
Arms race

In May, Google I / O 2019 announced a new framework for developing a declarative Android UI called Jetpack Compose. A month later, at WWDC 2019, a declarative UI framework for iOS called SwiftUI was announced. After these conferences, it became clear what mobile development was aiming for, and I wanted to figure out what can be done with these frameworks at the moment and what is the difference between them.
Cursory review
After a quick review, it became clear about the availability of cool documentation in iOS
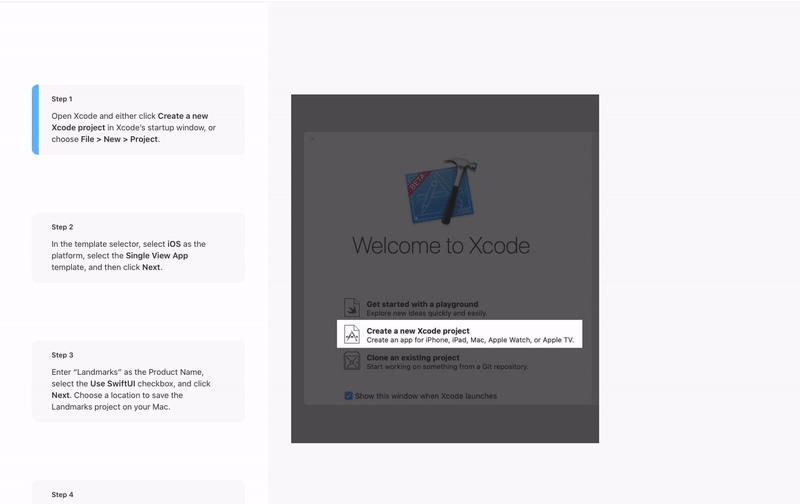
and the complete absence of documentation in android. To familiarize yourself with the framework on the official page, it is advised to see the demo application.

Hello world
Getting started with SwiftUI is very simple, just upgrade macOS to beta, install Xcode 11 Beta, and create a new project, while selecting the “Use SwiftUI” checkbox.

In the case of Jetpack Compose, everything is more complicated. We need to download the latest build from Jetpack. And based on the ui-demos module, by typing, figure out how to work with the framework and what dependencies are needed. A very sad first impression of Compose compared to SwiftUI.

Compose example
@Composable
fun HelloWorld() {
Text(text = "Hello world")
}
SwiftUI example
struct ContentView: View {
var body: some View {
Text("Hello SwiftUI!")
}
}
The layout of the UI looks very similar, which is logical, since it tends to a declarative look.
Visual editor
With SwiftUi comes a visual editor (canvas),
which:
- redraws the screen instantly after changing the code;
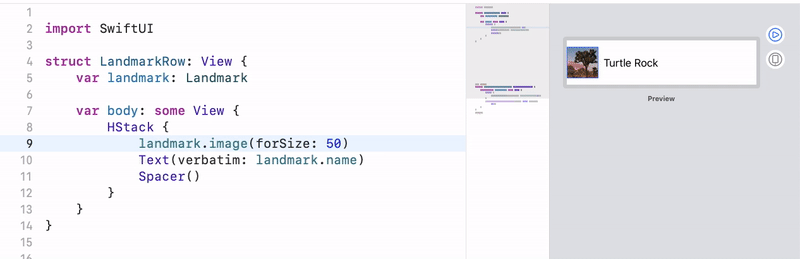
- Able to show several devices at once;

- makes it possible to change the attributes ui of elements from the editor;

- and the most interesting and cool - you can run the ui code from the editor and see how the screen works.

In the case of Jetpack Compose, there is no visual editor.
Work with lists
To work in SwiftUI just create a view of the string
struct ListRow: View {
let number:Int
var body: some View {
Text.init(verbatim: "Text \(number)")
}
}
and paste into the list.
List(Array(0...44)) { number in
ListRow.init(number: number)
}
The list is ready.

Compose does not yet have a list widget. At first glance, you can use Column.
Column {
listOf(
"Артем",
"Святослав",
"Женя",
"Никита",
"Алина",
"Леша",
"Вадим",
"Паша",
"Ваня"
)
.forEach { name ->
Text(text = name, style = +themeTextStyle { h2 })
}
}
But he is not scrolling.

What is most sad, even the example from Google is not finalized and will not be scrolled.
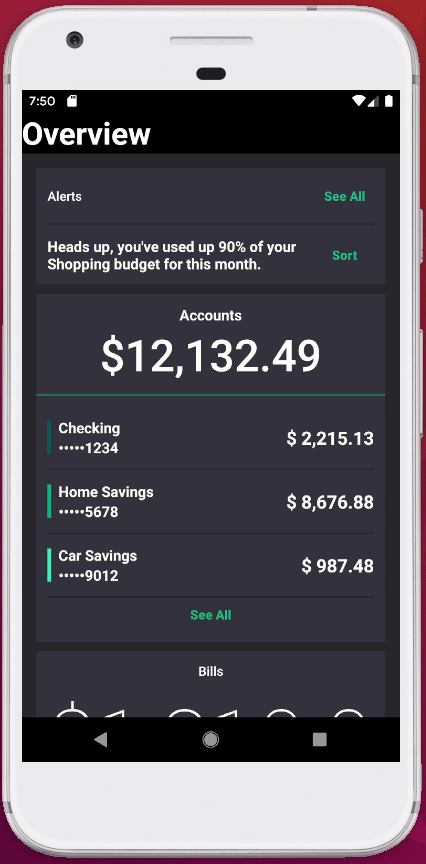
View hierarchy
In SwiftUI, the root element to replace the UIViewController is the new View component with a new life cycle. This suggests that Apple, having analyzed the pros and cons of the UIViewController implementation, tried to do everything in a new way, implementing the best features.
struct ContentView : View {
var body: some View {
Text("ContentView2 ")
.onDisappear(){
print("onDisappear")
}
.onAppear{
print("onAppear")
}
}
}
In the case of Compose, the root elements remain the good old Activity or Fragment, instead of the usual XML loading.
class MainActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Downloading widgets from Compose.
class ComposeActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
CraneWrapper {
MaterialTheme {
Text(text = "Hello world")
}
}
}
}
}
This leads to the fact that Android does not get rid of the legacy root view. It’s just that the old UI components no longer communicate with XML, but with Compose widgets.
Navigation
To navigate in SwiftUI, you must specify which view should be opened by clicking on the button.
NavigationButton(destination: LandmarkDetail()) {
LandmarkRow(landmark: landmark)
}
There is no navigation implementation inside Compose. But probably it should not be, since the root element is Activity or Fragment, in which navigation has
Supported version
SwiftUI works with iOS 13, coming out in September, which will no longer be supported on the iPhone 5S, iPhone6, iPhone 6 Plus. Developers should think about how they will be ready to abandon these models.
Compose plans to be supported on any version of Android.
Conclusions
After the conferences, I thought that there were two new powerful frameworks for ui. As a result, it turned out that Apple made a cool decision, from which many have an “exaltation state”. And Google announced a framework that is so crude that it is not clear how many points will work even at the concept level.