
ASP.NET Core: Creating a Web Service Front End for an Application
- Tutorial
We continue to share material on working with ASP.NET Core. In a previous article, we talked about deploying an ASP.NET Core application on Nano Server with IIS . Today we’ll talk about creating an external web service interface for your application.

1. ASP.NET Core on Nano Server .
2. Create a web service front end for the application .
3. Creation of the first web API using ASP.NET Core MVC and Visual Studio .
4. Deploy a web application to Azure Application Service using Visual Studio .
5. Your first Mac application using Visual Studio Code .
By default, Azure Service Fabric services do not provide a public interface for web services. In order to make the application functional for HTTP clients, it is necessary to create a web project that will work as an entry point and exchange data with individual services.
In this article, we will add a web service for the stateful counter service to the Visual Studio application. If you are not familiar with the basic process of creating applications in Visual Studio, here you are .
ASP.NET Core is a simple cross-platform web development tool with which you can create modern web user interfaces and web APIs. Let's add an ASP.NET web API project to an existing application:
1. With the application project open in Solution Explorer, right-click on Services and select Add> New Service Fabric Service .

2. On the Create a Service page, select ASP.NET Core and specify a name for this service.

3. The next page shows the ASP.NET Core project template set. Note that these are the same templates that are used when creating an ASP.NET Core project outside of the Service Fabric application. In this guide, we will use the Web API . However, you can use these methods to create a full-fledged web application.
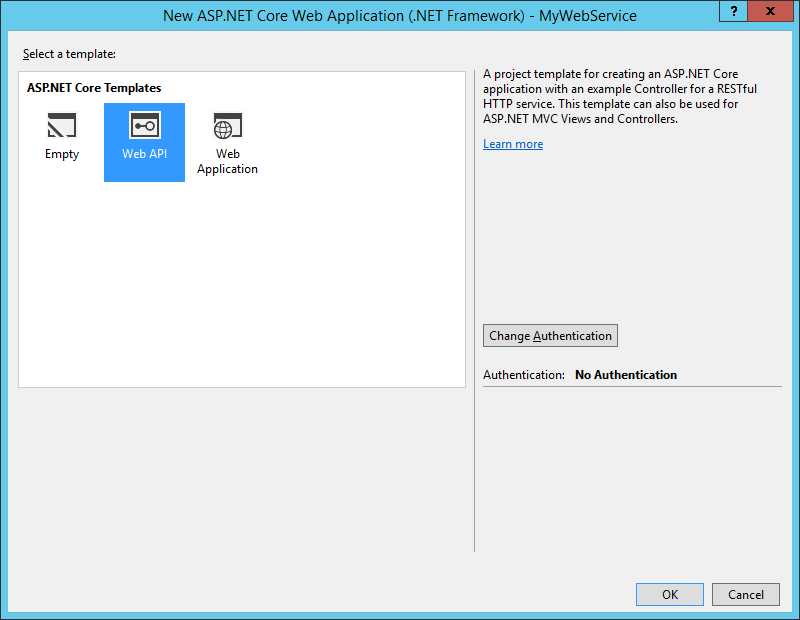
Note: for further work, you must install .NET Core 1.0 .
After creating the web API, the application will have two services. As you refine the application, you can add new services in the same way. Each of these services can be updated separately, including to a specific version.
Note: For more information on creating ASP.NET Core services, see the ASP.NET Core documentation..
To better understand the steps we have taken, let's deploy a new application and look at the default behavior associated with using the ASP.NET Core web API template:
1. To debug the application, press F5 in Visual Studio.
2. After the deployment is complete, Visual Studio launches a browser with the root directory of the ASP.NET Web API service, for example localhost : 33003. The port number is assigned arbitrarily and may vary. The web API template does not provide default behavior for the root directory, resulting in an error message in the browser.
3. Add a path to the address in the browser

After completing the steps in this guide, the default values will be replaced with the last value of the stateful service counter.
Service Fabric provides the freedom to interact with Reliable Services. In one application there can be both services available over TCP, and services available over the HTTP REST API, as well as services available over web sockets (for possible options and the selection of the ideal ratio, see the article ). Here we will use one of the simplest approaches and use the classes
An approach using
Let's start by creating an interface that will act as a contract between a stateful service and its clients, including the ASP.NET Core project:
1. In the solution explorer, right-click on the solution and select Add> New Project .
2. In the left navigation pane, select Visual C # , then select the Class Library template . Verify that the .NET Framework 4.5.2 is selected .

3. For the interface to be used
4. Locate the Microsoft.ServiceFabric.Services package and install it.

5. In the class library, create an interface using a single interface
Now that the interface is defined, you need to implement it in the state-tracking service:
1. In the state-tracking service, add a link to the class library project that contains this interface.

2. Find a class that inherits from
3. Now implement the single method specified in the interface
After the interface is implemented, the
Note: The equivalent of a method for opening a communication channel for a stateless service is
In our example, we will replace the existing method
The state-prepared service we prepared is ready to receive traffic from other services. Therefore, it remains only to add code to interact with it from the ASP.NET web service:
1. In the ASP.NET project, add a reference to the class library that contains the interface
2. From the Build menu, open Configuration Manager . You should see something like the following:

Note that for the MyStatefulService.Interface class library project, Any CPU is selected. To work correctly with Service Fabric, a 64-bit platform must be used. Open the Platform drop-down menu, select New and configure the 64-bit platform:

3. Add the Microsoft.ServiceFabric.Services package to the ASP.NET project, as you did previously for the class library project. A class will be added
4. In the Controllers folder, open the class
The first line of code is the key. To add an ICounter proxy to stateful services, you must provide two types of data: the section identifier and the service name.
Partitioning can be used to scale state-tracking services: you can split their state into different segments based on the key you specify, for example, customer ID or zip code. In this application, a stateful service has only one partition, so the key does not matter. Any key specified will result in the same section. For more information on creating partitions, see the article .
The service name is a unique resource code in the format fabric: //.
With this information, Service Fabric can accurately identify the computer to which the request should be sent. The class
After creating the proxy server, you must call the GetCountAsync method and get its result.
5. Press the F5 key to start the modified application. As last time, the browser will automatically start and the root directory of the web project will load. Add the path “api / values”, the current return value of the counter will be displayed:
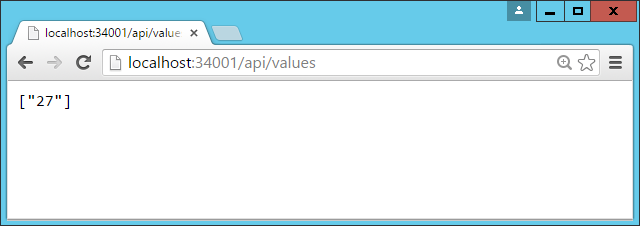
Refresh your browser to track changes in the counter.
It is important to remember that the ASP.NET Core web server specified in the template and known as Kestrel does not support the processing of direct Internet traffic . For operational scenarios, consider hosting ASP.NET Core endpoints behind an API Management service or other gateway with Internet access. Note that Service Fabric is not supported for deployment on IIS.
Here we describe the addition of a web interface to provide communication with a stateful service. However, a similar model can be used to exchange data with subjects. It is even easier.
When you create a subject project in Visual Studio, an interface project is automatically created. This interface can be used to create a proxy in the web project for the subject to interact with the subject. A communication channel is provided automatically. Therefore, you do not need to take any configuration steps
Deployed on a local cluster, Service Fabric can typically be deployed to a cluster with multiple computers. This app will work properly. This is because the local cluster is a five-node configuration located on the same computer.
However, one key feature is associated with web services. If the cluster is not included in the load balancer as provided in the Azure environment, you need to make sure that web services are deployed on each computer. This is necessary because the load balancer will simply cycle through traffic between computers. This can be done by specifying the
When running a web service locally, on the contrary, you need to make sure that only one instance of the service is running. Otherwise, conflicts will arise with several processes that are listening on the same path and port. Therefore, for local deployments, the web service instance counter must be set to "1".
More information on setting different values for different environments can be found in the article .

The first series of articles on ASP.NET Core
1. ASP.NET Core on Nano Server .
2. Create a web service front end for the application .
3. Creation of the first web API using ASP.NET Core MVC and Visual Studio .
4. Deploy a web application to Azure Application Service using Visual Studio .
5. Your first Mac application using Visual Studio Code .
By default, Azure Service Fabric services do not provide a public interface for web services. In order to make the application functional for HTTP clients, it is necessary to create a web project that will work as an entry point and exchange data with individual services.
In this article, we will add a web service for the stateful counter service to the Visual Studio application. If you are not familiar with the basic process of creating applications in Visual Studio, here you are .
Adding an ASP.NET Core Service to an Application
ASP.NET Core is a simple cross-platform web development tool with which you can create modern web user interfaces and web APIs. Let's add an ASP.NET web API project to an existing application:
1. With the application project open in Solution Explorer, right-click on Services and select Add> New Service Fabric Service .

2. On the Create a Service page, select ASP.NET Core and specify a name for this service.

3. The next page shows the ASP.NET Core project template set. Note that these are the same templates that are used when creating an ASP.NET Core project outside of the Service Fabric application. In this guide, we will use the Web API . However, you can use these methods to create a full-fledged web application.
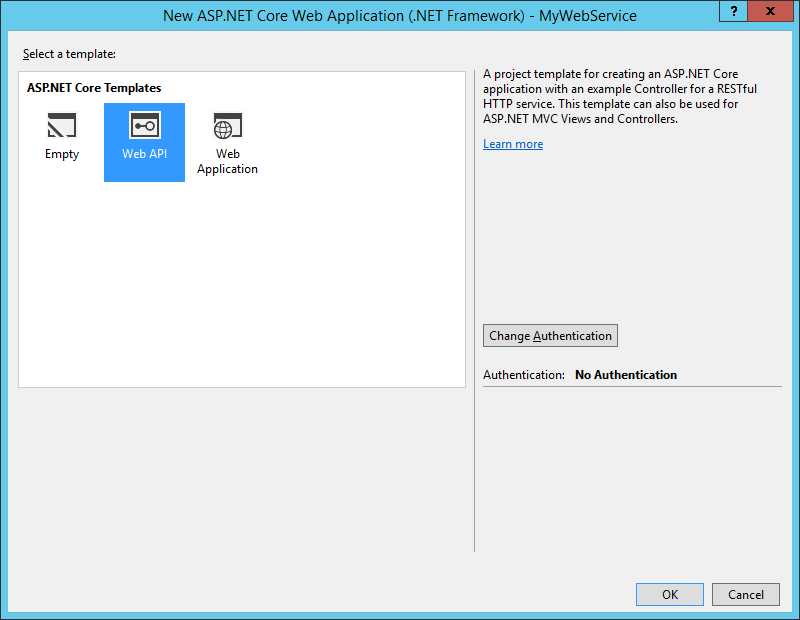
Note: for further work, you must install .NET Core 1.0 .
After creating the web API, the application will have two services. As you refine the application, you can add new services in the same way. Each of these services can be updated separately, including to a specific version.
Note: For more information on creating ASP.NET Core services, see the ASP.NET Core documentation..
Application launch
To better understand the steps we have taken, let's deploy a new application and look at the default behavior associated with using the ASP.NET Core web API template:
1. To debug the application, press F5 in Visual Studio.
2. After the deployment is complete, Visual Studio launches a browser with the root directory of the ASP.NET Web API service, for example localhost : 33003. The port number is assigned arbitrarily and may vary. The web API template does not provide default behavior for the root directory, resulting in an error message in the browser.
3. Add a path to the address in the browser
/api/values
. This will call the methodGet
ValuesController object in the web API template. It will return the default response provided by the template, a JSON array containing two lines: 
After completing the steps in this guide, the default values will be replaced with the last value of the stateful service counter.
Service Connection
Service Fabric provides the freedom to interact with Reliable Services. In one application there can be both services available over TCP, and services available over the HTTP REST API, as well as services available over web sockets (for possible options and the selection of the ideal ratio, see the article ). Here we will use one of the simplest approaches and use the classes
ServiceProxy/ServiceRemotingListener
provided in the SDK. An approach using
ServiceProxy
(based on remote procedure calls or RPC) defines an interface that acts as a public service contract. This interface is then used to create a proxy class to interact with the service.Interface Creation
Let's start by creating an interface that will act as a contract between a stateful service and its clients, including the ASP.NET Core project:
1. In the solution explorer, right-click on the solution and select Add> New Project .
2. In the left navigation pane, select Visual C # , then select the Class Library template . Verify that the .NET Framework 4.5.2 is selected .

3. For the interface to be used
ServiceProxy
, it must be derived from the IService interface. This interface is included in one of the NuGet Service Fabric packages. To add a package, right-click on the class library project and select Manage NuGet Packages .4. Locate the Microsoft.ServiceFabric.Services package and install it.

5. In the class library, create an interface using a single interface
GetCountAsync
and extend the interface from IService.namespace MyStatefulService.Interfaces
{
using Microsoft.ServiceFabric.Services.Remoting;
public interface ICounter: IService
{
Task GetCountAsync();
}
}
Implementing an interface in a stateful service
Now that the interface is defined, you need to implement it in the state-tracking service:
1. In the state-tracking service, add a link to the class library project that contains this interface.

2. Find a class that inherits from
StatefulService
, for example MyStatefulService
, and extend it to implement the interface ICounter
.using MyStatefulService.Interfaces;
...
public class MyStatefulService : StatefulService, ICounter
{
// ...
}
3. Now implement the single method specified in the interface
ICounter
, - GetCountAsync
.public async Task GetCountAsync()
{
var myDictionary =
await this.StateManager.GetOrAddAsync>("myDictionary");
using (var tx = this.StateManager.CreateTransaction())
{
var result = await myDictionary.TryGetValueAsync(tx, "Counter");
return result.HasValue ? result.Value : 0;
}
}
Providing a stateful service with a service remoting listener
After the interface is implemented, the
ICounter
last step in making it possible to call a service with state monitoring from other services is to open a communication channel. For stateful services, Service Fabric provides an overridable method called CreateServiceReplicaListeners
. Using this method, you can specify one or more communication listeners based on the type of communication that you want to use in the service. Note: The equivalent of a method for opening a communication channel for a stateless service is
CreateServiceInstanceListeners
. In our example, we will replace the existing method
CreateServiceReplicaListeners
and use the instance ServiceRemotingListener
that creates the RPC endpoint that the clients call with ServiceProxy
.using Microsoft.ServiceFabric.Services.Remoting.Runtime;
...
protected override IEnumerable CreateServiceReplicaListeners()
{
return new List()
{
new ServiceReplicaListener(
(context) =>
this.CreateServiceRemotingListener(context))
};
}
Using the ServiceProxy Class to Interact with a Service
The state-prepared service we prepared is ready to receive traffic from other services. Therefore, it remains only to add code to interact with it from the ASP.NET web service:
1. In the ASP.NET project, add a reference to the class library that contains the interface
ICounter
. 2. From the Build menu, open Configuration Manager . You should see something like the following:

Note that for the MyStatefulService.Interface class library project, Any CPU is selected. To work correctly with Service Fabric, a 64-bit platform must be used. Open the Platform drop-down menu, select New and configure the 64-bit platform:

3. Add the Microsoft.ServiceFabric.Services package to the ASP.NET project, as you did previously for the class library project. A class will be added
ServiceProxy
. 4. In the Controllers folder, open the class
ValuesController
. Please note that at the moment the method Get
returns a built-in string array of values value1 and value2, corresponding to those that we saw earlier in the browser. Replace this implementation with the following code:using MyStatefulService.Interfaces;
using Microsoft.ServiceFabric.Services.Remoting.Client;
...
public async Task> Get()
{
ICounter counter =
ServiceProxy.Create(new Uri("fabric:/MyApplication/MyStatefulService"), new ServicePartitionKey(0));
long count = await counter.GetCountAsync();
return new string[] { count.ToString() };
}
The first line of code is the key. To add an ICounter proxy to stateful services, you must provide two types of data: the section identifier and the service name.
Partitioning can be used to scale state-tracking services: you can split their state into different segments based on the key you specify, for example, customer ID or zip code. In this application, a stateful service has only one partition, so the key does not matter. Any key specified will result in the same section. For more information on creating partitions, see the article .
The service name is a unique resource code in the format fabric: /
With this information, Service Fabric can accurately identify the computer to which the request should be sent. The class
ServiceProxy
also consistently switches operations to another computer if a failure occurs on the computer that hosts the status-tracking service section. This abstraction makes it easy to write client code for working with other services. After creating the proxy server, you must call the GetCountAsync method and get its result.
5. Press the F5 key to start the modified application. As last time, the browser will automatically start and the root directory of the web project will load. Add the path “api / values”, the current return value of the counter will be displayed:
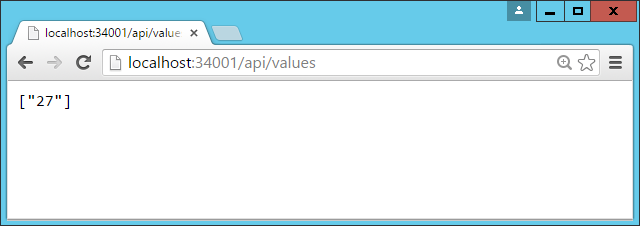
Refresh your browser to track changes in the counter.
It is important to remember that the ASP.NET Core web server specified in the template and known as Kestrel does not support the processing of direct Internet traffic . For operational scenarios, consider hosting ASP.NET Core endpoints behind an API Management service or other gateway with Internet access. Note that Service Fabric is not supported for deployment on IIS.
Exchange of data with subjects
Here we describe the addition of a web interface to provide communication with a stateful service. However, a similar model can be used to exchange data with subjects. It is even easier.
When you create a subject project in Visual Studio, an interface project is automatically created. This interface can be used to create a proxy in the web project for the subject to interact with the subject. A communication channel is provided automatically. Therefore, you do not need to take any configuration steps
ServiceRemotingListener
, as is the case with stateful tracking services.How web services work on a local cluster
Deployed on a local cluster, Service Fabric can typically be deployed to a cluster with multiple computers. This app will work properly. This is because the local cluster is a five-node configuration located on the same computer.
However, one key feature is associated with web services. If the cluster is not included in the load balancer as provided in the Azure environment, you need to make sure that web services are deployed on each computer. This is necessary because the load balancer will simply cycle through traffic between computers. This can be done by specifying the
InstanceCount
special value “−1” for the service parameter .When running a web service locally, on the contrary, you need to make sure that only one instance of the service is running. Otherwise, conflicts will arise with several processes that are listening on the same path and port. Therefore, for local deployments, the web service instance counter must be set to "1".
More information on setting different values for different environments can be found in the article .