Game design from scratch: how to start making games without experience
Nikita Golubev, commercial author and translator, specifically for the Netology blog, translated the article of the game developer Angela He on how to create your first game without programming skills.
Just 2 years ago, I was a 17-year-old schoolgirl and did not know anything about programming. This did not prevent me from starting to learn and in a few months to release my first game on Steam. Today I have more than 10 games for PC, Internet and mobile devices and over 1.9 million players.
It doesn’t matter that you can do it now - if you wish, you can make games too. Two years ago, this seemed impossible: it was the most difficult thing I did in life, and it was worth it. Now I understand that in game development, as in any other business, you grow only when you try, make mistakes, and improve.
All that I know, I learned myself, and now I will teach you.
In the article for each stage, I will highlight:
- Tips that I learned from my and others' experience.
- Tools that I find most useful.
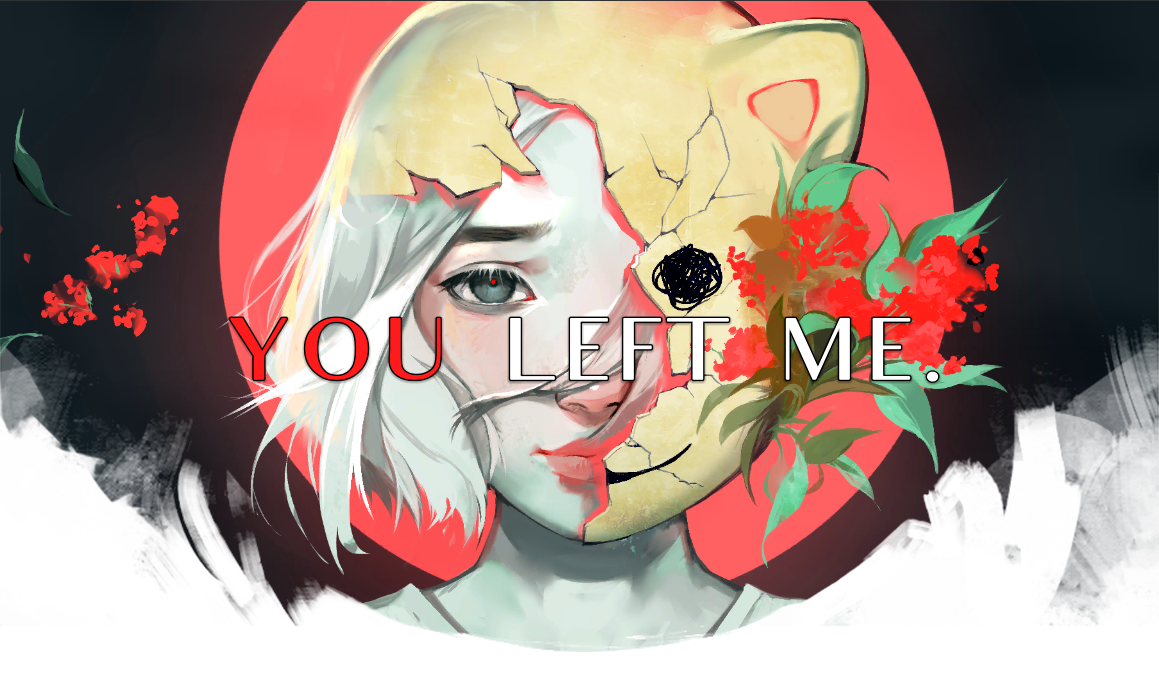
You have a great idea. But how to make it on paper?
Everyone has his own path. Some compose design documents of 60 pages, others will write a list of illegible notes. I do not know how it is more convenient for you, but be sure to note the following:
Keeping notes:
Teamwork:
I make games on unity. Then we will talk about it, but do not be afraid to use another engine.
Inspiration:
If you cannot program, first read the “Programming” section. It is unlikely that you want to spend time on graphics and throw it out because you can’t write code for it.
Even if you cannot draw, the game can be made beautiful using three visual principles: color, shape and volume.
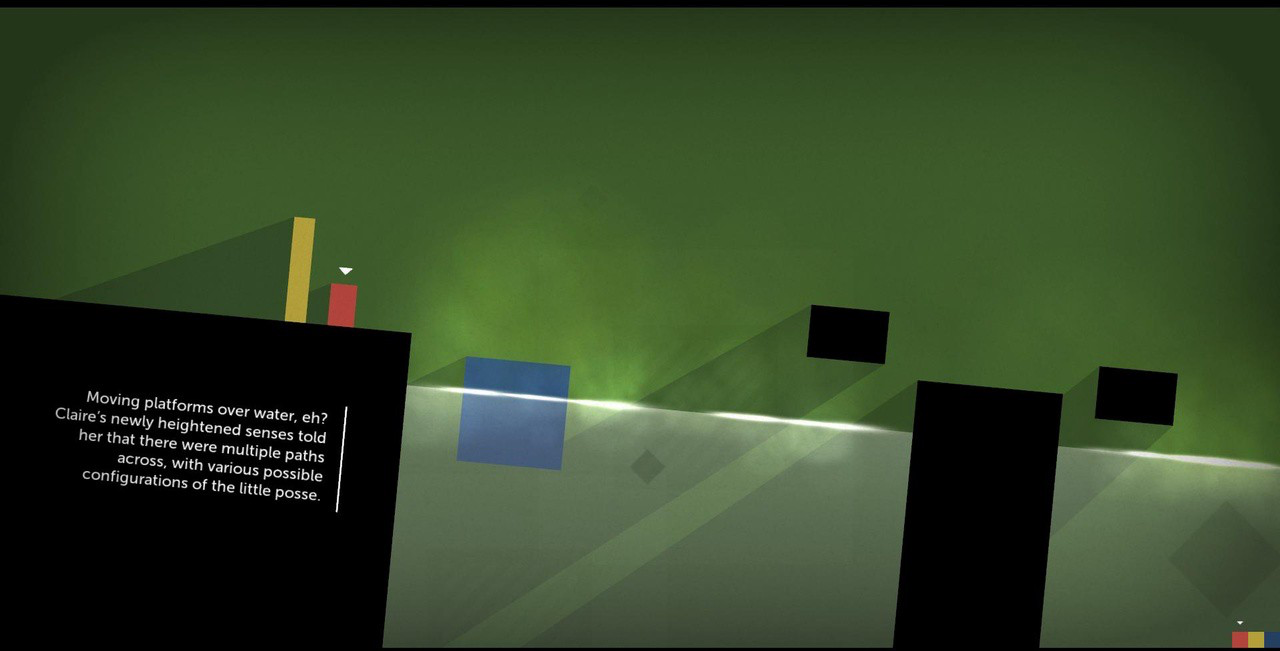
Thomas Was Alone is a simple and beautiful game.
Think about how to make the game unique with the help of color schemes, fonts and icons without losing the convenience for the player. Is important information clear and readable?

Unsuccessful and successful font
Animation can be implemented in two ways:
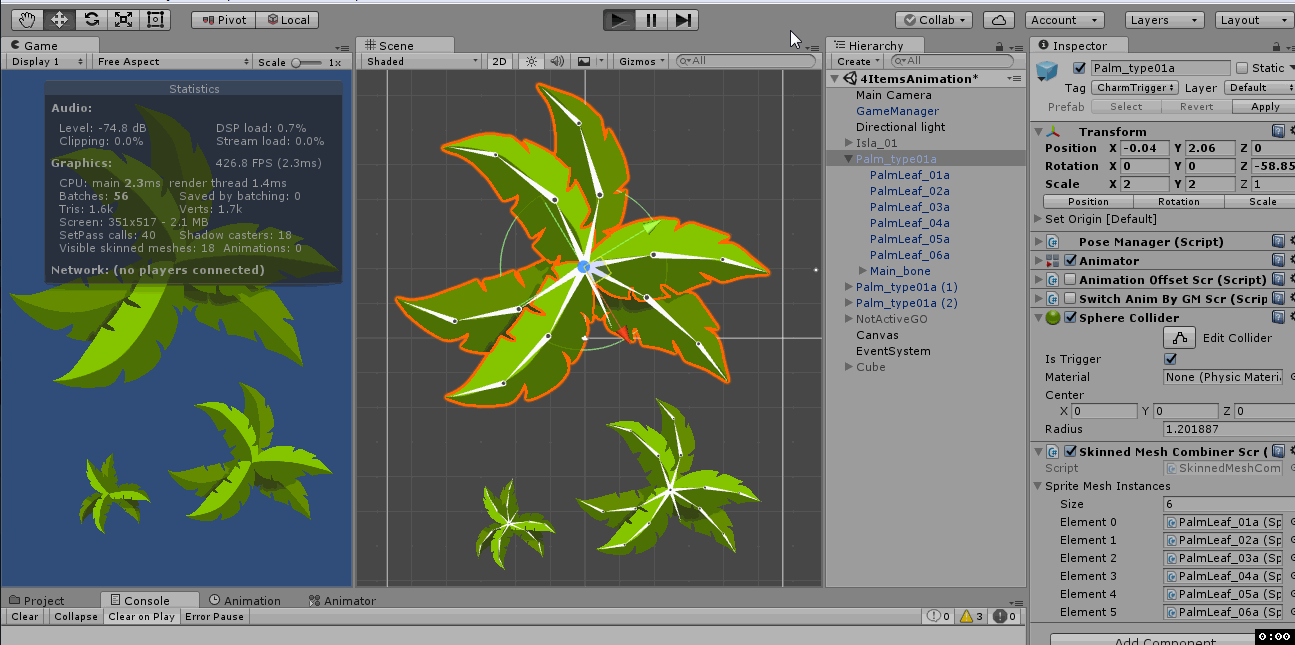
Tips that apply not only to game graphics, but also to other programs:

Without tiles and with tiles

The stain expands, but the angles remain the same.
Interface creation:
Principles of creating an interface:
Creating 2D objects:
Creating 3D objects:
Free game assets:
Inspiration:
Select the game engine, development environment and start to dive into the code.
The knowledge below is enough to get you started. All examples are written in C ++, one of the programming languages in Unity3D. ( Translator’s note: in fact, Unity uses C #, which is similar to C ++ ).
Then, when the function is output, two expressions are executed inside it.
If something goes wrong in the code, exceptions come to the rescue. They kind of say: “So, wait, here you did something illogical. Check back again. ”
What else you need to know:
A little inspiration. I know programming is scary at first. Everything seems meaningless, you constantly stumble and in the face of constant mistakes you want to give up, but this does not mean that programming is not for you.
Like any skill, programming needs to be learned. And maybe it will be quite interesting.
Other important programming fundamentals:
Unity-specific things:
Game engines:
Development environments:
Free Unity
Assets : There are a lot of free assets in the Unity Asset Store, bitbucket and GitHub. In my projects I use at least two. They simplify life, but are far from perfect. Noticed a mistake - correct and tell the developer about it.
Audio is able to create mood and immerse in the game, but it needs memory.
First decide: do you want sound? If so, will there be music, sound effects, voice acting or narration in the game.
In any case, you need to record and mix in such a way that the sound fits the mood of the game. For example, Bastion uses organic and instrumental sounds that fit well into the game world. Crypt of the Necrodancer included a mixture of electronic rhythms and eight-bit rock to convey the tempo and brightness of the game.
Audio applications:
Creating retro effects:
Free sounds:
There is a small chance - at 99.99 percent, that there are mistakes in the game. And that means it's time to do a bug test.
So, the bug is found. Now what?
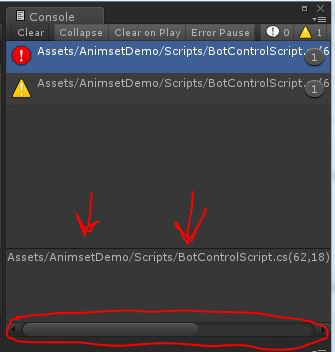
When done with errors, proceed to optimize performance and memory usage. Users will be able to run the game faster and not experience problems with heating devices.
Scripts:
Graphics:
Memory:
Platform Optimization:
It's time to show the world your creation.
Promotion - the most exciting stage. If in doubt, the game design community will help you. Remember that you are not alone, and since you have gone through so much, you need to finish the job to the end.
The presskit () service helps developers make a description of the game for the press.
Publication platforms:
Magazines about games:
Festivals:
Game Conferences:
There is no easy way to create a game. There is only your determination and effort.
You will make mistakes, feel at a dead end and cry. This is normal - it means you are growing above yourself.
Just 2 years ago, I was a 17-year-old schoolgirl and did not know anything about programming. This did not prevent me from starting to learn and in a few months to release my first game on Steam. Today I have more than 10 games for PC, Internet and mobile devices and over 1.9 million players.
It doesn’t matter that you can do it now - if you wish, you can make games too. Two years ago, this seemed impossible: it was the most difficult thing I did in life, and it was worth it. Now I understand that in game development, as in any other business, you grow only when you try, make mistakes, and improve.
All that I know, I learned myself, and now I will teach you.
To make the game, you need to go through 6 stages:
- Concept
- Graphics
- Programming
- Sound
- Preparation for publication
- Publication
In the article for each stage, I will highlight:
- Tips that I learned from my and others' experience.
- Tools that I find most useful.
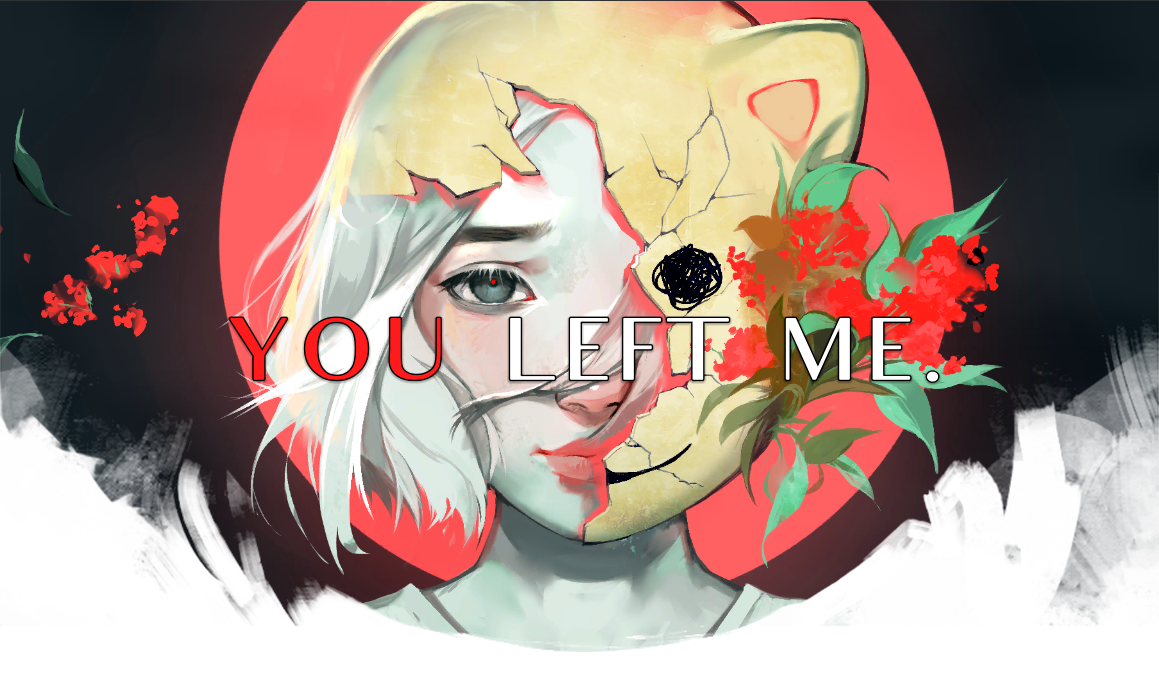
Concept
The board
You have a great idea. But how to make it on paper?
Everyone has his own path. Some compose design documents of 60 pages, others will write a list of illegible notes. I do not know how it is more convenient for you, but be sure to note the following:
- Chips. What makes your idea cool? This is the main question. As soon as you can catch and fix it, the remaining steps will seem easier. Does your game raise sharp topics? Become a new classic? Or will it be different from everything that was before?
- Mechanics. What does the player do and why? This is your gameplay. It can be as simple as pressing Q, W, O, P keys in a QWOP game one after another , or as complex as a combination in Dwarf Fortress.
- Legend. What makes the players remember your game? What emotions will they leave? Every game has a story. If story is not obvious, the player himself will create it. The story is different: the increase in numbers in the game 2048, the rise of empires in Civilization, or silent interaction in the Monument Valley. Think about what kind of legend will be behind your game.
- Mood. What is the impression of the game? What visual effects and music will contribute to this? The first impression that the player will be able to hook on and then make him return to the game is important. You may want a retro effect with pixel graphics and 8-bit music or a modern look with flat geometry - think about it.
- Participate in hackathons. You and the other participants will need to make a game in the allotted time. At least you will be inspired and meet like-minded people. Try Ludum Dare , one of the biggest gamejams.
- Create a list of ideas. Write down every new thought. In moments of stupor, you can always look at the list and find something interesting. This is my personal Google Doc of ideas and notes .
When inspiration comes, drop everything and write. The next time the thought goes, no need to suck the idea out of your finger.
Instruments
Keeping notes:
- Notes on Mac OS.
- Google Docs.
- Trello.
Teamwork:
- Google Drive.
- Github Requires git and unity .gitignore.
- Unity Collab. The easiest tool, but with limitations in the free version.
I make games on unity. Then we will talk about it, but do not be afraid to use another engine.
Inspiration:
- Jesse Shell's book The Art of Game Design .
- Website for game developers Gamasutra .
Graphics
The board
If you cannot program, first read the “Programming” section. It is unlikely that you want to spend time on graphics and throw it out because you can’t write code for it.
Even if you cannot draw, the game can be made beautiful using three visual principles: color, shape and volume.
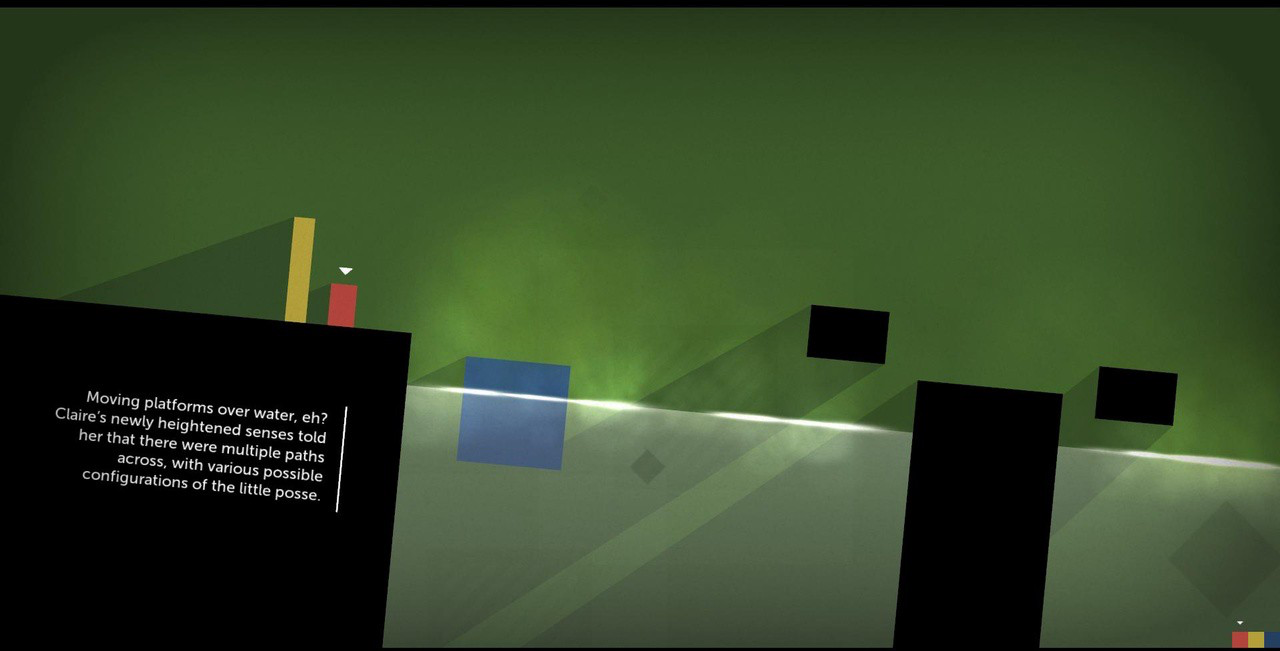
Thomas Was Alone is a simple and beautiful game.
Interface
Think about how to make the game unique with the help of color schemes, fonts and icons without losing the convenience for the player. Is important information clear and readable?

Unsuccessful and successful font
2D animation
Animation can be implemented in two ways:
- Frame by frame animation. When you draw each frame. For this, use the sprite tables and Sprite Packer in Unity.
- Skeletal animation. Each moving limb is drawn, then its position and turns are animated. It is faster, easier and spends less RAM. For 2D animation on Unity, use pivots (anchor points) or Anima 2D plug-in .
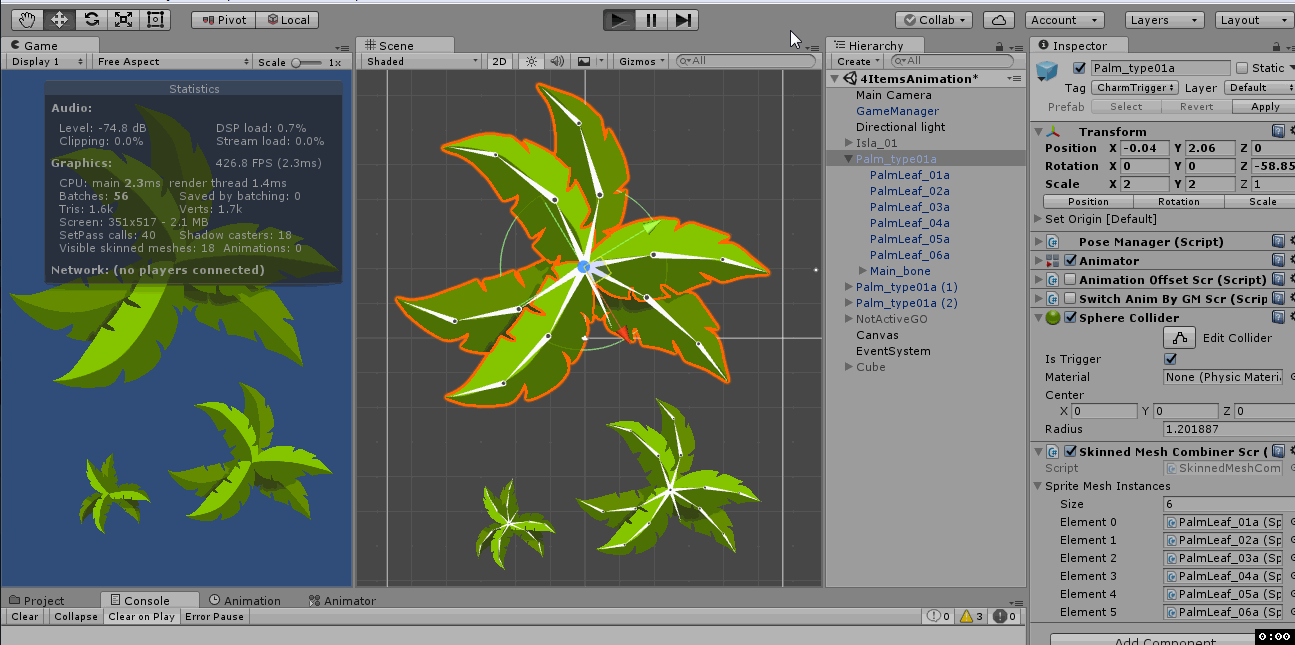
What else might come in handy
Tips that apply not only to game graphics, but also to other programs:
- Tile objects are used to create tiles and require less hard disk space.

Without tiles and with tiles
- 9-slice objects with non-scalable borders and a scalable center allow you to enlarge images without filling the memory.

The stain expands, but the angles remain the same.
- Make the resolution of each object so that it is divisible by 4 or is a power of 2.
- In Photoshop, you can save each layer to a separate file via File> Export> Fast Export to [image format].
Instruments
Interface creation:
- Photoshop.
- Sketch
Principles of creating an interface:
Creating 2D objects:
- Photoshop.
- Gimp.
- Paint Tool SAI - for anime style graphics.
Creating 3D objects:
- Blender is powerful software with complex learning.
- Maya - for animation.
- Max - for drawing.
Free game assets:
- Behance - fonts, icons and more.
- KennyNL - high-quality objects, ready to use in games.
- Open Game Dev Art is a huge graphics library created by other users.
Inspiration:
- Dribbble is a closed design community.
- Behance is a community of designers that anyone can join.
- itch.io is a community of indie game creators.
Programming
The board
Select the game engine, development environment and start to dive into the code.
The knowledge below is enough to get you started. All examples are written in C ++, one of the programming languages in Unity3D. ( Translator’s note: in fact, Unity uses C #, which is similar to C ++ ).
- Data types and variables. The code is based on data that is stored in variables. You can declare a variable like this:
int i = 0;
int is the data type, i is the variable name, = 0 is the value of the variable.
Frequently used data types:
int and long - for integers,
float and double - for floating-point numbers,
string - string data. - Conditions. Using the if statement, you can create conditions for executing code:
if (true){ // истина всегда истинна! doThings(); // я внутри выражения, и я выполняюсь! }
Using the else operator , you can extend the condition and display what to do if the condition is not true:int i = 1; if (i == 0){ doThings(); } elseif (i == 1){ doOtherThings(); // теперь запускаюсь я! }
- For / while loops While loops repeat parts of the code as long as the condition remains true. As soon as the condition ceases to be true, the cycle ends.
while (someBool == true){ // условие doThings(); // Буду выполняться, пока условие истинно }
For-loops are like while-loops. For while, we write this:int i = 0; while (i < condition){ doThings(); i++; // счётчик, по которому цикл продолжается }
The equivalent for-cycle will be as follows:for (int i = 0; i < condition; i++){ doThings(); }
- Data structures We have data to interact with. In addition, they can be stored in a special structure - an array, list, queue, stack or set.
A simple array example:/* Допустим, у нас есть числа от 0 до 9. Будем хранить их в массиве! */int[] arr = newint[10]; /* Скобки [] нужны для объявления массива. Мы присваиваем массив из 10 элементов переменной arr. Теперь он выглядит так: arr = [ 0 0 0 0 0 0 0 0 0 0 ] */for (int i=0; i<10; i++){ arr[i]=i; // Мы присваиваем позиции i значение i.//Заметили, что счёт начинается с нуля? } /* После цикла наш массив будет выглядеть так: arr = [ 0 1 2 3 4 5 6 7 8 9 ] */
- Functions and exceptions. A function is a short line of code that replaces a huge number of lines of code. For example, display the function EatBread () , which contains the following:
voidEatBread(){ //<---это функция breadAte=true; printf("Я ОЩУЩАЮ УГЛЕВОДЫ, ОНИ БЕГУТ ВНУТРИ МЕНЯ"); }
Then, when the function is output, two expressions are executed inside it.
If something goes wrong in the code, exceptions come to the rescue. They kind of say: “So, wait, here you did something illogical. Check back again. ”
What else you need to know:
- Tongue. What language will you program? Most often, games are written in C ++, JavaScript or C #. Languages differ in syntax and scope.
- API (Application Programming Interface). As soon as you familiarize yourself with the database, proceed to study the application programming interface for a specific game engine. They are a collection of useful tools packaged in simple classes and functions. The API greatly simplifies the programmer’s life.
- See examples of projects on the selected game engine. You can find many free examples of games on the Unreal and Unity engines. This will allow you to see the result and the whole process of work as a whole, as well as draw ideas for your future game.
A little inspiration. I know programming is scary at first. Everything seems meaningless, you constantly stumble and in the face of constant mistakes you want to give up, but this does not mean that programming is not for you.
The code is a challenge to yourself. And to understand nothing at first is normal.
Like any skill, programming needs to be learned. And maybe it will be quite interesting.
Other important programming fundamentals:
- Object Oriented Programming. Makes code more natural.
- Naming convention Name classes, methods, and variables so that you and other programmers understand their purpose. For example, call the melee attack function,
meleeAttack ()
but notmA()
orprotecbutalsoattac()
- Decomposition. Make a function from the duplicate code and call it instead of copying duplicate lines.
- Singleton Design Pattern ("Singleton"). A programming template that allows data to be stored in one place.
- Avoid static variables. In addition to using singletons, I avoided static variables — they only live for the duration of the game, are slow and can behave unpredictably.
- Observer Design Pattern ("Observer"). Allows an object to learn about the status of other objects without losing computer time to check.
Unity-specific things:
- Coroutines. Allow to begin to perform an action, continue for the desired time, and then stop. I use them for the visual effects of explosions, sudden movements.
- Class ScriptableObject. It stores data at lower cost than the base class MonoBehaviour.
Instruments
Game engines:
- Its engine in C / C ++. Low entry threshold. ( Translator's note: in fact, creating your own engine requires great effort and deep knowledge of the principles of programming ).
- Unity. Supports 2D / 3D. Requires knowledge of javascript / c #. The average entry threshold. Development for multiple platforms.
- Unreal. Supports 2D / 3D. Requires knowledge of C ++. The average entry threshold. Development for multiple platforms.
- pixi.js. 2D only. Requires JavaScript knowledge. The average entry threshold. Development for the browser.
- GameMaker Studio. Supports 2D / 3D. Requires knowledge of a special language engine GML (Game Maker Language). For newbies. Development for multiple platforms.
- Corona. 2D only. Requires knowledge of Lua (similar to JavaScript). For newbies. Development for multiple platforms.
Development environments:
- Visual Studio Code (for MacOS) - does not freeze, has built-in help information and convenient "hot" keys.
- Visual Studio (for Windows).
- MonoDevelop - installed with Unity, sometimes freezes.
Free Unity
Assets : There are a lot of free assets in the Unity Asset Store, bitbucket and GitHub. In my projects I use at least two. They simplify life, but are far from perfect. Noticed a mistake - correct and tell the developer about it.
- TextMeshPro .
- LeanTween .
- Fungus .
- Corgi Engine .
- Dialogue System .
- Post Processing Stack .
- Keijiro Takahashi is working on Unity, an open source visual effects project available.
An important, even the main source for solving problems with code is Google!
Sound
The board
Audio is able to create mood and immerse in the game, but it needs memory.
First decide: do you want sound? If so, will there be music, sound effects, voice acting or narration in the game.
In any case, you need to record and mix in such a way that the sound fits the mood of the game. For example, Bastion uses organic and instrumental sounds that fit well into the game world. Crypt of the Necrodancer included a mixture of electronic rhythms and eight-bit rock to convey the tempo and brightness of the game.
Immersion solves. If the sounds do not match the mood of the game, the player will be difficult to immerse in your world.
Instruments
Audio applications:
- Logic Pro (MacOS only). The price is 200 $.
- FL Studio. Price 99-899 $. There is a free demo version.
- Reaper. Price $ 60-225.
- Audacity. Free program. It has few features, but is useful for cleaning audio.
Creating retro effects:
- Chiptone .
- Bfxr .
- Leshy SFMaker .
- as3sfxr .
Free sounds:
- Soundcloud . Here you can find infinitely many sounds and music licensed under a Creative Commons license. Start with this playlist . You can use it for free, but do not forget to specify the authorship.
- Incompetech .
- Bensound .
Preparation for publication
The board
There is a small chance - at 99.99 percent, that there are mistakes in the game. And that means it's time to do a bug test.
How to test the game on bugs?
- Let others play the game. It is advisable together with you, in case they encounter an error and cannot understand or explain it.
- Check out the game on different platforms. There may be no problems in the editor, but does it work where it will be run? Be especially attentive with Linux and Android.
So, the bug is found. Now what?
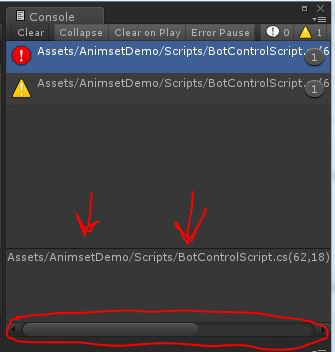
- Check the console for exceptions. If you find an exception, find the file and line where the exception worked. If it sounds like Martian, look for the solution on the network, and think about why an exception is triggered in this line.
- Write to the console. Try to display logs (system files) in the estimated places of error. Enter different variables and verify the values obtained with the expected ones. If there is a mismatch, correct it.
- Check the logs. The system records of your project will give more information than the console. Read the lines where the exception worked. Google everything you don't know.
- Sleep Everything will be fixed in the morning. This is just a bad dream :)
Typical mistakes
- NullReferenceException.
var.doThing(); //throws NullReferenceException: Object reference notsetto an instance of an object
What is the matter: the function is executed with a non-existent (null) variable.
Quick fix: check if the variable is null.if(var != null) { var.doThing(); // выполняем функцию безопасно! }
- SyntaxErrorException.
What is the matter: syntax errors.
Quick fix: the message indicates which character generated the error. Find and fix it.Note: Check which quotes you use.
"// correct quotes;
" // unnecessary quotes, they will bring you a lot of mistakes. - Pink or black screen.
Possible problem: the shader was not processed.
Possible reasons: you use 3D shaders in a 2D game or shaders that are not supported by the operating system. Make sure you use mobile shaders for mobile games.
When done with errors, proceed to optimize performance and memory usage. Users will be able to run the game faster and not experience problems with heating devices.
Optimization Tips
- Set the desired frame rate. For a visual novel, 20 frames per second will suffice, but for a shooter, 60 are needed. A low frame rate spends less time drawing.
- Animation / particle system / selective processing. Objects invisible to the player’s camera are not processed. Characters are animated, particles are updated, 3D-models are processed only in the field of view of the player.
- Compress textures and sounds. For texture compression, use Crunch . Streaming music and unpacking sound effects overload the game. Try to lower the audio quality. Compression can significantly reduce the quality of objects.
- Do not let Raycast touch extra objects. Raycast is like little rays firing from your fingers or mouse when you touch the screen or click. Find objects that should not react to player actions and remove them from Raycast calculations.
- Use the object pool. Frequent creation and deletion of a large number of objects reduces performance. Instead, combine them into a list, queue, or other structure. For example, bullets should be combined into one array.
- Optimize shaders. Set the material for each visualizer. The game does not have to create new materials at the beginning of the game, which will save resources. Let the visualizer include only what is functionally necessary.
- Use AssetBundles (literally "asset bundles") instead of the old system Resources in Unity. AssetBundles exports your files from Unity and puts them in one file, saving RAM.
Tools (Unity only)
Scripts:
Graphics:
Memory:
Platform Optimization:
- Guide to mobiles ;
- WebGL performance considerations ;
- Memory Considerations when targeting WebGL ;
- Olly's seven stages of optimizations for mobile VR.
Publication
The board
It's time to show the world your creation.
Promotion - the most exciting stage. If in doubt, the game design community will help you. Remember that you are not alone, and since you have gone through so much, you need to finish the job to the end.
You will not know if a game will become a hit until you publish it.
- Description
Make screenshots of the “About the game” pages and create descriptions for each platform on which you plan to release the game. - Networking
If you want everyone to learn about the game, write announcements for gaming media, participate in festivals and conferences.
Send a description of the game to the press a week before the release - give people time to write about it. It may happen that they will not write about it, but remember: journalists love beautiful stories about developers, a unique or controversial idea and a media kit.
Where to get addresses?- Find online contacts of authors that you like: mail, LinkedIn page, Twitter.
- Find the publication mail in the "About Us" section or at the bottom of the page.
Do not write to game publications that do not cover your genre or target gaming platform.
- Find online contacts of authors that you like: mail, LinkedIn page, Twitter.
- Streamers and video bloggers.
They will make a video of the game if:- The game will become popular on the platforms.
- You will write directly. Do not talk about yourself, briefly, beautifully and convincingly tell about the game. Use gifs and screenshots to attract attention.
Usually addresses of bloggers are listed on the page. If not, try to find contacts on the Internet.
A letter to Markiplier, a video blogger whose channel has more than 21 million subscribers
. - The game will become popular on the platforms.
- Social networks
This is a great tool for promotion: Agar.io gained popularity on 4chan, Butterfly Soup jumped in downloads after attention on Twitter.
Which is better: publish through the publisher or yourself. Want to follow the Devolver Digital Hotline, Miami, or learn from the Farmville and Doki Doki Literature Club?
To collaborate with a publisher , you must first find it. After that there will be a small pile of paperwork, but you will get enough funds to develop the game.
If you are going to publish yourself , get ready to spend a lot of time studying marketing. You can fail the promotion campaign, but in the process you will gain valuable knowledge and save money.
The number of installations of the game is growing.
I prefer to publish games on my own. I like to learn, and I believe that a really good game will be successful regardless of progress. - Click on the "Publish" button!
Happened! Now relax, take something tasty and relax. You worked hard and deserved it.
Do not worry, if the game has not received the expected attention - this is normal. My first game has only 255 downloads on Steam.
The main thing is that you made the game and learned a lot. Now this is enough, and there is always the opportunity to try again with new knowledge.
Instruments
The presskit () service helps developers make a description of the game for the press.
Publication platforms:
- Steam (PC) - $ 100 per publication.
- Origin (PC).
- GOG (PC) - free publication after permission.
- Mac App Store (MacOS) - $ 100 per year, an Apple developer account is required.
- itch.io (PC / Web) is a free publication.
- Game Jolt (PC / Web) - free publication.
- Armor Games - free publication.
- Kongregate (Web) - free publication.
- Newgrounds (Web) - free publication.
- GitHub (Web) is a free publication on a site ending on ___.github.io .
- Amazon (Web / Mobile) is a free publication.
- Google Play (Mobile) - $ 25 per publication.
- iOS App Store (Mobile) - Apple developer account required.
Magazines about games:
- DTF (in Russian).
- Kanobu (in Russian).
- IndieGames .
- Siliconera .
- FreeGamesPlanet .
- PCGamer .
- Kotaku .
- Rock Paper Shotgun .
- Polygon .
- Giant Bomb .
- EuroGamer .
Festivals:
- Independent Games Festival (IGF) . Submission of applications until October 1.
- Indiecade . International festival of indie games. Acceptance of applications until May – June.
- Swedish Game Awards . Swedish Game Prize. Call for applications until June.
- South by Southwest Festival (SXSW) . Call for applications until December.
- The Game Awards . Submission of applications until November.
Game Conferences:
- DevGAMM - held in Moscow.
- Game Developer's Conference (GDC) .
- Penny Arcade Expo (PAX) .
- Electronic Entertainment Expo (E3) .
- Tokyo Game Show .
Conclusion
There is no easy way to create a game. There is only your determination and effort.
For every Half-Life, Minecraft and Uncharted there are oceans of blood, sweat and tears.
Ken Levine, creator of Bioshock
You will make mistakes, feel at a dead end and cry. This is normal - it means you are growing above yourself.
From the Editor
- Online course " Game design from scratch: Become a professional in the gaming industry "
- Online course " Product Creation: Analytics, Development, Promotion "
- Online profession " Python-developer "
- Online Profession " Ruby on Rails Developer "
- Online course " PR to digital: strategy, reputation, tools "
- Online course " Design of mobile applications: interfaces, architecture, visual concept "