
MintEye CAPTCHA solution in 23 lines of code
- Transfer
As an avid HAD reader, I was interested in this post describing how to hack MintEye audio captcha . The graphical version also looked pretty interesting, so I thought it would be fun to crack it too.
Here is one example of a MintEye graphic captcha:
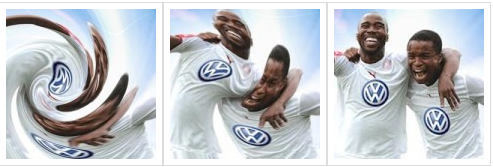
For the solution, you just need to move the slider and select the undistorted version of the image. In the comments on HAD, there were several rather naive in my opinion suggestions for solving a captcha based on the search for straight lines. However, such options will not work with examples similar to the above (i.e. containing a small number of straight lines).
After some thought, I found a good, reliable and, surprisingly, simple way to solve the captcha. Here is his entire implementation in Python:
(Please note that most of the code relates to opening the image and plotting. Functions such as automatic image parsing and returning the finished result will be left to spammers as homework).
The essence of the method is as follows: the larger the image is “twisted”, the longer the border. This can be seen in the picture above, but there is a more obvious example:

Pay attention to the increase in the length of the black rectangle. In order to use this, we need to calculate the sum of the lengths of the borders of the image. The simplest way to do this is to take a derivative of the image (the Sobel operator is used in the implementation proposed above) and add the results (it is recommended to read the articleabout how the Sobel operator works). Then we just select the image with the smallest “border length” - it will be the right answer.
The results are impressive, 13 of the 13 captcha I downloaded were resolved correctly in this way. These graphs show the image number on the X axis and the “border length” on the Y axis. The red dot indicates the correct answer. Interestingly, the often completely undistorted image on the chart is displayed as a peak. This usually means that we missed with the correct answer to one picture left or right (which, however, is accepted by MintEye as the correct answer). This usually happens because the undistorted image in some places looks sharper and the borders are highlighted more precisely, which affects the final sum of the lengths.


In conclusion, I would like to say that this CAPTCHA by its architecture is not resistant to hacking. A simple “twisting” of an image can always be easily noticed by the method described above, regardless of the original image.
Here is one example of a MintEye graphic captcha:
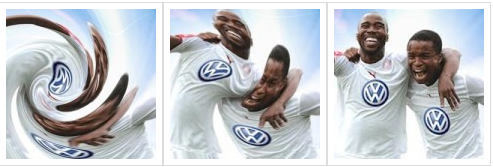
For the solution, you just need to move the slider and select the undistorted version of the image. In the comments on HAD, there were several rather naive in my opinion suggestions for solving a captcha based on the search for straight lines. However, such options will not work with examples similar to the above (i.e. containing a small number of straight lines).
After some thought, I found a good, reliable and, surprisingly, simple way to solve the captcha. Here is his entire implementation in Python:
import cv2
import sys
import numpy as np
import os
import matplotlib.pyplot as plt
if __name__ == '__main__':
for dir in range(1,14):
dir = str(dir)
total_images = len(os.listdir(dir))+1
points_sob = []
for i in range(1,total_images):
img = cv2.imread(dir+'/'+str(i)+'.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
sob = cv2.Sobel(gray, -1, 1, 1)
points_sob.append(np.sum(sob))
x = range(1,total_images)
res = np.argmin(points_sob)+1
print res
plt.plot(res,points_sob[res-1], marker='o', color='r', ls='')
plt.plot(x, points_sob)
plt.savefig(dir+'.png')
plt.show()
(Please note that most of the code relates to opening the image and plotting. Functions such as automatic image parsing and returning the finished result will be left to spammers as homework).
The essence of the method is as follows: the larger the image is “twisted”, the longer the border. This can be seen in the picture above, but there is a more obvious example:

Pay attention to the increase in the length of the black rectangle. In order to use this, we need to calculate the sum of the lengths of the borders of the image. The simplest way to do this is to take a derivative of the image (the Sobel operator is used in the implementation proposed above) and add the results (it is recommended to read the articleabout how the Sobel operator works). Then we just select the image with the smallest “border length” - it will be the right answer.
The results are impressive, 13 of the 13 captcha I downloaded were resolved correctly in this way. These graphs show the image number on the X axis and the “border length” on the Y axis. The red dot indicates the correct answer. Interestingly, the often completely undistorted image on the chart is displayed as a peak. This usually means that we missed with the correct answer to one picture left or right (which, however, is accepted by MintEye as the correct answer). This usually happens because the undistorted image in some places looks sharper and the borders are highlighted more precisely, which affects the final sum of the lengths.


In conclusion, I would like to say that this CAPTCHA by its architecture is not resistant to hacking. A simple “twisting” of an image can always be easily noticed by the method described above, regardless of the original image.