Creating a multi-module Gradle SpringBoot + Angular project in IDEA
Quite often in my practice, a bunch of Spring + Angular is used. I want to share my experience in preparing such a project in the IntelliJ IDEA development environment.
Create an empty Gradle project
First, create an empty Gradle project in IDEA, while uncheck all the libraries and frameworks.

Let our artifact be “demo” and the group “com.habr”. After creating the project, the settings.gradle and build.gradle files with the following contents will be automatically generated:
build.gradle
group 'com.habr'
version '1.0'
settings.gradle
rootProject.name = 'demo'
Create a module for Spring
Next, we will add a module to our project that will host the server part of our application, i.e. Spring
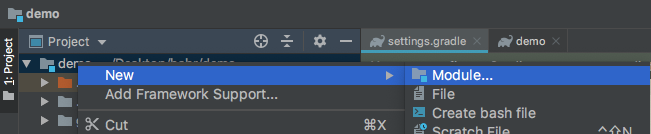
When creating this module, we will use the Spring Initializr.
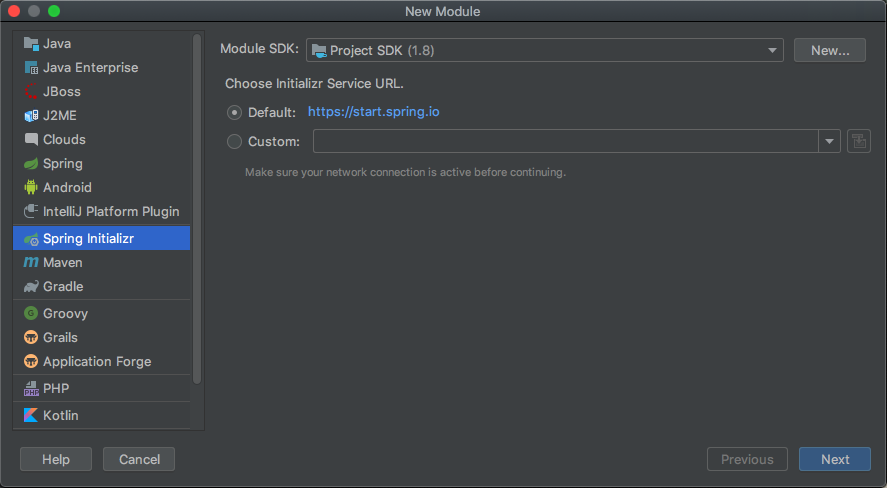
Let's name the module “demo-server”, indicate the type of the Gradle project, the group com.habr, and the name of the main package org.habr.demo.

Next, you need to select all the necessary components of the Spring framework, in this example I selected only “web”, but you can select everything that you need.

After creating the demo-server module, note that 2 files were created in it: build.gradle and settings.gradle. From the settings.gradle of this module it is necessary to transfer the following lines to the beginning of the settings.gradle file of the project itself, and delete the settings.gradle of the module.
pluginManagement {
repositories {
gradlePluginPortal()
}
}
In addition, you need to add the created module to the settings.gradle of the project, as a result we get:
settings.gradle
pluginManagement {
repositories {
gradlePluginPortal()
}
}
rootProject.name = 'demo'
include 'demo-server'
After that, we delete the following lines from the build.gradle file of the demo-server module:
group = 'com.habr'
version = '0.0.1'
On this, the creation of the first module can be considered completed, but we will return to it in order to associate it with the second.
Create a module for Angular
We create the demo-ui module as a java gradle project.
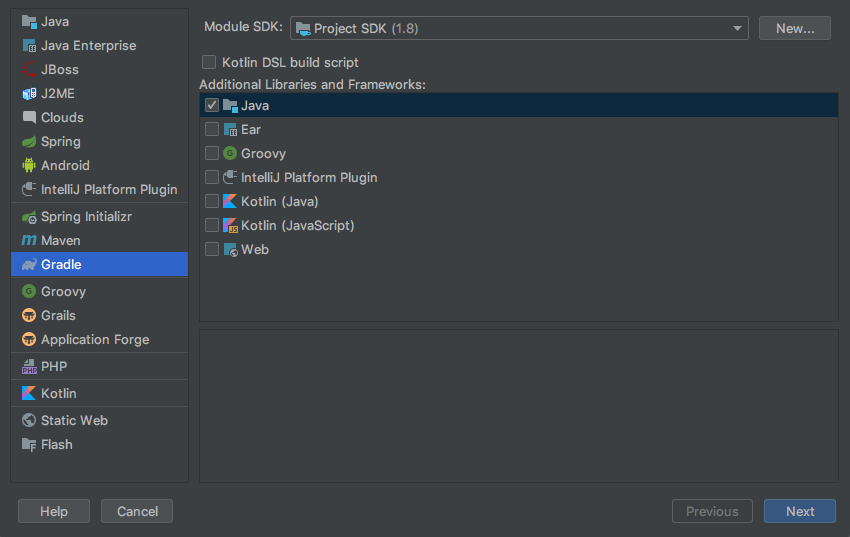
Let's name the created module "demo-ui".

In the created project, you need to delete the src folder. After performing this operation, open the terminal in the root folder of this module.

In the terminal that opens, run the command:
ng new demo-ui
The command will install Angular, and a folder with the same name will be created inside the demo-ui module.

All the contents of the created folder, except for node-modules, must be moved to the root of the module, and the folder itself must be deleted.

Now configure the assembly, for this we bring the build.gradle of the demo-ui module to the following form:
plugins {
id 'java'
id "com.moowork.node" version "1.3.1"
}
node {
version = '10.14.1'
npmVersion = '6.4.1'
download = true
}
jar.dependsOn 'npm_run_build'
jar {
//здесь "demo-ui" - имя Angular приложения, указанное в команде ng new
from 'dist/demo-ui' into 'static'
}
The module is ready.
We bind modules
It remains to simply add the dependency to the build.gradle of the demo-server module.
implementation project(':demo-ui')
As a result, we get the following build.gradle in the "demo-server" module:
plugins {
id 'org.springframework.boot' version '2.1.5.RELEASE'
id 'java'
}
apply plugin: 'io.spring.dependency-management'
sourceCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
implementation project(':demo-ui')
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Launch
To run the application, just run the Gradle task.
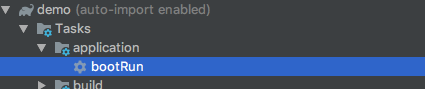
After which the application will be built and launched, it can be opened in a browser.

Well, if you want to get a jar file, then just run the build task.
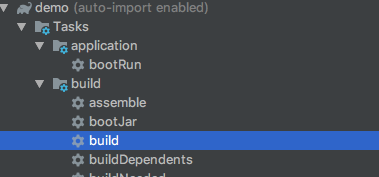
The assembled file will be waiting for you in demo-server / build / libs.
