Simple Yii2 mail sending application
After seeing how cleverly the printer in the office sends letters from anyone anywhere, I decided to implement a simple sender of letters. Among the available tools were Yii 2 framework with the swiftmailer module built into it, virtual Ubuntu on VirtualBox (you can also without it if you install php and a web server locally).
So, let's begin.
Install the basic Yii 2 application in the server folder. After receiving the following image, you can move on:

Next, you need to change the application configuration file /config/web.php . The parameter 'useFileTransport' is initially set to true , for the purpose of catching errors. With this value, letters in a file format get to the / runtime / mail folder. There you can check the main headers of the letter and make sure the settings are correct.
For correct sending, you must use the existing mail service as a transport. Here is a sample configuration for gmail.com mail:
For sending within the corporate environment, you can use both the server name and its IP address. In my case, there is a corporate environment built on the basis of Microsoft products. I consider it as an example. If the Exchange server is not configured for mandatory user verification, then you can specify absolutely nothing in the 'username' and 'password' fields . However, there is no guarantee that the sent letter will not get into the Spam folder of the recipient.
To prevent the message from being filtered by anti-spam, you need to enter the domain username and password for authorization on the Exchange server.
Next, create the MailerForm.php model in the / models folder :
Let's make the mailer.php view for the form in the / views / site / folder :
Next, you need to add an action in the controller /controllers/SiteController.php . At the very beginning of the site you must remember to add the use app \ models \ MailerForm to connect the MailerForm model namespace. And in the class itself add the actionMailer () method .
After these manipulations, following the link index.php? R = site / mailer, you can get to the mail sending form.
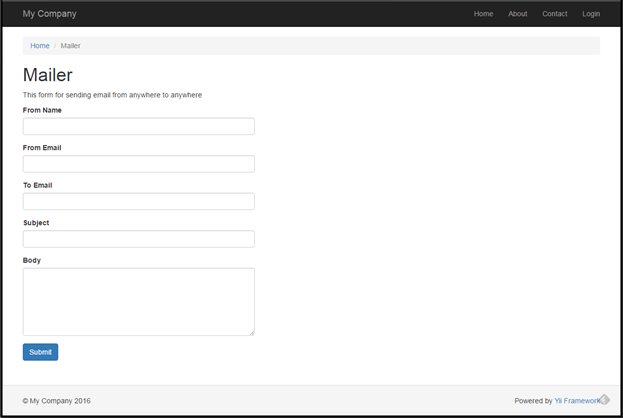
The final touch will add a menu item for ease of use. In the file /views/layouts/main.php in we find the following block:
And add the line:
All is ready!
PS: In no case do not abuse fake emails with fake senders. And do not send spam.
If you are too lazy to do everything with your hands, you can simply clone the project from https://github.com/danvop/mailer . There is also a deployment instruction.
So, let's begin.
Install the basic Yii 2 application in the server folder. After receiving the following image, you can move on:

Next, you need to change the application configuration file /config/web.php . The parameter 'useFileTransport' is initially set to true , for the purpose of catching errors. With this value, letters in a file format get to the / runtime / mail folder. There you can check the main headers of the letter and make sure the settings are correct.
For correct sending, you must use the existing mail service as a transport. Here is a sample configuration for gmail.com mail:
//config/web.php
'mail' => [
'class' => 'yii\swiftmailer\Mailer',
'useFileTransport' => false,
'transport' => [
'class' => 'Swift_SmtpTransport',
'host' => 'smtp.gmail.com',
'username' => 'username@gmail.com',
'password' => 'password',
'port' => '587',
'encryption' => 'tls',
],
],
For sending within the corporate environment, you can use both the server name and its IP address. In my case, there is a corporate environment built on the basis of Microsoft products. I consider it as an example. If the Exchange server is not configured for mandatory user verification, then you can specify absolutely nothing in the 'username' and 'password' fields . However, there is no guarantee that the sent letter will not get into the Spam folder of the recipient.
'mailer' => [
'class' => 'yii\swiftmailer\Mailer',
'useFileTransport' => false,
'transport' => [
'class' => 'Swift_SmtpTransport',
'host' => 'exchange.example.com', //вставляем имя или адрес почтового сервера
'username' => '',
'password' => '',
'port' => '25',
'encryption' => '',
],
],
To prevent the message from being filtered by anti-spam, you need to enter the domain username and password for authorization on the Exchange server.
Next, create the MailerForm.php model in the / models folder :
MailerForm.php
//models/MailerForm.php
validate()) {
Yii::$app->mailer->compose()
->setTo($this->toEmail)
->setFrom([$this->fromEmail => $this->fromName])
->setSubject($this->subject)
->setTextBody($this->body)
->send();
return true;
}
return false;
}
}
Let's make the mailer.php view for the form in the / views / site / folder :
mailer.php
//views/site/mailer.php
title = 'Mailer';
$this->params['breadcrumbs'][] = $this->title;
?>
title) ?>
session->hasFlash('mailerFormSubmitted')) : ?>
Your email has been sent
This form for sending email from anywhere to anywhere
'mailer-form']); ?>
field($model, 'fromName') ?>
field($model, 'fromEmail') ?>
field($model, 'toEmail') ?>
field($model, 'subject') ?>
field($model, 'body')->textArea(['rows' => 6]) ?>
'btn btn-primary', 'name' => 'contact-button']) ?>
Next, you need to add an action in the controller /controllers/SiteController.php . At the very beginning of the site you must remember to add the use app \ models \ MailerForm to connect the MailerForm model namespace. And in the class itself add the actionMailer () method .
load(Yii::$app->request->post()) && $model->sendEmail()) {
Yii::$app->session->setFlash('mailerFormSubmitted');
return $this->refresh();
}
return $this->render('mailer', [
'model' => $model,
]);
}
//…существующий код…
}
After these manipulations, following the link index.php? R = site / mailer, you can get to the mail sending form.
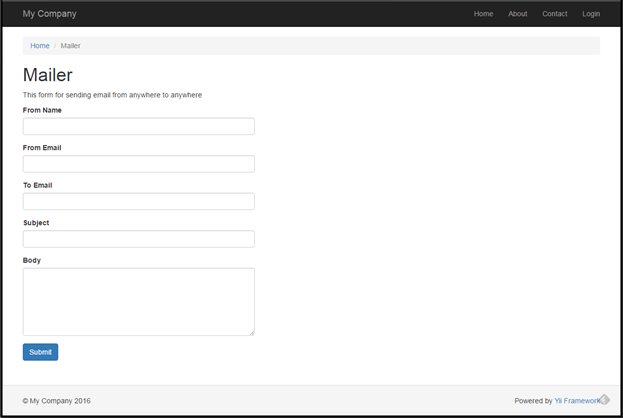
The final touch will add a menu item for ease of use. In the file /views/layouts/main.php in we find the following block:
echo Nav::widget([
'options' => ['class' => 'navbar-nav navbar-right'],
'items' => [
['label' => 'Home', 'url' => ['/site/index']],
['label' => 'About', 'url' => ['/site/about']],
['label' => 'Contact', 'url' => ['/site/contact']],
And add the line:
['label' => 'Mailer', 'url' => ['/site/mailer']],
All is ready!
PS: In no case do not abuse fake emails with fake senders. And do not send spam.
If you are too lazy to do everything with your hands, you can simply clone the project from https://github.com/danvop/mailer . There is also a deployment instruction.