
Implementing Onboarding Experience in your application
Hello to all the guests of the Habr!
In this article, I would like to talk about introducing the Onboarding Experience into an application that you are working on or are going to work on.
“Onboarding Experience” is a small presentation within the application that shows the capabilities of your application in the form of such a slide show. This practice of demonstrating the functionality of the application is used by many companies, such as, for example, Google.
Example “Onboarding Experience” in a Google Drive app:
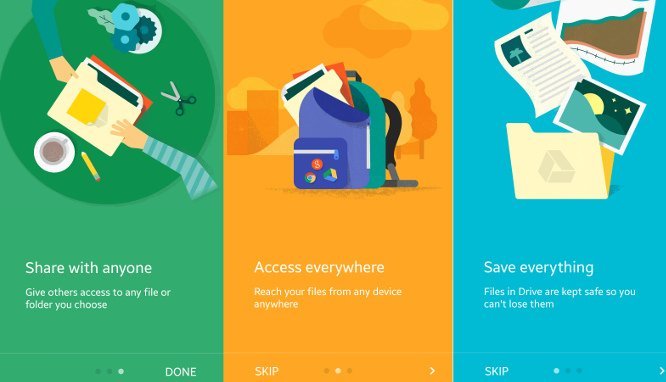
Also, “Onboarding Experience” is called “App Intro” or “Product Tour”. You can call it what you want, because its essence does not change.
So, my story about introducing this feature began after one of the customers of the mobile application asked me to implement such a thing, because he really liked how it looks in other applications. I will say right away that I have not implemented App Intro in his application, but already figured out how to do it quickly and painlessly.
I started looking for a library on GitHub, implying that someone exactly implemented this and did something similar and I need to change the graphics in a few clicks, add a few slides and just play a little under my own requirements.
Having spent not so much time as I expected - I found what I need here .
After reading a little about, I found the best option for implementing this library. You can go deeper and customize everything to your needs. For example, you can use this library with Fragments or just with Activity, change the appearance of the animation, set the vibration when swiping, and much more. I will tell and show the most minimal implementation option.
So, I’ll highlight the implementation in seven points, for convenient and understandable readability, and also highlight the main aspects in bold:
1) Add dependencies to the build.grade (lower level) of your project: 2) Create a SampleSlide.java fragment :
3) Create the CustomIntro.java class :
4) In the layout folder, create how many layouts are needed for Intro (if there are three, then intro_1.xml, intro_2.xml, intro_3.xml)
I think you will figure out where and what to change for different layouts, I gave an example of one of intro.xml
5) In MainActivity.java we write the following code in the OnCreate method to display Intro once at the first start. We do this using thread:
5) In Manifest, declare our Activity :
6) In styles.xml, do not forget to make your own custom theme for displaying Intro in full screen without Toolbar:
7) And the last action, in the drawable folder we put those images that will be used on the screens .
Here you can experiment, for example, with vector graphics or make it more elegant, as Google does. I put the usual .png files with a resolution of 256x256.
Here's what I got:
PS I hope this article will be useful to you. In it, I did not pursue any personal goals. I just tried to expound what I understood, having worked with a good library.
In this article, I would like to talk about introducing the Onboarding Experience into an application that you are working on or are going to work on.
“Onboarding Experience” is a small presentation within the application that shows the capabilities of your application in the form of such a slide show. This practice of demonstrating the functionality of the application is used by many companies, such as, for example, Google.
Example “Onboarding Experience” in a Google Drive app:
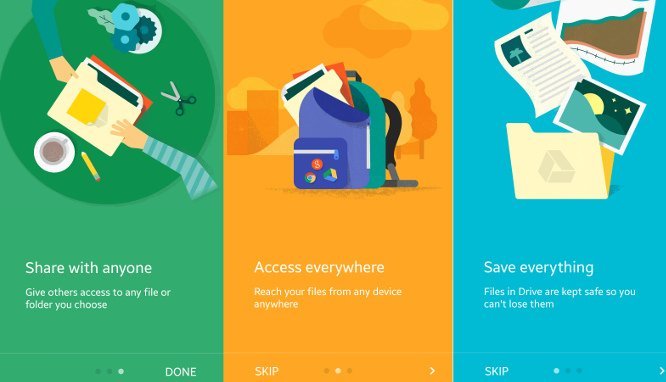
Also, “Onboarding Experience” is called “App Intro” or “Product Tour”. You can call it what you want, because its essence does not change.
So, my story about introducing this feature began after one of the customers of the mobile application asked me to implement such a thing, because he really liked how it looks in other applications. I will say right away that I have not implemented App Intro in his application, but already figured out how to do it quickly and painlessly.
I started looking for a library on GitHub, implying that someone exactly implemented this and did something similar and I need to change the graphics in a few clicks, add a few slides and just play a little under my own requirements.
Having spent not so much time as I expected - I found what I need here .
After reading a little about, I found the best option for implementing this library. You can go deeper and customize everything to your needs. For example, you can use this library with Fragments or just with Activity, change the appearance of the animation, set the vibration when swiping, and much more. I will tell and show the most minimal implementation option.
So, I’ll highlight the implementation in seven points, for convenient and understandable readability, and also highlight the main aspects in bold:
1) Add dependencies to the build.grade (lower level) of your project: 2) Create a SampleSlide.java fragment :
repositories {
mavenCentral()
}
dependencies {
compile 'com.github.paolorotolo:appintro:3.3.0'
}
public class SampleSlide extends Fragment {
private static final String ARG_LAYOUT_RES_ID = "layoutResId";
public static SampleSlide newInstance(int layoutResId) {
SampleSlide sampleSlide = new SampleSlide();
Bundle args = new Bundle();
args.putInt(ARG_LAYOUT_RES_ID, layoutResId);
sampleSlide.setArguments(args);
return sampleSlide;
}
private int layoutResId;
public SampleSlide() {}
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if(getArguments() != null && getArguments().containsKey(ARG_LAYOUT_RES_ID))
layoutResId = getArguments().getInt(ARG_LAYOUT_RES_ID);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(layoutResId, container, false);
}
}
3) Create the CustomIntro.java class :
public class CustomIntro extends AppIntro2 {
@Override
public void init(Bundle savedInstanceState) {
// Здесь указываем количество слайдов, например нам нужно 3
addSlide(SampleSlide.newInstance(R.layout.intro_1)); //
addSlide(SampleSlide.newInstance(R.layout.intro_2));
addSlide(SampleSlide.newInstance(R.layout.intro_3));
}
private void loadMainActivity(){
Intent intent = new Intent(this, MainActivity.class);
startActivity(intent);
}
@Override
public void onNextPressed() {
// Do something here
}
@Override
public void onDonePressed() {
finish();
}
@Override
public void onSlideChanged() {
// Do something here
}
}
4) In the layout folder, create how many layouts are needed for Intro (if there are three, then intro_1.xml, intro_2.xml, intro_3.xml)
I think you will figure out where and what to change for different layouts, I gave an example of one of intro.xml
5) In MainActivity.java we write the following code in the OnCreate method to display Intro once at the first start. We do this using thread:
// Declare a new thread to do a preference check
Thread t = new Thread(new Runnable() {
@Override
public void run() {
SharedPreferences getPrefs = PreferenceManager
.getDefaultSharedPreferences(getBaseContext());
boolean isFirstStart = getPrefs.getBoolean(FIRST_START, true);
if (isFirstStart) {
Intent i = new Intent(MainActivity.this, CustomIntro.class);
startActivity(i);
SharedPreferences.Editor e = getPrefs.edit();
e.putBoolean(FIRST_START, false);
e.apply();
}
}
});
// Start the thread
t.start();
}
5) In Manifest, declare our Activity :
6) In styles.xml, do not forget to make your own custom theme for displaying Intro in full screen without Toolbar:
7) And the last action, in the drawable folder we put those images that will be used on the screens .
Here you can experiment, for example, with vector graphics or make it more elegant, as Google does. I put the usual .png files with a resolution of 256x256.
Here's what I got:
PS I hope this article will be useful to you. In it, I did not pursue any personal goals. I just tried to expound what I understood, having worked with a good library.