
Microsoft Bot Framework on Linux under Node.JS
- Tutorial
To create and launch our first chat bot using Microsoft's Bot Framework Microsoft's Bot Framework for Linux, we need to install the following components:
After creating the simplest bot, we will analyze how you can start and debug it.
The installation commands given here are suitable for Debian / Ubuntu / Mint distributions; for the rest, instructions can be found here . In the terminal, run the following commands:
We need the Bot Framework Emulator to debug our bot. Open the next page in the browser. In the terminal, we check the architecture of our machine with the command:
According to the architecture, download the corresponding file from the Github page, in my case this is the file:
After downloading, add the file execution rights and run.
Installs easily in a couple of clicks on this link .
Open the Visual Studio Code, choose a new folder that will contain the project and run the domestic terminal:
Click a few times
Here in the same folder in VS Code (or any other editor) we create the app.js file and add the following code to it:
We save the file, now we are ready to launch and debug our bot.
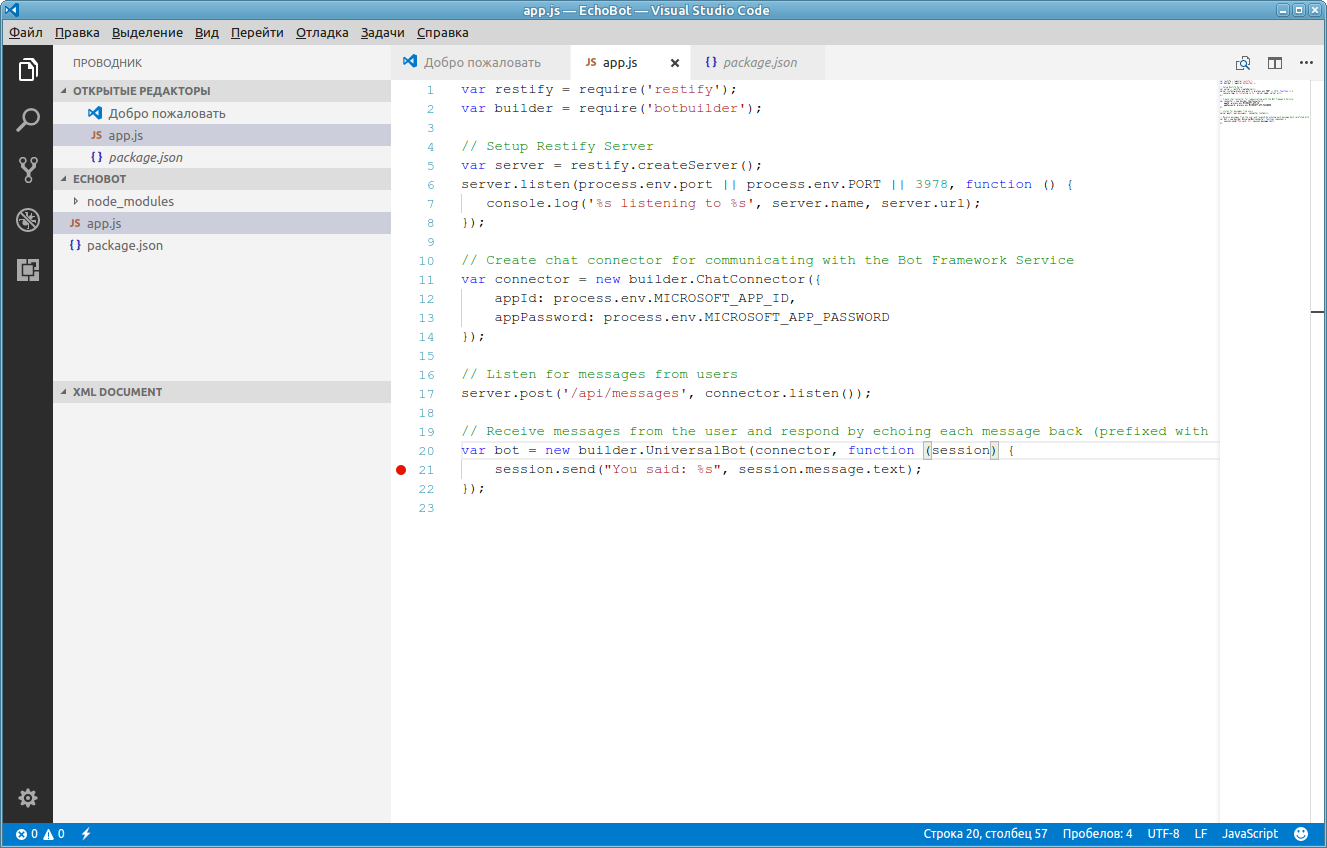
You can start the bot in the terminal with the command:
Or in the debugger Visual Studio Code -

That's all. More complex examples of building bots on node.js can be found here .
PS It would be interesting to write a similar article in relation to C # /. NET Core for Linux, but it seems that BotBuilder is not yet ready for .NET Core , although work is underway and there is some success as the full .NET 4.6 infrastructure becomes available for. NET Core.
- Node JS
- Bot Framework Emulator
- Visual Studio Code (optional)
After creating the simplest bot, we will analyze how you can start and debug it.
Install Node.JS
The installation commands given here are suitable for Debian / Ubuntu / Mint distributions; for the rest, instructions can be found here . In the terminal, run the following commands:
curl -sL https://deb.nodesource.com/setup_8.x | sudo -E bash -
sudo apt-get install -y nodejs
sudo apt-get install -y build-essential
Installing Bot Framework Emulator under Linux
We need the Bot Framework Emulator to debug our bot. Open the next page in the browser. In the terminal, we check the architecture of our machine with the command:
arch
According to the architecture, download the corresponding file from the Github page, in my case this is the file:
botframework-emulator-3.5.29-x86_64.AppImage
After downloading, add the file execution rights and run.
Install Visual Studio Code
Installs easily in a couple of clicks on this link .
Creating our first chat bot
Open the Visual Studio Code, choose a new folder that will contain the project and run the domestic terminal:
Ctrl-`
. We recruit a team to create a new project:npm init
Click a few times
Enter
later yes
. Now install 2 packages node.js: botbuilder and restify with the commands:npm install --save botbuilder
npm install --save restify
npm install --save dotenv-extended
Here in the same folder in VS Code (or any other editor) we create the app.js file and add the following code to it:
Echo Bot Code
// This loads the environment variables from the .env file
require('dotenv-extended').load();
var restify = require('restify');
var builder = require('botbuilder');
// Setup Restify Server
var server = restify.createServer();
server.listen(process.env.port || process.env.PORT || 3978, function () {
console.log('%s listening to %s', server.name, server.url);
});
// Create chat connector for communicating with the Bot Framework Service
var connector = new builder.ChatConnector({
appId: process.env.MICROSOFT_APP_ID,
appPassword: process.env.MICROSOFT_APP_PASSWORD
});
// Listen for messages from users
server.post('/api/messages', connector.listen());
// Receive messages from the user and respond by echoing each message back (prefixed with 'You said:')
var bot = new builder.UniversalBot(connector, function (session) {
session.send("You said: %s", session.message.text);
});
We save the file, now we are ready to launch and debug our bot.
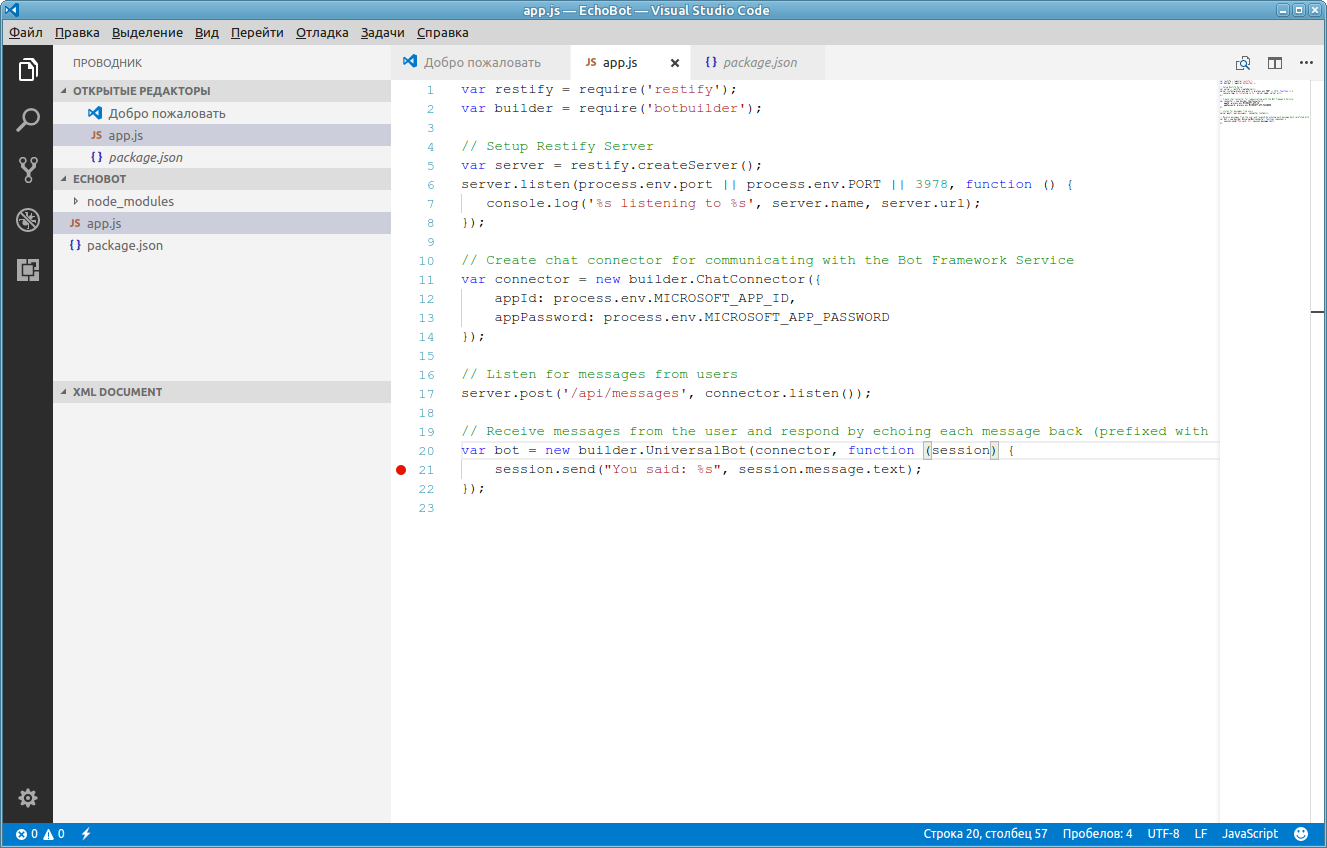
Start and debug chat bot
You can start the bot in the terminal with the command:
node app.js
Or in the debugger Visual Studio Code -
F5
. To set a breakpoint, move the cursor to the desired line and press - F9
. After starting the bot, we return to the emulator, connect to the address http://127.0.0.1:3978/api/messages
and type: Hi
and we see the answer of our bot: 
That's all. More complex examples of building bots on node.js can be found here .
PS It would be interesting to write a similar article in relation to C # /. NET Core for Linux, but it seems that BotBuilder is not yet ready for .NET Core , although work is underway and there is some success as the full .NET 4.6 infrastructure becomes available for. NET Core.