
SimpleMembership Guide in ASP.NET MVC 4
- Tutorial
With the advent of ASP.NET MVC 4 and WebMatrix, the mvc team strives to make things easier for the developer. Based on reviews, one of the areas for improvement was asp.net security.
The ASP.NET MVC 4 Internet project template has added some very useful new features that are built using SimpleMembership. SimpleMembership brought easy customization of roles and users, and added support for OAuth. However, the new provider is not compatible with the existing ASP.NET Membership Provider.
In this post I will talk about what SimpleMembership is and how to use it in an ASP.NET MVC 4 project.
The ASP.NET Membership System was introduced in 2005. It was developed to solve common problems, such as registering on a site using a bunch of login and password, storing a profile in a SQL Server database. Expansion mechanisms have also been added to override the standard logic of MembershipProvider and RoleProvider. 8 years ago, this mechanism coped with its task, but today it is an inconvenient tool. If the user profile requires additional fields, then all of them are stored in one column and require access to the API calls of the appropriate provider for access.
ASP.NET WebPages and WebMatrix redefine many things, such as the Razor view engine and SimpleMembership.
The standard provider works fine if the following conditions are met: all information will be stored in the full version of the SQL Server database and that all the necessary data is presented as a set of attributes (UserName, Password, IsApproved, CreationDate ...) and other information will be provided using Profile Provider.
Most full-featured ASP.NET providers require the full version of SQL Server (because they rely on the work of stored procedures, on SQL Server cache and other server features). In addition, the default providers will not work on SQL Azure.
To work with a database other than SQL Server, you need to redefine the set of provider methods that are highly oriented towards storing data in a relational database. Firstly, this is a large amount of work on redefining these methods, and secondly, most likely there will be a lot of undefined methods containing
Existing data providers are strictly focused on this model, in which the user has a username and password. Of course, additional information can be added via the API, but such a model is not suitable for OAuth (the user does not have a password there).
A role-oriented system will not always be appropriate, it may be more convenient to use a model of access rights to individual objects or actions (Claims).
Also, a rigid database scheme with a large number of blob columns is required.
SimpleMembership was developed just to solve the problems voiced above.
Matthew Osborn, in his post Using SimpleMembership With ASP.NET WebPages, explains that SimpleMembership is designed to integrate seamlessly with your database.

Now you need to talk about this SimpleMembership: add a connection string to the database.
And determine the initialization:
After starting the site and processing the initialization attribute, SimpleMembership will create the necessary tables for it to work. Our users table will be used as a user table.

SimpleMembership works with the entire line of SQL Server (SQL Azure, SQL Server CE, SQL Server Express, and LocalDB). Everything is implemented as SQL calls, which is much better than using stored procedures.
The problem with ASP.NET Membership is that it stores additional information about the account itself. This means that you cannot directly access profile data. While SimpleMembership doesn’t care what table and how user data is stored. You can easily change the table with users, for example, add an address.
Now you can easily access this field not using the provider itself, but directly from the database. This allows you to accept SimpleMembership as a layer between the database and the ASP.NET Membership system.
How it is implemented, I will omit, you can see in the original entry SimpleMembership . It is important to know that SimpleMembership is inherited from ExtendedMembershipProvider.
In the default template, the following mechanism for working with SimpleMembership is implemented:

WebSecurity works with any ExtendedMembershipProvider. By default, SimpleMembershipProvider is used, but you can implement your own.
Although this provider works by default in a project with an ASP.NET MVC 4 Internet template, some (including myself) create a project from an empty template. You need to add 2 links: WebMatrix.Data and WebMatrix.WebData. Or install these libraries through NuGet with identifiers of the same name).
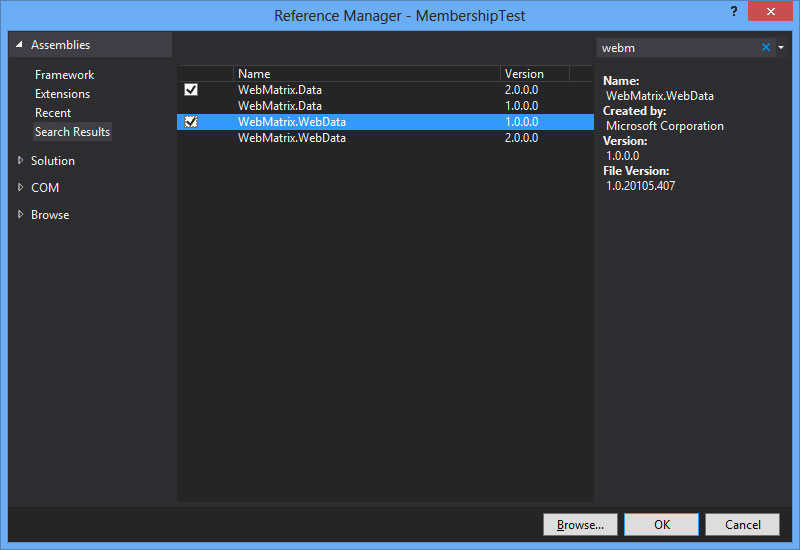
Now you need to add the provider and indicate the use of WebMatrix
Since WSAT cannot be used, there are 2 ways to create users and roles. If the EntityFramework Code First model is used, it will be convenient to add Micragion with the creation of default users:
Or, you can create a user, a role directly in the database editor, and assign a role to the user.
The ASP.NET MVC 4 Internet project template has added some very useful new features that are built using SimpleMembership. SimpleMembership brought easy customization of roles and users, and added support for OAuth. However, the new provider is not compatible with the existing ASP.NET Membership Provider.
In this post I will talk about what SimpleMembership is and how to use it in an ASP.NET MVC 4 project.
What is SimpleMembership
- SimpleMembership is designed to replace the previous version of ASP.NET Membership Provider (ASP.NET Role)
- SimpleMembership solves the same tasks, but makes them easier for the developer and adapts to modern security requirements.
- The controller
AccountController
in the Interner ASP.NET MVC 4 project template requires SimpleMembership and is not compatible with older versions - The annoying news is that the Web Site Administration Tool (WSAT) is not compatible with SimpleMembership.
The ASP.NET Membership System was introduced in 2005. It was developed to solve common problems, such as registering on a site using a bunch of login and password, storing a profile in a SQL Server database. Expansion mechanisms have also been added to override the standard logic of MembershipProvider and RoleProvider. 8 years ago, this mechanism coped with its task, but today it is an inconvenient tool. If the user profile requires additional fields, then all of them are stored in one column and require access to the API calls of the appropriate provider for access.
ASP.NET WebPages and WebMatrix redefine many things, such as the Razor view engine and SimpleMembership.
Why using ASP.NET Membership is not recommended
The standard provider works fine if the following conditions are met: all information will be stored in the full version of the SQL Server database and that all the necessary data is presented as a set of attributes (UserName, Password, IsApproved, CreationDate ...) and other information will be provided using Profile Provider.
Requires full default SQL Server
Most full-featured ASP.NET providers require the full version of SQL Server (because they rely on the work of stored procedures, on SQL Server cache and other server features). In addition, the default providers will not work on SQL Azure.
Difficulties working with another database
To work with a database other than SQL Server, you need to redefine the set of provider methods that are highly oriented towards storing data in a relational database. Firstly, this is a large amount of work on redefining these methods, and secondly, most likely there will be a lot of undefined methods containing
System.NotImplementedException
that does not paint the code.Model Orientation User> Role
Existing data providers are strictly focused on this model, in which the user has a username and password. Of course, additional information can be added via the API, but such a model is not suitable for OAuth (the user does not have a password there).
A role-oriented system will not always be appropriate, it may be more convenient to use a model of access rights to individual objects or actions (Claims).
Also, a rigid database scheme with a large number of blob columns is required.
SimpleMembership enhanced user system
SimpleMembership was developed just to solve the problems voiced above.
Matthew Osborn, in his post Using SimpleMembership With ASP.NET WebPages, explains that SimpleMembership is designed to integrate seamlessly with your database.
SimpleMembership requires that there are 2 columns in the user table: “ID” and “UserName”. The important part here is that these columns can have any name.

Now you need to talk about this SimpleMembership: add a connection string to the database.
And determine the initialization:
WebSecurity.InitializeDatabaseConnection("DefaultConnection", "Users", "Id", "Name", autoCreateTables: true);
After starting the site and processing the initialization attribute, SimpleMembership will create the necessary tables for it to work. Our users table will be used as a user table.

SimpleMembership works with the entire line of SQL Server (SQL Azure, SQL Server CE, SQL Server Express, and LocalDB). Everything is implemented as SQL calls, which is much better than using stored procedures.
Using EntityFramework with Code First
The problem with ASP.NET Membership is that it stores additional information about the account itself. This means that you cannot directly access profile data. While SimpleMembership doesn’t care what table and how user data is stored. You can easily change the table with users, for example, add an address.
[Table("Users")]
public class UserProfile
{
[Key]
[DatabaseGeneratedAttribute(DatabaseGeneratedOption.Identity)]
public int Id { get; set; }
public string Name { get; set; }
public string Address { get; set; }
}
Now you can easily access this field not using the provider itself, but directly from the database. This allows you to accept SimpleMembership as a layer between the database and the ASP.NET Membership system.
How it is implemented, I will omit, you can see in the original entry SimpleMembership . It is important to know that SimpleMembership is inherited from ExtendedMembershipProvider.
ASP.NET MVC 4 Internet template
In the default template, the following mechanism for working with SimpleMembership is implemented:

- AccountModel.cs describes the basic user account and includes attributes for the database
- InitializeSimpleMembershipAttribute.cs just contains information for initializing the provider: which database to use, which fields and other settings.
- AccountController.cs contains calls to the WebSecurity class of the WebMatrix library.
WebSecurity works with any ExtendedMembershipProvider. By default, SimpleMembershipProvider is used, but you can implement your own.
Setting up SimpleMembership
Adding SimpleMembership to an existing project
Although this provider works by default in a project with an ASP.NET MVC 4 Internet template, some (including myself) create a project from an empty template. You need to add 2 links: WebMatrix.Data and WebMatrix.WebData. Or install these libraries through NuGet with identifiers of the same name).
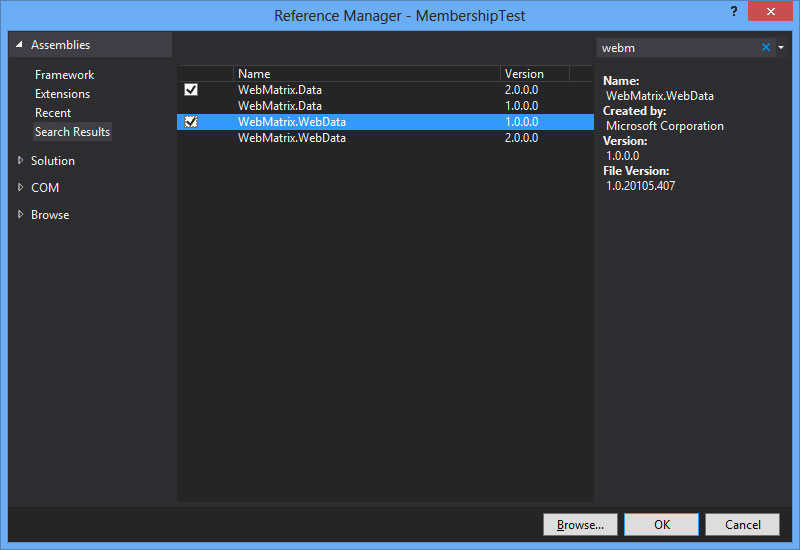
Now you need to add the provider and indicate the use of WebMatrix
Since WSAT cannot be used, there are 2 ways to create users and roles. If the EntityFramework Code First model is used, it will be convenient to add Micragion with the creation of default users:
public partial class AddDefaultUser : DbMigration
{
public override void Up()
{
if (!WebSecurity.Initialized)
{
WebSecurity.InitializeDatabaseConnection("DefaultConnection", "Users", "Id", "UserName",
autoCreateTables:
true);
}
var roles = (SimpleRoleProvider)Roles.Provider;
var membership = (SimpleMembershipProvider)Membership.Provider;
if (!roles.RoleExists("Admin"))
{
roles.CreateRole("Admin");
}
if (membership.GetUser("Admin", false) == null)
{
membership.CreateUserAndAccount("Admin", "SuperAdminPassword");
}
if (!roles.GetRolesForUser("Admin").Contains("Admin"))
{
roles.AddUsersToRoles(new[] { "Admin" }, new[] { "Admin" });
}
}
public override void Down()
{
throw new NotImplementedException();
}
}
Or, you can create a user, a role directly in the database editor, and assign a role to the user.
References
- SimpleMembership, Membership Providers, Universal Providers and the new ASP.NET 4.5 Web Forms and ASP.NET MVC 4 templates
- Adding ASP.NET SimpleMembership to an existing MVC 4 application
- Using SimpleMembership With ASP.NET WebPages Matthew M. Osborn
- Seeding & Customizing ASP.NET MVC SimpleMembership
- ASP.NET MVC Authorization (Why You Shouldn't Use MembershipProvider)