Weather Station Arduino
Weather Station Arduino
I decided to write a detailed article telling all aspects of creating an Arduino weather station, since it is difficult to cover the whole process at once. My version of the weather station is convenient in that its data is stored on a computer.
- local network built using a Wifi router
- a computer
Arduino UNO (I used the Chinese replica.)
- USB cable for connecting to Arduino.
barometer BME280 5V I2C (temperature, humidity, pressure sensor)
- soldering station with hairdryer
- solder 0.7 mm
- flux.
Wi-Fi module ESP8266 version ESP-01
- 4 cables mom to dad for breadboard.
- 4 cables male to female for breadboard
- 4 cables 50 cm long for the breadboard.
- 8 thin heat-shrinkable tubes 10 cm long each.
- 1 large heat-shrink tubing of such a diameter so that it includes “4 cables 50 cm long for the breadboard” along with other heat-shrink tubing
Network configuration
Each computer on the network should see the device on which the server is located. It is advisable to install the same workgroups on all computers on the network.

Click to enlarge.
You should disable the bradmauser and other firewalls.
In the network settings, in the properties of the wireless adapter, in the access tab, put at the beginning the checkbox “allow other users to access” and then - click on the settings;
put all the checkmarks in the settings, you will see the names of services instead of numbers.

Click to enlarge.

Click to enlarge.
You must open port 80 and 3306 in the router. I will show by the example of the Tenda router: firstly, find out your Local IP address , for this, click on the wireless network icon. Next, in the opened panel, next to the name of your network, Properties will appear . After, scroll down.

Click to enlarge.
Next, open the router’s web page, I have it http://192.168.0.1 , enter the password for the router, click Advanched settings, then Virtal Server. Then enter the Local IP first and port 80. Then repeat the operation, but enter port 3306 .

Click to enlarge.
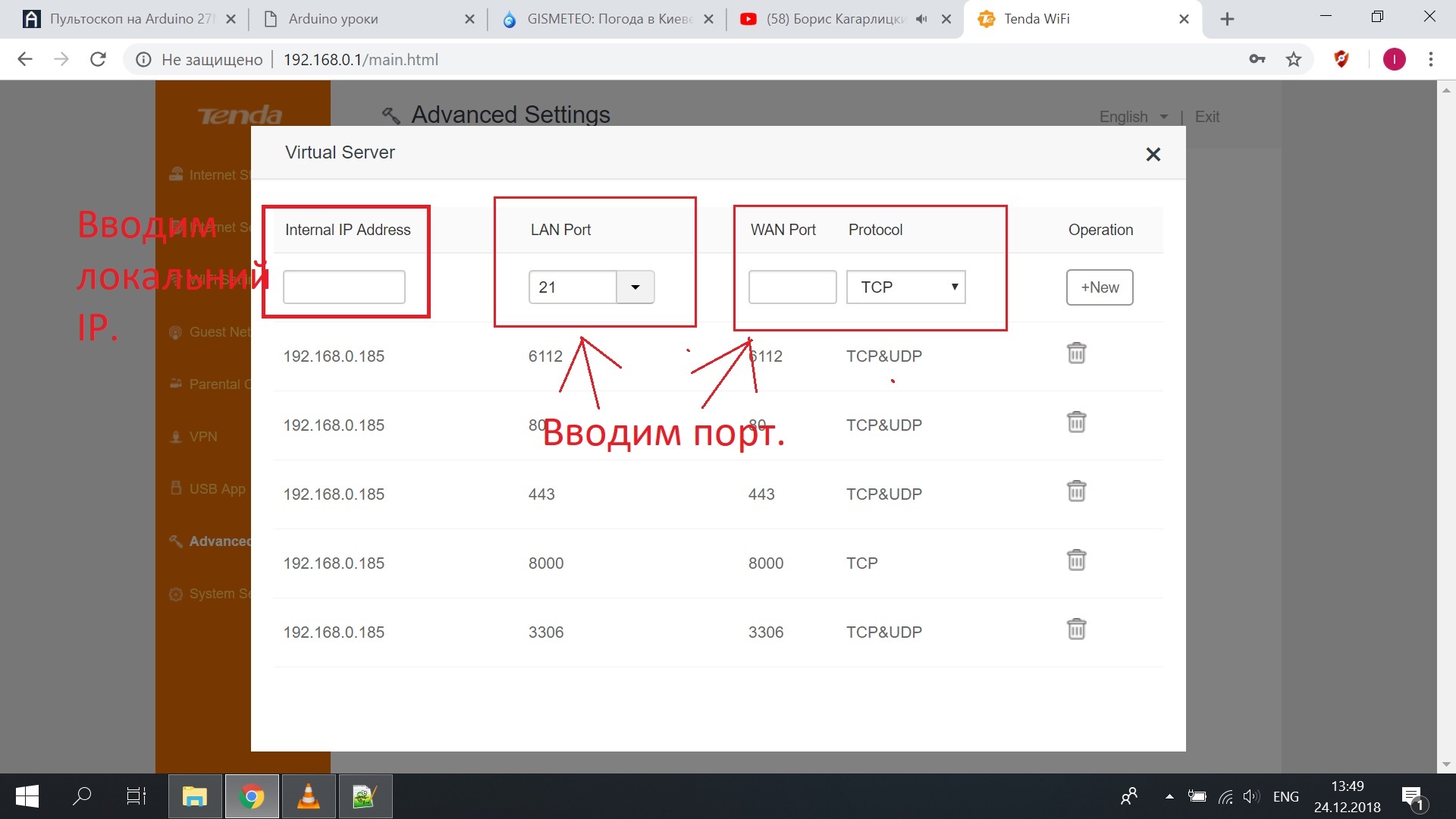
Click to enlarge.
We need the following components:
- Apache - data from the weather station will be sent here.
- PHP - will pick up data from the weather station, and save the database.
- Mysql - databases where the temperature will be stored, since using only PHP means it is impossible to provide display for several computers and other devices.
- HeidiSQL is a viewer and database editor (you can use any other, for example: “PhpMyAdmin”).
I will not describe in detail Apache server settings , because there is a lot of information on the Internet. The main thing is to configure Apache and MySQL to be visible on the local network.
After that, create a table in the database - the temperature will be saved there.
An example of my handler page (sensor readings) on the server:
$link = mysqli_connect("имя сервера в апач", "root", "пароль к бд", "база данных");
if (!$link) {
echo "Ошибка: Невозможно установить соединение с MySQL." . PHP_EOL;
echo "Код ошибки errno: " . mysqli_connect_errno() . PHP_EOL;
echo "Текст ошибки error: " . mysqli_connect_error() . PHP_EOL;
exit;
}
echo "Соединение с MySQL установлено!" . PHP_EOL;
echo "Информация о сервере: " . mysqli_get_host_info($link) . PHP_EOL;
if(isset($_GET['temp'])){
$c=$_GET['temp'];
$vlah=$_GET['vlah'];
$davlen=$_GET['davlen'];
$sql = "UPDATE `temp1` SET `C`='$c',`vlah`='$vlah',`davlen`='$davlen' WHERE `id`='1'";// Занесение данных в бд
mysqli_query($link,$sql);
}
echo"
";
$sql1="SELECT * FROM `temp1`";//Отображение данных
$result = mysqli_query($link,$sql1);
$row = mysqli_fetch_all($result,MYSQLI_NUM);
echo "Температура ";
echo $row[count($row)-1][0];
echo " C";
echo"
";
echo "Давление ";
echo $row[count($row)-1][1];
echo "hPa";
echo"
";
echo "Влажность ";
echo $row[count($row)-1][2];
echo "%";
3. Configuring esp8266
Connect the esp-01 to the adapter and connect it to the Arduino as follows: Rx-RX, TX-TX, GND-GND, VCC-5V ;
Ground the RESET Arduino port to GND ;

Click to enlarge.
Connect your arduino to your computer, open the Arduino IDE , then Serial Monitor . Then specify Rate 115200 , and
Both NL & CR .

Click to enlarge.
- Enter the AT command .
- Highlight OK .
- Enter AT + RESTORE , it will ask the module for factory settings.
- Enter AT + CWMODE_CUR = 1 , this will put the module into client mode.
- Enter AT + CWJAP_CUR = "Network_name", "Password" - connection to wifi.
- Highlight OK if a connection has occurred.
Configure bme280 and final build
Connect the pins according to the diagram (attention on the diagram shows esp8266 without an adapter, but in reality it needs to be connected to the adapter, and from the
adapter 4 pins have the same function as shown in the diagram.)
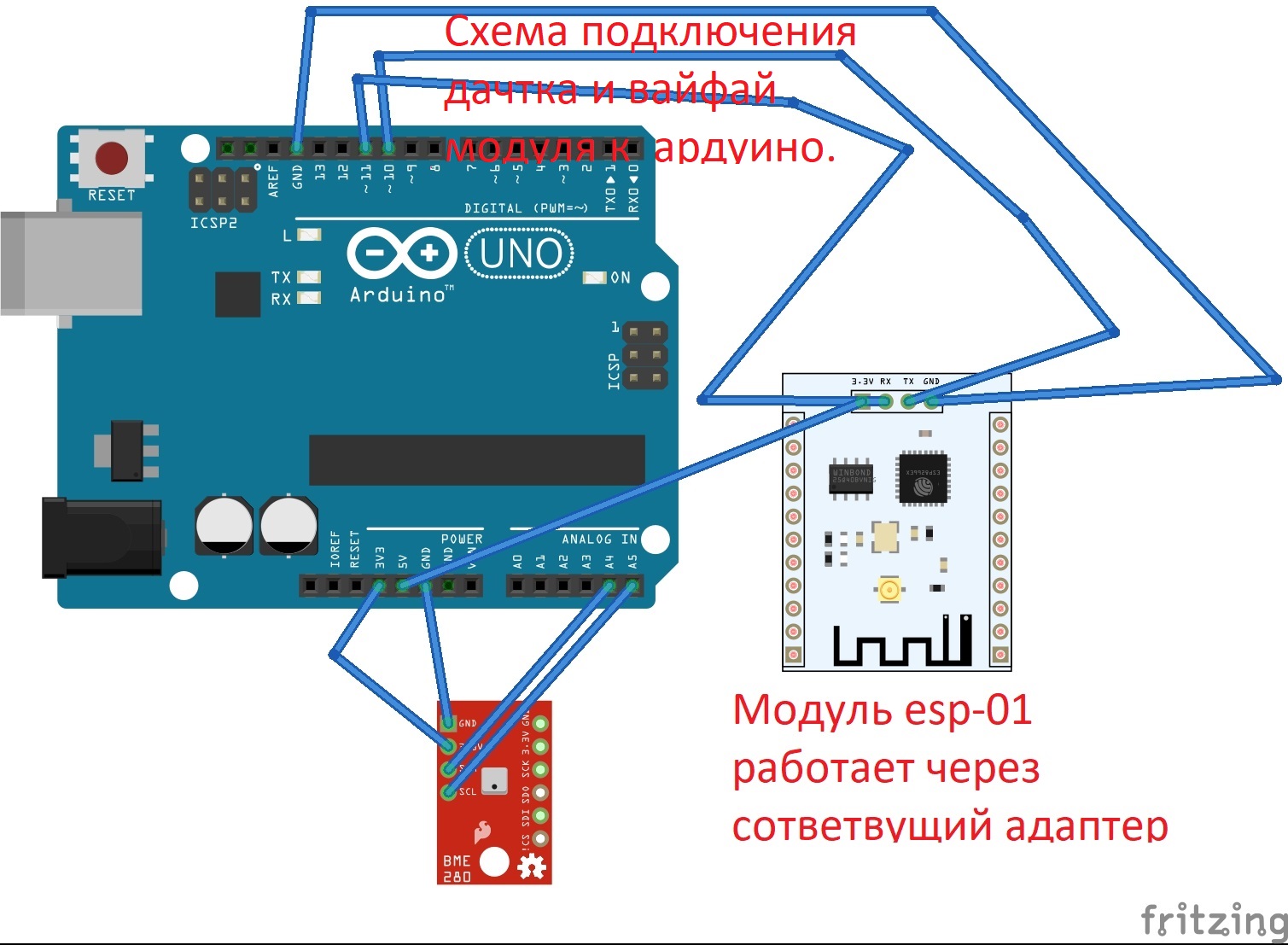
Click to enlarge.
Open the Arduino IDE and paste the following code:
#include
void setup(){
Wire.begin();
Serial.begin(9600);
while (!Serial);
Serial.println("\nI2C Scanner");
}
void loop(){
byte error, address;
int nDevices;
Serial.println("Scanning...");
nDevices = 0;
for(address = 8; address < 127; address++ ){
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0){
Serial.print("I2C device found at address 0x");
if (address<16)
Serial.print("0");
Serial.print(address,HEX);
Serial.println(" !");
nDevices++;
}
else if (error==4) {
Serial.print("Unknow error at address 0x");
if (address<16)
Serial.print("0");
Serial.println(address,HEX);
}
}
if (nDevices == 0)
Serial.println("No I2C devices found\n");
else
Serial.println("done\n");
delay(5000);
Open the Serial Monitor , and remember the highlighted IC2 adress.
Download the Sensor Library , Touch Library libraries and unzip them in C: \ Users \ user \ Documents \ Arduino . Open
C: \ Users \ user \ Documents \ Arduino \ libraries \ Adafruit_BME280_Library-master and change the address:
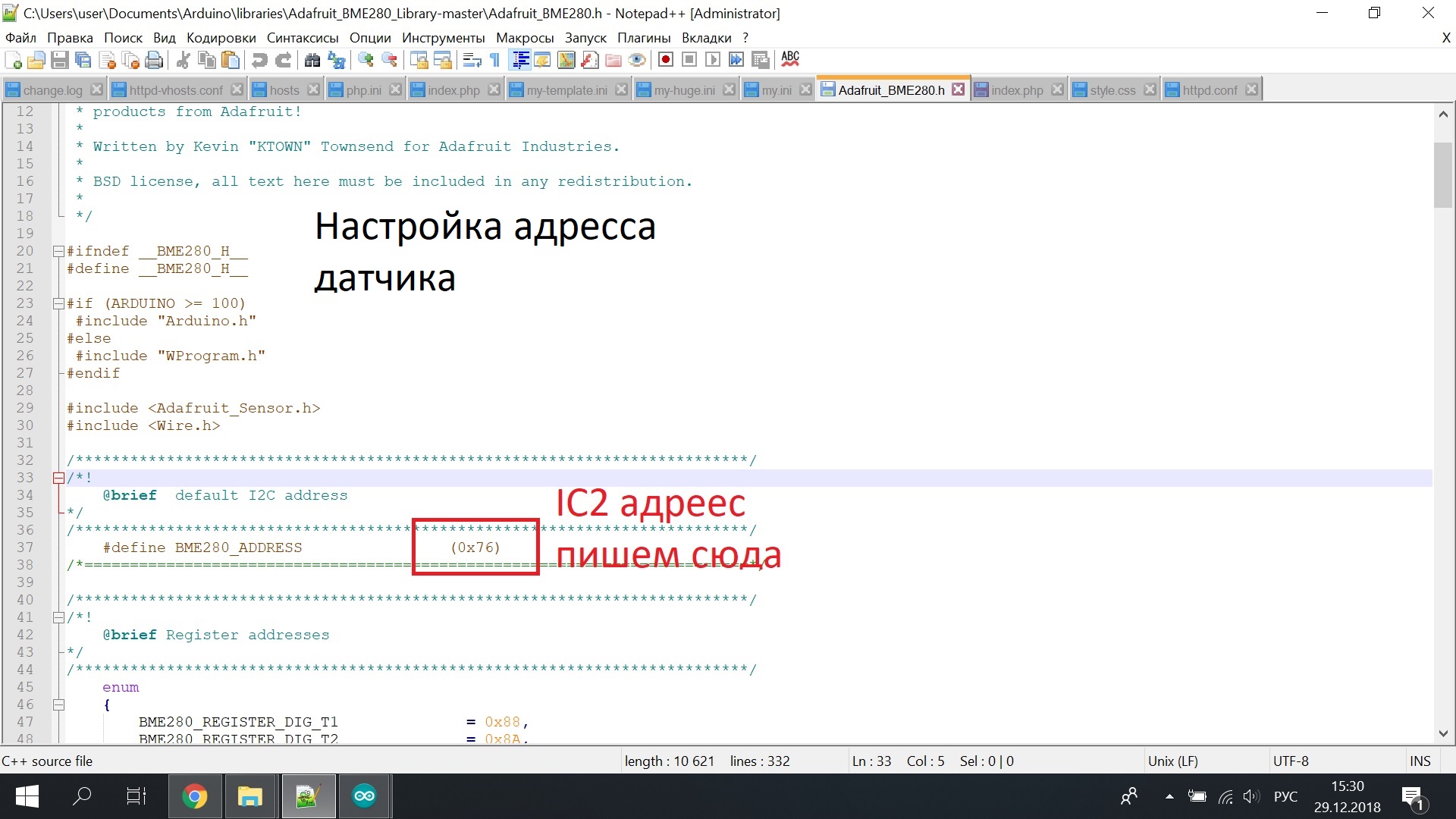
Click to enlarge.
Open the Arduino IDE and put the code:
#include
#define RX 10// Пин подключения к esp8266
#define TX 11// Пин подключения к esp8266
String AP = "Aleks"; // Имя сети
String PASS = "1brui47ci881"; // Пароль
String HOST = "192.168.0.185";//ваш локальный ип
String PORT = "80";//порт апача
String field = "field1";
int countTrueCommand;
int countTimeCommand;
boolean found = false;
int valSensor = 1;
SoftwareSerial esp8266(RX,TX);
#include
#include
#include
#include
#define BME_SCK 13
#define BME_MISO 12
#define BME_MOSI 11
#define BME_CS 10
#define SEALEVELPRESSURE_HPA (1013.25)
Adafruit_BME280 bme; // I2C
//Adafruit_BME280 bme(BME_CS); // hardware SPI
//Adafruit_BME280 bme(BME_CS, BME_MOSI, BME_MISO, BME_SCK); // software SPI
unsigned long delayTime;
void setup() {
Serial.begin(9600);
bool status;
// default settings
// (you can also pass in a Wire library object like &Wire2)
status = bme.begin();
if (!status) {
Serial.println("Could not find a valid BME280 sensor, check wiring!");
while (1);
}
esp8266.begin(115200);
sendCommand("AT",5,"OK");
sendCommand("AT+CWMODE=1",5,"OK");
sendCommand("AT+CWJAP=\""+ AP +"\",\""+ PASS +"\"",20,"OK");
sendCommand("AT+CIPMUX=1",5,"OK");
}
void loop() {
float vlah=bme.readPressure() / 100.0F;
sendCommand("AT+CIPSTART=0,\"TCP\",\""+ HOST +"\","+ PORT,15,"OK");
String cmd = "GET /?temp="+String(bme.readTemperature())+"&vlah="+String(vlah)+"&davlen="+String(bme.readHumidity())+" HTTP/1.1\r\nHost: 192.168.0.185\r\n\r\n";// GET запрос на локальный сервер,temp,vlah,davlen переменые в которые заносяться данные с датчика и заносяться в бд.
sendCommand("AT+CIPSEND=0," +String(cmd.length()+4),4,">");
esp8266.println(cmd);delay(1500);countTrueCommand++;
sendCommand("AT+CIPCLOSE=0",5,"OK");
delay(100000);
}
void printValues() {
Serial.print("Temperature = ");
Serial.print(bme.readTemperature());
Serial.println(" *C");
Serial.print("Pressure = ");
Serial.print(bme.readPressure() / 100.0F);
Serial.println(" hPa");
Serial.print("Humidity = ");
Serial.print(bme.readHumidity());
Serial.println(" %");
Serial.println();
}
void sendCommand(String command, int maxTime, char readReplay[]) {
Serial.print(countTrueCommand);
Serial.print(". at command => ");
Serial.print(command);
Serial.print(" ");
while(countTimeCommand < (maxTime*1))
{
esp8266.println(command);//at+cipsend
if(esp8266.find(readReplay))//ok
{
found = true;
break;
}
countTimeCommand++;
}
if(found == true)
{
Serial.println("OYI");
countTrueCommand++;
countTimeCommand = 0;
}
if(found == false)
{
Serial.println("Fail");
countTrueCommand = 0;
countTimeCommand = 0;
}
found = false;
}
Done. Temperature data will be transferred as variables to the database.
As you can see, my weather station requires an initial knowledge of web programming, but the result is worth it.