
Intel Software Guard Extensions Tutorial Part 2, application device
- Transfer
The second part of the Intel Software Guard Extensions (Intel SGX) tutorial series is a general description of the application we will be developing: a simple password manager. Since we are creating this application from scratch, we can foresee the use of Intel SGX from the very beginning. This means that in addition to the requirements for the application, we will look at how the requirements for Intel SGX and the general architecture of the application influence each other.
Read the first part or a list of all the published training materials in the article Introducing the Intel Software Guard Extensions tutorial series .
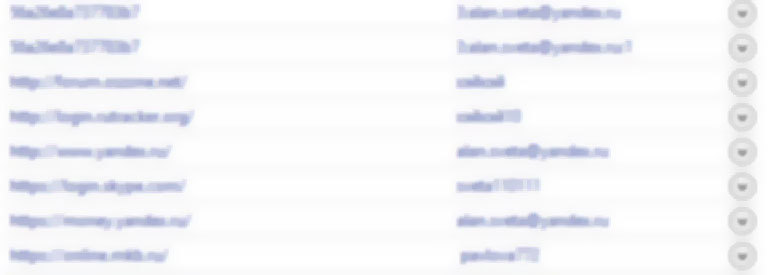
Users, as a rule, know what password managers are and what they are used for, but it’s always useful to go over the general information once more before dealing with the details of the application’s device.
The main goals of the password manager are:
Password management is an urgent problem for Internet users, and over the years several studies have been undertaken to solve this problem. A 2007 Microsoft study — almost ten years ago — indicated that each user had an average of 25 accounts that required passwords. Later, in 2014, Dashlane specialists calculated that their users in the USA had an average of 130 accounts, and the average number of accounts per user around the world was about 90. However, the problems do not end there: people, as a rule, very rarely choose strong passwords , often use the same password on many sites, which caused the scandalous attacks of malefactors. All these problems are caused by only two main factors: firstly, it is often difficult for people to remember strong passwords that are resistant to cracking; secondly, if you use more than one password, but many, the situation becomes even more difficult, because now, in addition to the passwords themselves, you must also remember which account each password is associated with.
When using the password manager, it’s enough to remember only one very strong passphrase to get access to the password database (password storage). After entering the password manager, you can find all the passwords stored there, copy and paste them into the authentication fields on the necessary sites. Of course, the main weak point of the password manager is the password database: this is a favorite target of attackers, since it stores all the user's passwords. Therefore, the password database is encrypted using strong encryption algorithms, and the main password phrase of the user is used to decrypt the data contained in them.
Our goal in this tutorial is to create a simple password manager that provides the same basic functions as similar commercial products, while adhering to security recommendations and using this program as an exercise to learn Intel SGX. I decided to call this training password manager “Tutorial Password Manager with Intel Software Guard Extensions” (yes, it’s not easy to pronounce, but the name speaks for itself). This program is not intended to be used as a commercial product and, of course, will not contain all the protective mechanisms inherent in commercial solutions, but this level of detail is not required for training.
Basic application requirements will help limit the scope of the application so that you can focus on Intel SGX integration, rather than on the intricacies of application design and development. Let me remind you that we are not faced with the task of creating a commercial product: the Tutorial Password Manager program with Intel SGX does not require the ability to run in many operating systems or on all possible CPU architectures. Only a valid starting point is required.
Therefore, the basic requirements for the application are as follows:
The first requirement may seem strange when you consider that this series of training materials is dedicated to developing applications using Intel SGX, but applications designed for the real world should include the ability to install on legacy systems. For some applications, it may be advisable to allow execution only on platforms that support Intel SGX, but in Tutorial Password Manager we have chosen a more flexible approach. A platform that supports Intel SGX will receive a more secure runtime, but the program will still work on other platforms. This usage model is quite suitable for the password manager, since users can synchronize their passwords with other, older systems. In addition, it is an opportunity to learn how to implement double code branches.
The second requirement gives us access to certain encryption algorithms in the code branch without Intel SGX support and to some libraries that we need. The requirement to use a 64-bit operating system simplifies application development by accessing 64-bit native code types. In addition, the speed of some encryption algorithms optimized for 64-bit code is increased.
The third requirement gives us access to the RDRAND instruction in the code branch without Intel SGX support. This greatly simplifies the generation of random numbers and provides access to a source with high entropy. Systems that support the RDSEED instruction can use it. (For instructions on RDRAND and RDSEED, seeIntel Random Number Generation Software Implementation Guide ).
The fourth requirement supports the shortest possible list of software required by the developer (and user). No third-party libraries, platforms, applications, or utilities are required to download and install. However, this requirement has a not too pleasant side effect: if you abandon third-party platforms, then we have only four options for creating a user interface. These options are:
The first two options are implemented using native / unmanaged code, and the last two options require .NET *.
We will develop a graphical user interface for the Tutorial Password Manager using the Windows Presentation Foundation in C #. This decision will affect our requirements as follows:
Why is it decided to use WPF? Mainly due to the fact that this simplifies the design of the user interface and introduces the level of complexity we need. In particular, since the interface relies on the .NET Framework, we get the opportunity to discuss the mixing of managed code and, in particular, high-level languages with enclave code. Note that choosing WPF instead of Windows Forms was completely arbitrary: our environment would work in either case.
As you may recall, enclaves must be written in native C or C ++ code, and the functions of the bridge interacting with the enclave must be native C functions (but not C ++). Both the Win32 and MFC APIs provide the ability to develop a password manager using completely native C / C ++ code, but the development tasks associated with these two methods are useless for developers seeking to learn application development using Intel SGX. Using a graphical user interface based on managed code, we get not only the benefits of integrated development tools, but also the opportunity to discuss certain issues that are useful for Intel SGX application developers. In short, you are not here to learn how to use MFC or Win32, but you might be interested in how to connect .NET with enclaves.
To bind managed and unmanaged code, we will use the C ++ / CLI (i.e. C ++, modified for Common Language Infrastructure). This greatly simplifies data packaging and is so convenient that many developers call this method “IJW” (It Just Works - it just works).

Figure 1. Minimal structures of the components of Intel Software Guard Extensions applications for native code and C #.
In fig. Figure 1 shows the effect on the minimum component composition of the Intel SGX application when moving from native code to C #. When using only native code, the application layer can directly interact with the enclave DLL, since the functions of the enclave bridge can be embedded in the application executable. In a mixed application, enclave bridge functions will need to be distinguished from the managed code block, since these functions must be completely native code. On the other hand, a C # application cannot directly interact with bridge functions, and in the C ++ / CLI model this means that you need to create another intermediate layer: a DLL library that transfers data between the managed C # application and the enclave bridge DLL that consists of only from native code.
The password manager is based on the password database, which we will also call the password store. This is an encrypted file that will contain information about the end user accounts and passwords. The basic requirements for our training application are as follows:
The requirement for storage portability means that we must be able to copy the storage file to another computer and still access its contents regardless of whether the other computer supports Intel SGX extensions. In other words, the capabilities of users should be the same: the password manager should work on any computer (naturally, if the hardware and OS comply with the specified system requirements).
Storage encryption during storage means that the storage file must be encrypted when it is not in active use. In this case, the storage must be encrypted on the disk (if there was no portability requirement, then the encryption requirement could be solved using the sealing function in Intel SGX) and should not be decrypted in RAM for longer than necessary.
Authenticated encryption ensures that the encrypted store has not been modified after encryption. Also, thanks to this, we get a convenient means of checking the user's passphrase: if the decryption key is incorrect, the decryption will fail when authenticating the authentication tag. In this case, we do not need to study the decrypted data to make sure that it is correct.
Any account information is confidential for various reasons, and last but not least, information about which accounts and sites can be attacked, but passwords are perhaps the most important information in the repository. Knowing what exactly needs to be attacked is, of course, good, but if you know the passwords, then you won’t need to attack at all, which is much better. Therefore, we introduce additional requirements for passwords stored in the repository:
This is nested multi-level encryption. The passwords of all user accounts are encrypted when placed in the store, and the store itself is encrypted when written to disk. This approach reduces the vulnerability of passwords after decrypting the repository. It is advisable to decrypt the entire store so that the user can view all their credentials, but displaying all passwords in plain text is hardly acceptable.
The account password is decrypted only when the user requires it. This limits the vulnerability of the password in RAM and on the screen.
Since our encryption requirements are already defined, it is time to choose certain encryption algorithms, and in this regard, the existing requirements for our application significantly limit the available options. The Tutorial Password Manager program should work both on platforms that support Intel SGX, and without Intel SGX, while third-party libraries are prohibited. This means that you must select the algorithm, key size, and authentication tag size that are supported by both the Windows CNG API and Intel SGX trusted encryption library. In practice, this means that we have only one possible algorithm: AES-GCM with a 128-bit key. This is probably not the best encryption mode for use in the application, especially sincethe effective strength of the 128-bit GCM authentication tag is less than 128 bits , but for our purposes this will be enough. Remember: our task is not to create a commercial product, but a tutorial on using Intel SGX.
The choice of GCM affects other encryption characteristics in our application, in particular, the length of the initialization vector (12 bytes is the optimal value for this algorithm) and the authenticity tag.
After selecting the encryption algorithm, you can go to the encryption key and user authentication. How does a user authenticate with password manager to open their vault?
The easiest way is to generate the encryption key directly from the user’s passphrase or password using the key generation function (KDF). This simple approach is fully functional, but it has one significant drawback: if the user changes the password, then the encryption key will also change with it. Instead, we take a more common approach and encrypt the encryption key.
In this case, the primary encryption key is generated randomly based on a source with high entropy and never changes. The user passphrase or password is used to generate the secondary encryption key, and this secondary key is used to encrypt the primary key. This approach has several important advantages:
Not all of these features are important for Tutorial Password Manager, but it is a smart security practice.
Here, the primary key is called the storage key, and the secondary key, formed from the user's passphrase, is called the master key. The user authenticates by entering their passphrase, and the password manager generates a master key from it. If the master key successfully decrypts the storage key, then the user passes the verification and the storage can be decrypted. If the passphrase is incorrect, decryption of the storage key is not possible, which does not allow decrypting the storage.
The last requirement regarding the creation of the key generation function based on the SHA-256 algorithm is due to a limitation arising from the need to use the hashing algorithm, which is supported both in the Windows CNG API and in the Intel SGX encryption library.
The last of the basic requirements relates to what exactly is placed in the repository. In this tutorial, we will follow a simple path. In fig. Figure 2 shows the layout of the main user interface window.

Figure 2. Preliminary layout of the main Tutorial Password Manager window.
The final requirement is code simplification. If you fix the number of accounts in the repository, you can more easily determine the upper limit of the size of the repository. This will be important when designing an enclave. Of course, real password managers are deprived of such luxury, but in the tutorial we can quite afford it.
In the third part of this tutorial, we’ll take a closer look at the Tutorial Password Manager app for Intel SGX. We will define secrets, decide which parts of the application should be inside the enclave, how the enclave will interact with the main application and how the enclave affects the object model. Follow the news!
Read the first part of the tutorial in this series, Intel Software Guard Extensions> Tutorial: Part 1, Intel SGX Foundation , or a list of all published training materials in the article Introducing the Intel Software Guard Extensions tutorial series .
Read the first part or a list of all the published training materials in the article Introducing the Intel Software Guard Extensions tutorial series .
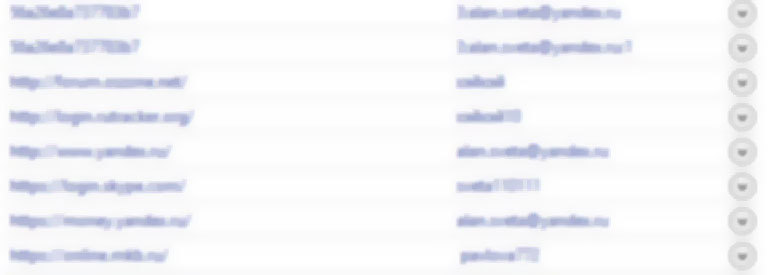
Password Managers - Short Description
Users, as a rule, know what password managers are and what they are used for, but it’s always useful to go over the general information once more before dealing with the details of the application’s device.
The main goals of the password manager are:
- Reduce the number of passwords that the user needs to remember.
- Providing end users with the ability to create stronger passwords than they could think of on their own.
- Improve the usability of different passwords for each account.
Password management is an urgent problem for Internet users, and over the years several studies have been undertaken to solve this problem. A 2007 Microsoft study — almost ten years ago — indicated that each user had an average of 25 accounts that required passwords. Later, in 2014, Dashlane specialists calculated that their users in the USA had an average of 130 accounts, and the average number of accounts per user around the world was about 90. However, the problems do not end there: people, as a rule, very rarely choose strong passwords , often use the same password on many sites, which caused the scandalous attacks of malefactors. All these problems are caused by only two main factors: firstly, it is often difficult for people to remember strong passwords that are resistant to cracking; secondly, if you use more than one password, but many, the situation becomes even more difficult, because now, in addition to the passwords themselves, you must also remember which account each password is associated with.
When using the password manager, it’s enough to remember only one very strong passphrase to get access to the password database (password storage). After entering the password manager, you can find all the passwords stored there, copy and paste them into the authentication fields on the necessary sites. Of course, the main weak point of the password manager is the password database: this is a favorite target of attackers, since it stores all the user's passwords. Therefore, the password database is encrypted using strong encryption algorithms, and the main password phrase of the user is used to decrypt the data contained in them.
Our goal in this tutorial is to create a simple password manager that provides the same basic functions as similar commercial products, while adhering to security recommendations and using this program as an exercise to learn Intel SGX. I decided to call this training password manager “Tutorial Password Manager with Intel Software Guard Extensions” (yes, it’s not easy to pronounce, but the name speaks for itself). This program is not intended to be used as a commercial product and, of course, will not contain all the protective mechanisms inherent in commercial solutions, but this level of detail is not required for training.
Basic Application Requirements
Basic application requirements will help limit the scope of the application so that you can focus on Intel SGX integration, rather than on the intricacies of application design and development. Let me remind you that we are not faced with the task of creating a commercial product: the Tutorial Password Manager program with Intel SGX does not require the ability to run in many operating systems or on all possible CPU architectures. Only a valid starting point is required.
Therefore, the basic requirements for the application are as follows:
Decision requirements and operating principles |
|
The first requirement may seem strange when you consider that this series of training materials is dedicated to developing applications using Intel SGX, but applications designed for the real world should include the ability to install on legacy systems. For some applications, it may be advisable to allow execution only on platforms that support Intel SGX, but in Tutorial Password Manager we have chosen a more flexible approach. A platform that supports Intel SGX will receive a more secure runtime, but the program will still work on other platforms. This usage model is quite suitable for the password manager, since users can synchronize their passwords with other, older systems. In addition, it is an opportunity to learn how to implement double code branches.
The second requirement gives us access to certain encryption algorithms in the code branch without Intel SGX support and to some libraries that we need. The requirement to use a 64-bit operating system simplifies application development by accessing 64-bit native code types. In addition, the speed of some encryption algorithms optimized for 64-bit code is increased.
The third requirement gives us access to the RDRAND instruction in the code branch without Intel SGX support. This greatly simplifies the generation of random numbers and provides access to a source with high entropy. Systems that support the RDSEED instruction can use it. (For instructions on RDRAND and RDSEED, seeIntel Random Number Generation Software Implementation Guide ).
The fourth requirement supports the shortest possible list of software required by the developer (and user). No third-party libraries, platforms, applications, or utilities are required to download and install. However, this requirement has a not too pleasant side effect: if you abandon third-party platforms, then we have only four options for creating a user interface. These options are:
- Win32 APIs
- Microsoft Foundation Classes (MFC)
- Windows Presentation Foundation (WPF)
- Windows forms
The first two options are implemented using native / unmanaged code, and the last two options require .NET *.
User interface platform
We will develop a graphical user interface for the Tutorial Password Manager using the Windows Presentation Foundation in C #. This decision will affect our requirements as follows:
Decision requirements and operating principles |
|
Why is it decided to use WPF? Mainly due to the fact that this simplifies the design of the user interface and introduces the level of complexity we need. In particular, since the interface relies on the .NET Framework, we get the opportunity to discuss the mixing of managed code and, in particular, high-level languages with enclave code. Note that choosing WPF instead of Windows Forms was completely arbitrary: our environment would work in either case.
As you may recall, enclaves must be written in native C or C ++ code, and the functions of the bridge interacting with the enclave must be native C functions (but not C ++). Both the Win32 and MFC APIs provide the ability to develop a password manager using completely native C / C ++ code, but the development tasks associated with these two methods are useless for developers seeking to learn application development using Intel SGX. Using a graphical user interface based on managed code, we get not only the benefits of integrated development tools, but also the opportunity to discuss certain issues that are useful for Intel SGX application developers. In short, you are not here to learn how to use MFC or Win32, but you might be interested in how to connect .NET with enclaves.
To bind managed and unmanaged code, we will use the C ++ / CLI (i.e. C ++, modified for Common Language Infrastructure). This greatly simplifies data packaging and is so convenient that many developers call this method “IJW” (It Just Works - it just works).

Figure 1. Minimal structures of the components of Intel Software Guard Extensions applications for native code and C #.
In fig. Figure 1 shows the effect on the minimum component composition of the Intel SGX application when moving from native code to C #. When using only native code, the application layer can directly interact with the enclave DLL, since the functions of the enclave bridge can be embedded in the application executable. In a mixed application, enclave bridge functions will need to be distinguished from the managed code block, since these functions must be completely native code. On the other hand, a C # application cannot directly interact with bridge functions, and in the C ++ / CLI model this means that you need to create another intermediate layer: a DLL library that transfers data between the managed C # application and the enclave bridge DLL that consists of only from native code.
Password Store Requirements
The password manager is based on the password database, which we will also call the password store. This is an encrypted file that will contain information about the end user accounts and passwords. The basic requirements for our training application are as follows:
Decision requirements and operating principles |
|
The requirement for storage portability means that we must be able to copy the storage file to another computer and still access its contents regardless of whether the other computer supports Intel SGX extensions. In other words, the capabilities of users should be the same: the password manager should work on any computer (naturally, if the hardware and OS comply with the specified system requirements).
Storage encryption during storage means that the storage file must be encrypted when it is not in active use. In this case, the storage must be encrypted on the disk (if there was no portability requirement, then the encryption requirement could be solved using the sealing function in Intel SGX) and should not be decrypted in RAM for longer than necessary.
Authenticated encryption ensures that the encrypted store has not been modified after encryption. Also, thanks to this, we get a convenient means of checking the user's passphrase: if the decryption key is incorrect, the decryption will fail when authenticating the authentication tag. In this case, we do not need to study the decrypted data to make sure that it is correct.
Passwords
Any account information is confidential for various reasons, and last but not least, information about which accounts and sites can be attacked, but passwords are perhaps the most important information in the repository. Knowing what exactly needs to be attacked is, of course, good, but if you know the passwords, then you won’t need to attack at all, which is much better. Therefore, we introduce additional requirements for passwords stored in the repository:
Decision requirements and operating principles |
|
This is nested multi-level encryption. The passwords of all user accounts are encrypted when placed in the store, and the store itself is encrypted when written to disk. This approach reduces the vulnerability of passwords after decrypting the repository. It is advisable to decrypt the entire store so that the user can view all their credentials, but displaying all passwords in plain text is hardly acceptable.
The account password is decrypted only when the user requires it. This limits the vulnerability of the password in RAM and on the screen.
Encryption algorithms
Since our encryption requirements are already defined, it is time to choose certain encryption algorithms, and in this regard, the existing requirements for our application significantly limit the available options. The Tutorial Password Manager program should work both on platforms that support Intel SGX, and without Intel SGX, while third-party libraries are prohibited. This means that you must select the algorithm, key size, and authentication tag size that are supported by both the Windows CNG API and Intel SGX trusted encryption library. In practice, this means that we have only one possible algorithm: AES-GCM with a 128-bit key. This is probably not the best encryption mode for use in the application, especially sincethe effective strength of the 128-bit GCM authentication tag is less than 128 bits , but for our purposes this will be enough. Remember: our task is not to create a commercial product, but a tutorial on using Intel SGX.
The choice of GCM affects other encryption characteristics in our application, in particular, the length of the initialization vector (12 bytes is the optimal value for this algorithm) and the authenticity tag.
Decision requirements and operating principles |
|
Encryption Keys and User Authentication
After selecting the encryption algorithm, you can go to the encryption key and user authentication. How does a user authenticate with password manager to open their vault?
The easiest way is to generate the encryption key directly from the user’s passphrase or password using the key generation function (KDF). This simple approach is fully functional, but it has one significant drawback: if the user changes the password, then the encryption key will also change with it. Instead, we take a more common approach and encrypt the encryption key.
In this case, the primary encryption key is generated randomly based on a source with high entropy and never changes. The user passphrase or password is used to generate the secondary encryption key, and this secondary key is used to encrypt the primary key. This approach has several important advantages:
- The data does not have to be re-encrypted when the user password or passphrase changes.
- The encryption key never changes, so it can, for example, be written on paper in hexadecimal format and stored in a physically safe place. In this case, the data can be decrypted even if the user forgets the password. Since the key never changes, it is enough to write it down only once.
- Theoretically, you can provide data access to multiple users. Each user will encrypt a copy of the primary key with their own passphrase.
Not all of these features are important for Tutorial Password Manager, but it is a smart security practice.
Decision requirements and operating principles |
|
Here, the primary key is called the storage key, and the secondary key, formed from the user's passphrase, is called the master key. The user authenticates by entering their passphrase, and the password manager generates a master key from it. If the master key successfully decrypts the storage key, then the user passes the verification and the storage can be decrypted. If the passphrase is incorrect, decryption of the storage key is not possible, which does not allow decrypting the storage.
The last requirement regarding the creation of the key generation function based on the SHA-256 algorithm is due to a limitation arising from the need to use the hashing algorithm, which is supported both in the Windows CNG API and in the Intel SGX encryption library.
Account information
The last of the basic requirements relates to what exactly is placed in the repository. In this tutorial, we will follow a simple path. In fig. Figure 2 shows the layout of the main user interface window.
Decision requirements and operating principles |
|

Figure 2. Preliminary layout of the main Tutorial Password Manager window.
The final requirement is code simplification. If you fix the number of accounts in the repository, you can more easily determine the upper limit of the size of the repository. This will be important when designing an enclave. Of course, real password managers are deprived of such luxury, but in the tutorial we can quite afford it.
In future releases
In the third part of this tutorial, we’ll take a closer look at the Tutorial Password Manager app for Intel SGX. We will define secrets, decide which parts of the application should be inside the enclave, how the enclave will interact with the main application and how the enclave affects the object model. Follow the news!
Read the first part of the tutorial in this series, Intel Software Guard Extensions> Tutorial: Part 1, Intel SGX Foundation , or a list of all published training materials in the article Introducing the Intel Software Guard Extensions tutorial series .