
Create an application for Windows Phone 7 from start to finish. Part 6. Support for landscape orientation, the use of controls
- Transfer
Previous part
In this part you will learn:
Portrait orientation is standard on Windows Phone applications. In order to implement support for landscape orientation, you have to write additional code. To indicate that the application supports both portrait and landscape orientation, you should set the SupportedOrientations property to PortraitOrLandscape in either XAML or code. Then you must make sure that the contents of the pages of your application are displayed correctly in landscape orientation.
Interface design recommendation:
If the application supports text input, you should support landscape orientation because of the possible existence of a hardware keyboard.
There are various ways to ensure that content is displayed correctly in both portrait and landscape orientations. The two main methods are scrolling and grid layout. These methods can be used alone or in combination with each other.
Scrolling uses the StackPanel control, which is located in the ScrollViewer control. Use this method if the content is displayed as a list or if various controls follow one after another on the page. The StackPanel allows you to set the order of the children one after another, and the ScrollViewer control allows you to scroll the contents of the StackPanel if the user interface elements do not fit on the screen.
Scroll portrait and landscape support
Support for portrait and landscape orientation using the grid

The controls in the application are used to display data and allow the user to interact with the application.
You can use a large number of controls by simply dragging them from the Toolbox onto the surface of the designer.

The following table will provide you with some guidance on which control you should use depending on your application requirements. The table shows the Silverlight controls (such as Button and TextBox), as well as controls specifically designed for Windows Phone (such as Panorama and Pivot). There are other controls available in the Silverlight for Windows Phone Toolkit .
* It is not stylized under the Metro design, but can be used if it is properly designed.
Performance Improvement Tip:
If you use the ProgressBar in indeterminate mode, this can affect application performance. If you need indeterminate progress bar, consider creating your own control.
Performance Improvement Tip:
When using resource-intensive controls (such as Bing Maps or video) and if your application runs out of memory, you can call the DeviceExtendedProperties.GetValue method to monitor memory usage.
The following image shows an example of how to use some of the controls on the Fuel Tracker summary page. Please note that this page uses the Pivot control to expand usable space.

Windows Phone has two special controls that effectively expand the layout area. The Panorama and Pivot controls provide the ability to horizontally navigate the contents of the page, which allows the user to quickly flip through and scale the content as necessary. The Panorama control creates a panoramic view of the elements. The Pivot control provides an easy way to manage views of large datasets in an application. The Pivot control can be used as a navigation interface to filter large sets or to switch between views.
On the summary page in the Fuel Tracker application, the Pivot control element is used, which allows the user to quickly switch between the information about the last refueling and the summary information about all refueling. Initially, this information was located on one page. But such markup created the feeling of a very cluttered page, so two different views were put in a Pivot control.
Design recommendations for Panorama and Pivot controls:
When adding controls for entering text into the application, you must leave space for the on-screen keyboard, which is also called the soft input panel (SIP). You must make sure that the keyboard does not hide buttons or other navigation controls. One way to prevent this behavior is to place the navigation items in the application bar.
To prevent user input errors, you should use the most appropriate on-screen keyboard by setting the InputScope property for the control. Two examples of InputScope values are shown in the following table.
Providing the correct keyboard layout will limit the possibility of a user making a mistake, but you must also implement user input validation.
Interface design recommendation:
If the application supports text input, you should support landscape orientation because of the possible existence of a hardware keyboard.
A gesture event occurs when the user interacts with the screen, for example, by tapping or swiping a finger over it. Gesture processing is automatically included in all user interface elements and transforms all gestures into standard events. For example, a Button control contains a Click event that occurs when a button is pressed. You create a method called an event handler to handle the event. You can create an event handler in the Properties window or in XAML code.
You can create an event handler using the Events tab of the Properties window. The Properties window lists all the events available for the selected control. The following image shows some of the events for Button.
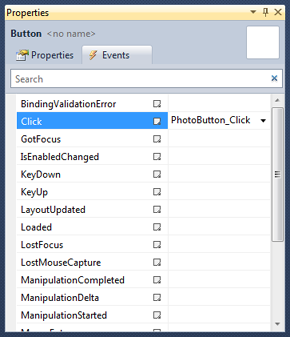
To create an event handler with a default name, you can simply double-click the event name in the Properties window. An event handler is created and a code-behind file is opened in the code editor. The following code snippet shows the Click event handler for the Button control.
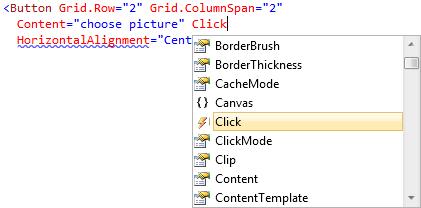
Once you have defined the event, you can specify the name of the event handler or double-click in the IntelliSense window that appears. The following image shows the IntelliSense window that appears to help you create a new event handler.

The following piece of XAML code attaches the Click event of a Button control to a PhotoButton_Click event handler .
In this part you will learn:
- How to implement portrait and landscape support.
- What controls are available and how to choose the right one.
- How to choose between Panorama and Pivot controls.
- How to use controls to enter text.
- How to handle control events.
Support portrait and landscape orientation
Portrait orientation is standard on Windows Phone applications. In order to implement support for landscape orientation, you have to write additional code. To indicate that the application supports both portrait and landscape orientation, you should set the SupportedOrientations property to PortraitOrLandscape in either XAML or code. Then you must make sure that the contents of the pages of your application are displayed correctly in landscape orientation.
Interface design recommendation:
If the application supports text input, you should support landscape orientation because of the possible existence of a hardware keyboard.
There are various ways to ensure that content is displayed correctly in both portrait and landscape orientations. The two main methods are scrolling and grid layout. These methods can be used alone or in combination with each other.
Scrolling uses the StackPanel control, which is located in the ScrollViewer control. Use this method if the content is displayed as a list or if various controls follow one after another on the page. The StackPanel allows you to set the order of the children one after another, and the ScrollViewer control allows you to scroll the contents of the StackPanel if the user interface elements do not fit on the screen.
Scroll portrait and landscape support
- Change the property of the SupportedOrientations page to PortraitOrLandscape.
- Replace the standard Grid with ScrollViewer and StackPanel.
Support for portrait and landscape orientation using the grid
- Change the property of the SupportedOrientations page to PortraitOrLandscape.
- Use the Grid as a panel for content.
- If necessary, create an OrientationChanged event handler and add code to move the elements to the Grid.

The controls in the application are used to display data and allow the user to interact with the application.
Selection of controls
You can use a large number of controls by simply dragging them from the Toolbox onto the surface of the designer.

The following table will provide you with some guidance on which control you should use depending on your application requirements. The table shows the Silverlight controls (such as Button and TextBox), as well as controls specifically designed for Windows Phone (such as Panorama and Pivot). There are other controls available in the Silverlight for Windows Phone Toolkit .
Application requirement | Control |
---|---|
Navigation | PhoneApplicationFrame PhoneApplicationPage ApplicationBarIconButton ApplicationBarMenuItem HyperlinkButton |
Markup | Border Canvas ContentControl Grid Panorama Pivot StackPanel ScrollViewer VirtualizingStackPanel |
Text display | Textblock |
Text input | TextBox PasswordBox |
Display a list of items | Listbox |
Buttons | Button HyperlinkButton |
Control to select from a list | CheckBox RadioButton Slider ComboBox * |
Image display | Image |
Work with cards | Bing maps |
Video display | Mediaelement |
HTML rendering | Webbrowser |
Progress | Progressbar |
Pop-up messages | Popup |
Performance Improvement Tip:
If you use the ProgressBar in indeterminate mode, this can affect application performance. If you need indeterminate progress bar, consider creating your own control.
Performance Improvement Tip:
When using resource-intensive controls (such as Bing Maps or video) and if your application runs out of memory, you can call the DeviceExtendedProperties.GetValue method to monitor memory usage.
The following image shows an example of how to use some of the controls on the Fuel Tracker summary page. Please note that this page uses the Pivot control to expand usable space.

Choose between Panorama and Pivot controls
Windows Phone has two special controls that effectively expand the layout area. The Panorama and Pivot controls provide the ability to horizontally navigate the contents of the page, which allows the user to quickly flip through and scale the content as necessary. The Panorama control creates a panoramic view of the elements. The Pivot control provides an easy way to manage views of large datasets in an application. The Pivot control can be used as a navigation interface to filter large sets or to switch between views.
On the summary page in the Fuel Tracker application, the Pivot control element is used, which allows the user to quickly switch between the information about the last refueling and the summary information about all refueling. Initially, this information was located on one page. But such markup created the feeling of a very cluttered page, so two different views were put in a Pivot control.
Design recommendations for Panorama and Pivot controls:
- Use Panorama elements as a starting point for more details.
- Use the Pivot control to filter large amounts of data, providing the ability to view multiple data sets or a way to switch between different display modes of the same data.
- Do not use the Pivot control for navigation tasks such as in the wizard.
- Vertical scrolling of a list or grid in Panorama sections is acceptable as long as it is within the boundaries of the section and is not parallel to horizontal scrolling.
- Never place a Pivot control inside another Pivot control.
- Never place a Pivot control inside a Panorama control.
- You should minimize the number of pages containing Pivot in your application.
- The Pivot control should only be used to display items or data of this type.
- You should not use application panel buttons to navigate in a Pivot control. If Pivot requires navigation aids, you are probably using it for other purposes.
Using controls to enter text
When adding controls for entering text into the application, you must leave space for the on-screen keyboard, which is also called the soft input panel (SIP). You must make sure that the keyboard does not hide buttons or other navigation controls. One way to prevent this behavior is to place the navigation items in the application bar.
To prevent user input errors, you should use the most appropriate on-screen keyboard by setting the InputScope property for the control. Two examples of InputScope values are shown in the following table.
Keyboard | Layout |
---|---|
TelephoneNumber | Layout with 12 numeric keys![]() |
Text | Standard layout with features such as auto-correction and text suggestion![]() |
Interface design recommendation:
If the application supports text input, you should support landscape orientation because of the possible existence of a hardware keyboard.
Handling Event Controls
A gesture event occurs when the user interacts with the screen, for example, by tapping or swiping a finger over it. Gesture processing is automatically included in all user interface elements and transforms all gestures into standard events. For example, a Button control contains a Click event that occurs when a button is pressed. You create a method called an event handler to handle the event. You can create an event handler in the Properties window or in XAML code.
You can create an event handler using the Events tab of the Properties window. The Properties window lists all the events available for the selected control. The following image shows some of the events for Button.
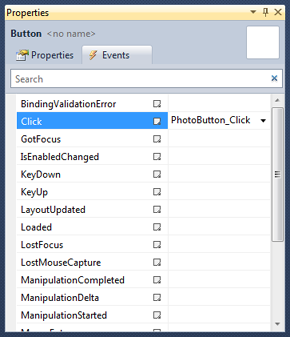
To create an event handler with a default name, you can simply double-click the event name in the Properties window. An event handler is created and a code-behind file is opened in the code editor. The following code snippet shows the Click event handler for the Button control.
- private void PhotoButton_Click(object sender, RoutedEventArgs e)
- {
-
- }
* This source code was highlighted with Source Code Highlighter.
You can also create an event handler in XAML. In XAML editing mode, enter the name of the event that you want to process. When you start typing in XAML editing mode, the IntelliSense window appears. The following image shows how to define a Click event in XAML code. 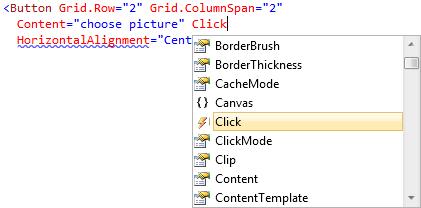
Once you have defined the event, you can specify the name of the event handler or double-click

The following piece of XAML code attaches the Click event of a Button control to a PhotoButton_Click event handler .
* This source code was highlighted with Source Code Highlighter.
Next part