Application development for an old PDA (Cybiko Xtreme) in 2019
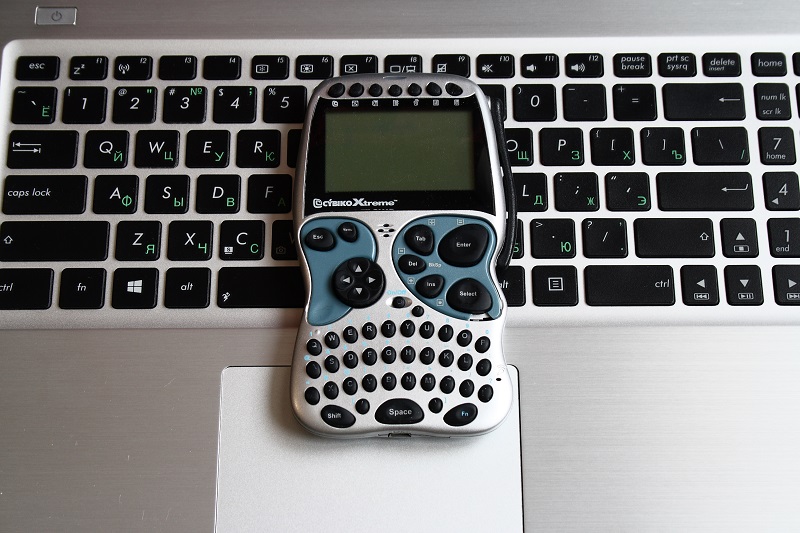
Introduction
Hi Habr! I would like to share my experience writing an application for a very old handheld computer, which is seriously ahead of its time, namely the 2001 Cybiko Xtreme. Those who are interested in how the mobile developer lived in the early 2000s, welcome to cat.
About Cybiko Xtreme
So, this device came to me in the distant 2002 and caused complete delight. I could not appreciate the communication capabilities, since in my city I never met people with such PDAs, however, in general, the ability to play many games without having to sit at a computer was fantastic. In many ways, the creators of the device focused on the network capabilities of the PDA. The developers chose a radio mesh network to connect the devices to each other. Directly, the devices were interconnected at a distance of up to 100 meters, however, it was possible to connect to each other through another device, and in theory, with a dense network coverage, the distance was unlimited. The device had the opportunity to fill out its profile (it seems even to attach a photo, although there would be little sense on the display in grayscale), chat with other users with similar interests, search for new acquaintances, etc. It resembles all this social network, although then such a term, as far as I know, did not exist yet. If there were a lot of devices, I think the project would take off, however, unfortunately, something went wrong with the creators.
Having found this CCP in my old things, I wanted to ponostalgize. It wasn’t very interesting to play old games (nevertheless, they don’t look especially compared to new ones), but to try to develop an application and feel how the first mobile developers lived was much more interesting.
Application idea
Since, by the nature of my activity, I am connected with information security and this topic is extremely interesting to me, I decided to create an application in the same area. The simplest (and at least something useful?) Seemed to me the application “password manager”. This application allows you to see how the development of a graphical user interface, and work with the file system (storage of an encrypted container with passwords).
As a cryptography to protect the container, I decided to use the so-called XOR encryption. The key is the master password. The cryptographic strength of this encryption strongly depends on the key length, and it is not recommended to use it in serious systems, however, this encryption has one serious plus - it is very easy to implement. Since the application was created solely for entertainment purposes, such encryption is ideal.
Emulator
Connecting Cybiko Xtreme to a modern computer is a rather complicated process, so I decided to conduct work on test launches of the application on the emulator (fortunately it is).
The appearance is presented in the figure below.

There are some features for downloading applications to the emulator. According to the instructions found here, the application can be sent using the "Send" button in the "Console" window, however, no matter how I tried, I did not succeed. The Planet Cybiko forum (unfortunately, the forum is currently closed) has a link to a tool that allows you to create your own Cybiko ram disks. Therefore, it is enough to create a ram-disk with a compiled application and restart the emulator with it.
SDK
Finding an SDK on the Internet was not very difficult. After unpacking and analyzing the directory structure, I first drew attention to the doc directory, which turned out to be a very useful help explaining how, in principle, to compile the application, and what structure the project should have.
I found many sample applications in the demos directory. Of particular interest were the applications from the tutorial directory, which step by step explained the development principles for Cybiko Xtreme. Everything is simple, accessible and understandable.
Code writing
The structure of a project that is minimal in content is presented below:
Project structure
project
res
root.inf
src
source1.c
…
make.bat
The root.inf file contains the meta-data of the application, namely information about its type (application, game or library), version, minimum version of the operating system (just like in Android), a unique name, etc.
The make.bat file contains commands for building the application using sdk. In fact, the entire assembly is performed by the Cybiko C Compiller call command:
"C:\Program Files (x86)\Cybiko\Cybiko_SDK\bin\vcc" -R0 src/*.c res/*.help res/*.inf -o passwords_master.app
Important note: when building on Windows 7 for vcc.exe, you need to set the compatibility mode to Windows XP (Service Pack 2), otherwise there are problems with starting the compiler.
The src directory contains the source code for the program. The compilation result is a * .app file.
To describe the development of the entire application, one article is definitely not enough, so I will describe only the very beginning. If someone has questions, ask in the comments or in PM.
It all starts with initializing the application context in the main function by calling:
Initialization
long init_module(struct module_t * main_module);
After initialization, the main_module structure contains pointers to the graphics context and the current application process.
Next, you need to create the main form. This is done as follows:
Create a master form
struct cFrameForm * ptr_main_form = malloc(sizeof(struct cFrameForm));
cFrameForm_ctor(ptr_main_form, "Passwords Master", main_module.m_process);
Check if the password container is on the file system:
Checking Container Availability
int check_database() {
struct FileInput * ptr_file_input = (struct FileInput *) malloc(sizeof(struct FileInput));
FileInput_ctor_Ex(ptr_file_input, DATABASE_FILE);
if (FileInput_is_good(ptr_file_input)) {
FileInput_dtor(ptr_file_input, FREE_MEMORY);
return 0;
}
FileInput_dtor(ptr_file_input, FREE_MEMORY);
return -1;
}
We show the master password input dialog box. If the container already exists, then we try to decrypt with the entered master password. If there was no container, then use the entered master password to create a new container.
Enter Master Password
void get_master_password(struct cFrameForm * ptr_form, char * master_password) {
// Show dialog enter master password
struct cDialog * ptr_pass_dialog;
ptr_pass_dialog = (struct cDialog *) malloc(sizeof(struct cDialog));
cDialog_ctor(ptr_pass_dialog, 0, "Enter master password", mbEdit | mbOk | mbCancel | mbs1, 15, ptr_form->CurrApplication);
cDialog_SetEditText(ptr_pass_dialog, "");
do {
// If pressed cancel - close application
if (cDialog_ShowModal(ptr_pass_dialog) == mbOk)
cDialog_GetEditText(ptr_pass_dialog, master_password);
else {
ptr_form->ModalResult = mrQuit;
break;
}
}
while (strlen(master_password) == 0);
// Releases the dialog
cDialog_dtor(ptr_pass_dialog, FREE_MEMORY);
}
All further work of the application is built around a cycle of processing messages from the operating system until the main form is closed.
Message processing
while (ptr_form->ModalResult != mrQuit) {
struct Message * ptr_message = cWinApp_get_message(ptr_form->CurrApplication, 0, 1, MSG_USER);
switch(ptr_message->msgid) {
case MSG_SHUTUP: // Processes the system exit signal.
case MSG_QUIT:
ptr_form->ModalResult = mrQuit;
break;
case MSG_KEYDOWN: // Processes keyboard input
ptr_key_param = Message_get_key_param(ptr_message);
switch(ptr_key_param->scancode) {
case KEY_ESC:
...
break;
case KEY_ENTER:
...
break;
case KEY_DEL:
...
break;
default:
cFrameForm_proc(ptr_form, ptr_message);
}
break;
default:
cWinApp_defproc(module.m_process, ptr_message);
}
Message_delete(ptr_message);
}
The result of work at startup in the emulator is presented below:

The full source code can be found on github at the link if you wish
.
Launch on a real device
As I already said, launching on a real device is difficult, because Cybiko software for synchronizing with a computer does not support modern operating systems. According to the instructions found here , I was able to synchronize Cybiko with Windows 2000 running in Virtual Box. Of the nuances, I can note that the program saw Cybiko only when it was connected via USB and automatically forwarded to the virtual machine. This is probably why the author of the manual writes that he needs to be added as a permanent device for this machine in the USB Virtual Box settings.
The result of synchronization in the picture below:
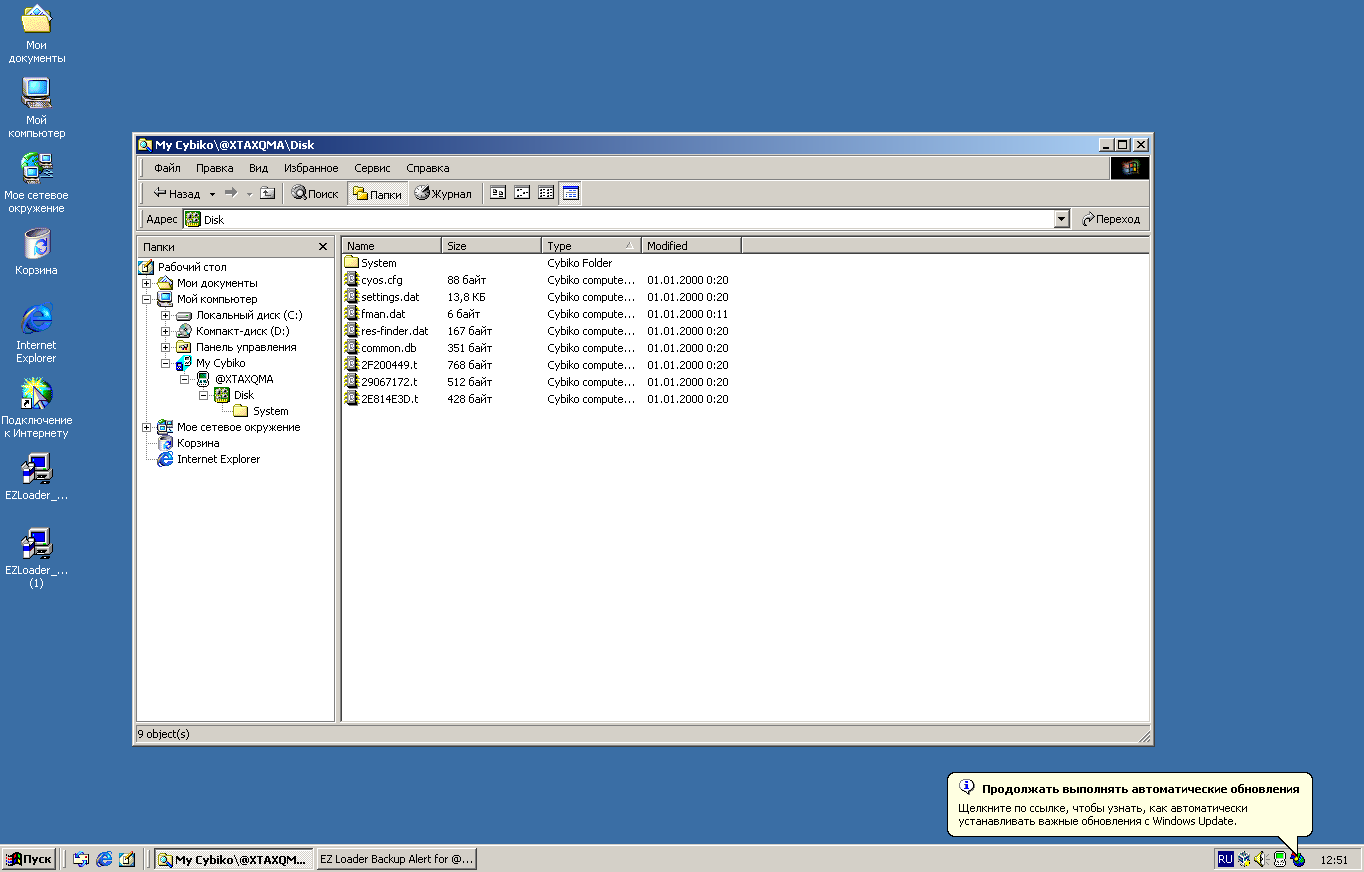
The result of my application is presented in the following video:
Conclusion
I have a lot of wonderful memories connected with Cybiko Xtreme, and once again to touch this wonderful device was damn nice for me. I want to say many thanks to its creators for the work done. I also want to thank the creators of the emulator, forums and sites on Cybiko, as well as my wife for their help in shooting the video and editing this article.
Thank you all for your attention!