
Selenide: convenient tests on Selenium WebDriver
- Tutorial
Many have heard about Selenium WebDriver - one of the most popular tools for writing acceptance / integration tests.
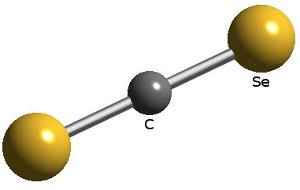
Using Selenium, we very quickly noticed that we had to write the same code from time to time to initialize the browser at the beginning, close it at the end, take screenshots after each fallen test, etc. ( pruflink ).
Therefore, we decided to separate this repeating code into a separate library. So Selenide was born .
Selenide is a wrapper around Selenium WebDriver that allows you to quickly and easily use it when writing tests, focusing on the logic and not the fuss with the browser.
Here is an example test. As you can see, the code is minimal. Called "open" - and the browser opened.
When the open method is called, Selenide launches the browser itself and opens the localhost : 8080 / login page (the port and host are configured, of course). It also takes care that the browser closes at the end.
Selenide provides additional methods for actions that cannot be done with the Selenium WebDriver team. This is selecting a radio button, selecting an item from the drop-down list, creating a screenshot, clearing the browser cache, etc.
And the Ajax question stands apart: when testing applications using Ajax, you have to invent code that is waiting for something (when the button turns green). Selenide provides a rich API to wait for various events to occur:
1. Add a Selenide dependency to your project:
2. Import a couple of classes:
Done! Write tests, edren-loaf!
Yes, we at our company use Selenide in several real projects:
So you can be sure that the project is not crude, it is actually used and maintained.
There is also a small reference open-source project that uses Selenide: the Hangman game .
The Selenium library took its name from the chemical element (Selenium). And selenides are compounds of selenium with other elements.
Here we have it:
Chemical to health!
UPD . In March 2013, Selenide 2.0 was released, in which the API was greatly updated. Using Selenide is now even easier and more convenient.
And we also launched a website in Russian: ru.selenide.org
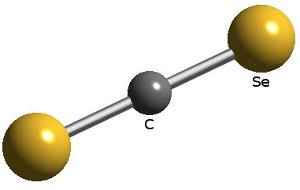
Using Selenium, we very quickly noticed that we had to write the same code from time to time to initialize the browser at the beginning, close it at the end, take screenshots after each fallen test, etc. ( pruflink ).
Therefore, we decided to separate this repeating code into a separate library. So Selenide was born .
What is Selenide
Selenide is a wrapper around Selenium WebDriver that allows you to quickly and easily use it when writing tests, focusing on the logic and not the fuss with the browser.
Here is an example test. As you can see, the code is minimal. Called "open" - and the browser opened.
@Test
public void testLogin() {
open("/login");
$(By.name("user.name")).sendKeys("johny");
$("#submitButton").click();
waitUntil(By.id("username"), hasText("Hello, Johny!"));
$("#username").shouldHave(cssClass("green-text"));
assertThat($("#insuranceDetailsHeader").getText(), equalTo("Страховые полисы"));
assertThat($$("#paymentScheduleTable tr").size(), equalTo(7));
}
When the open method is called, Selenide launches the browser itself and opens the localhost : 8080 / login page (the port and host are configured, of course). It also takes care that the browser closes at the end.
Extra goodies Selenide
Selenide provides additional methods for actions that cannot be done with the Selenium WebDriver team. This is selecting a radio button, selecting an item from the drop-down list, creating a screenshot, clearing the browser cache, etc.
@Test
public void canFillComplexForm() {
open("/client/registration");
setValue(By.name("user.name"), "johny");
selectRadio("user.gender", "male");
selectOption(By.name("user.preferredLayout"), "plain");
selectOptionByText(By.name("user.securityQuestion"), "What is my first car?");
followLink(By.id("submit"));
takeScreenShot("complex-form.png");
}
@Before
public void clearCache() {
clearBrowserCache();
}
And the Ajax question stands apart: when testing applications using Ajax, you have to invent code that is waiting for something (when the button turns green). Selenide provides a rich API to wait for various events to occur:
@Test
public void pageUsingAjax() {
waitFor("#username");
waitUntil("#username", hasText("Hello, Johny!"));
waitUntil("#username", hasAttribute("name", "user.name"));
waitUntil("#username", hasClass("green-button"));
waitUntil("#username", hasValue("Carlson"));
waitUntil("#username", appears);
waitUntil("#username", disappears);
}
I want to try, where to start?
1. Add a Selenide dependency to your project:
com.codeborne selenide 1.6
2. Import a couple of classes:
include static com.codeborne.selenide.Navigation.*
include static com.codeborne.selenide.DOM.*
Done! Write tests, edren-loaf!
Does anyone really use this?
Yes, we at our company use Selenide in several real projects:
- Java + ANT + JUnit
- Java + Gradle + JUnit
- Scala + ANT + ScalaTest
So you can be sure that the project is not crude, it is actually used and maintained.
There is also a small reference open-source project that uses Selenide: the Hangman game .
Where does this name come from - Selenide?
The Selenium library took its name from the chemical element (Selenium). And selenides are compounds of selenium with other elements.
Here we have it:
- Selenide = Selenium + JUnit
- Selenide = Selenium + TestNG
- Selenide = Selenium + ScalaTest
- Selenide = Selenium + anything
Chemical to health!
UPD . In March 2013, Selenide 2.0 was released, in which the API was greatly updated. Using Selenide is now even easier and more convenient.
And we also launched a website in Russian: ru.selenide.org