
Simple Twitter bot in Python
In this article, I would like to share the experience of writing a small twitter bot in Python.
I was prompted to write a bot by the famous “pichalka bot” on Twitter, which automatically sends replays to everyone who mentions the word “pichalka” in their tweet. Since at that moment I was actively studying Python, it was decided to write on it.
As a library for working with the Twitter API, I took tweepy . This is a fairly simple and convenient library; In addition, her repository on Google Code has several code samples and good documentation . It is also in the repositories of Ubuntu and Debian, so it can be easily installed by the team
1. For the bot to work, you must register a new application on Twitter. Follow the link and fill in all the fields.
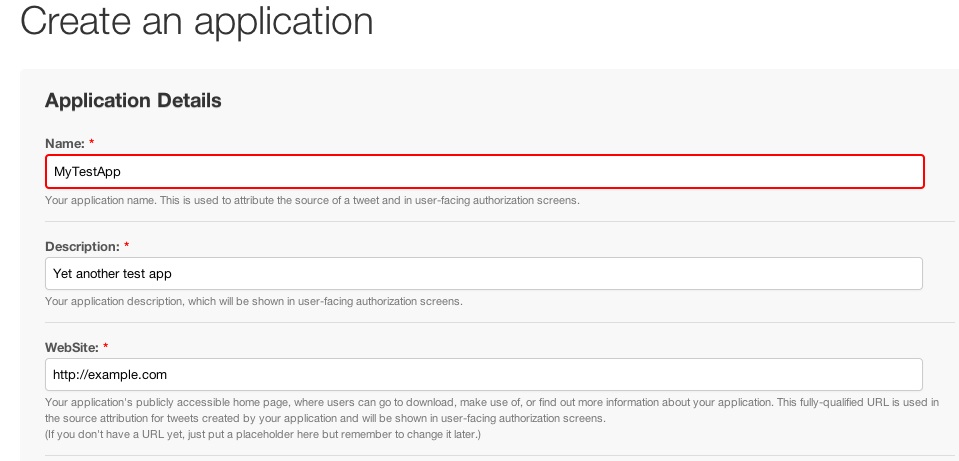
After that, you will receive 2 keys for OAuth authorization.
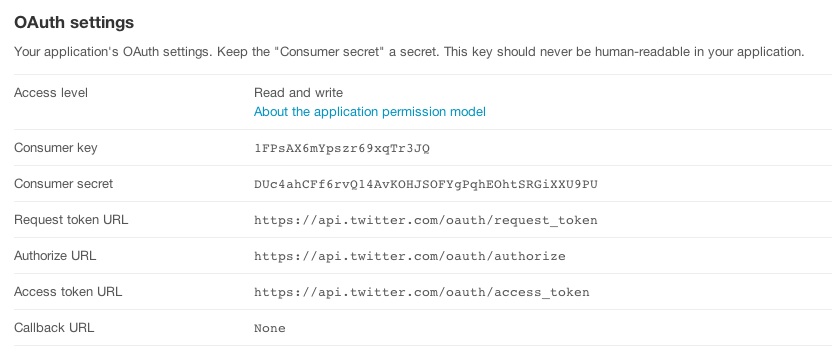
It is also necessary to change application permissions. Settings tab, Access -> Read and Write
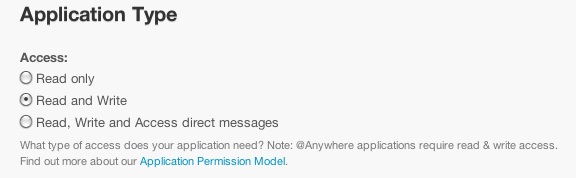
2. Now you need to get 2 more keys, individual for each user. To do this, you can click the "Create access token" button on the application page, or use the small sample code from the tweepy documentation.
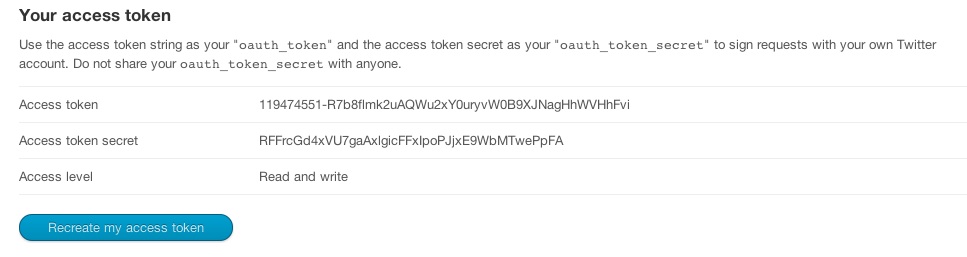
Option with sample code:
Save it to a file, paste the keys obtained when registering the application, and run it. Go to the proposed address, and Twitter will give you a pin code that you will need to enter into the console. Upon successful authorization, you will receive the same two user keys.

3. Now you can go to the code of the bot itself:
For correct operation, you need to insert the received keys into variables in the code: the keys from the application page are consumer_key and consumer_secret, user keys are access_key and access_secret. You also need to adjust the keywords to search for tweets and the answers to them in the variable search_reply_words.
That's all for today.
Thanks for attention! Hope it was interesting and helpful.
Introduction
I was prompted to write a bot by the famous “pichalka bot” on Twitter, which automatically sends replays to everyone who mentions the word “pichalka” in their tweet. Since at that moment I was actively studying Python, it was decided to write on it.
Training
As a library for working with the Twitter API, I took tweepy . This is a fairly simple and convenient library; In addition, her repository on Google Code has several code samples and good documentation . It is also in the repositories of Ubuntu and Debian, so it can be easily installed by the team
sudo apt-get install python-tweepy
.Development
1. For the bot to work, you must register a new application on Twitter. Follow the link and fill in all the fields.
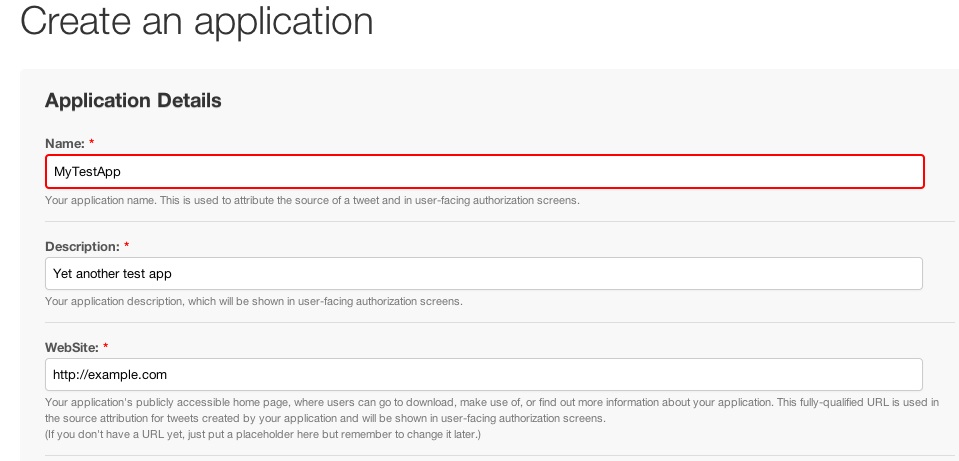
After that, you will receive 2 keys for OAuth authorization.
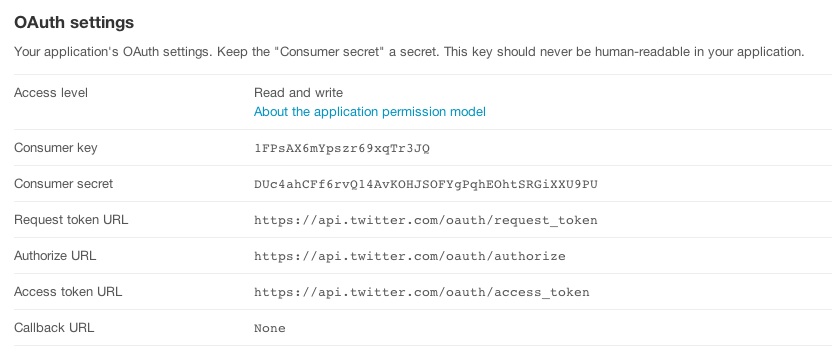
It is also necessary to change application permissions. Settings tab, Access -> Read and Write
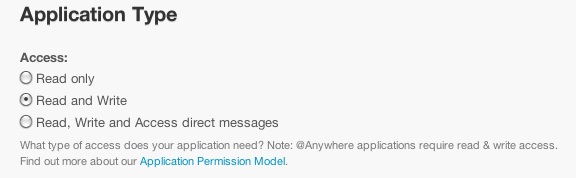
2. Now you need to get 2 more keys, individual for each user. To do this, you can click the "Create access token" button on the application page, or use the small sample code from the tweepy documentation.
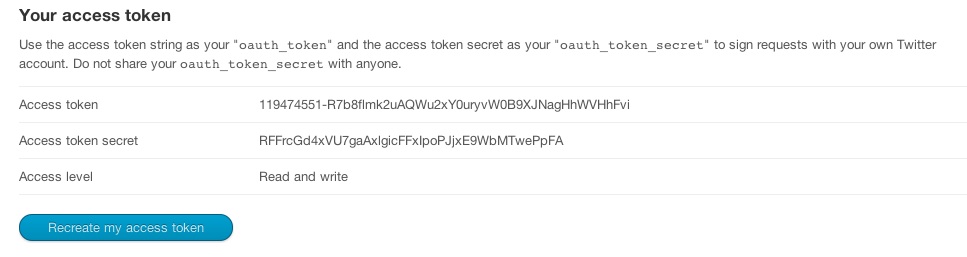
Option with sample code:
import tweepy, webbrowser
CONSUMER_KEY = 'paste your Consumer Key here'
CONSUMER_SECRET = 'paste your Consumer Secret here'
auth = tweepy.OAuthHandler(CONSUMER_KEY, CONSUMER_SECRET)
auth_url = auth.get_authorization_url()
webbrowser.open(auth_url)
verifier = raw_input('PIN: ').strip()
auth.get_access_token(verifier)
print "ACCESS_KEY = '%s'" % auth.access_token.key
print "ACCESS_SECRET = '%s'" % auth.access_token.secret
Save it to a file, paste the keys obtained when registering the application, and run it. Go to the proposed address, and Twitter will give you a pin code that you will need to enter into the console. Upon successful authorization, you will receive the same two user keys.

3. Now you can go to the code of the bot itself:
#coding: utf-8
import oauth, tweepy, sys, locale, threading
from time import localtime, strftime, sleep
replyed=['']
search_reply_words={'печалька':' каждый раз, когда вы говорите "печалька", умирают хомячки.','пичалька':' каждый раз, когда вы говорите "пичалька", умирают хомячки.'}
update_time=60 #время обновления
def Tweet(twit,id_reply):
if len(twit)<=140 and len(twit)>0:
api.update_status(twit,id_reply) #обновляем статус (постим твит)
return True
else:
return False
def init(): #инициализируемся
global api
#consumer_key = ""
#consumer_secret = ""
#access_key=""
#access_secret=""
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_key, access_secret)
api=tweepy.API(auth)
class TwiBot(threading.Thread):
def __init__ (self, keyword,answer):
self.keyword = keyword
self.answer=answer
threading.Thread.__init__(self)
def run (self):
global replyed,api
request=api.search(self.keyword) #ищем твиты с указанным словом
for i in request:
if i.from_user!='thevar1able' and i.id not in replyed: # если твит не наш и мы на него еще не отвечали...
try:
Tweet('@'+i.from_user+self.answer,i.id) #...отвечаем
print strftime('[%d/%m %H:%M:%S]',localtime())+' Reply to @'+i.from_user+'('+str(i.from_user_id)+')'
except:
print strftime('DUP [%d/%m %H:%M:%S]',localtime())+' Reply to @'+i.from_user+'('+str(i.from_user_id)+')'
replyed.append(i.id)
return True
init() # инициализируемся
while not False: # вечно
for word in search_reply_words:
TwiBot(word, search_reply_words[word]).start() #запускаем поток с нужным словом для поиска
print strftime('[%d/%m %H:%M:%S]',localtime())+' Updating for word "'+str(word)+'"...'
sleep(1)
sleep(update_time)
For correct operation, you need to insert the received keys into variables in the code: the keys from the application page are consumer_key and consumer_secret, user keys are access_key and access_secret. You also need to adjust the keywords to search for tweets and the answers to them in the variable search_reply_words.
That's all for today.
Thanks for attention! Hope it was interesting and helpful.