Java command line interfaces: picocli
- Transfer
Hello!
The next start of the “Java Developer” group , where we all continue our experiment that the learning process does not have to be continuous (“Should the countermeasure be continuous?”). That is, we slightly reworked and shuffled the program, and we divided it into three steps, which can be easily passed with a break between them. In general, it is interesting both for students and for us, if only no one was discouraged, otherwise the program became even a bit more complicated, although it was not easy before. Well, traditionally an interesting article related to our course.
Go!
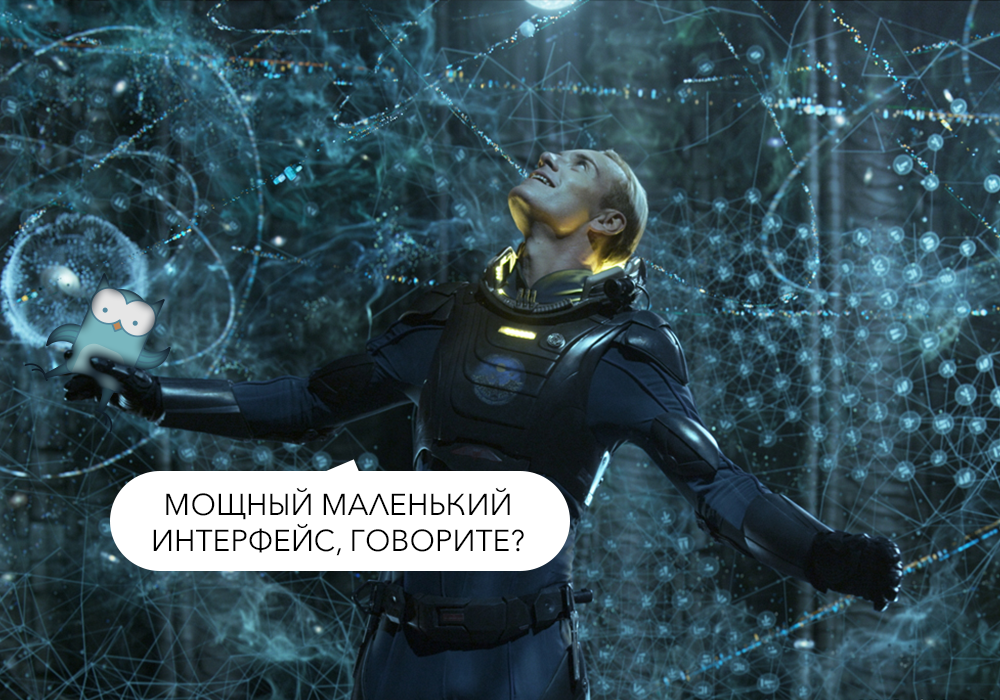
On the official website, picocli is described as “a powerful little command line interface”, which “is a single-file Java framework for parsing command line arguments and creating flawless, easily customizable auxiliary messages. With flowers". This post presents a brief overview of Picocli 0.9.7 and its use for parsing command line arguments in Java code.
Like other command line processing libraries written in Java, which are described in this series, picocli is open source . Since all picocli is implemented in a single java file, if you prefer, you can easily use the source code directly. The picocli website emphasizes that “picocli’s distinctive feature is that it allows users to run applications using picocli without requiring picocli itself as an external dependency: all source code is placed in one file to encourage application authors to include it in the program source code” . If you prefer to use picocli as a library, in the Maven Repository has a JAR-file with the set of compiled .class files (Picocli represents one Java-file, but contains numerous sub-classes and annotation s).
The easiest way to get an idea of the Picocli single-file nature is to take and look at the file itself. The source code for CommandLine.java is available on the Picocli download page . The following two screenshots show the output from javap when executing the CommandLine class and when executing one of its internal annotations and one of its internal classes.
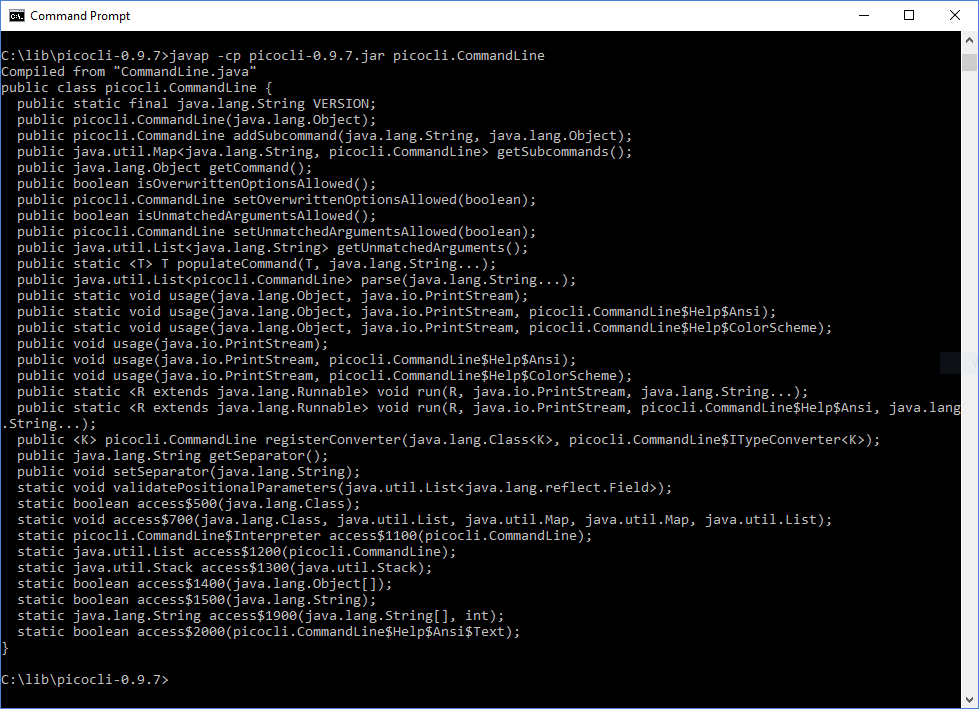
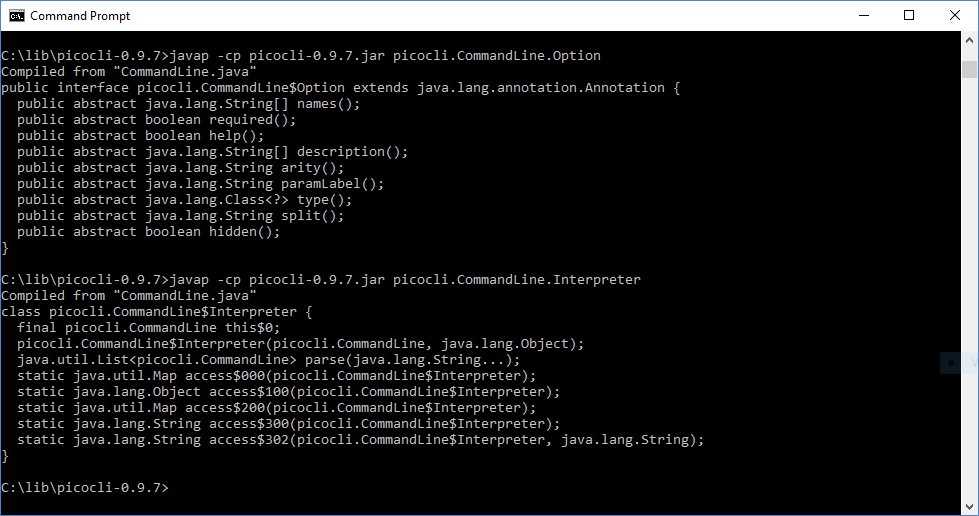
Regardless of whether we compile CommandLine.java into our own class / JAR file or use the already compiled JAR from Maven, the source code of the application using Picocli will obviously be the same. The “definition” stage in parsing arguments using Picocli is performed by annotating the fields of the instance that will store the values associated with the command line parameters. This is shown in the code snippet below.
“Definition” stage in Picocli
The above code example demonstrates that Picocli allows you to specify several flag names (in my example, single-character names with one hyphen and multi-character names with two hyphens are indicated). This example also shows that required = options can be set to required = true, and help = true can be specified for additional options such as printing usage details and eliminating errors related to the lack of required parameters. Note that in Picocli 0.9.8 additional support has been added for more specific types of auxiliary messages versionHelp and usageHelp .
The “parse” stage in Picocli is performed in CommandLine.populateCommand (T, String ...)where T is an instance of a class with Picocli-annotated fields, and the remaining lines are the arguments to be analyzed. This is shown in the following code snippet.
The "parsing" stage in Picocli
The “polling” stage in Picocli consists in simply referring to Picocli-annotated instance fields, which were passed to the CommandLine.populateCommand (T, String ...) method at the “parsing” stage. A simple example of such a “survey” is shown in the following listing.
The “polling” stage in Picocli
Displaying auxiliary messages or usage information to a user when the command line says -h or --help is as easy as “polling” the Picocli-annotated field for which help = true was specified to determine if this boolean value is set or not and, if installed, call one of the overloaded methods of the Command.usage. I had to use one of the static versions of this method, as shown in the following listing.
Auxiliary messages / usage information in Picocli
The following several screenshots demonstrate a simple application written using picocli. The first screenshot shows the type of error message and the stack trace if the required flag is missing. The second screenshot shows how the long and short names listed in the annotations are used. The third image shows the function of displaying auxiliary messages in action.
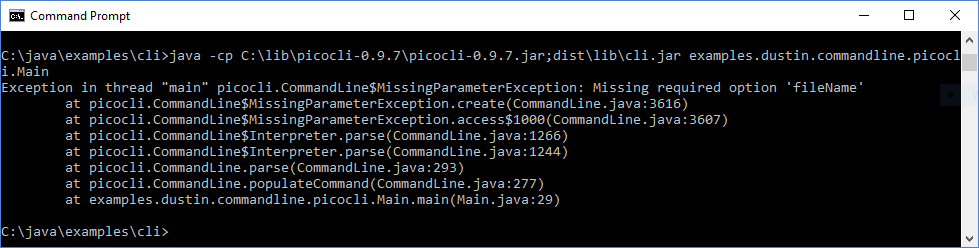

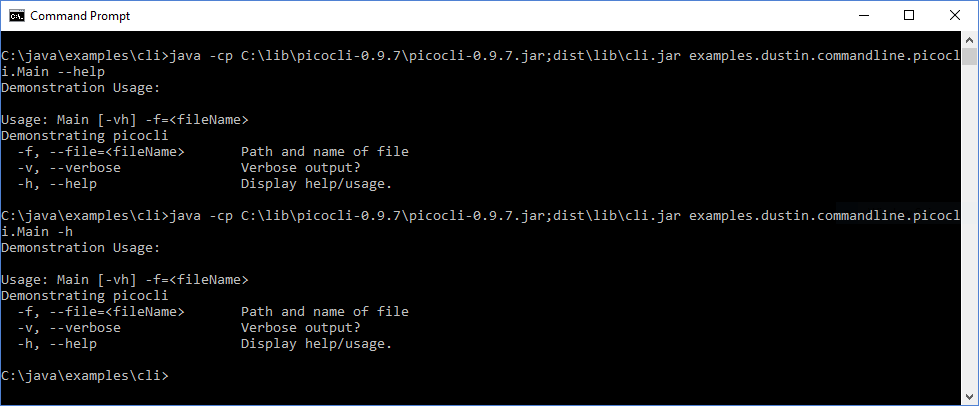
One of the additional features of Picocli, which is absent in many other libraries of parsing command line arguments written in Java, is support for color syntaxbut. In the first listing of this post were the lines defined in the annotations with @ | | @ syntax. In the screenshot above, which demonstrates the use of auxiliary messages, these characters were transferred as is, without special processing. However, if I run this sample code in Cygwin, I will see what these signs do.

In the screenshot above, we see that Picocli automatically applied the color syntax (yellow and white) to the options for auxiliary messages and also applied a bold and underlined bold syntax to areas describing the auxiliary messages, where the syntax @ | | @.
The following are additional features of Picocli that should be considered when choosing a framework or library to help with parsing command line arguments in Java.
The listings in this post are fully accessible on GitHub .
Picocli is a supported and updated library for parsing Java command line arguments. It contains several new features and approaches from some of the other available command line processing libraries, written in Java, but a couple of different features have been added to it (such as the color syntax and the whole library encapsulated into one original Java file). Picocli is fairly easy to use and attractive by itself, but most likely it will stand out among other libraries for an individual developer if this developer wants to support color syntax or the ability to add a source code file to a developer project without additional JAR files or compiled ones. class files.
THE END
As always, we are waiting for your questions and comments.
The next start of the “Java Developer” group , where we all continue our experiment that the learning process does not have to be continuous (“Should the countermeasure be continuous?”). That is, we slightly reworked and shuffled the program, and we divided it into three steps, which can be easily passed with a break between them. In general, it is interesting both for students and for us, if only no one was discouraged, otherwise the program became even a bit more complicated, although it was not easy before. Well, traditionally an interesting article related to our course.
Go!
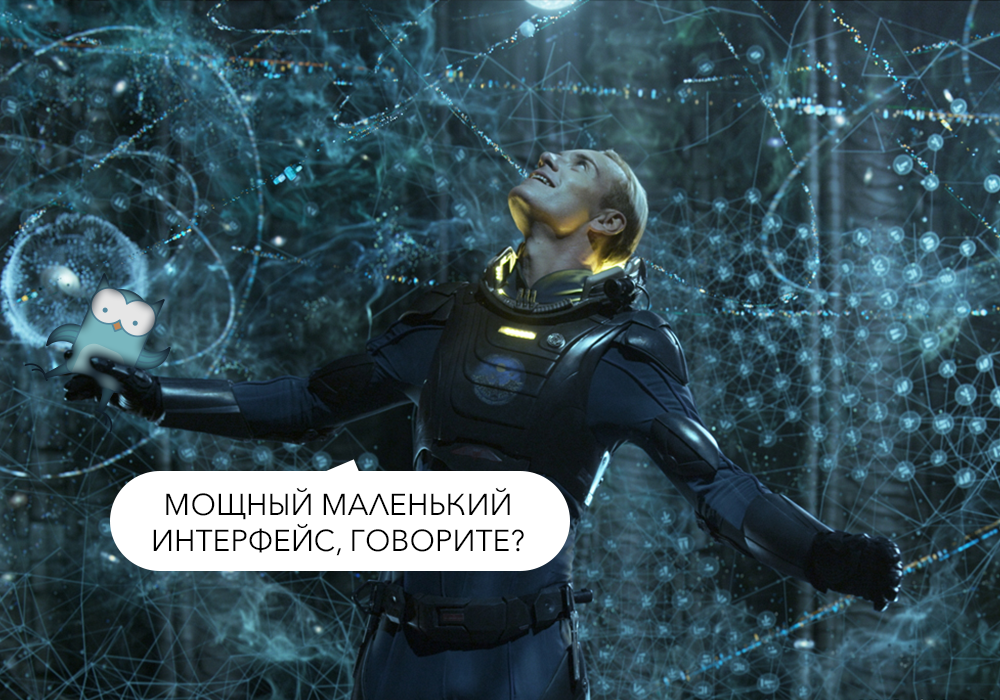
On the official website, picocli is described as “a powerful little command line interface”, which “is a single-file Java framework for parsing command line arguments and creating flawless, easily customizable auxiliary messages. With flowers". This post presents a brief overview of Picocli 0.9.7 and its use for parsing command line arguments in Java code.
Like other command line processing libraries written in Java, which are described in this series, picocli is open source . Since all picocli is implemented in a single java file, if you prefer, you can easily use the source code directly. The picocli website emphasizes that “picocli’s distinctive feature is that it allows users to run applications using picocli without requiring picocli itself as an external dependency: all source code is placed in one file to encourage application authors to include it in the program source code” . If you prefer to use picocli as a library, in the Maven Repository has a JAR-file with the set of compiled .class files (Picocli represents one Java-file, but contains numerous sub-classes and annotation s).
The easiest way to get an idea of the Picocli single-file nature is to take and look at the file itself. The source code for CommandLine.java is available on the Picocli download page . The following two screenshots show the output from javap when executing the CommandLine class and when executing one of its internal annotations and one of its internal classes.
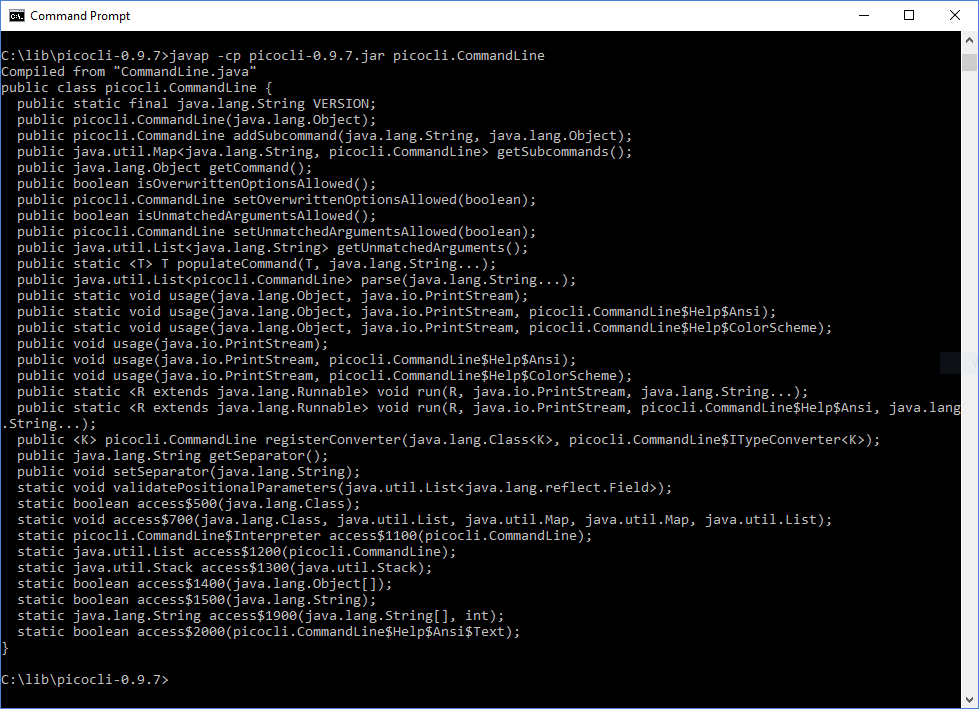
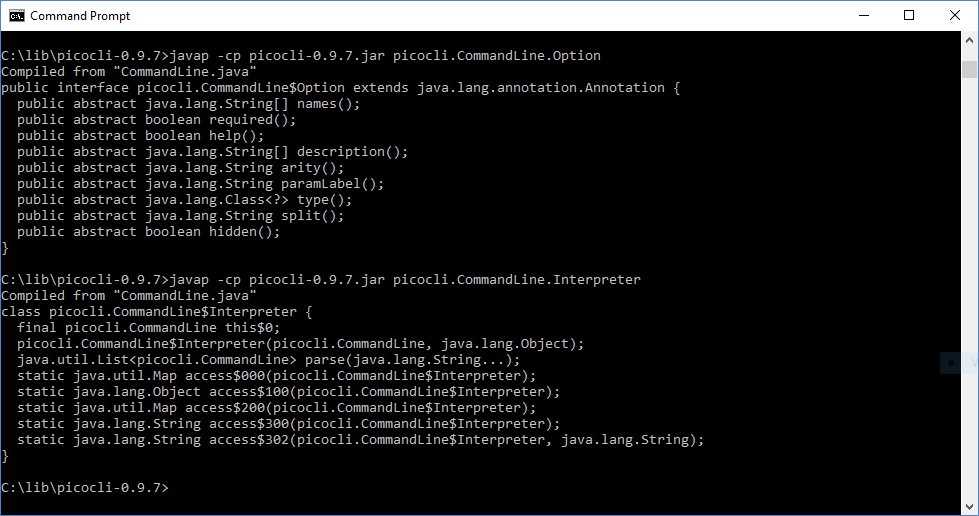
Regardless of whether we compile CommandLine.java into our own class / JAR file or use the already compiled JAR from Maven, the source code of the application using Picocli will obviously be the same. The “definition” stage in parsing arguments using Picocli is performed by annotating the fields of the instance that will store the values associated with the command line parameters. This is shown in the code snippet below.
“Definition” stage in Picocli
/*
Демонстрирует обработку командной строки Java с помощью picocli.
*/@Command(
name="Main",
description="Demonstrating picocli",
headerHeading="Demonstration Usage:%n%n")
publicclassMain{
@Option(names={"-v", "--verbose"}, description="Verbose output?")
privateboolean verbose;
@Option(names={"-f", "--file"}, description="Path and name of file", required=true)
private String fileName;
@Option(names={"-h", "--help"}, description="Display help/usage.", help=true)
boolean help;
The above code example demonstrates that Picocli allows you to specify several flag names (in my example, single-character names with one hyphen and multi-character names with two hyphens are indicated). This example also shows that required = options can be set to required = true, and help = true can be specified for additional options such as printing usage details and eliminating errors related to the lack of required parameters. Note that in Picocli 0.9.8 additional support has been added for more specific types of auxiliary messages versionHelp and usageHelp .
The “parse” stage in Picocli is performed in CommandLine.populateCommand (T, String ...)where T is an instance of a class with Picocli-annotated fields, and the remaining lines are the arguments to be analyzed. This is shown in the following code snippet.
The "parsing" stage in Picocli
final Main main = CommandLine.populateCommand(new Main(), arguments);
The “polling” stage in Picocli consists in simply referring to Picocli-annotated instance fields, which were passed to the CommandLine.populateCommand (T, String ...) method at the “parsing” stage. A simple example of such a “survey” is shown in the following listing.
The “polling” stage in Picocli
out.println(
"The provided file path and name is " + main.fileName
+ " and verbosity is set to " + main.verbose);
Displaying auxiliary messages or usage information to a user when the command line says -h or --help is as easy as “polling” the Picocli-annotated field for which help = true was specified to determine if this boolean value is set or not and, if installed, call one of the overloaded methods of the Command.usage. I had to use one of the static versions of this method, as shown in the following listing.
Auxiliary messages / usage information in Picocli
if (main.help)
{
CommandLine.usage(main, out, CommandLine.Help.Ansi.AUTO);
}
The following several screenshots demonstrate a simple application written using picocli. The first screenshot shows the type of error message and the stack trace if the required flag is missing. The second screenshot shows how the long and short names listed in the annotations are used. The third image shows the function of displaying auxiliary messages in action.
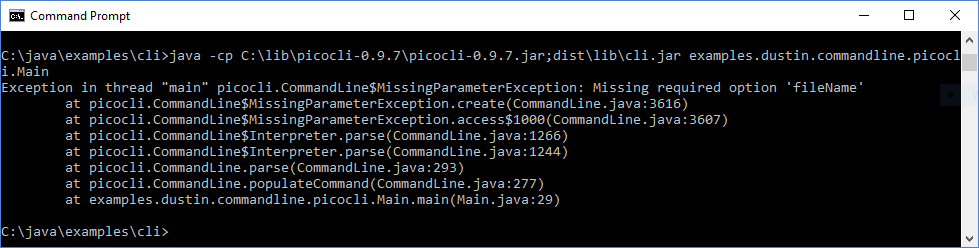

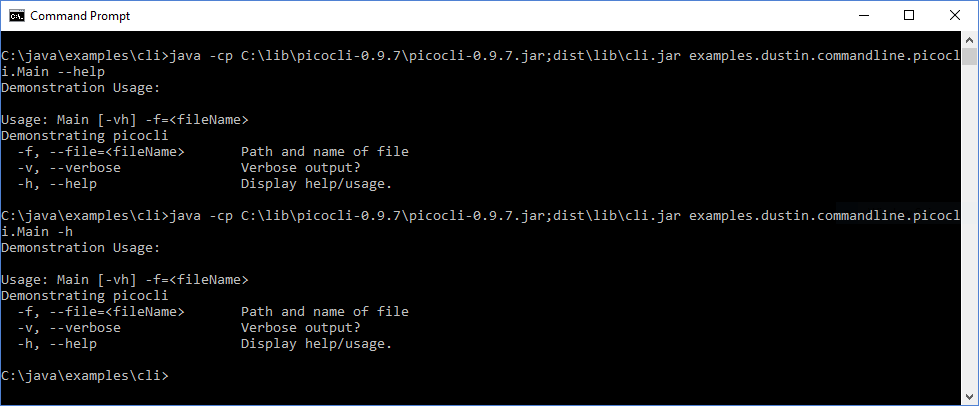
One of the additional features of Picocli, which is absent in many other libraries of parsing command line arguments written in Java, is support for color syntaxbut. In the first listing of this post were the lines defined in the annotations with @ | | @ syntax. In the screenshot above, which demonstrates the use of auxiliary messages, these characters were transferred as is, without special processing. However, if I run this sample code in Cygwin, I will see what these signs do.

In the screenshot above, we see that Picocli automatically applied the color syntax (yellow and white) to the options for auxiliary messages and also applied a bold and underlined bold syntax to areas describing the auxiliary messages, where the syntax @ | | @.
The following are additional features of Picocli that should be considered when choosing a framework or library to help with parsing command line arguments in Java.
- Picocli is an open source project with an Apache 2.0 license.
- Picocli does not require the download of third-party libraries or frameworks.
- All Picocli source code is contained in a single .java file, and this code can be copied and pasted into its own configuration management system and assembled with the rest of the application code, which means that even a Picocli JAR file is not necessary.
- The CommandLine.java (Picocli 0.9.7) source code file is just over 3700 lines (including spaces and comments) and weighs almost 200 KB. The picocli-0.9.7.jar file weighs about 83 KB.
- Picocli timely and frequently updated. Version 0.9.8 was released yesterday (after I wrote most of this post).
- Picocli has very detailed documentation and is in many ways more up-to-date than the documentation for some of the other command line processing libraries written in Java.
- Picocli's color syntax support is easy to use, and the color syntax usage reference across platforms is documented in the Supported Platforms section.
- Using Picocli annotations for instance-level fields is similar to using annotations in some other command line processing libraries and has the same advantages.
- The basic features of Picocli are very convenient and easy to learn, but Picocli also supports the ability to customize several aspects of command line processing using Picocli.
The listings in this post are fully accessible on GitHub .
Picocli is a supported and updated library for parsing Java command line arguments. It contains several new features and approaches from some of the other available command line processing libraries, written in Java, but a couple of different features have been added to it (such as the color syntax and the whole library encapsulated into one original Java file). Picocli is fairly easy to use and attractive by itself, but most likely it will stand out among other libraries for an individual developer if this developer wants to support color syntax or the ability to add a source code file to a developer project without additional JAR files or compiled ones. class files.
THE END
As always, we are waiting for your questions and comments.