1.3 SFML and Linux
- Transfer
- Tutorial
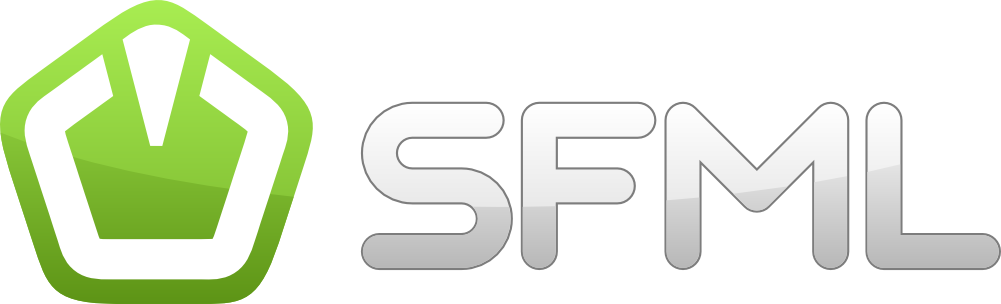
From the translator: this article is the third in the translation cycle of the official SFML library guide. A past article can be found here. This series of articles aims to provide people who do not know the original language with the opportunity to get acquainted with this library. SFML is a simple and cross-platform multimedia library. SFML provides a simple interface for developing games and other multimedia applications. The original article can be found here . Let's get started.
Table of contents:
0.1 Introduction
1. Getting Started
2. System module
3. Window module
4. Graphics module
5. Audio module
6. Network module
1. Getting Started
- SFML and Visual Studio
- SFML and Code :: Blocks (MinGW)
- SFML and Linux
- SFML and Xcode (Mac OS X)
- Compiling SFML with CMake
2. System module
3. Window module
- Opening and managing windows
- Event handling
- Work with keyboard, mouse and joysticks
- Using OpenGL
4. Graphics module
- 2D drawing objects
- Sprites and textures
- Text and Fonts
- Forms
- Designing your own objects with vertex arrays
- Position, rotation, scale: transforming objects
- Adding special effects with shaders
- 2D camera and view control
5. Audio module
- Playing sounds and music
- Audio recording
- Custom audio streams
- Spatialization: 3D sounds
6. Network module
- Socket Communication
- Use and expansion of packages
- Web Requests Using HTTP
- FTP File Transfer
Introduction
This article is the first one you should read if you are using SFML on Linux. It will tell you how to set up your project.
Install SFML
There are different approaches to installing SFML on Linux:
- Installing from your repository repository
- Downloading the precompiled SDK and copying files manually
- Getting the source code, assembly and installation
The first option is preferable. If the version of SFML that you want to install is available in the official repository, then install it using the package manager of your operating system. For example, in Debian you should do the following:
sudo apt-get install libsfml-dev
The third option requires more work: you need to satisfy all the dependencies of SFML. Make sure CMake is installed and run a few commands. This will result in a package tailored for your system. If you want to go this route, read the article on self-assembly SFML .
Finally, the second option is a great choice for quick installation if SFML is not available as an official package. You can download the SDK from the download page . Unzip it and copy the files to the right place: either in a separate path in your personal directory (for example, / home / me / sfml ), or in a standard directory (for example / usr / local ).
Compiling a program using SFML
In this article, we will not talk about integrated development environments such as Code :: Blocks or Eclipse. We will look at the commands needed to compile and link into an executable file using SFML. Writing a Makefile or setting up a project in the IDE is beyond the scope of this article. There is enough material on the Internet for these topics.
If you use Code :: Blocks, you can use the article on setting up Code :: Blocks for Windows ; most of the guidelines should work for Linux as well. You can not tell the compiler and linker where to look for header files and libraries if you installed SFML in the standard way.
Create a source file. In this article, we will call it “main.cpp”. Put the following code in the main.cpp file:
#include
int main()
{
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML works!");
sf::CircleShape shape(100.f);
shape.setFillColor(sf::Color::Green);
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
Now let's compile it:
g++ -c main.cpp
If SFML is installed in a non-standard way, you need to tell the compiler where to find the SFML header files (files with the .hpp extension):
g++ -c main.cpp -I<путь-к-установке-SFML>/include
Here <path-to-install-SFML> is the directory into which you copied SFML. For example / home / me / sfml .
Then you need to link the compiled file to the SFML library in order to get the final executable file. SFML consists of five modules: system, window, graphics, audio and network, and libraries for each of them. To build your application with the SFML library, you must add the option "-lsfml-xxx" to the build command. For example, "-lsfml-graphics" for the graphics module (the prefix "lib" and the extension ".so" libraries should be omitted).
g++ main.o -o sfml-app -lsfml-graphics -lsfml-window -lsfml-system
If you installed SFML in a non-standard way, you need to tell the linker where to find the SFML libraries (files with the .so extension).
g++ main.o -o sfml-app -L<путь-к-установке-SFML>/lib -lsfml-graphics -lsfml-window -lsfml-system
Now we can run the assembled program:
./sfml-app
If SFML is installed in a non-standard way, you need to tell the dynamic linker where to find the SFML libraries by defining the environment variable LD_LIBRARY_PATH:
export LD_LIBRARY_PATH=/lib && ./sfml-app
Run the program, and if everything was done correctly, you should see this:
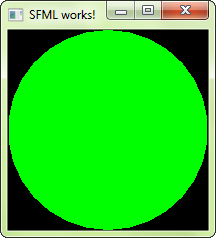
Next article: SFML and Xcode (Mac OS X)