
JavaScript developer job interview
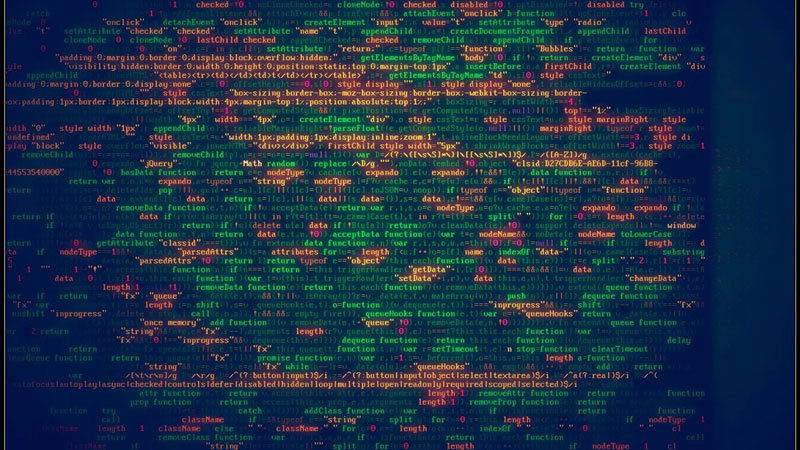
I recently read a good QA job post and thought a similar post would be useful for JavaScript developers. Ultimately, the web is moving forward by leaps and bounds, and there are more than enough candidates for the position of JavaScript programmer (of course, there are always fewer good ones).
I really want to get away from the pointless demagogy on the topic “it is imperative to know, but it doesn’t”, so I’ll build my article around the most popular questions and answers (from the experience of my own interviews, and interviews in which I spoke as the interviewing party), which happened for time of my professional practice.
General JavaScript Matters
OOP in JavaScript . One of the most popular questions in my opinion. From time to time you can hear variations a la “What is prototype inheritance”, “Encapsulation in JavaScript”. Information can be found here or here .
Memory leaks in JS . A huge topic, you can talk about 10 minutes, it seems to me. I consider the most laconic example
var a = {}; a.a = a;
. Such code will create a leak in IE <= 7 version, because donkey up to version 8 cannot clean cyclical dependencies. It should be enough for a simple demonstration of understanding the mechanism of work with leaks. Next, there will be questions a la “How to create a leak using the N framework”. You can read the details here . Closures in JS . No, well, really, what an interview without a question aboutfaults ? The question is partly related to the previous one (“Encapsulation in JavaScript”), but most often it is considered separately.
Self-invoking functions . Also a very common question at interviews. Still sometimes jQuery approaches this: “Why are all the plugins isolated in the design
(function() {})();
?”. The scope of variables. The keyword is this . And, frankly, everything related to the bubbling of variables and functions . Like, how is the record different
var a = function() {};
from the record function a() {};
. Talk about this . How to change the context of a function. Partial application of the function . Well, here you can leave without comment, everything rests on knowing the difference between
.call
, .apply
and.bind
and some standard language-level solutions. You can read about all this in one wonderful article . The difference between the == and === operators . For the answer here
Write a regular expression to check for strings matching the dd.mm.yyyy date format . Well, everything is clear without comment.
What will be the result of executing the following code:
for (var i = 0; i < 10; i++) {
setTimeout(function () {
console.log(i);
}, 0);
}
In addition to the question, I want to say that usually the second question is “How to make the numbers output in order?”
What will the expression + new Date () return? What is different from Date.now () . Great question. The answer is that + new Date (); creates an instance of the Date object and thanks to + translates it into a number format. In the second case, the static constructor method is called, which is more priority, because firstly, it does not require the creation of an instance, and secondly, it is more understandable.
Write a function that takes a string with the file name and returns the extension (the fragment after the last dot) . I will just leave a link to the answer .
Cycle performance. Sometimes (but not always) such a question takes place. Personally, I like him. At the very least, a person should know why
for
faster than Array.prototype.forEach
. But it is advisable to familiarize yourself with the information in this article . On this, as a rule, standard questions end. Next come the questions regarding the specifics of the vacancy. I mean, more "profile" for the company. For example, if you need a JS developer with knowledge of Backbone / Marionette (Chaplin), then the next block of questions will be about jQuery, underscore / lodash and other related technologies, no matter how obvious it sounds. There are a lot of questions on these topics, but I will give a couple of my favorites:
JQuery Related Questions
Why is there an exclamation mark in front of jQuery source code?
Tell us about $ .Deferred . I can advise one thing: besides the knowledge of $ .Deferred , it would be nice to mention the native Promise'ah
How to create a memory leak using jQuery .
I love simple examples, so I usually give the following:
var jqSelector = $("#selector"),
nativeSelector = document.getElementById("selector");
// Удаляем элемент "нативным" способом
nativeSelector.parentNode.removeChild(nativeSelector);
// Выводим закешированное значение селектора jQuery
console.log(jqSelector); // Привет, jQuery.cache!
In general, in terms of questions about native JS, it was already similar, and there is also a link to examples using jQuery.
jQuery.extend . To tell everything about the function, you may be asked to write an analogue. By the way, it might be useful to read an article about impurities in JS .
Make an AJAX request to the server using jQuery . Not a very popular question, but if you ask nothing, they will ask something like him. Of course, you must know how to use the functions
$.ajax
, $.get
, $.post
and set the settings $.ajaxSettings
. All this has long been a Habré .The remaining questions are more likely to be "extras", I believe that there is nothing more to ask about jQuery. Of course, there will be questions like “How to add a class to an object using jQuery”, but I think it will not be a surprise and will not cause problems.
Underscore / Lodash Related Questions
These are, frankly, very rare. But still, here is a short list of what I can recall:
How is _.throttle different from _.debounce . Again, the answer is on Habr .
How the _.extend function works . An analogue of the question about jQuery.extend, a link to the dock above.
Tell us about the Underscore template engine . By the way, it is possible that this is only the beginning, and you will be asked about the jQuery template engine and all the rest. I would advise reading this article with just one eye .
Lodash vs Underscore . Question for breeding holivar at interviews
What's next?
And then there are special questions regarding the frameworks and libraries that the company uses in its projects. Usually, the answers to questions from the “native” JS category are most valued, but it’s not surprising: technologies appear and disappear, and it’s not at all surprising if the programmer hasn’t heard something, but the answers to this category of questions show how quickly a person can learn and understand how a particular library / framework works.
Of course, if you have something to add to this article - write in PM, I will definitely supplement it with your information.
Thanks f0rk for interesting questions.