
Spring MVC - JavaConfig or project configuration without XML files
Good day dear Khabravchians. Somehow I had to write a small project, since I had the opportunity to freely choose technologies, I decided to use the Spring Framework . I want to say right away that I am not a guru in this framework, and I will be grateful to the edits.
Having searched a little on the hub, I could not find exactly what I want to tell and demonstrate to you now. Therefore, I decided to write a post on this topic. We will talk about JavaConfig , namely how to configure a Spring MVC project without using * .xml configurations, for example: (mvc-dispatcher-servlet.xml).
On the subject of Spring MVC there is good material here and here, but these examples use xml configurations. I want to show how to create the Spring MVC project structure using JavaConfig .
JavaConfig is an alternative to the usual XML configuration, but using not xml markup, but Java syntax.
I like to use JavaConfig, primarily because it is Java, we see java code, which is convenient, and secondly, most of the configuration errors can be caught at the time of writing the configuration, your favorite IDE will help you in my case, this is Intellij IDEA, but even if the IDE says that everything is cool, then you have a chance to catch a configuration error at the time of compilation.
1. The main advantage, in my opinion, is the Java syntax;
2. You get all the features of the IDE with respect to working with code, automatic code generation, auto-substitution of values, tooltips, etc .;
3. More flexibility through the use of objects and their methods;
4. There is no need for a complete assembly and deployment of the project in order to catch a fault in the xml configuration. (but there are exceptions, for example: NullPointerException ).
JavaConfig existed earlier as a separate project, but since Spring Core 3.0 this project has become part of the core project. Now in order to be able to write configuration using JavaConfig, it is enough to use Spring Core 3+
First, let's look at the structure and configuration of the Spring MVC project using XML configurations, and after that we will translate it into JavaConfig.
This is what the project structure looks like:
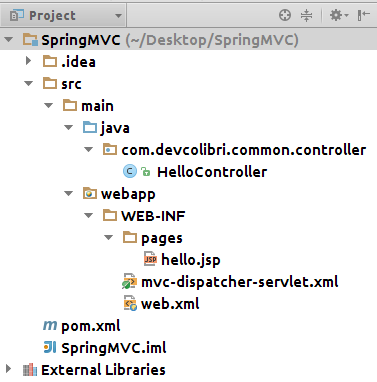
Let's start by adding dependencies in pom.xml :
Now create a simple jsp index.jsp page :
And the last thing that doesn't concern the configuration is the HelloController.java controller :
Now we have everything we need to get a working Spring MVC project, in addition to configuration. Further we will consider it.
Please note that I do not explain all the details of the Spring MVC project, since there were already posts on this subject on the hub
Now we’ll look at how to configure a project using an XML file. Let's start with mvc-dispatcher-servlet.xml it is this file that will configure our project, basically here we describe all our beans .
The contents of the mvc-dispatcher-servlet.xml file:
In this configuration, we indicate where to look for all our controllers, services and other components using the tag: context: component-scan , and also initialize the InternalResourceViewResolver, which is responsible for displaying the View in our case, this is jsp page.
Since JavaConfig are simple java classes, we will create a config package for them as shown in Fig. below, and also here is our new project structure already without web.xml and mvc-dispatcher-servlet.xml .
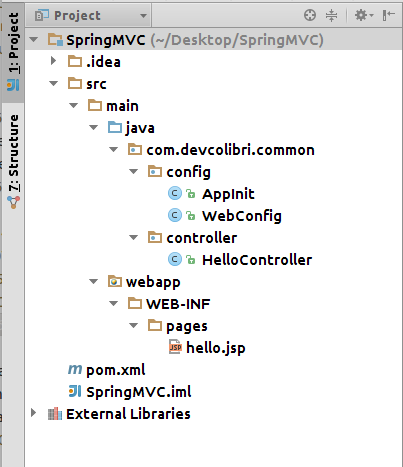
As you can see from the structure of the project, we have two classes in the config package , they are the replacement of the xml configuration.
Let's start with WebConfig :
@Configuration - this annotation actually says that this class is Java Configuration ;
@EnableWebMvc - this annotation allows our project to use MVC;
@ComponentScan ("com.devcolibri.common") - similar to the component-scan that was in mvc-dispatcher-servlet.xml , it tells where to look for project components.
Bean - indicates that this is a bean initialization, and it will be created using DI.
WebMvcConfigurerAdapter - inheriting from this class, we get the opportunity to configure ResourceLocations .
addResourceHandlers (ResourceHandlerRegistry registry) - overriding this method, we can specify where our project resources, such as css, image, js and others, will lie.
InternalResourceViewResolver - a similar configuration with mvc-dispatcher-servlet.xml.
Now we need to register the configuration in Spring Context, this will allow us to make our AppInit class :
Two more dependencies must be added to pom.xml:
And now we need to add the maven-war-plugin with the help of which we can disable the need for the web.xml file.
That's all. I hope this material was useful.
ps I'm not a guru, and I will be happy to edit the code.
Having searched a little on the hub, I could not find exactly what I want to tell and demonstrate to you now. Therefore, I decided to write a post on this topic. We will talk about JavaConfig , namely how to configure a Spring MVC project without using * .xml configurations, for example: (mvc-dispatcher-servlet.xml).
On the subject of Spring MVC there is good material here and here, but these examples use xml configurations. I want to show how to create the Spring MVC project structure using JavaConfig .
What is JavaConfig?
JavaConfig is an alternative to the usual XML configuration, but using not xml markup, but Java syntax.
I like to use JavaConfig, primarily because it is Java, we see java code, which is convenient, and secondly, most of the configuration errors can be caught at the time of writing the configuration, your favorite IDE will help you in my case, this is Intellij IDEA, but even if the IDE says that everything is cool, then you have a chance to catch a configuration error at the time of compilation.
Benefits:
1. The main advantage, in my opinion, is the Java syntax;
2. You get all the features of the IDE with respect to working with code, automatic code generation, auto-substitution of values, tooltips, etc .;
3. More flexibility through the use of objects and their methods;
4. There is no need for a complete assembly and deployment of the project in order to catch a fault in the xml configuration. (but there are exceptions, for example: NullPointerException ).
JavaConfig existed earlier as a separate project, but since Spring Core 3.0 this project has become part of the core project. Now in order to be able to write configuration using JavaConfig, it is enough to use Spring Core 3+
Standard configuration using XML
First, let's look at the structure and configuration of the Spring MVC project using XML configurations, and after that we will translate it into JavaConfig.
This is what the project structure looks like:
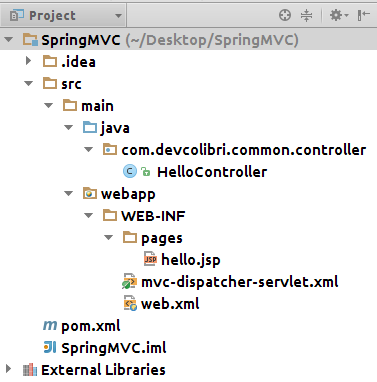
Let's start by adding dependencies in pom.xml :
3.2.9.RELEASE org.springframework spring-core ${spring.version} org.springframework spring-web ${spring.version} org.springframework spring-webmvc ${spring.version}
Now create a simple jsp index.jsp page :
Message : ${message}
And the last thing that doesn't concern the configuration is the HelloController.java controller :
package com.devcolibri.common.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
@RequestMapping("/welcome")
public class HelloController {
@RequestMapping(method = RequestMethod.GET)
public String printWelcome(ModelMap model) {
model.addAttribute("message", "Spring 3 MVC - Hello World");
return "hello";
}
}
Now we have everything we need to get a working Spring MVC project, in addition to configuration. Further we will consider it.
Please note that I do not explain all the details of the Spring MVC project, since there were already posts on this subject on the hub
Now we’ll look at how to configure a project using an XML file. Let's start with mvc-dispatcher-servlet.xml it is this file that will configure our project, basically here we describe all our beans .
The contents of the mvc-dispatcher-servlet.xml file:
/WEB-INF/pages/ .jsp
In this configuration, we indicate where to look for all our controllers, services and other components using the tag: context: component-scan , and also initialize the InternalResourceViewResolver, which is responsible for displaying the View in our case, this is jsp page.
JavaConfig Based Configuration
Since JavaConfig are simple java classes, we will create a config package for them as shown in Fig. below, and also here is our new project structure already without web.xml and mvc-dispatcher-servlet.xml .
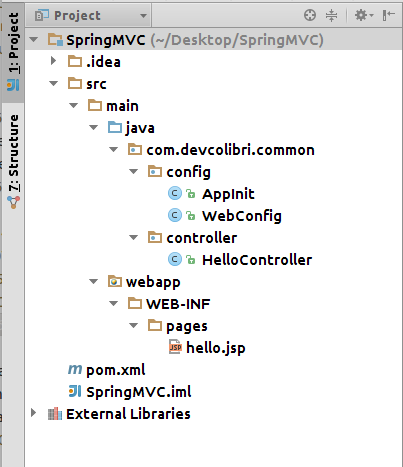
As you can see from the structure of the project, we have two classes in the config package , they are the replacement of the xml configuration.
Let's start with WebConfig :
package com.devcolibri.common.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
import org.springframework.web.servlet.view.JstlView;
@Configuration
@EnableWebMvc
@ComponentScan("com.devcolibri.common")
public class WebConfig extends WebMvcConfigurerAdapter {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/WEB-INF/pages/**").addResourceLocations("/pages/");
}
@Bean
public InternalResourceViewResolver setupViewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/pages/");
resolver.setSuffix(".jsp");
resolver.setViewClass(JstlView.class);
return resolver;
}
}
Annotations:
@Configuration - this annotation actually says that this class is Java Configuration ;
@EnableWebMvc - this annotation allows our project to use MVC;
@ComponentScan ("com.devcolibri.common") - similar to the component-scan that was in mvc-dispatcher-servlet.xml , it tells where to look for project components.
Bean - indicates that this is a bean initialization, and it will be created using DI.
Configuration
WebMvcConfigurerAdapter - inheriting from this class, we get the opportunity to configure ResourceLocations .
addResourceHandlers (ResourceHandlerRegistry registry) - overriding this method, we can specify where our project resources, such as css, image, js and others, will lie.
InternalResourceViewResolver - a similar configuration with mvc-dispatcher-servlet.xml.
/WEB-INF/pages/ .jsp
Now we need to register the configuration in Spring Context, this will allow us to make our AppInit class :
package com.devcolibri.common.config;
import org.springframework.web.servlet.support.AbstractAnnotationConfigDispatcherServletInitializer;
public class AppInit extends AbstractAnnotationConfigDispatcherServletInitializer {
// Этот метод должен содержать конфигурации которые инициализируют Beans
// для инициализации бинов у нас использовалась аннотация @Bean
@Override
protected Class[] getRootConfigClasses() {
return new Class[]{
WebConfig.class
};
}
// Тут добавляем конфигурацию, в которой инициализируем ViewResolver
@Override
protected Class[] getServletConfigClasses() {
return new Class[]{
WebConfig.class
};
}
@Override
protected String[] getServletMappings() {
return new String[]{"/"};
}
}
Add the necessary dependencies and plugins
Two more dependencies must be added to pom.xml:
javax.servlet javax.servlet-api 3.0.1 jstl jstl 1.2
And now we need to add the maven-war-plugin with the help of which we can disable the need for the web.xml file.
org.apache.maven.plugins maven-war-plugin false
That's all. I hope this material was useful.
ps I'm not a guru, and I will be happy to edit the code.