
Upload files to the Xamarin.Forms application. Part 2
- Transfer
- Tutorial
Last week we talked about sending files to the Xamarin.Forms app for iOS, as promised, in the second part we will talk about Android.
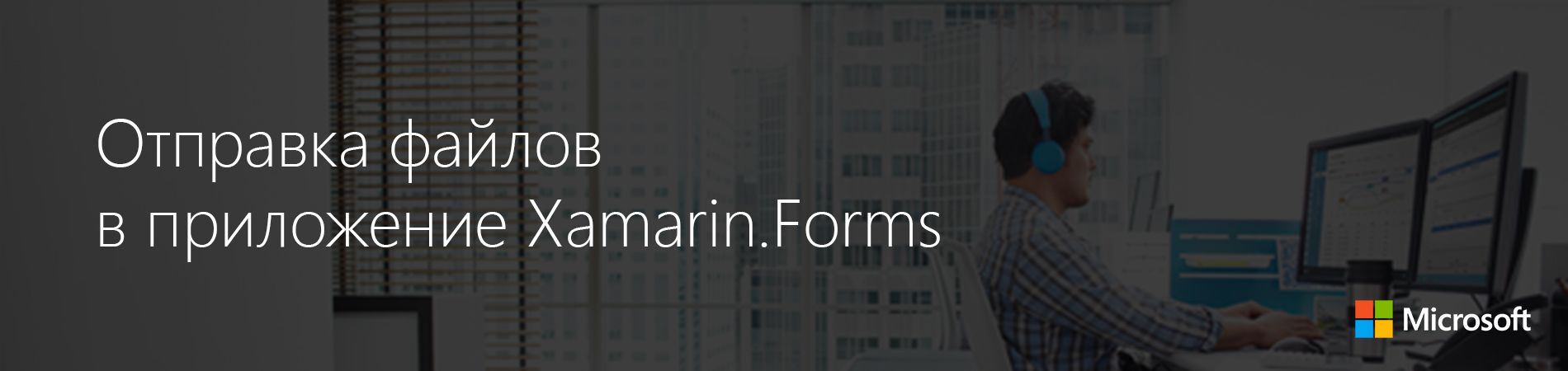
Uploading files to the Xamarin.Forms app: Part 1 (iOS) and Part 2 (Android) .
Again the script that will be discussed:
Let's get started.
Just as Intents are used to exchange data between Activity components, you can use Intents for the same between applications.
When it is clear which Activity component you need to open in the application, you need to create an explicit Intent. When an explicit Intent is created, the type of the class of the Activity component that should be launched is indicated, then the OS starts working when it finds StartActivity ().
Simple enough. But what happens when the developer of the source application does not know which Activity component should be launched, since this Activity component is in a separate application? The application “sends” a notification that there is something that needs to be delivered to another application and “asks” if it can perform an action on this.
This is where the implicit Intent comes to the rescue. The developer creates an implicit Intent without specifying the Activity component that should be launched.
However, the implicit Intent still needs enough information so that the Android OS can determine which application should receive the information. The creation of implicit Intents will not be considered here, but only their application. In this article we are told about Intent'ah detail.

All information about explicit and implicit Intents leads to the concept of Intent filters. Intent filters are a way in which an application that accepts a file is registered with Android to process incoming data.
Intent filters are associated with Activity components in the application, which should run when the file is received. A description of the intent filter appears in the AndroidManifest.xml file along with a description for the Activity component.
To determine which application will be launched and displayed in the options when the user sends a file of a certain type to another application, Android “looks through” all the Intent filters registered by the OS and filters the applications by comparing the criteria of Intent filters with the criteria declared in implicit Intent 'e.
How to create an intent filter?
As noted, an Intent filter description appears along with a description of the Activity component that should be run when the file is received.
An example of declaring an Activity component and an Intent filter in an AndroidManifest.xml file is as follows:
There are a couple of points to note here. First, one Activity component can have more than one Inent filter. The second is the keywords included in the site
This completes the work with the Android part. It is time for a Xamarin.Forms application.
First you need to understand how to add an Intent filter to the Android part of the Xamarin.Forms application. Xamarin provides many tools, so there is no need to often touch the AndroidManifest.xml file. If you need to add a value in this file, you can contact the visual designer (double-clicking the file) or various class attributes.
It so happened that you can add Intent filters using the class
There is also a class
Now that the application is recognized by Android as suitable, you need to consider the situation when something falls into it.
When a file arrives, function
This information is
Depending on the application sending the data, you can also see the URI of the file to be sent to Extras Intent
Regardless of where it came from
All
These are regular Xamarin.Forms
If it finds data in the property
When everything is done, the Android application will display the PDF files as in the image on the right.
In general, having understood how Android exchanges data between applications, which is similar to exchanging data between Activity components in one application, the task of receiving files in Android becomes easily solvable. You must declare an Intent filter in the class
Alexander Alekseev - Xamarin-developer, freelancer. Has been working with the .NET platform since 2012. He participated in the development of a procurement automation system at Digamma. Since 2015, he went into freelance and switched to mobile development using Xamarin. Currently working at StecPoint on an iOS application.
Leads the resource XamDev.ru and the community "Xamarin Developers" in social networks: VK , Facebook , Telegram .
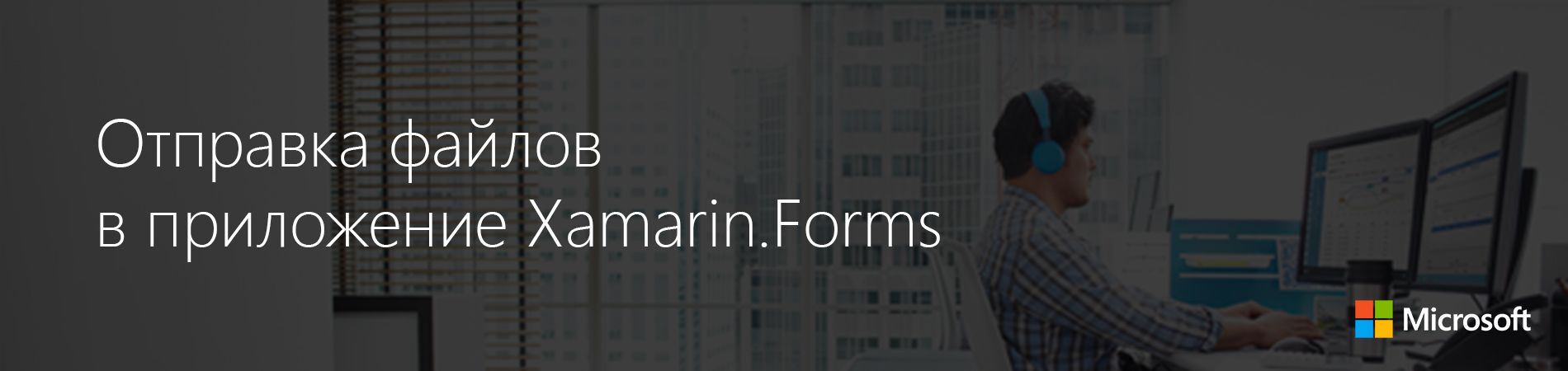
Uploading files to the Xamarin.Forms app: Part 1 (iOS) and Part 2 (Android) .
Scenario
Again the script that will be discussed:
- Writing an Xamarin.Forms Application.
- This application allows you to process files of a certain type (in the demo application discussed in this post these are PDF files).
- The OS should know that the application is capable of handling files of this type.
- The application should appear in the list of applications to send the file.
Let's get started.
Android Registration
Just as Intents are used to exchange data between Activity components, you can use Intents for the same between applications.
Explicit and Implicit Intents
When it is clear which Activity component you need to open in the application, you need to create an explicit Intent. When an explicit Intent is created, the type of the class of the Activity component that should be launched is indicated, then the OS starts working when it finds StartActivity ().
Simple enough. But what happens when the developer of the source application does not know which Activity component should be launched, since this Activity component is in a separate application? The application “sends” a notification that there is something that needs to be delivered to another application and “asks” if it can perform an action on this.
This is where the implicit Intent comes to the rescue. The developer creates an implicit Intent without specifying the Activity component that should be launched.
However, the implicit Intent still needs enough information so that the Android OS can determine which application should receive the information. The creation of implicit Intents will not be considered here, but only their application. In this article we are told about Intent'ah detail.

Intent filters
All information about explicit and implicit Intents leads to the concept of Intent filters. Intent filters are a way in which an application that accepts a file is registered with Android to process incoming data.
Intent filters are associated with Activity components in the application, which should run when the file is received. A description of the intent filter appears in the AndroidManifest.xml file along with a description for the Activity component.
To determine which application will be launched and displayed in the options when the user sends a file of a certain type to another application, Android “looks through” all the Intent filters registered by the OS and filters the applications by comparing the criteria of Intent filters with the criteria declared in implicit Intent 'e.
How to create an intent filter?
Intent Filter Announcement
As noted, an Intent filter description appears along with a description of the Activity component that should be run when the file is received.
An example of declaring an Activity component and an Intent filter in an AndroidManifest.xml file is as follows:
There are a couple of points to note here. First, one Activity component can have more than one Inent filter. The second is the keywords included in the site
. These keywords help Android filter out applications that can perform certain operations. Here is an explanation of the values in this case of getting the file:
- the Activity component is only going to respond to an implicit Intent, that is, send data to other applications.
- it is always necessary. Also, there should always be such a value to get an implicit Intent.Is a data type. Or the MIME type that the application can handle.
This completes the work with the Android part. It is time for a Xamarin.Forms application.
Integration with Xamarin.Forms
First you need to understand how to add an Intent filter to the Android part of the Xamarin.Forms application. Xamarin provides many tools, so there is no need to often touch the AndroidManifest.xml file. If you need to add a value in this file, you can contact the visual designer (double-clicking the file) or various class attributes.
It so happened that you can add Intent filters using the class
IntentFilterAttribute
. Since there is only one Activity component in a Forms application, you need to MainActivity
supplement the class with this attribute. To generate the XML code in the AndroidManifest.xml file that is identical to the one described above, the class declaration MainActivity
should look like this:[Activity(Label = "OpenFiles.Droid", Icon = "@drawable/icon", Theme = "@style/MyTheme", MainLauncher = true, ConfigurationChanges = ConfigChanges.ScreenSize | ConfigChanges.Orientation)]
[IntentFilter(new[] { Intent.ActionSend }, Categories = new[] { Intent.CategoryDefault }, DataMimeType = @"application/pdf")]
public class MainActivity : global::Xamarin.Forms.Platform.Android.FormsAppCompatActivity {
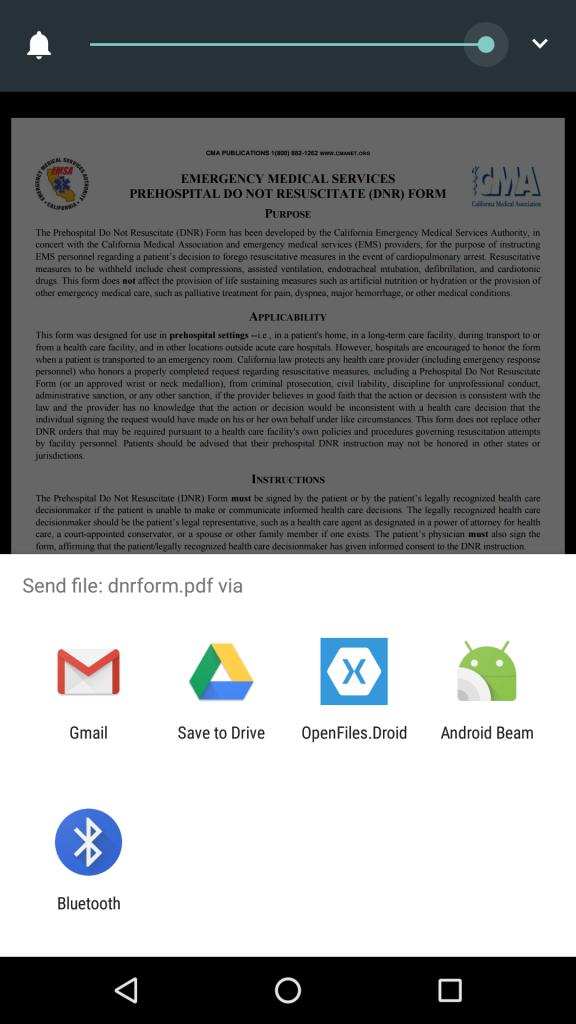
ActivityAttribute
that will create an Activity node in the manifest. The class then IntentFilterAttribute
adds an intent filter to this Activity node. Full class documentation IntentFilterAttribute
can be found here . It defines the action, category and type of input data. Action and category are arrays and they can be defined. They may also have parameters that help filter by matching criteria. With this add-on, Android class recognizes the application as capable of handling PDF files.Inbound File Processing
Now that the application is recognized by Android as suitable, you need to consider the situation when something falls into it.
When a file arrives, function
OnCreate
c is MainActivity
called. The Activity property Intent
contains information that is necessary to obtain the base file. While receiving the file, you can expect the necessary information in the ClipData
property object Intent
. This information is
Android.Net.Uri
which is used with the help ContentResolver
to start the data stream. You can then save this data stream in the application as a local file. Depending on the application sending the data, you can also see the URI of the file to be sent to Extras Intent
Intent.ExtraStream
. Regardless of where it came from
Uri
, you must make sure that the file is saved in the application. In this case, you will be sure that the file will be fully accessible. All
OnCreate
shown below.App _mainForms;
protected override void OnCreate(Bundle bundle)
{
TabLayoutResource = Resource.Layout.Tabbar;
ToolbarResource = Resource.Layout.Toolbar;
base.OnCreate(bundle);
global::Xamarin.Forms.Forms.Init(this, bundle);
var mainForms = new App();
LoadApplication(mainForms);
if (Intent.Action == Intent.ActionSend)
{
// Пример данных хранящийхся в Extras
var uriFromExtras = Intent.GetParcelableExtra(Intent.ExtraStream) as Android.Net.Uri;
var subject = Intent.GetStringExtra(Intent.ExtraSubject);
// Получаем данные из ClipData
var pdf = Intent.ClipData.GetItemAt(0);
// Открываем поток из URI
var pdfStream = ContentResolver.OpenInputStream(pdf.Uri);
// Сохраняем
var memOfPdf = new System.IO.MemoryStream();
pdfStream.CopyTo(memOfPdf);
var docsPath = System.Environment.GetFolderPath(System.Environment.SpecialFolder.Personal);
var filePath = System.IO.Path.Combine(docsPath, "temp.pdf");
System.IO.File.WriteAllBytes(filePath, memOfPdf.ToArray());
mainForms.DisplayThePDF(filePath);
}
}
These are regular Xamarin.Forms
OnCreate
. The significant difference is that after calling Xamarin.Forms LoadApplication
, it checks to see if it has been loaded with Intent
information from another application by invoking an implicit Intent. 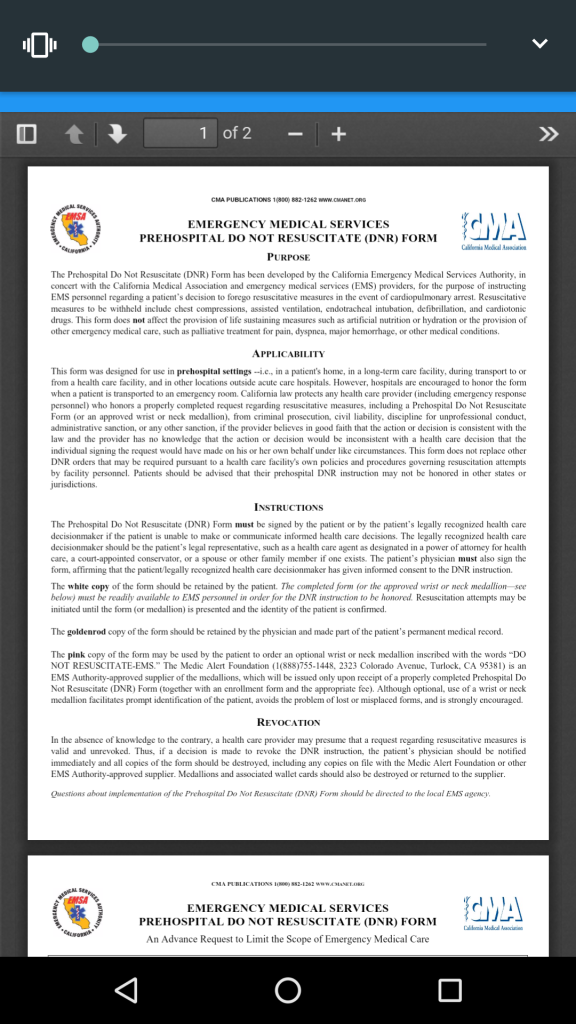
Intent
that receives the action Intent.ActionSend
(that is, the file is sent to the application), then the file is copied to the local storage of the application, then the function is called DisplayThePDF()
. DisplayThePDF()
displays a modal page in a web browser, and this web browser displays the downloaded PDF file. This browser works through a custom renderer. The code for this renderer is written using the Xamarin documentation . When everything is done, the Android application will display the PDF files as in the image on the right.
Total
In general, having understood how Android exchanges data between applications, which is similar to exchanging data between Activity components in one application, the task of receiving files in Android becomes easily solvable. You must declare an Intent filter in the class
MainActivity
in an Android project in Xamarin.Forms using IntentFilterAttribute
. After that, the corresponding markup is placed in the AndroidManifest.xml file, and now all you need to do is process the incoming data using a function OnCreate()
that, ideally, simply transfers the work of the function already existing in the Xamarin.Forms project.Thank you for the translation.
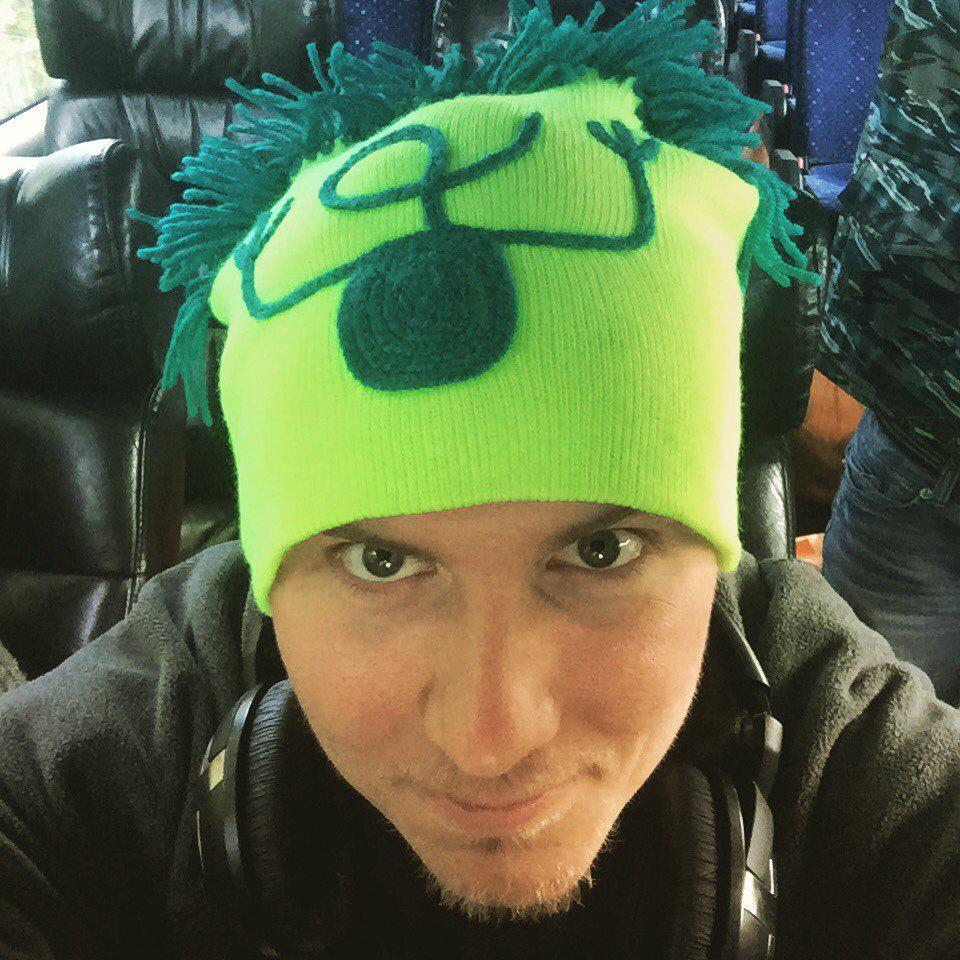
Leads the resource XamDev.ru and the community "Xamarin Developers" in social networks: VK , Facebook , Telegram .