
EPAM Quest: Five Tasks from .NET Interviews
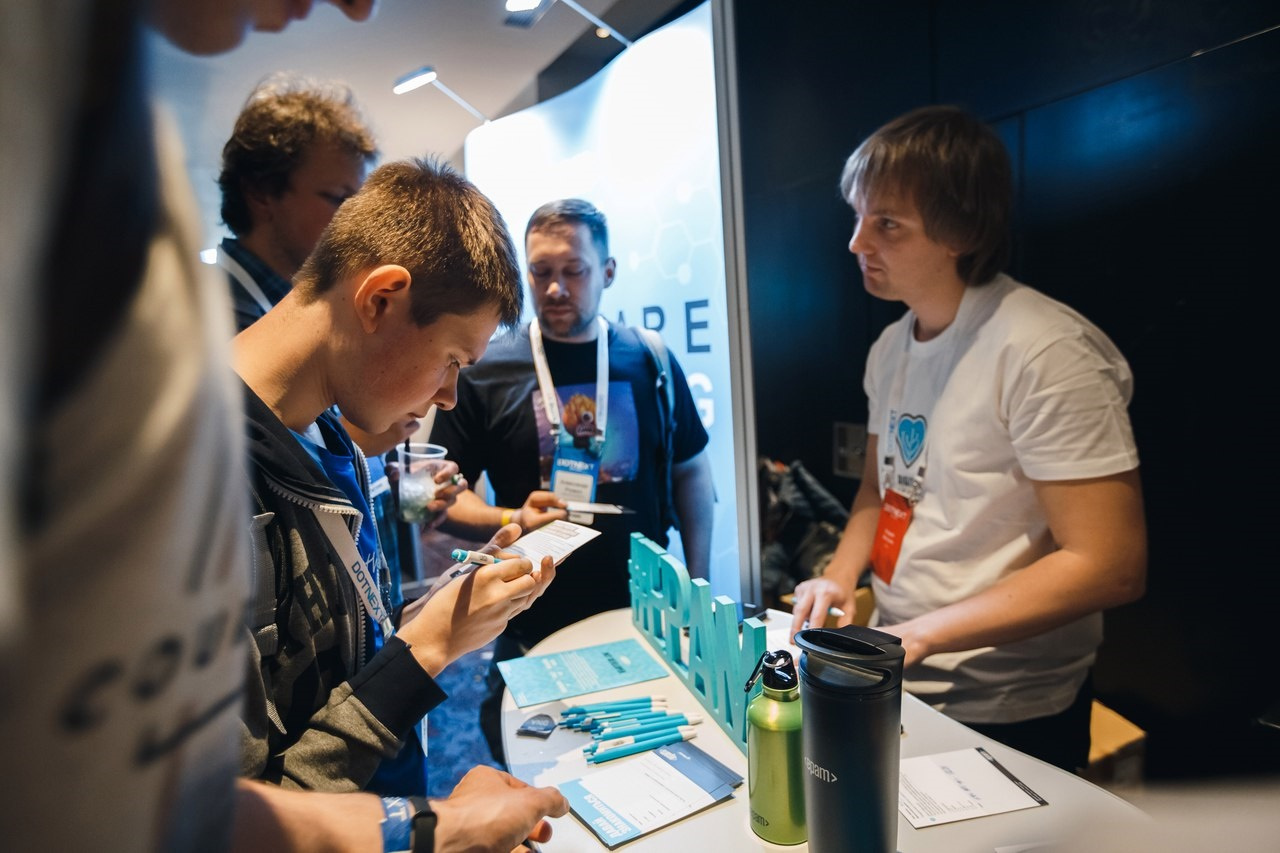
Before joining EPAM, I attended about 20 interviews in St. Petersburg IT companies, and many were given tasks. I synthesized my experience and came up with five tasks that are similar to those that give on online testing and face-to-face interviews.
On May 19-20, the DotNext conference was held in St. Petersburg, where we invited the participants to go through the quest, which consisted of these tasks. Answering the task correctly, the participant received the following.
The first three can be handled by juniors, they are often asked similar questions in interviews. You can ask the fourth one to anyone, but I would count on middle level specialists: not all juniors understand the increasing complexity of algorithms.
The fifth task was done by units. Its complexity is not in calculating what will happen in the select (many have dealt with it just that), but in finding the next number in the sequence, and this requires good mathematical knowledge. It seems to me that the mathematical base is very important for the programmer.
Conference participants came up and asked how to solve problems and where you can see their complete list, and I promised that I would publish them here. The correct answers and comments are hidden so that those who see the tasks for the first time can solve them without prompting.
So, let's begin.
1) What result will this program print to the console?
class Program
{
static void Main(string[] args)
{
var numbers = new int[] { 7, 2, 5, 5, 7, 6, 7 };
var result = numbers.Sum() + numbers.Skip(2).Take(3).Sum();
var y = numbers.GroupBy(x => x).Select(x =>
{
result += x.Key;
return x.Key;
});
Console.WriteLine(result);
}
}
Answer
56. We take into account the features of LINQ. Binary representation: 111000.
A comment
Similar tasks are often given at interviews. If we talk about the quest, then about 80% of the participants gave the correct answer. The most common mistake is that people did not notice that there was a delayed execution of the request. Read more about it here .
2) What result will this program print to the console?
class Program
{
private static string GetNumber(int input)
{
try
{
throw new Exception(input.ToString());
}
catch (Exception e)
{
throw new Exception((int.Parse(e.Message) + 3).ToString());
}
finally
{
throw new Exception((++input).ToString());
}
return (input += 4).ToString();
}
static void Main(string[] args)
{
string result;
try
{
result = GetNumber(1);
}
catch (Exception e)
{
result = e.Message;
}
Console.WriteLine(int.Parse(result) * 100);
}
}
Answer
200. We take into account the specifics of finally. Binary representation: 11001000.
A comment
He himself faced a similar question at the interview. If we talk about the participants of the quest, about 70% were able to answer correctly. They left feedback and suggestions, among which - reduce the amount of extra code. We discussed this and came to the conclusion that it is still better to reduce the readability of the code for artificially misleading.
3) What result will this program print to the console?
class MagicValue
{
public int Left { get; set; }
public int Right { get; set; }
public MagicValue(int left, int right)
{
Left = left;
Right = right;
}
public static void Apply(MagicValue magicValue)
{
magicValue.Left += 3;
magicValue.Right += 4;
magicValue = new MagicValue(5, 6);
}
public static void ApplyRef(ref MagicValue magicValue)
{
magicValue.Left += 7;
magicValue.Right += 8;
magicValue = new MagicValue(9, 10);
}
}
class Program
{
static void Main(string[] args)
{
var magicValue = new MagicValue(1, 2);
MagicValue.ApplyRef(ref magicValue);
MagicValue.Apply(magicValue);
Console.WriteLine(magicValue.Left * magicValue.Right);
}
}
Answer
168. We take into account the features of ref. Binary representation: 10101000.
A comment
This is a very popular question in interviews. I myself solved a similar problem, probably, on every second. You can read on this topic here . By the way, only 45% of the quest participants were able to find the right solution. Often the mistakes were not in understanding the principle of ref, but in mathematics.
4) Given 9 functions. Each function corresponds to a number from 1 to 9. Arrange the functions in order of increasing complexity, and then substitute 9 numbers of sorted functions sequentially in the formula _ _ _ - _ _ _ + _ _ _ = "?". Write what "?" Is equal to.
1.
2.
3.
4.
5.
6.
7.
8.
9.
Answer
1221. Binary representation: 10011000101.
Solution:
6.
1.
3.
2.
4.
9.
8.
5.
7.
6 1 3 - 2 4 9 + 8 5 7 = 1221
Solution:
6.
1.
3.
2.
4.
9.
8.
5.
7.
6 1 3 - 2 4 9 + 8 5 7 = 1221
A comment
Such a task is less common in interviews, but it is a good start for those who study the complexity of algorithms. There are many resources with formulas, and finding them is simple. In addition, the problem can be solved by "substitution" on paper. At the interview they can ask a question related to the complexity of some algorithms, and it's great when the answer is supported by general knowledge. If we talk about the quest, only 30% of the participants coped with the task.
5) Let the carTable table contain the following values:
id | model | price |
---|---|---|
1 | Nissan | 1000 |
2 | BMW | 2000 |
3 | Toyota | 1000 |
4 | Renault | 2000 |
5 | Peugeot | 1000 |
6 | Opel | 2000 |
The result of the SQL query below will be 2 values - <1> and <2>.
;WITH someTable AS
(SELECT 1 val
UNION ALL
SELECT val + 1 FROM someTable WHERE val BETWEEN 1 AND 3)
SELECT carTable.price / SUM(CASE WHEN carTable.price = 1000 THEN 1 ELSE 2 END) / 250 AS result
FROM someTable
INNER JOIN carTable ON carTable.id = someTable.val
GROUP BY carTable.price
ORDER BY carTable.price ASC
<1> and <2> - is a first sequence number <1>, <2>, 6, 34, 270 .
Find the following sequence number.
Answer
2698. Binary representation: 101010001010.
Solution:
The result of the query will be numbers 2 and 2 (BETWEEN will select 1,2,3,4. Join will work on the first 4 records, GROUP BY will group them, SELECT will be calculated as
,
. The sequence is calculated as
where
- the number of items already received.
Those.:





Solution:
The result of the query will be numbers 2 and 2 (BETWEEN will select 1,2,3,4. Join will work on the first 4 records, GROUP BY will group them, SELECT will be calculated as
Those.:
A comment
This is by far the most difficult task. Only 15% of the quest participants coped with it.
Conclusion
Thanks to everyone who solved the problems, and especially to those who managed to get to the end! It was interesting to read your reviews and comments. And those who carefully read the conditions and gave answers in binary form are doubly well done!
Photo taken from the archive of the DotNext conference.