
Small testing of two libraries for working with ZIP archives (C # language)
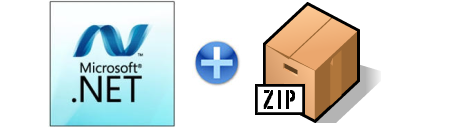
More than once I had to work with zip archives using C #, in my cases it was downloading the archive with the database, then I extracted the database to disk, if it was not a text file, then I dynamically connected the database, otherwise I just read the data I needed. For these purposes I used ZipStorer , I like it because it just does the work assigned to it, it weighs little and is a class that, with a minimal amount of gestures, appears in the executable file. Today I decided to try something else, for which I chose two SharpZipLib and DotNetZip libraries , after which I did a little testing to understand which one might be useful to me in the future.
Since I already mentioned ZipStorer, I’ll show an example of working with it, just add the ZipStorer.cs class and write such a method, after which everything will work:
private void Unzip(string fileInput, string saveFile)
{
// Open an existing zip file for reading
using (ZipStorer zip = ZipStorer.Open(fileInput, FileAccess.Read))
{
// Read the central directory collection
List dir = zip.ReadCentralDir();
// Look for the desired file
string DBfile = string.Empty;
foreach (ZipStorer.ZipFileEntry entry in dir)
{
if (Path.GetFileName(entry.FilenameInZip).ToLower().IndexOf(".txt") != -1)
{
DBfile = Path.GetFileName(entry.FilenameInZip);
zip.ExtractFile(entry, saveFile + DBfile);
//Now we can connect to database or read file
}
}
}
}
* This source code was highlighted with Source Code Highlighter.
Now we find out how easy it will be to do such things for SharpZipLib and DotNetZip. Examples of working with SharpZipLib can be found at this link or the official source , for DotNetZip examples of work will be immediately in the archive with the library .
Licenses:
SharpZipLib - GPL
DotNetZip - Ms-PL
This time, I decided not to translate information from the developers' sites, I can only say that both libraries have support for the Compact Framework and can be used in web applications. The size of both libraries is about 200 kilobytes, though for DotNetZip I take the "Reduced" version, the full version takes about 400 kilobytes, which is twice as much. Now we pass directly to the test, unlike ZipStorer, we will archive the data, and we will compare the compression ratio and speed. We will archive with the default level, for DotNetZip the CompressionLevel property is set to “Default” or “Level6”, and the GetLevel () method for SharpZipLib returns “6”.
Libraries have classes with the same name, so I connected the spaces this way:
using test1 = Ionic.Zip;
using test2 = ICSharpCode.SharpZipLib.Zip;
To archive using DotNetZip, the following code is sufficient:
string ZipFileToCreate = Application.StartupPath + @"\test1.zip";
string FileToZip = textBoxFile.Text;
using (test1.ZipFile zip = new test1.ZipFile())
{
test1.ZipEntry ze = zip.AddFile(FileToZip);
zip.Save(ZipFileToCreate);
}
* This source code was highlighted with Source Code Highlighter.
For SharpZipLib you need to write a little more:
string file = textBoxFile.Text;
using (test2.ZipOutputStream s = new test2.ZipOutputStream(File.Create(Application.StartupPath + @"\test2.zip")))
{
byte[] buffer = new byte[4096];
test2.ZipEntry entry = new test2.ZipEntry(Path.GetFileName(file));
s.PutNextEntry(entry);
using (FileStream fs = File.OpenRead(file))
{
int sourceBytes;
do
{
sourceBytes = fs.Read(buffer, 0, buffer.Length);
s.Write(buffer, 0, sourceBytes);
} while (sourceBytes > 0);
}
s.Finish();
s.Close();
}
* This source code was highlighted with Source Code Highlighter.
I took both pieces of code from official examples and deleted comments to save space. You can see the results of the work in this table: The
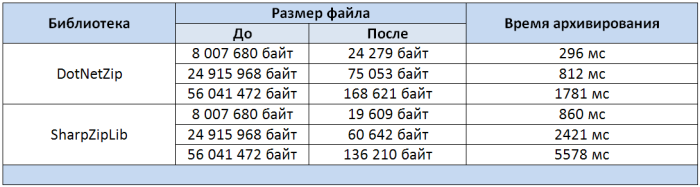
files for the test were generated artificially, so such a beautiful compression result, to see how the situation will change with real data, I will download the list of domains from the registrar's site, the archive that I downloaded will be the standard, its size It is equal to 28 043 005 bytes, I will extract the data from the archive and check two libraries again:

Conclusion
Based on the results, the speed characteristics are better for DotNetZip, but at the same time the data compression in this library is worse, let me remind you that I did not change the compression ratio and it was equal to 6 for both libraries. Personally, I liked DotNetZip visually more, I’ll dwell on it , the number of downloaded this library more than 100 thousand suggests that my choice is not alone.