
Check the speed of promises
In this article I decided to post quite interesting, in my opinion, the results of the benchmark of our own production. This benchmark was created to find out the speed of native and bluebird promises.
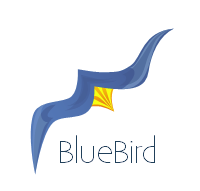
Source code of the benchmark (option number 1):
Source code of the benchmark (option number 2):
This benchmark as a result displays the time for which all promises are resolved. Benchmark results on my machine:
Runtime:
Maximum Allocated Memory (rss):
Mean Allocated Memory (rss):
Conclusion: bluebird promises work 2-5 times faster than native promises, and also require much less RAM.
If I'm wrong about something, then a big request is to inform in the comments. I will be very glad to hear from you any amendments.
» Link to bluebird library
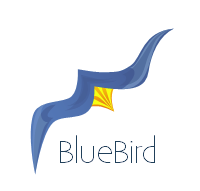
Source code of the benchmark (option number 1):
var Promise = require('bluebird'); // Если хотите проверить скорость работы нативных промисов то необходимо закомментировать данную строку.
var crypto = require('crypto');
var iterations = 1000000;
var start = Date.now();
var arrayPromises = [];
var promiseWrap = function () {
return new Promise(function (resolve, reject) {
crypto.randomBytes(256, (err, buf) => {
if (err) reject(err);
resolve(buf);
});
});
};
for(var i = 0; i < iterations; i++){
arrayPromises.push(promiseWrap());
}
if(arrayPromises.length === iterations){
Promise.all(arrayPromises).then(function (result) {
var finish = Date.now()-start;
console.log("Бенчмарк промисов выполнен!");
console.log("Время выполнения "+finish/1000+" сек.");
});
}
Source code of the benchmark (option number 2):
var Promise = require('bluebird'); // Если хотите проверить скорость работы нативных промисов то необходимо закомментировать данную строку.
var crypto = require('crypto');
var iterations = 1000000;
var start = Date.now();
var arrayPromises = [];
var promiseWrap = function () {
return new Promise(function (resolve, reject) {
setTimeout(function () {
resolve(Math.random(1));
},0)
});
};
for(var i = 0; i < iterations; i++){
arrayPromises.push(promiseWrap());
}
if(arrayPromises.length === iterations){
Promise.all(arrayPromises).then(function (result) {
var finish = Date.now()-start;
console.log("Бенчмарк промисов выполнен!");
console.log("Время выполнения "+finish/1000+" сек.");
});
}
This benchmark as a result displays the time for which all promises are resolved. Benchmark results on my machine:
Runtime:
Native Promises (option # 1 node v6.4.0) - 19.808 sec.
Bluebird Promises (option # 1 node v6.4.0) - 9.654 sec.
Native Promises (option # 1 node v6.5.0) - 19.957 sec.
Bluebird Promises (option # 1 node v6.5.0) - 9.723 sec.
Native Promises (option No. 2 node v6.5.0) - 10.61 sec.
Bluebird Promises (option # 2 node v6.5.0) - 2.208 sec.
Maximum Allocated Memory (rss):
Native promises (option No. 2 node v6.5.0) - 1282 Mb.
Bluebird Promises (option # 2 node v6.5.0) - 601 Mb.
Mean Allocated Memory (rss):
Native promises (option No. 2 node v6.5.0) - 368 Mb.
Bluebird Promises (option # 2 node v6.5.0) - 297 Mb.
Conclusion: bluebird promises work 2-5 times faster than native promises, and also require much less RAM.
If I'm wrong about something, then a big request is to inform in the comments. I will be very glad to hear from you any amendments.
» Link to bluebird library