
.NET zoo under the prism of NET Core
Hello! Today we look at the various chips and changes that have appeared in the .NET Core platform and compare them with the Framework. I broke the article into modules for a better understanding. This article will be interesting to those who are going to switch to .NET Core or are already using it.
We currently have three platforms: .NET Framework, Mono, and .NET Core. Each platform includes the following technologies:
NET Framework - WPF, WinForms, ASP.NET (DNX implementation), WCF
NET Core - UWP, ASP.NET Core, WA, Xamarin (.NET Standard), Avalonia and other
Mono - Xamarin ( PCL, Share project), Unity, ASP.NET, WinForms (cross platform)
NET Core (3.0) - Everything is the same as .NET Core above + WPF and WinForms, ML.NET
There is also a .NET Standard. This is a set of classes, methods and interfaces that allow you to write and use common code for all the platforms listed above. You can also write console applications on it. In short, this is the same PCL, but it works on all the platforms listed above.
I will not focus your attention on this, I simply list the OS support for NET Core projects:
• Windows
• Linux
• MacOS
Additionally supports launching under ARM processors on Linux and Windows.
As part of cross-compatibility, the application development platform includes a modular infrastructure. It is issued through NuGet, and you can access the batch functions, rather than one large assembly. As a developer, you can create lightweight applications containing only the necessary NuGet packages, which will make your program safer and more productive.
The modular infrastructure also enables faster updates to the .NET Core platform, as affected modules can be updated and released individually.
Now let's go deeper and see in more detail what we have under the hood in the projects. When creating a new project, each of you came across the file MyProject1.csproj (the name may differ). This file is responsible for compilation settings for this project, dependencies of other projects or libraries (libraries), and much more.
I have for you an example of how I decided to rewrite one project to .NET Standard. Let's take a look at how it was before (Framework):
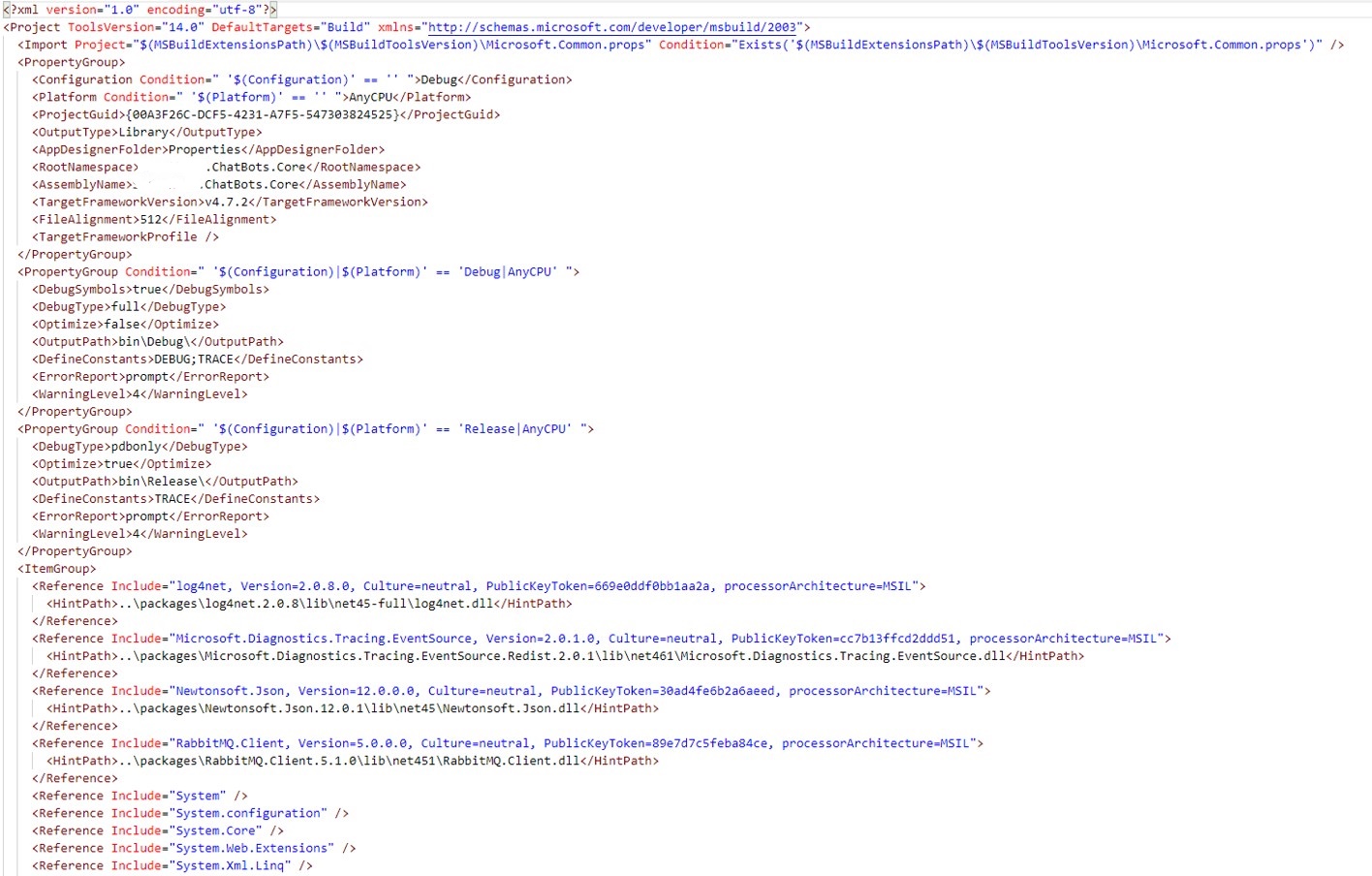
Unfortunately, this file does not fit on my PC completely (there are still references). And now let's see how it became after the transfer:

In NET Core and .NET Standard, csproj has been greatly simplified. Particularly “ballistic” ones may notice that some have changed. I removed the unnecessary and replaced with more convenient tools. After rewriting csproj, I noticed that working with NuGet packages began to take noticeably less time, and as you understand, editing the new version of csproj is much more convenient, because it is not cluttered with extra lines.
• Modified Random
• Modified HttpClient
• Optimized loops
• Optimized List, Array
• Optimized Stream, MemoryStream
• And much more
In this article I will not consider all the changes. This will be a separate article. But let's look at a small example on the List collection:
I ran it through benchmarkdotnet.org on both platforms. After the tests, I got the following results:
Core 2.2.4 x64 RyuJIT
Method : BenchmarkList
Mean : 370.1 ms
Error : 0.3761 ms
StdDev : 0.3518 ms
Framework 4.7.2 x64 RyuJIT
Method : BenchmarkList
Mean : 481.9 ms
Error : 1.210 ms
StdDev : 1.011 ms
As you can see, the speed of operation is significantly different (at times) in favor of Core.
Microsoft is trying not only to give developers convenient development tools, but also improves the basic things that lead to improvements and optimizations of your projects.
This is a feature that makes the runtime more adaptive to use the JIT compiler to improve startup performance and maximize throughput.
Compiles the project as quickly as possible.
Optimizes the most common methods.
This functionality makes your project build faster and gives you almost the same performance. We tested this functionality, and this is a smart feature for NET Core projects, which reduces compilation time. Multilevel compilation slightly slows down the operation of your application, I do not recommend including it on a production server, but for debugging there is more than an actual function that saves programmers time.
Microsoft is trying to improve the lives of developers of the .NET platform. All of the above “goodies” that have appeared in our company allow us to make the environment more open and expandable. I hope you appreciate it. Do not be afraid to switch to a new technology stack and use different features.
Thanks for attention. I hope you enjoyed it.
Stack technology
We currently have three platforms: .NET Framework, Mono, and .NET Core. Each platform includes the following technologies:
NET Framework - WPF, WinForms, ASP.NET (DNX implementation), WCF
NET Core - UWP, ASP.NET Core, WA, Xamarin (.NET Standard), Avalonia and other
Mono - Xamarin ( PCL, Share project), Unity, ASP.NET, WinForms (cross platform)
NET Core (3.0) - Everything is the same as .NET Core above + WPF and WinForms, ML.NET
NET Standard
There is also a .NET Standard. This is a set of classes, methods and interfaces that allow you to write and use common code for all the platforms listed above. You can also write console applications on it. In short, this is the same PCL, but it works on all the platforms listed above.
Cross platform
I will not focus your attention on this, I simply list the OS support for NET Core projects:
• Windows
• Linux
• MacOS
Additionally supports launching under ARM processors on Linux and Windows.
Dependency
As part of cross-compatibility, the application development platform includes a modular infrastructure. It is issued through NuGet, and you can access the batch functions, rather than one large assembly. As a developer, you can create lightweight applications containing only the necessary NuGet packages, which will make your program safer and more productive.
The modular infrastructure also enables faster updates to the .NET Core platform, as affected modules can be updated and released individually.
Csproj
Now let's go deeper and see in more detail what we have under the hood in the projects. When creating a new project, each of you came across the file MyProject1.csproj (the name may differ). This file is responsible for compilation settings for this project, dependencies of other projects or libraries (libraries), and much more.
I have for you an example of how I decided to rewrite one project to .NET Standard. Let's take a look at how it was before (Framework):
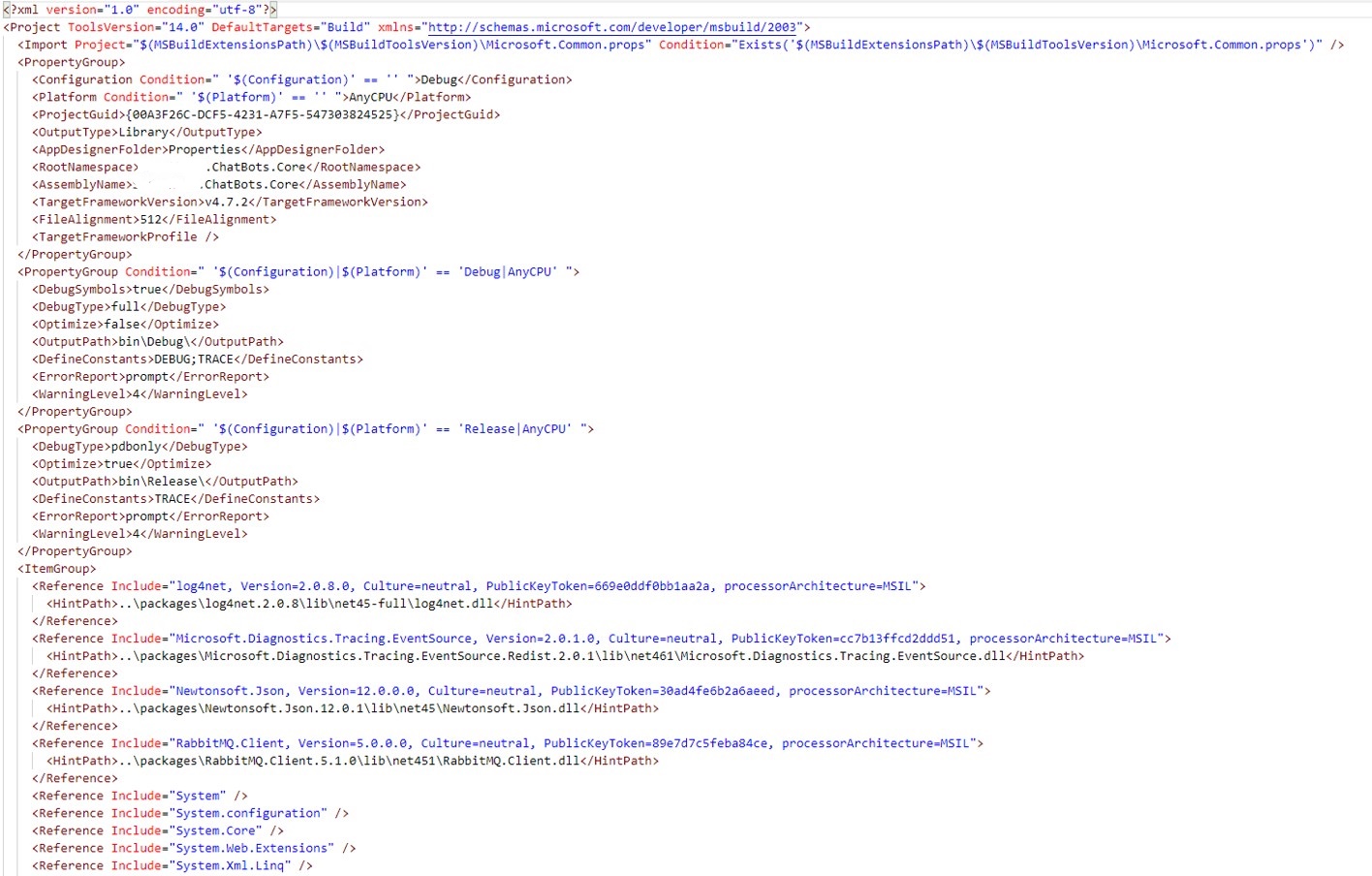
Unfortunately, this file does not fit on my PC completely (there are still references). And now let's see how it became after the transfer:

In NET Core and .NET Standard, csproj has been greatly simplified. Particularly “ballistic” ones may notice that some have changed. I removed the unnecessary and replaced with more convenient tools. After rewriting csproj, I noticed that working with NuGet packages began to take noticeably less time, and as you understand, editing the new version of csproj is much more convenient, because it is not cluttered with extra lines.
Performance & Improvements
• Modified Random
• Modified HttpClient
• Optimized loops
• Optimized List, Array
• Optimized Stream, MemoryStream
• And much more
In this article I will not consider all the changes. This will be a separate article. But let's look at a small example on the List collection:
var list = new List();
for (int i = 0; i < 100000000; i++)
{
list.Add(i);
list.RemoveAt(0);
}
I ran it through benchmarkdotnet.org on both platforms. After the tests, I got the following results:
Core 2.2.4 x64 RyuJIT
Method : BenchmarkList
Mean : 370.1 ms
Error : 0.3761 ms
StdDev : 0.3518 ms
Framework 4.7.2 x64 RyuJIT
Method : BenchmarkList
Mean : 481.9 ms
Error : 1.210 ms
StdDev : 1.011 ms
As you can see, the speed of operation is significantly different (at times) in favor of Core.
Microsoft is trying not only to give developers convenient development tools, but also improves the basic things that lead to improvements and optimizations of your projects.
Tier compilation
This is a feature that makes the runtime more adaptive to use the JIT compiler to improve startup performance and maximize throughput.
netcoreapp2.2 7.3 true AnyCPU;x64
Compiles the project as quickly as possible.
Optimizes the most common methods.
This functionality makes your project build faster and gives you almost the same performance. We tested this functionality, and this is a smart feature for NET Core projects, which reduces compilation time. Multilevel compilation slightly slows down the operation of your application, I do not recommend including it on a production server, but for debugging there is more than an actual function that saves programmers time.
Conclusion
Microsoft is trying to improve the lives of developers of the .NET platform. All of the above “goodies” that have appeared in our company allow us to make the environment more open and expandable. I hope you appreciate it. Do not be afraid to switch to a new technology stack and use different features.
Thanks for attention. I hope you enjoyed it.