HTML5 game development in Intel XDK. Part 3. Introducing the Snake
- Transfer

Part 1 » Part 2 » Part 3 » Part 4 » Part 5 » Part 6 » Part 7 // End)

Today we’ll talk about the architecture of the Snake game, built on the Cocos2d-JS platform, and begin to create it. Please read this material by looking at the Cocos2d-JS documentation and download the game resource set . We will use them in the development.

Game Architecture Overview
If you have never played Snake, here is the finished project . Download it to your computer, import into Intel XDK and try it out. To do this, go to the Project tab and click on the Open An Intel XDK Project button, which is located in the lower left corner.

Snake is a simple game. At first, only the head of a snake is displayed on the screen. When controlling a snake, you need to aim it at something edible that appears randomly on the screen. In our case, this is a “cookie” represented by a red dot. Having found a treat, the snake eats it and increases in size. At the same time, the speed of its movement also increases. The game ends if a grown snake, in pursuit of yummy, inadvertently clings to its tail.
In our version of the game, a character can go beyond the boundaries of the screen and appear on its other side. To control the game on the computer, use the keys W, S, A, D. They, respectively, allow you to direct the snake up, down, left and right. On devices with a touch screen, the same function is performed by a gesture of touching the screen with a pull in the desired direction. On the emulator, this can be reproduced by clicking on the screen with the mouse, and without releasing the left button, dragging the pointer in the direction where the snake should move.
Implementation
The game consists of three scenes. These are the menu (MenuScene), the game screen (GameScene), and the game completion screen (GameOver).
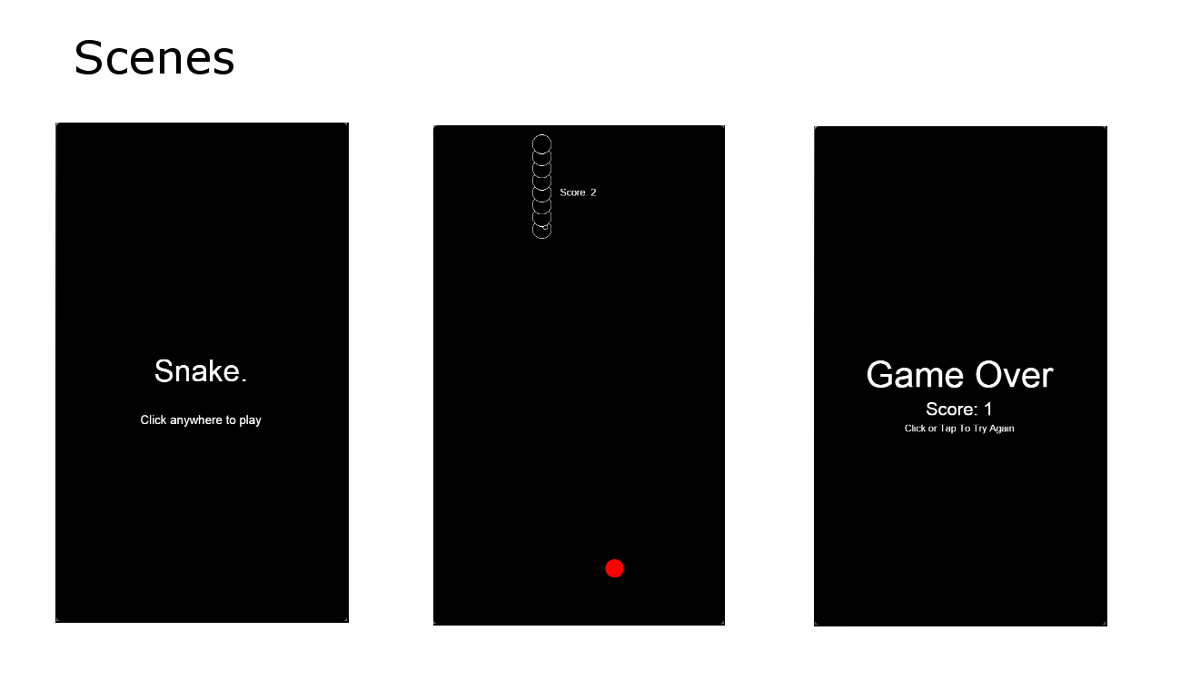
MenuScene game include two sprites that display text. This is the name of the game and an invitation to touch the screen (or click on it) to start the game. There are no layers on this scene. The GameOverScene scene is also arranged. The only difference is that it contains three sprites, the data for one of which, displaying the number of points scored, is taken from GameScene.
Main game screen
The GameScene scene, which is the main game screen, is the place where almost everything called the “game” takes place. Mastering this guide, you will create a game here in parts.
Remove unnecessary from the project template
The standard project template that the Intel XDK gives us contains components that we will not use. We will remove all unnecessary from it.
- Open Explorer or another system file manager.
- Enter the folder with game resources (www / assets), which is located in the project folder.
- Remove from it everything that is there.
- Extract into this folder everything that is in the archive with the game’s resources.
- Open the resource.js file.
var asset = { HelloWorld_png : "asset/HelloWorld.jpg", CloseNormal_png : "asset/CloseNormal.png", CloseSelected_png : "asset/CloseSelected.png" }; var g_resources = []; for (var i in asset) { g_resources.push(asset[i]); }
- Add information about new resources to the asset variable by deleting the old data. Do not change the rest of the code in the file.
var asset = { SnakeHead_png : "asset/snake_head.png", SnakeBody_png : "asset/snake_body.png", SnakeBiscuit_png : "asset/snake_biscuit.png" };
- Delete the app.js file
- Open the project.json file
"jsList" : [ "src/resource.js", "src/app.js" ]
- Remove app.js file description from jsList
- Add a description of the game.js file to jsList
"jsList" : [ "src/resource.js", "src/game.js" ]
- Open the index.html file
- Find the tags
- Replace what they contain with Snake.
As a result, the project file structure should look like the one shown below.

If deleted files are still visible in the Brackets editor file manager, app.js, for example, restart the XDK.
The game frame is ready, however, if you open it now in the emulator, nothing will work. In order to fix this, we will take up the implementation of GameScene.
GameScene Implementation
- Create the game.js. file To do this, go to the Develop tab, execute the menu command File> New (File> New) and save the file in the www / src folder, giving it the name game.js.
- Add the following code to the file:
var GameScene = cc.Scene.extend({ onEnter:function(){ this._super(); } });
Here we describe the GameScene variable and call the onEnter method of the parent of the new layer. - Below “this._super ();” add the following code:
var GameScene = cc.Scene.extend({ snake_layer: {}, onEnter: function(){ this._super(); // Место для нового кодаthis.snake_layer = new SnakeLayer(); this.addChild(this.snake_layer, 0); } });
This is where the SnakeLayer layer is created, which we will implement later. This layer is added as a descendant of GameScene. GameScene only includes a SnakeLayer layer, and already in the layer will contain the logic of the game.
We deal with the head of a snake
In order to make the game character look like a snake, we create it from many fragments that follow the head. This is done due to the fact that the snake must be able to increase in size and make 90-degree turns. The classic Snake game is designed that way. When creating our own version, we follow the established tradition.
Let's proceed with the implementation of the plan by adding the following class to game.js.
var SnakePart = cc.Sprite.extend({
ctor: function(sprite){
/* Вызов конструктора суперкласса с передачей ему спрайта, представляющего фрагмент тела змеи */
this._super(sprite);
},
move: function(posX, posY){
/* Обновляем текущую позицию */
this.x = posX;
this.y = posY;
},
})
This class is used to describe one snake segment. It already contains the move method, which allows you to independently move each of the segments.
The snake itself will be in the SnakeLayer layer. This is the driving force and brain of our character.
We describe the layer by adding the following code to game.js.
var SnakeLayer = cc.Layer.extend({
ctor: function(){
this._super();
}
});
We have already encountered a similar description in GameScene, for example. Only, instead of the onEnter method, here is the constructor of the object. The point here is that we do not just “switch” to the layer (under the direction of the control object), but create it. In addition, in this class there will be a property symbolizing a fragment of the body of the snake (for now - only its head). We will use this variable in order to place the corresponding sprites on the layer.
During work on the game, the constructor of the SnakeLayer layer will grow - commands to initialize various objects will be added to it.
Let's start by creating the head of a snake. This is, in fact, one of the segments of the body with special properties and its own graphic representation.
In order to initialize the snake’s head (snakeHead variable), you need to add the following code to SnakeLayer.
var SnakeLayer = cc.Layer.extend({
snakeHead: null,
ctor: function(){
/* Вызов конструктора суперкласса */
this._super();
/* Создание головы змеи */
this.snakeHead = new SnakePart(asset.SnakeHead_png);
/* Добавление головы в качестве объекта-потомка слоя */
this.addChild(this.snakeHead);
},
});
Here we add an object of the SnakePart class as a descendant object of the SnakeLayer layer. We are almost ready to start the project in the emulator and see something interesting there, but first you need to correct the code of the control object.
- Go to main.js file
- Find the line "runScene"
cc.director.runScene(newHelloWorldScene());
- Replace HelloWorldScene with GameScene.
cc.director.runScene(newGameScene());
Save the changes and run the project in the emulator.
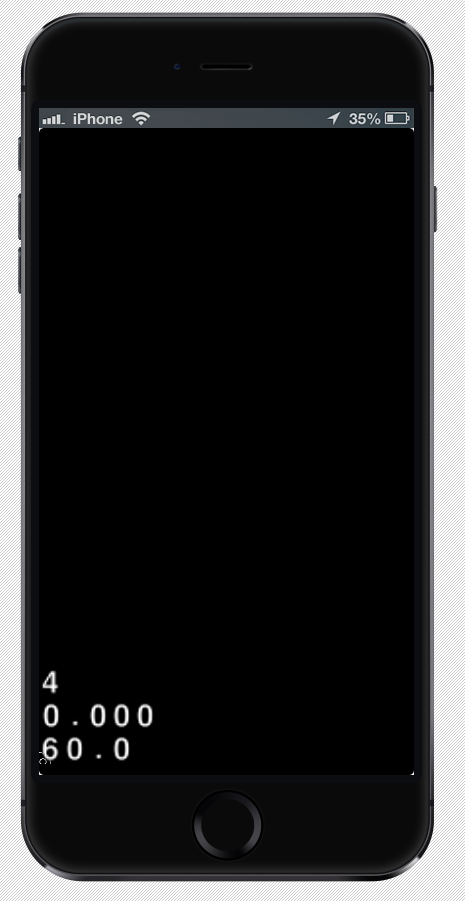
The result of our work is quite noticeable, but the head of the snake rolled into a corner, and even blocked by a frame rate counter. We remove the counter. To do this, do the following.
- Open the project.json file
- Change “showFPS: true,” to “showFPS: false,”
findings
To summarize today's lesson.
- The game that we will develop is a version of the classic Snake.
- The game includes three scenes: a menu (Menu), a game screen (Game), a game completion screen (GameOver).
- The game character, a snake, consists of several parts
That's what you can do now.
- Add game resources to the project and delete them.
- Update resourse.js to account for new or missing resources.
- Delete code files from the project and add them.
- Update the title of the game.
- Create a game scene (GameScene).
- Create layers (using SnakeLayer as an example).
- Implement a snake body part (SnakePart).
- Initialize the head of the snake.
- Disable the output of frame rate information.
At this stage of work on the project, we have a game screen and a snake head in the corner. Next time we’ll take care of the coordinate system and let the snake move to a more interesting place.

Part 1 » Part 2 » Part 3 » Part 4 » Part 5 » Part 6 » Part 7 // End)
