pylab.xlabel('Значение')
pylab.ylabel('Количества раз')
pylab.title('Подбрасывание кубика ' + str(trials) + ' раз')
pylab.show()
Fig. 1. Programming listing for the model of tossing one cube
In the presented program, we set the number of tests: how many times the cube is thrown, and the array: what value falls out in each test. The result is displayed as a histogram (Fig. 2).
Considering the result, we can assume that the distribution is uniform. Increasing the number of tests to 10,000 times, you can see that the histogram has a view similar to a histogram of uniform distribution.
The next test will consider the model of tossing two dice. The code of the program will change slightly (Fig. 3).

Fig. 2. Simulation results of one die roll
...
# Массив значений
values = []
for i in range(trials):
values.append(numpy.random.randint(1, 7) + numpy.random.randint(1, 7))
pylab.hist(values, bins=[0.5, 1.5, 2.5, 3.5, 4.5, 5.5, 6.5,
7.5, 8.5, 9.5, 10.5, 11.5, 12.5, 13.5],
facecolor='green', edgecolor='white')
...
Fig. 3. Part of the modified listing for programming the model of tossing two dice
In the presented listing, a significant change is the assignment of a value to an array in which we write out the dropped values of two dice. The second change, less significant, is the histogram presentation of the corresponding value options. The result of the program is shown in Fig. 4.
Having received the simulation result of the tossing of two cubes, we consider the problem of modeling the normal (Gauss) distribution and how much it correlates with the problem of modeling the tossing of several cubes. The program code also changes slightly: only one line of assigning values to the array changes (Fig. 5).
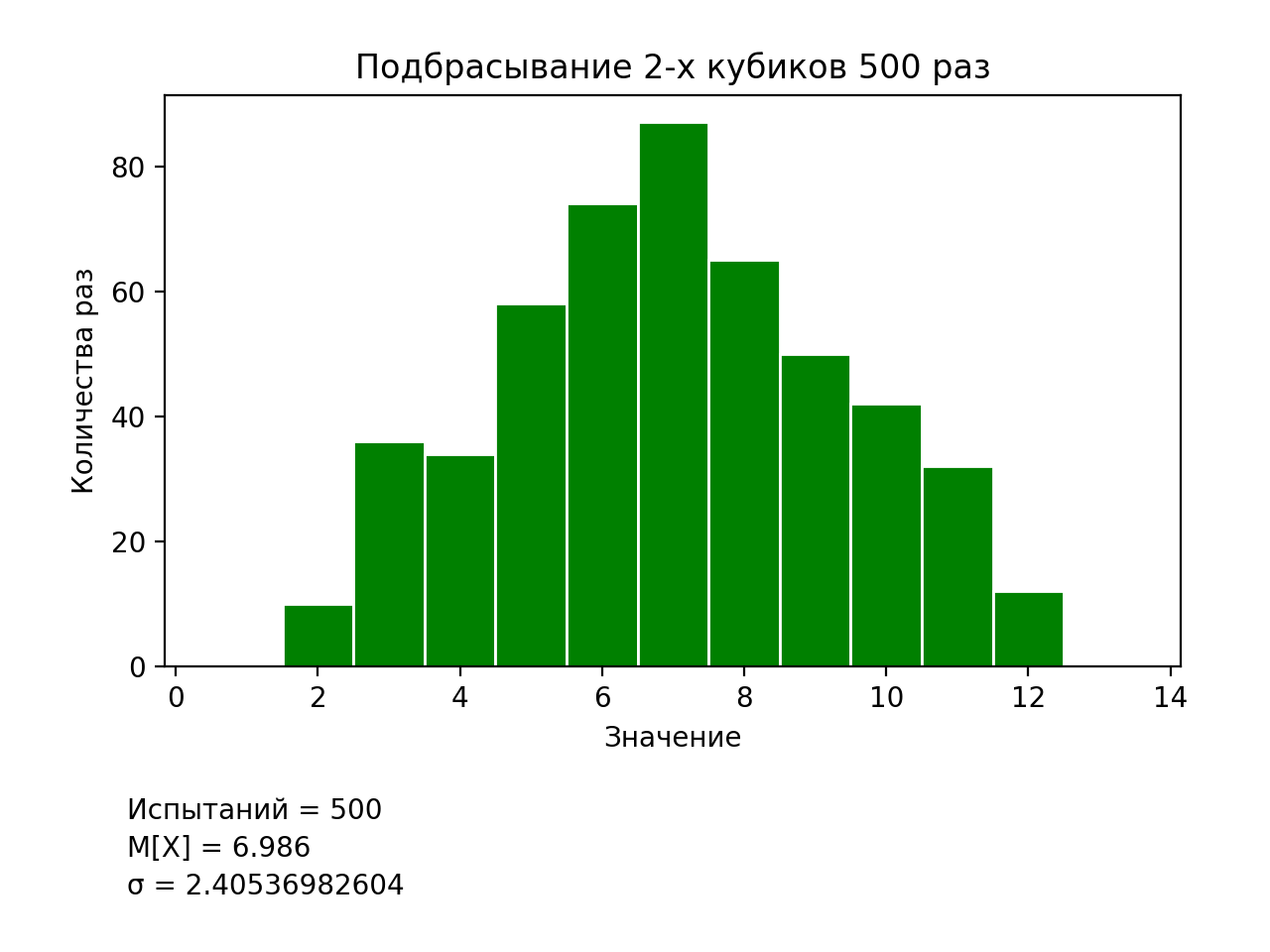
Fig. 4. The simulation results of the toss of two dice
...
# Массив значений
values = []
for i in range(trials):
mu = 7
sigma = 2.4
values.append(random.normalvariate(mu, sigma))
...
Fig. 5. Part of the modified listing for programming the normal distribution model.
The listing used uses the normalvariate method of the random library, which generates values with a normal probability distribution with the parameters mu and sigma, which are the average value and standard deviation of the distribution, respectively. The result of the program is shown in Fig. 6.

Fig. 6. Normal distribution simulation results
The final step is modeling the exponential distribution. The exponential distribution is often used in modeling the distribution (duration) of intervals between the moments of receipt of applications in queuing systems of various types. The listing also differs slightly from the rest of the program listings presented in our study (Fig. 7).
The simulation result is shown in Fig. 8.
...
lambd = 10
# Массив значений
values = []
for i in range(trials):
values.append(random.expovariate(lambd))
pylab.hist(values, 20, facecolor='lightgreen', edgecolor='white')
...
Fig. 7. Part of the modified listing for programming the exponential distribution model
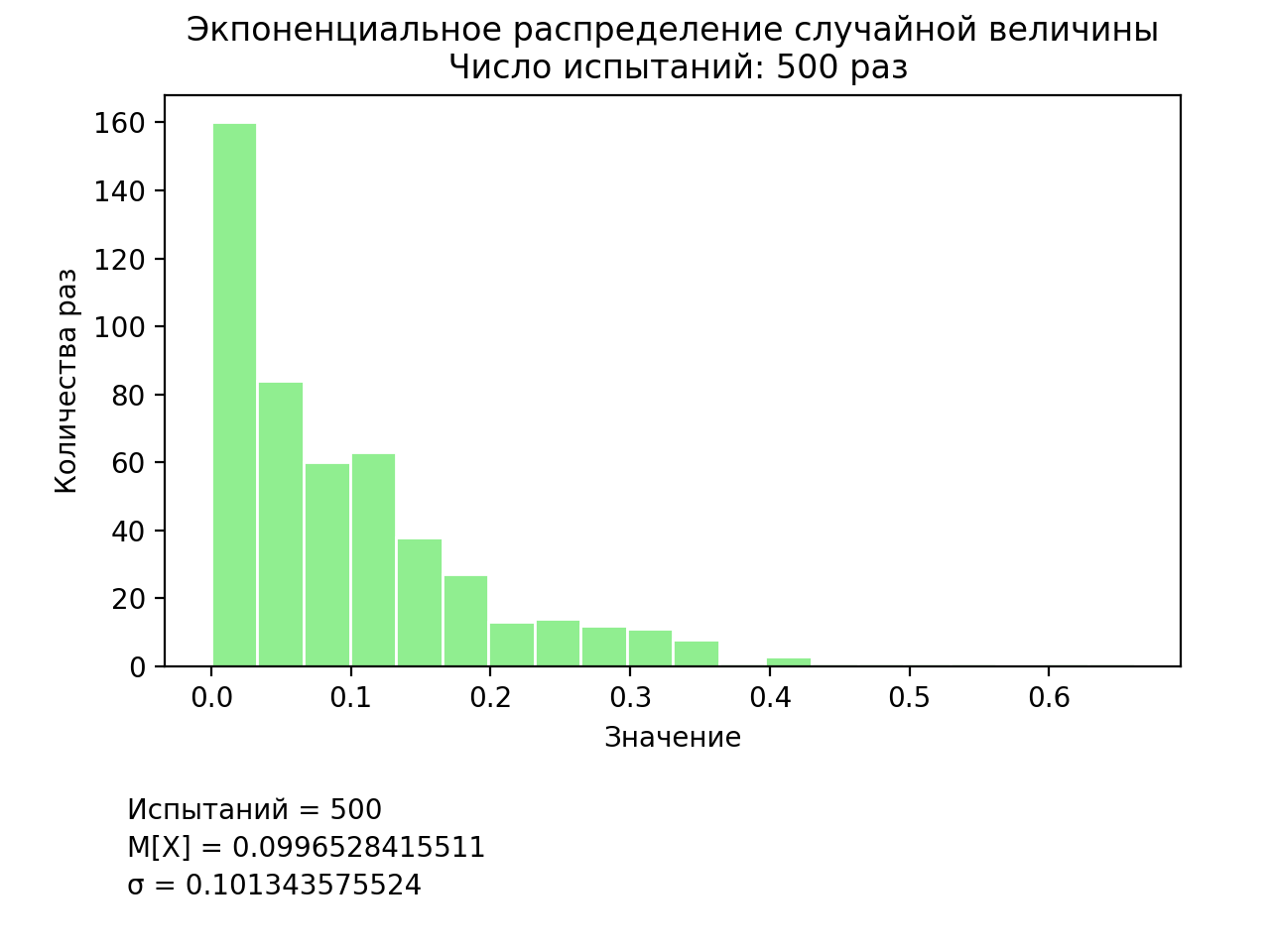
Fig. 8. Exponential Distribution Modeling Results
Conclusion
This article examined several software implementations of probability distribution models in Python. These models provide the basis for conducting your own experiments and research.
At this stage, only some methods and approaches are presented, and in the future, the author will present additional methods for modeling using probability distributions, the Python programming language, and queuing theory.