
SwiftyBeaver Guide for iOS: Logging Platform for Swift
- Transfer
- Tutorial
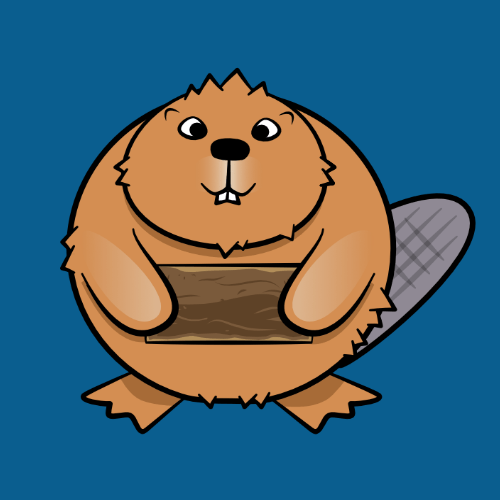
Other errors are more sinister. They lurk in the background and happen when you least expect it. They are the horrors of nightmares and Listerine commercials.
In this lesson you will find a secret weapon with which you can deal with these ominous errors that lurk in the background. When used properly, this weapon looks like a flashlight in the deepest and darkest crevices of your app. These terrible mistakes will not have a single chance.
This weapon is SwiftyBeaverSwift-based logging system for iOS and macOS.
In this guide, you will learn how to debug your application using SwiftyBeaver and get the following useful skills along the way:
- When SwiftyBeaver Logging Is Better Than Print () and NSLog ()
- How to Access SwiftyBeaver Logs in Crypto Cloud
- How to use logging levels for different types of log messages
- How to filter records to make it easier to find errors
Note: Before starting the lesson, you should be familiar with Cocoapods and the CoreLocation framework . Check out these guides first to get the skills you need.
Start
Download the initial project . This archive contains the project and all the necessary files to complete the lesson.
You are about to create an app to track your children. If they go too far from home, the application will warn them.
This app is called Ten PM (Do you know where your children are?).
Open TenPM.xcworkspace and see the project. Now open Main.storyboard and you will see two view controllers inside the navigation controller:
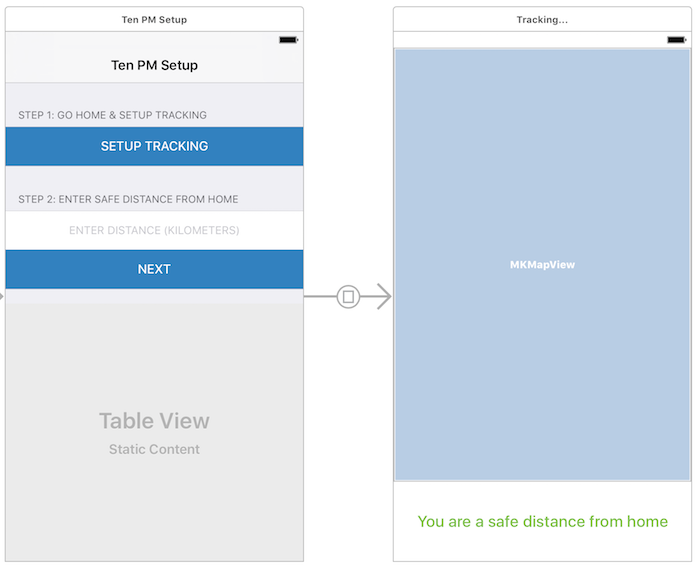
The view controller on the left is the one that parents can use to tell the application where the “home” is and also set the maximum distance that children can move away. Class - TrackingSetupViewController , which requests access to geolocation services and stores the entered distance to limit the distance.
The view controller on the right is the one that children will use. It displays a map with a green circle representing a safe area. It also contains a block of text below to let them know if they are currently in a safe area or not. The class is the TrackingViewController , which uses MKMapView to display children-friendly territory.
And here are a few more notes:
- LocationTracker.swift : Acts as a delegate to the CLLocationManager. This handles location updates and permission changes for geolocation services.
- ParentProfile.swift : Saves important user data to NSUserDefaults. This data includes the location of the home and the distance their children can move away from home.
We use the initial application
Run the offer on the iOS simulator. You will see the view controller that is represented by the TrackingSetupViewController class :
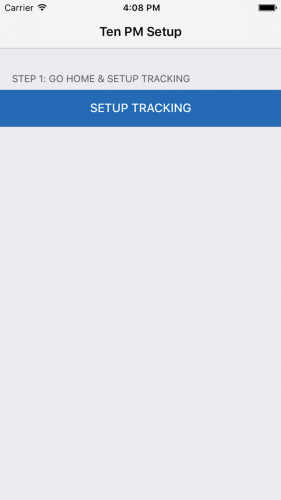
Before you set up tracking, you must be sure that the simulator provides information about the starting location. To do this, select the simulator, then in the panel select Debug \ Location \ Apple .
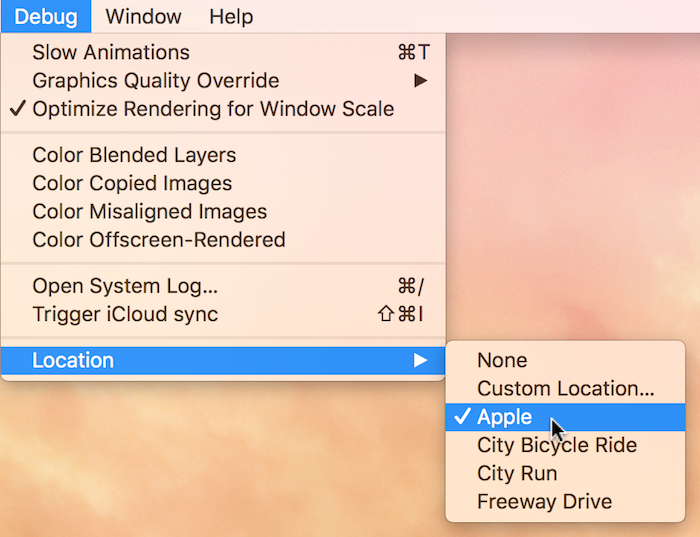
Press the SETUP TRACKING button and the application will ask for permission to start collecting location data. Since this screen is for parents there is one extra window to confirm that you are a parent and are at home. Select Yes and then Allow . Once the application determines your location and saves it as a home, to use it as a center for a safe zone for children.
Now enter 1 in the text box below as a safe distance from home. This means that you do not want to be further than 1 kilometer from the simulated location of Apple.

Press the NEXT button and you will be taken to the view supported by TrackingViewController . On this screen, there is one annotation with your current location and a green circle indicating your safe area.
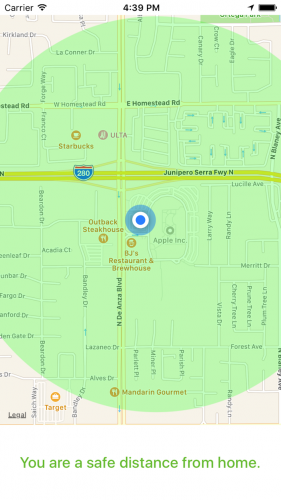
Now that everything is set up, it's time to pretend that it's 2011, you got the iPhone 4 prototype and go for a walk. Let's pretend to be in a new location, outside the safe zone, where you made an appointment with a Gawker reporter.
From the iOS Simulator menu, go back to Debug \ Location , but this time select Custom Location . Enter 37.3393 as latitude and -122.0426 as longitude, then click OK .
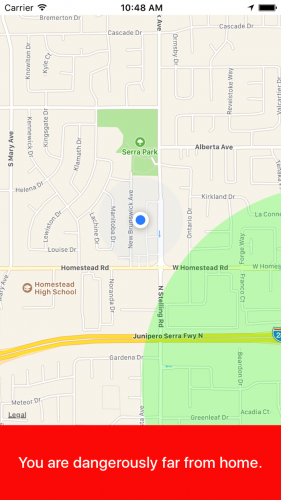
You should see the blue dot move to a new location and the message at the bottom of the screen changes to tell you that you are at a dangerous distance from home.
This is a brief description of the functionality of the Ten PM application . This is a fairly simple application that can be easily expanded. You could start sending notifications to parents when their child moves out of the safe zone, etc.
But today you are not going to do it. Instead, you will use this application to simulate some unpleasant background errors, which you then fix using SwiftyBeaver.
Install SwiftyBeaver
To get started, install SwiftyBeaver using Cocoapods. Find the project root directory and find the Podfile there . Open it in the editor that is most convenient for you and add the following line under the line # Pods for TenPM :
pod 'SwiftyBeaver'
Open Terminal, go to the directory with these Podfile and enter the following command:
pod install
After a minute or maybe a little more, the installation of SwiftyBeaver in your project will be completed. Now open AppDelegate.swift and add the following line in a suitable place at the top of the file:
import SwiftyBeaver
Compile this project and you will see that SwiftyBeaver is now available in the project because it was successfully compiled.
Note: this installation guide works with Cocoapods and Swift 3. More detailed installation instructions can be found on the repository page of the SwiftyBeaver project on GitHub .
Writing Your First Logs With SwiftyBeaver
Fuh. Are you ready to write some code?
Take a look at AppDelegate.swift again. This is where you now configure logging for SwiftyBeaver. Have you noticed a blank method in your starting project?
setupSwiftyBeaverLogging () is called every time your application starts, and as the name suggests, you will use it to prepare SwiftyBeaver for use. Go to this method and add the following:
let console = ConsoleDestination()
SwiftyBeaver.addDestination(console)
SwiftyBeaver is an unusual logging tool. It has several inputs called Destinations , which you can configure to suit your needs.
Destination determines where your logs appear.
ConsoleDestination () creates the Console Destination , which you added as the active destination for SwiftyBeaver.
In the application (_: didFinishLaunchingWithOptions :) method, add the following line of code, after calling the setupSwiftyBeaverLogging () method :
SwiftyBeaver.info("Hello SwiftyBeaver Logging!")
This code displays the info level log in the console when the application starts. You will learn more about log levels a bit later.
Launch Ten PM again. Check the console when the application starts and you will see the entry:
12: 06: 05.402
Cool! You log in to the console using SwiftyBeaver. The heart image refers to the level of the logs, which are explained below.
Brief explanation of log levels
I’m sure that at this stage you are wondering why SwiftyBeaver forces you to use a method called info () instead of more understandable and logical names such as log () or print () .
This is due to what is called Log Levels .
Not all logs are equally important. Some logs are useful for providing the programmer with additional contextual information. Other logs are needed for more serious problems, such as errors and warnings. When studying logs, it’s quite useful to have entries that are classified by threat level for the application. This helps to filter less important messages faster in order to fix errors faster.
SwiftyBeaver follows the conventions and uses these 5 levels of logs:
- Verbose : lowest priority level. Use them for contextual information.
- Debug : Use this level to display variables or other results that will help you fix a bug or solve a problem.
- Info : commonly used for information useful in a more general context of support. In other words, the information is useful for non-developers to search for problems.
- Warning : use this level when you reach a state that does not necessarily cause a problem, but decisively leads the application in this direction.
- Error : the most serial and highest priority of logs. Use it only when your application has caused a serious error.
How to record different levels of logs with SwiftyBeaver
Just as you used the info () method for logging at the info level, you can use four other methods: verbose () , debug () , warning () , and error () for logging at the other four levels.
Give it a try. Go back to the application (_: didFinishLaunchingWithOptions :) method and replace the info () call with the following code:
SwiftyBeaver.debug("Look ma! I am logging to the DEBUG level.")
Now run your application. You should see a different color for the heart icon and another message at the debug level .
14: 48: 14.155
Note that the color of the icon has changed to green to indicate debug log level. This is one of the reasons why SwiftyBeaver is better than print () and NSLog () . You can quickly look through the logs and find messages at a level that interests you.
Note: try not to abuse the higher levels of logging. Warnings and errors should be reserved for situations requiring proper attention.
Configuring SwiftyBeaver Crypto Cloud
One of SwiftyBeaver’s coolest features is the ability to log directly to the cloud. SwiftyBeaver has an application for macOS that allows you to view logs in real time. If you ever wondered what was going on in your application installed on thousands of devices, now you can know it.
Download the SwiftyBeaver for Mac app . Open it and fill out the form to create an account.

Next, you will be redirected directly to the file save window. This is a little strange, because they do not report what this file is for.
Name this file TenPMLogs , select any location of your choice and click Save .
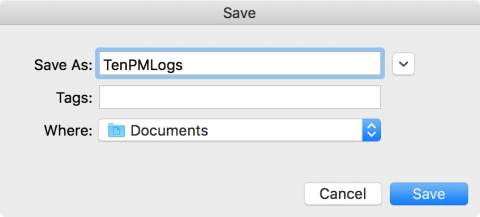
The file that you created stores the logs for one application, for this reason you named it TenPMLogs. When you open this file using the SwiftyBeaver Mac App, you can see the logs related to the Ten PM application. After saving the file, you will be given a choice. You can register a new application or view the logs from a previously registered application. You continue on the New App tab .
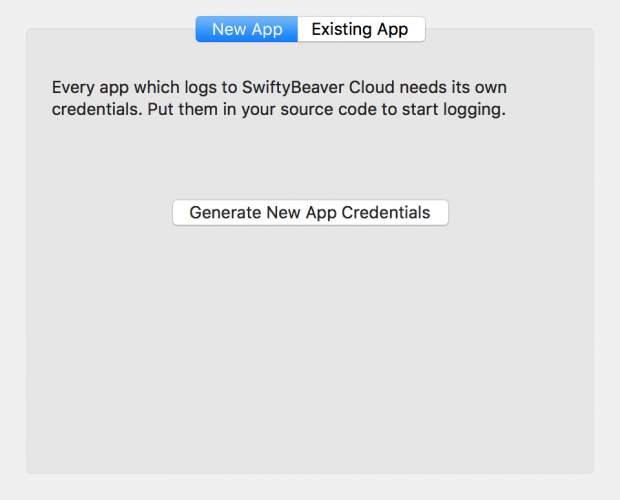
Click the Generate New App Credentials button . You should see the following screen showing the generated identifier and keys for your application:
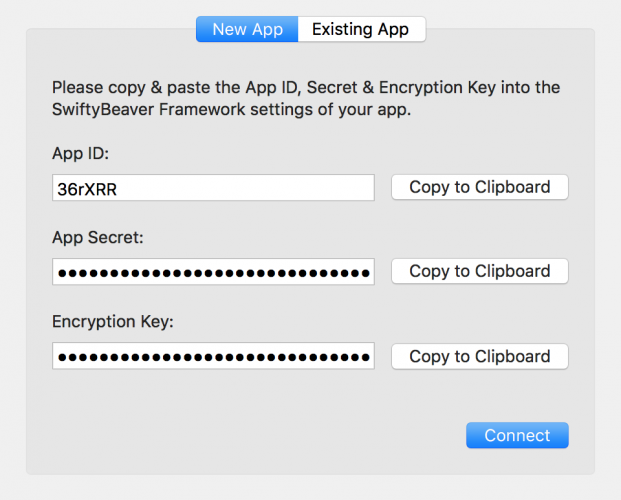
Now it's time to add another destination for the logs using the newly created Crypto Cloud security credentials. Leave this window open and return to the setupSwiftyBeaverLogging () method in AppDelegate.swift .
Add these lines to the bottom of this method, replacing the lines with the appropriate value from the SwiftyBeaver Mac App:
let platform = SBPlatformDestination(appID: "Enter App ID Here",
appSecret: "Enter App Secret Here",
encryptionKey: "Enter Encryption Key Here")
SwiftyBeaver.addDestination(platform)
Return to the SwiftyBeaver Mac App and click the Connect button . Now launch the Ten PM app.
Note: if you stupid like me and clicked the Connect button before you copied the credentials to your application, you can click on the settings button (gear) in the SwiftyBeaver Mac App to view them after connecting.
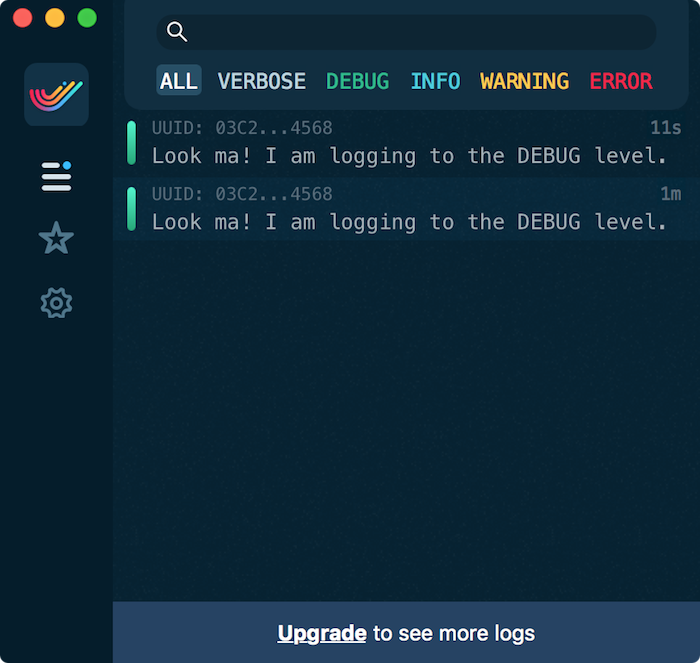
Now check it out! Your logs appear in the SwiftyBeaver Mac App. If you do not see the recording immediately, then do not worry. Sometimes it takes several minutes before log entries fall into the cloud. In the end, they will appear anyway. SwiftyBeaver also automatically provides one-hour storage for your logs if you have not switched to a paid account. For most debugging tasks this will not be a problem. It’s just worth noting if you are wondering why your older logs are no longer visible.
Filtering logs by log level, selected entries and minimum log levels
The really cool thing about SwiftyBeaver Mac App is the ability to filter logs by log level. This greatly simplifies the process of digging into the logs to find the cause of a critical error.
You may have already noticed the different tabs at the top. Each of these tabs represents a logging level. You can view several levels at a time or you can only see warnings and errors.
You can also mark a post as a favorite. You can see all the selected entries by clicking on a star in the left menu.
Minimum Log Filtering
This is another feature you really love. SwiftyBeaver allows you to set the minimum level for a given destination. If you want to use your Crypto Cloud account for serious warnings and errors, you can do this.
First, replace the current logging code in applicationDidFinishLaunching () with the following:
SwiftyBeaver.verbose("Watch me bloviate. I'm definitely not important enough for the cloud.")
SwiftyBeaver.debug("I am more important, but the cloud still doesn't care.")
SwiftyBeaver.info("Why doesn't the crypto cloud love me?")
SwiftyBeaver.warning("I am serious enough for you to see!")
SwiftyBeaver.error("Of course I'm going to show up in your console. You need to handle this.")
Now you are logging a message at each level. Launch the application and you should see how all these entries get into the Crypto Cloud.
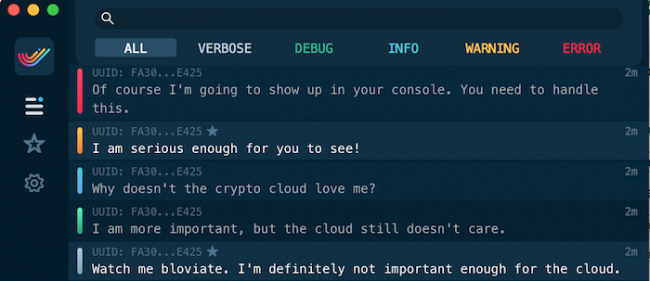
In the setupSwiftyBeaverLogging () method, add the following before adding this platform to destination:
platform.minLevel = .warning
Launch the application again. Take a look at the new look of your Crypto Cloud console.

You should only see warnings and errors lately. No other entries will go to Crypto Cloud. You still see everything in the Xcode console!
Note: You can set a minimum level for any type of logging (destination) in SwiftyBeaver. You can create several Crypto Cloud logs for different levels of logging. SwiftyBeaver has a lot of room for different ways of logging.
Correction of hard-to-reach errors
It was fun to talk about logging into Crypto Cloud, but you have some ominous errors that you need to fix. Well, or at least you have ominous errors that you intentionally simulate and then correct them.
Start by clearing all early entries. Remove all logging from application (_: didFinishLaunchingWithOptions :) . Also remove the setting for platform.minLevel so that all entries are displayed by default. For this test, you will need to see all the log entries.
Error modeling
Now you are ready to use cloud-based logging and this is the time to simulate an unpleasant background bug.
Open LocationTracker.swift and find the locationManager method (_: didUpdateLocations :) there . Paste the following code there after declaring two guard values at the top of the method:
let bug = true
if bug == true {
return
}
It's pretty stupid, but pretend here that somewhere in LocationTracker there is an error that prevents tracking the user's location. This code here prevents notifications that the user has entered or left the safe zone. When the “error” is “disabled” this code will work fine.
Run the application to make sure that there is a “mistake”.

As long as you remain at the location previously set using the coordinates, you will see an error. Despite the fact that you are clearly outside the safe zone, the text says that you are at a safe distance from home.
Tracking bugs with SwiftyBeaver
How now could we track this error with SwiftyBeaver? If you received error reports on failed location tracking but don’t have any good error assumptions, add logging wherever possible to get as much information as possible from users.
To get started, import the SwiftyBeaver at the top of the LocationTracker :
import SwiftyBeaver
Next, add an entry at the top of the locationManager method (_: didUpdateLocations :) :
SwiftyBeaver.info("Got to the didUpdateLocations() method")
Now add a few lines at the bottom and insert this line right after declaring the bug constant :
SwiftyBeaver.debug("The value of bug is: \(bug)")
Next, add the information when we check the value of this constant, but before return :
SwiftyBeaver.error("There's definitely a bug... Aborting.")
Finally, add an entry at the end of the locationManager (_: didUpdateLocations :) method :
SwiftyBeaver.info("Got to the end the didUpdateLocations method")
This information should be enough to start calculating what happens in your code. This is what the entire contents of the locationManager method should look like (_: didUpdateLocations :) :
SwiftyBeaver.info("Got to the didUpdateLocations() method")
guard let homeLocation = ParentProfile.homeLocation else {
ParentProfile.homeLocation = locations.first
return
}
guard let safeDistanceFromHome = ParentProfile.safeDistanceFromHome else {
return
}
let bug = true
SwiftyBeaver.debug("The value of bug is: \(bug)")
if bug == true {
SwiftyBeaver.error("There's definitely a bug... Aborting.")
return
}
for location in locations {
let distanceFromHome = location.distance(from: homeLocation)
if distanceFromHome > safeDistanceFromHome {
NotificationCenter.default.post(name: TenPMNotifications.UnsafeDistanceNotification, object: nil)
} else {
NotificationCenter.default.post(name: TenPMNotifications.SafeDistanceNotification, object: nil)
}
}
SwiftyBeaver.info("Got to the end the didUpdateLocations method")
In the simulator, set the location on Apple as you did before. Now run the application. Despite the fact that logs are now available to you in the Xcode console, ignore them and imagine that you are tracking entries in the Crypto Cloud from a remote user.
Enter a safe distance of 1 kilometer and press NEXT . After downloading the map, change your location to latitude 37.3397 and longitude -122.0426 through a special location.
And again you went beyond your safe zone without updating the text.

You should notice the following entries repeating in SwiftyBeaver Crypto Cloud after setting the filter to ALL :
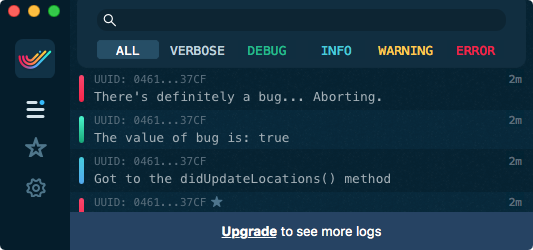
Wow, this is really useful! If you return to your code in the LocationTracker class, you can compare this with the logs and you will see how far your code runs before it stops. Here it is explicitly in if bug == true where the error entry was displayed.
To “fix” this “error” simply set the bug constant to false where it is declared in the locationManager method (_: didUpdateLocations :) :
let bug = false
Launch the app. Simulate the starting position in Apple with a shift outside the safe zone. This time you will see a warning about the safe zone, which worked correctly.
You should also see the following entries in your Crypto Cloud console.

It looks as if the application passed the error and again successfully responds to location changes. You have successfully fixed this error!
Where to go?
You can find a completed project for this lesson here . Also check out file logging with SwiftyBeaver. If you have an iPhone and you want to be 100% sure that you will receive all your notes (even if there is no Internet connection). For more interesting cases, check out post formatting .