
Tricks of the developer for iOS. Splash screen
- From the sandbox
- Tutorial
Introduction
Greetings, habravchane!
During the development of applications for iOS, I have accumulated some tricks that I would like to share with you.
I’ll immediately warn you that I try to keep up with the times, so the examples will be under iOS> = 5.0 and use Storyboard and ARC, but nothing prevents them from being ported to 4. *.
If you have been developing for iOS for a long time, most of what I said will be obvious to you, but at the same time I would like you to join the discussion and tell how you implement this.
To those who are interested, I ask for a cut (The post contains screenshots of the Interface Builder areas, so I warn about traffic).
Splash screen
By default, iOS provides a mechanism for displaying an application’s download image in the form of specifying png files for specific permissions, you will not surprise anyone now. But iOS is famous for its transitions, animations and more, it looks cool and thanks to the Core Animation API it is not at all difficult.
I always liked the Skype.app start screen, when the application logo went up after loading, and input elements appeared on the screen. Nice and easy.
Using the Storyboard, the task becomes trivial:
Suppose we have some screen that we get on when the application starts:
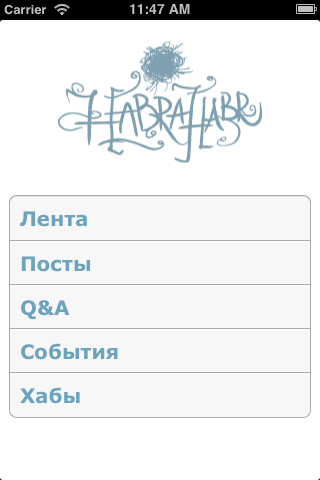
And our Default.png looks something like this:
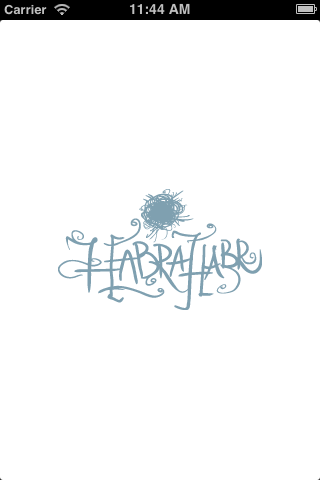
If we start the application now, then first we will see Default.png, and then immediately get to the start screen. It doesn’t look very, right? To fix this, we will create another screen, the initial state of which will be identical in appearance to our Default.png and will contain the outlet UIImageView. make it the starting one and indicate the base class, for example, SplashScreenViewController:

View is very simple, background + UIImageView with the logo in the center. It will be connected to the SplashScreenViewController through an outlet called imageView.
Now create a Segue from the newly created screen to the start screen.
For those who are just studying the Storyboard, or just forgot, I remind you that in order to create a Segue not by event, but simply as a connection, you should start pulling it from the StatusBar of the original ViewController and calling it manually (as I will show later in code):
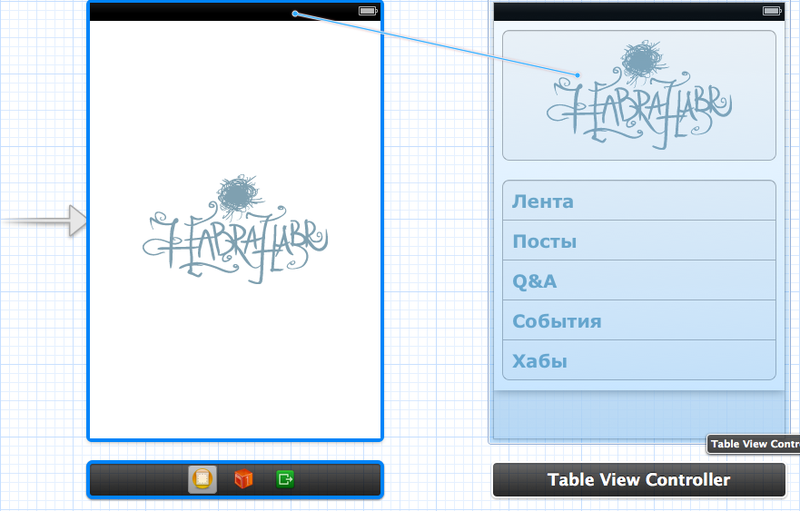
Parameters:
- identifier: splash
- Style: Custom
- Segue Class: SplashSegue (we will create this class a bit later)
The code for this example will look something like this:
@interface SplashSegue : UIStoryboardSegue
@end
@interface SplashScreenViewController : UIViewController
@property IBOutlet UIImageView *imageView;
@end
@implementation SplashScreenViewController
- ( void ) viewDidAppear:(BOOL)animated
{
[super viewDidAppear:animated];
// Анимируем стандартными средствами Cocoa
[UIView animateWithDuration:1.0 delay:0.2 options:0
animations:^
{
// Сделаем простую анимацию смещения логотипа вверх (в моём примере
// логотип встаёт на позицию, которая совпадает с онной в стартовом экране,
// чтобы переход не был заметен
CGRect frame = self.imageView.frame;
frame.origin.y = 15.0;
self.imageView.frame = frame;
}
completion:^( BOOL completed )
{
// По окончанию анимации выполним наш переход к стартовому экрану
[self performSegueWithIdentifier:@"splash" sender:self];
}];
}
@end
@implementation SplashSegue
- ( void ) perform
{
// Для перехода к стартовому экрану будем использовать стандартный presentModalViewController.
// Обратите внимание на параметр animated, я намеренно установил его в NO, т.к. анимацию перехода
// мы выполнили заранее.
[[self sourceViewController] presentModalViewController:[self destinationViewController] animated:NO];
}
@end
Now, if we launch our application, first we will see the logo with Default.png, then (invisibly to the user) the screen will change to our SplashScreen, the animation of the logo will play upward, after which the screen will change to the start screen as well.
This simple-looking task of animating the transition from the boot screen to the start screen teaches us:
- Programmatically create Segue between screens in Storyboard
- Implement your own Segue logic
- Don’t be afraid to use Cocoa’s built-in tools and do what is evaluated by human days for human hours :)
I myself have been working on this platform relatively recently and sometimes I miss such lessons, and I’m sure that there will be those who will also find it useful, so do not judge strictly if it turned out to be useless for you;)
UPD:
Added a video demonstration of the effect in the emulator