
Work with HealthKit. Part 1
In a series of articles, our Techmas colleagues will share their experiences with HealthKit and creating fitness apps. The first article is an introduction to technology and reviews an application that selects personal data from Health.
The HealthKit platform was published by Apple in iOS 8. It is an API for third-party applications that allows you to use to collect information about the user's health status. HealthKit includes the default Health app on iOS8 and iOS9 that displays all available data: physical activity, nutrition, pressure, calories, sleep time, and other personal characteristics.
Immediately after the launch of the platform, developers faced a number of problemsthat led to a temporary ban on its integration. Now everything is fixed and debugged, and there are more and more applications in the AppStore using HealthKit.

So, HealthKit is an aggregate with an interface for access to all information:
Benefits of its use for application developers:
Note that HealthKit and the Health app are not yet available for the iPad.
HealthKit itself is a hierarchy of immutable classes inherited from the abstract class HKObject.
Each object has properties:
Objects in HealthKit can be of two types: characteristics and characteristics. Characteristics are data that does not change over time: gender, date of birth, blood type. Third-party applications cannot modify this data. Samples are available for adding new data. These are class objects inherited from HKSample. They have the following properties:
Below we will consider creating an application that requests the use of HealthKit and displays data on the characteristics of the user.
Only those applications for which the use of HealthKit APIs is the main functionality can use HealthKit. For example, an organizer and record application will not display health data. In addition, there are a number of other limitations:
The entire list of restrictions is available on the Apple website .
Now add HealthKit to the test application. To do this, firstly, install the extension for HealthKit in the project settings.
It is important that the App ID must keep the HealthKit string, otherwise the option will not be available.
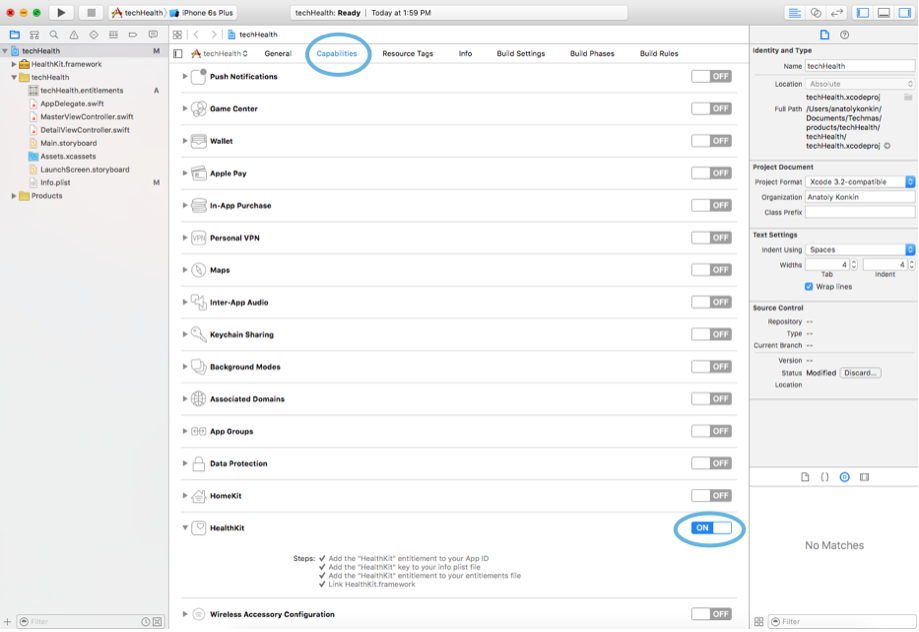
Next, we add user permission to use health data.
Import the extension to use HealthKit:
Now you need to define an object of class HKHealthStore, which is one of the main ones in HealthKit. HealthKitStore allows you to obtain rights to access HealthKit data, the ability to read characteristics and record new samples:
As we noted earlier, the user selects only individual characteristics to which the application has access. All types are descendants of HKObjectType.
Create a function
In it we define the data for reading and writing.
Reading data (specifications):
Data for reading and writing (sampling):
In addition, we add a check for the use of HKHealthStore on the device (HealthKit is currently not available on the iPad):
Finally, we request authorization by passing in the parameters two sets with types Set and set for reading and writing, respectively:
Add a function call to viewDidLoad () :
When the application starts, the window will be available:

The next step is to get the specifications. Add gender and age to the Health app:

Let's create the readProfile () function . Her code is given below:
To access the characteristics, we used the created healthKitStore object .
Possible identifiers and their types for characterization are accessed in the documentation by reference .
Now, using the following call, we get a set of user characteristics:
Sample code is available in git .
In the following articles, we will separately describe how to obtain samples and add training data.
The HealthKit platform was published by Apple in iOS 8. It is an API for third-party applications that allows you to use to collect information about the user's health status. HealthKit includes the default Health app on iOS8 and iOS9 that displays all available data: physical activity, nutrition, pressure, calories, sleep time, and other personal characteristics.
Immediately after the launch of the platform, developers faced a number of problemsthat led to a temporary ban on its integration. Now everything is fixed and debugged, and there are more and more applications in the AppStore using HealthKit.

General information
So, HealthKit is an aggregate with an interface for access to all information:

- HealthKit serves as a common data warehouse for a set of applications. For example, to count the number of steps, users can use both different applications and different devices. When you install each new application, the history will be saved.
- Different applications can exchange data with each other without the use of additional integration solutions. All information is available in the Health app.
- HealthKit provides the ability to configure individual rights for each application for certain indicators.
- Extend the functionality of applications through the use of additional health data from third-party applications.
Note that HealthKit and the Health app are not yet available for the iPad.
Bit of theory
HealthKit itself is a hierarchy of immutable classes inherited from the abstract class HKObject.
Each object has properties:
- UUID The unique identifier for the entry.
- Source Data source (can be either a device or an application).
- Metadata. Additional data about the record. Provides a dictionary containing both predefined and user keys.
Objects in HealthKit can be of two types: characteristics and characteristics. Characteristics are data that does not change over time: gender, date of birth, blood type. Third-party applications cannot modify this data. Samples are available for adding new data. These are class objects inherited from HKSample. They have the following properties:
- Type. Sample type: number of steps, sleep time, etc.
- Start time. Start of sampling time.
- End time. End of sample calculation time. If the sample is in the moment, then the value coincides with the beginning of time.
Application example
Below we will consider creating an application that requests the use of HealthKit and displays data on the characteristics of the user.
HealthKit Use Rights
Only those applications for which the use of HealthKit APIs is the main functionality can use HealthKit. For example, an organizer and record application will not display health data. In addition, there are a number of other limitations:
- Health data cannot be used for promotional purposes. At the same time, applications with HealthKit themselves can use ad units and earn money on ads.
- An application may share information from HealthKit only with the permission of the user and only with that application that is also authorized and uses HealthKit.
The entire list of restrictions is available on the Apple website .
Now add HealthKit to the test application. To do this, firstly, install the extension for HealthKit in the project settings.
It is important that the App ID must keep the HealthKit string, otherwise the option will not be available.
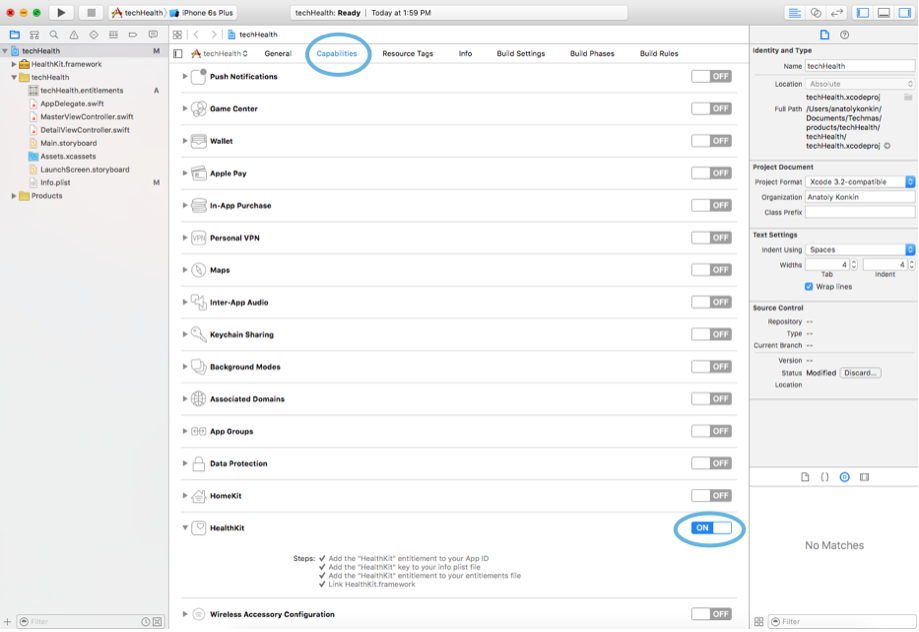
Next, we add user permission to use health data.
Import the extension to use HealthKit:
import HealthKit
Now you need to define an object of class HKHealthStore, which is one of the main ones in HealthKit. HealthKitStore allows you to obtain rights to access HealthKit data, the ability to read characteristics and record new samples:
let healthKitStore: HKHealthStore = HKHealthStore()
As we noted earlier, the user selects only individual characteristics to which the application has access. All types are descendants of HKObjectType.
Create a function
authorizeHealthKit(completion: ((success:Bool, error:NSError!) -> Void)!)
In it we define the data for reading and writing.
Reading data (specifications):
let healthKitTypesToRead = Set(arrayLiteral:
HKObjectType.characteristicTypeForIdentifier(HKCharacteristicTypeIdentifierDateOfBirth)!,
HKObjectType.characteristicTypeForIdentifier(HKCharacteristicTypeIdentifierBiologicalSex)!
)
Data for reading and writing (sampling):
let healthKitTypesToWrite = Set(arrayLiteral:
HKObjectType.quantityTypeForIdentifier(HKQuantityTypeIdentifierActiveEnergyBurned)!,
HKObjectType.quantityTypeForIdentifier(HKQuantityTypeIdentifierDistanceWalkingRunning)!
)
In addition, we add a check for the use of HKHealthStore on the device (HealthKit is currently not available on the iPad):
if !HKHealthStore.isHealthDataAvailable()
{
let error = NSError(domain: "ru.techmas.techmasHealthKit", code: 2, userInfo: [NSLocalizedDescriptionKey:"HealthKit is not available in this Device"])
if( completion != nil )
{
completion(success:false, error:error)
}
return;
}
Finally, we request authorization by passing in the parameters two sets with types Set
healthKitStore.requestAuthorizationToShareTypes(healthKitTypesToWrite, readTypes: healthKitTypesToRead) {
(success, error) -> Void in
if( completion != nil )
{
completion(success:success,error:error)
}
}
Add a function call to viewDidLoad () :
authorizeHealthKit { (authorized, error) -> Void in
if authorized {
print("HealthKit authorization received.")
}
else
{
print("HealthKit authorization denied!")
if error != nil {
print("\(error)")
}
}
When the application starts, the window will be available:

Reading specifications
The next step is to get the specifications. Add gender and age to the Health app:

Let's create the readProfile () function . Her code is given below:
func readProfile() -> (age:NSDate?, bioSex:HKBiologicalSexObject?)
{
// Reading Characteristics
var bioSex : HKBiologicalSexObject?
var dateOfBirth : NSDate?
do {
dateOfBirth = try healthKitStore.dateOfBirth()
bioSex = try healthKitStore.biologicalSex()
}
catch {
print(error)
}
return (dateOfBirth, bioSex)
}
To access the characteristics, we used the created healthKitStore object .
Possible identifiers and their types for characterization are accessed in the documentation by reference .
Now, using the following call, we get a set of user characteristics:
let profile = readProfile()
Sample code is available in git .
In the following articles, we will separately describe how to obtain samples and add training data.