
Working with Azure IoT devices from UWP applications
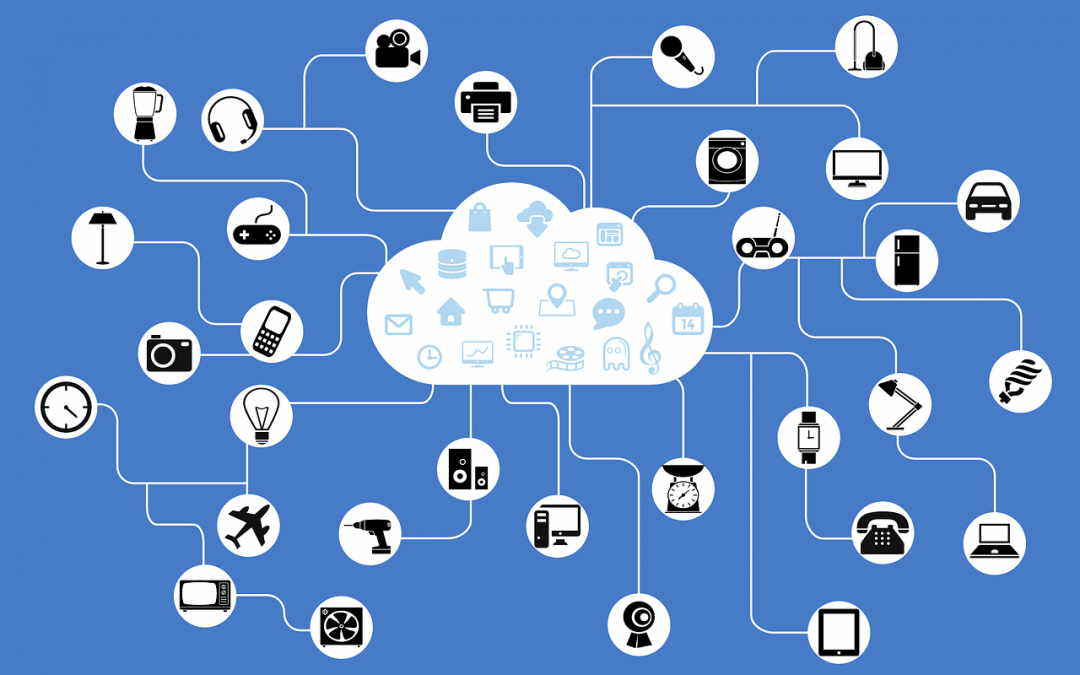
Continuing the article Sending data from Arduino to the Azure IoT Hub, I’ll now talk about how you can read and send data to the IoT Hub of the Azure cloud from a UWP application. This is done using the Microsoft.Azure.Devices.Client client library. You can use Device Explorer or iothub-explorer to monitor these sent to the message cloud .
In addition, I’ll talk about how to create a simple UWP application that sends data from the cloud to the device. Finally, I will give an example of how you can get a message from the Azure IoT hub on the Arduino MKR1000.
Simulate an Azure IoT device using a UWP application
Download the Connected Service for Azure IoT Hub (current version 1.5). Install. We create a universal application project. Add a link to the connected service.
Click "Configure". We will be offered 2 options to choose from.
The first option is classic. In case we have a regular project without special security requirements. The connection string to the IoT hub will be stored in code.
The second option is experimental. The device is registered on the Windows Device Portal. Then, after choosing the “TPM configuration” item in the menu, you need to install TPM (Trusted Platform Module) on the device and enter the key data from the Azure hub. As a result, the device will not store the primary Azure access key. Instead, the TPM device will generate short-lived SAS tokens.
Choosing the first option and entering the Azure user credentials, we get the hub selection window:
In my case, I don’t have to choose much, since I created only one hub. I add it.
I get an invitation to choose a device. Again, in my case, only one device is registered (I use the free features of Azure)
After selecting the device, the various necessary packages are
installed : Upon completion of the installation, a page with a manual will open that offers you to use:
SendDeviceToCloudMessageAsync()
to send messages. And to receive messages:
ReceiveCloudToDeviceMessageAsync()
Add a button and check if we get a message sent using DeviceExplorer:
private async void btnCheck_Click(object sender, RoutedEventArgs e)
{
string message = await AzureIoTHub.ReceiveCloudToDeviceMessageAsync();
}
If everything should be clear with the receipt, then when sending messages using SendDeviceToCloudMessageAsync, the same line of text is always sent. Consider the code that is in the AzureIoTHub.cs file:
public static async Task SendDeviceToCloudMessageAsync()
{
var deviceClient = DeviceClient.CreateFromConnectionString(deviceConnectionString, TransportType.Amqp);
#if WINDOWS_UWP
var str = "Hello, Cloud from a UWP C# app!";
#else
var str = "Hello, Cloud from a C# app!";
#endif
var message = new Message(Encoding.ASCII.GetBytes(str));
await deviceClient.SendEventAsync(message);
}
Initially, I did not quite understand why Task does not accept a string of text as a parameter to send it exactly, but sends a hard-coded value of "Hello ...". It turned out that this was done specifically so that the developers do not copy the code, but modify it. So we can take the creation of the deviceClient object somewhere into a separate method and call it in the right place for us (when loading the page, for example). Well, the task that sends the message, we can already make the accepting parameter. By the way, I managed to send a message in UTF8 encoding:
public static async Task SendDeviceToCloudMessageAsync(string texttosend)
{
var message = new Message(Encoding.UTF8.GetBytes(texttosend));
await deviceClient.SendEventAsync(message);
}
Now you can use Device Explorer to receive a message or send it to the UWP application.
"Link to the English article: Connect your Windows app to Azure IoT Hub with Visual Studio
" Link to the GitHub page of the Connected Service for Azure IoT Hub project (if something suddenly goes wrong, there is room for submitting a bug)
Once again I’ll clarify what might happen confusion. You can send a message both from the device to the cloud, and from the cloud to the device. Let me give you an example of a UWP application, which this time sends a message from the cloud to the device.
Send a message from the cloud to the device using the UWP application
In the NuGet package manager, you need to find the package by the phrase Microsoft.Azure.Devices and install it. Just in case, direct link: Microsoft Azure IoT Service SDK
Add namespaces:
using Microsoft.Azure.Devices;
using System.Threading.Tasks;
using System.Text;
And the following variables:
static ServiceClient serviceClient;
static string connectionString = "{строка подключения iot hub}";
Where the connection string is taken from the Azure portal:
We need a method that sends text to the device
private async static Task SendCloudToDeviceMessageAsync()
{
var commandMessage = new Message(Encoding.UTF8.GetBytes("light on"));
// даем команду включить светодиод
await serviceClient.SendAsync("pseudoDevice", commandMessage);
}
Here pseudoDevice is the id of the device to which the message will be sent.
It remains to add in MainPage after this.InitializeComponent ():
serviceClient = ServiceClient.CreateFromConnectionString(connectionString);
And somewhere in the event of pressing a button, you can call a task sending a message to the device:
private async void Button_Click(object sender, RoutedEventArgs e)
{
await SendCloudToDeviceMessageAsync();
}
Our UWP application is ready. Now you can send the “light on” command to our device.
Receiving a message from the Azure IoT hub Arduino MKR1000 board
Using the following sketch, you can get a message from the hub. In case a message is received with the text “light on”, the Arduino MKR1000 will turn on the LED.
A sketch needs to be configured a bit. Enter the data of your Wi-Fi network:
char ssid[] = "xxx"; // SSID имя вашей Wi-Fi точки доступа
char pass[] = "xxxxxxxx"; // пароль вашей сети
And the data of your Azure IoT hub:
char hostname[] = "xxxxxx.azure-devices.net"; // host name address for your Azure IoT Hub
char feeduri[] = "/devices/xxxxxxx/messages/devicebound?api-version=2016-02-03"; // здесь нужно вместо xxxxxxx ввести id устройства
char authSAS[] = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx";
How to get the authSAS (SAS Token) string using Device Explorer I described in a previous article . This is the line that begins with “SharedAccessSignature sr =”.