
Programming for PlayStation 2: CD (DVD) -ROM Library - Part One
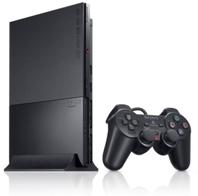
1. I apologize for not writing for a long time.
2. Ready PS2 SDK.
I wanted to watch out for the CD (DVD) -ROM Library, so you will find links and a guide for installing the PS2 SDK at the end of the second part of this article.
The topic is quite complicated.
PS2 SDK is available here . Narod.ru .
Magnet (link is not inserted normally thanks to the Habr parser): magnet:? Xt = urn: btih: F6114E45C5E392E6D213F2547C466FADB5DA5A13 & dn = sce.zip & tr = udp% 3a // tracker.openbittorrent.com% 3a80 / announce
Another link . Thanks Konstantinus and ahmpro
CD (DVD) -ROM Library.
Both IOP and EE are used to work with the drive.
The library responsible for working with the drive is called "libcdvd" (that is).
EE library to connect: "libcdvd.a".
Header file: "libcdvd.h".
IOP library to connect: "cdvdman.ilb".
IOP modules: “cdvdman.irx” and “cdvdfsv.irx”.
Sectors
The normal sector size for reading data is 2048 bytes for a DVD.
There is only one mode for them.
Well, the standard size for CDs (also 2048). However, the sector may be a different size, but at the moment, it does not matter.
Rotation speed and data transfer
PS2 uses the CAV method, so disk speed is maintained and is constant. Thus, the speed of reading data will be different on the external and internal tracks.
- CD, internal tracks - 10 speed = 1500 Kb / s.
- CD, external tracks - 24 speed = 3600 KB / s.
- DVD, internal tracks - 1.6 speed = 2000 Kb / s.
- DVD, external tracks - 4 speed = 5000 Kb / s.
Oddly enough, the rotation speed can be controlled in the following three ways:
- Using streaming mode (SCECdSpinStm): the rotation speed is controlled in such a way that reading can be performed reliably at a constant data rate.
- Normal data mode (SCECdSpinNom): at the beginning, an attempt will be made to read at maximum speed and, if the read fails, the speed will be reduced to the one at which reading will be normal.
- Dual-layer disc mode (SCECdSpinDvdDL0): the same as streaming mode, only for a dual-layer disc. Speed is read from track zero.
File system
The file system is used in ISO-9660 format.
There is a limit on the number of files and directories, however, it can be increased due to the number of letters in the names of files and folders.
The number of directories in a folder: 40. The
maximum nesting level: 8. The
number of files in a directory: 30.
The names of files and folders should consist only of numbers, letters of the English alphabet and underscore. All folder and file names must be uppercase.
A file name can include no more than 8 characters in the file name and 3 characters in the extension.
The folder name can include no more than 8 characters.
Work with disk
To read a disk, you need to access it and specify the mode.
After the processor checks it for legality. =)
Then again you need to access it.
After initializing the program, you need to initialize the drive with the sceCdInit () function.
The sceCdInit function accepts one of the following parameters:
- SCECdINIT - initializing the library, blocking access and waiting for the next command.
- SCECdINoD - initialization only.
- SCECdEXIT - closing the library.
The function returns a number: 0 - initialization failed, 1 - initialization went fine, 2 - initialization has already been completed.
After that, you need to specify the drive mode with the sceCdMmode () function, which accepts one of the two SCECdCD or SCECdDVD parameters for CD and DVD discs, respectively. Returns 1 or 0, which corresponds to the result or not.
Example:
int main(){
sceSifInitRpc(0);
sceCdInit(SCECdINIT); /* Требуется после страта с CD/DVD */
sceCdMmode(SCECdCD); /* Тип диска = CD-ROM */
/* Замена модуля по умолчанию */
while ( !sceSifRebootIop(IOPRP) );
while( !sceSifSyncIop() );
/* Инициализируем заного */
sceSifInitRpc(0);
sceCdInit(SCECdINIT);
sceCdMmode(SCECdCD); /* Тип диска = CD-ROM */
sceFsReset();
/* последующий код… */
* This source code was highlighted with Source Code Highlighter.
Reading files
To read a file, you first need to find out which sector it is on.
To do this, there is a sceCdSearchFile () function that takes the following arguments:
- Pointer to the file structure of the drive (type sceCdIFILE). It stores the LSN (logical sector number) and file size after the function runs.
- The absolute path to the file with the name and extension.
Returns 0 or 1, which means the file was not found or was found, respectively.
Those. something like this: ret = sceCdSearchFile (& fp, "\\ SYSTEM.CNF");
This information is cached.
There is a function sceCdRead () for reading a file, which takes the following parameters: LSN, number of sectors to read, buffer for reading, type of reading.
You need to remember that the function is not blocking, so you can find out that the data transfer is completed using the sceCdSync () function.
The sceCdSync () function accepts one parameter, which can be of two types:
- 0x00 - blocks the flow until the end of the team. In this case, the function will return 0 after completion.
- 0x01 - will check the status and return the result without blocking the stream. In this case, the function will return 0 or 1.
In order to indicate what type of reading you are using, there is the sceCdRMode () function, which accepts the following parameters:
- u_char - (from 0 to 255) the number of read retries until a read error was reported.
- u_char - disk rotation speed. One of the options:
- SCECdSpinStm - recommended speed;
- SCECdSpinNom - start reading the disk at maximum speed and drop to the one on which you can normally read.
- SCECdSpinDvdDL0 - read mode of two-layer disks.
- u_char - sector size. There is only one type of parameter: SCECdSecS2048, i.e. size 2048 bits.
- u_char - filled data for alignment.
The alignment data is used to ensure that the function always occupies the same memory size.
There is also a function through which you can find out about the type of error that occurred -
sceCdGetError ().
Returns the error type or SCECdErNO if there were no errors.
Using Callback
Instead of waiting, you can use callback functions. They are assigned using the sceCdCallback () function. It takes a parameter to a pointer to the address of the function. Keep in mind that a function is called regardless of which asynchronous function has ended. However, the reason code for the function call is passed in the first parameter.
You cannot assign a function while executing a callback function called (sorry for the tautology).
If you pass 0 instead of the parameter, then the callback will be removed.
The parameter passed to the function from the callback can take the following values:
- SCECdFuncRead - says that the sceCdRead () function has finished
- SCECdFuncSeek - the sceCdSeek () function has finished work;
- SCECdFuncStandby - the function sceCdStandby () has finished work;
- SCECdFuncStop - sceCdStop () function has finished work;
- SCECdFuncPause - the sceCdPause () function has finished working.
You cannot call a function like sceCdRead ();
The function returns 0 or the address to the previous callback.
Stream reading
For streaming reading, IOP memory is required. To use stream reading, you need to connect the cdvdstm.irx module through the sceSifLoadModule () function.
The stream is loaded into the IOP and data can be read from there.
The following functions are used for streaming reading.
sceCdStInit () - initialize the stream and set the buffer.
Parameters:
- stream capacity (determined using the number of sectors by 2048 bytes).
- the number of parts of the stream (according to the specification - rings). A buffer with 3 or more parts must have a capacity of more than 16 sectors.
- the address of the stream in memory.
To obtain a location in the IOP, you must use the sceSifAllocIopHeap () function from EE Kernel.
The function returns 0 or 1, which is False or True.
sceCdStStart () - start streaming filling.
Parameters:
- position to start reading from disk. Used by LSN.
- type of reading received through sceCdRMode ().
Returns the same as the previous one.
sceCdStRead () - read data from a stream.
Parameters:
- the number of sectors to read from the buffer.
- address for reading data (must be aligned to 64 bytes).
- reading type: STMNBLK - will return the information that is in the buffer; STMBLK - will return data whether there is or an error.
- variable for error number.
Returns the number of sectors read.
Error numbers can be as follows:
- 0x00 = SCECdErNO - no errors.
- 0x01 = SCECdErABRT - termination command returned.
- 0x13 = SCECdErNORDY - block not read.
- 0x14 = SCECdErCUD - the read method is not suitable for the disk in the drive.
- 0x20 = SCECdErIPI - abnormal position of the sector.
- 0x21 = SCECdErRILI - abnormal number of sectors to read.
- 0x30 = SCECdErREAD - problems while reading from disk.
- 0x31 = SCECdErTRMOPN - the cover opened while reading.
sceCdStPause () - pause streaming filling.
There are no parameters. Returns the success of the operation in the form of 0 or 1.
sceCdStResume () - return stream filling.
There are no parameters. Returns the success of the operation.
sceCdStSeek () - change of position for reading a stream.
The parameter accepts LSN. Returns the success of the operation.
sceCdStStat () - get the status of reading the stream to the buffer.
There are no parameters. Returns 0 in case of error or the number of sectors read.
sceCdStStop () - stop filling the status with a stream
. No parameters. Returns the success of the operation.
After the call to sceCdStInit () and initialization of the call to sceCdStStart (), the data will be read in the background, starting from the specified sector, and save it in the buffer. After sceCdStRead () is executed, the data in the buffer can be read sequentially.
Please note that the following libcdvd functions cannot be used to access the disk during streaming (I will explain them later):
- sceCdChangeThreadPriority ()
- sceCdGetToc ()
- sceCdInit ()
- sceCdMmode ()
- sceCdPause ()
- sceCdRead ()
- sceCdReadChain ()
- sceCdReadIOPm ()
- sceCdSearchFile ()
- sceCdSeek ()
- sceCdStandby ()
- sceCdStop ()
- sceCdSync ()
- sceCdDiskReady ()
- sceCdGetError ()
- sceCdGetReadPos ()
Need to know
The bottom line is that the drive is connected to the IOP and somehow you have to use the IOP functions.
The following data differs between IOP and EE:
- the command to complete the reading of data from the EE means the completion of the receipt of data in the EE processor, and not the completion of reading data from disk;
- Errors on the EE side affect subsequent actions on the IOP side;
- when transferring data to EE, memory alignment up to 64 bytes will always occur.
- the priority of the stream in the EE that will work with the disk will correspond to the priority in the IOP, so be careful, otherwise you may lose the normal processing of other IOP flows.
Towards the end of the first part
The topic is very complicated. In the second part, I will explain the rest of the article and how to install the PS2 SDK on Windows and Linux.
PS : I apologize for any typos.