New warning about incorrect array size calculation in gcc 5.1
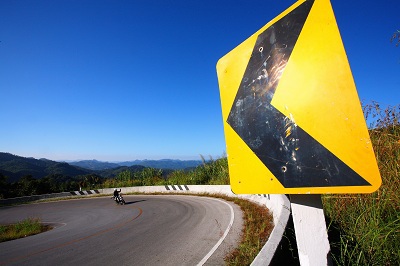
void something( char arr[100] )
{
// this loop is broken
for( size_t index = 0; index < sizeof(arr)/sizeof(arr[0]); index++ ) {
//WHATEVER
}
}
Although the parameter is declared as an array of known size, from the point of view of the C and C ++ compilers, it is a char * pointer , so sizeof (arr) will give the same value as sizeof (char *) - most likely 4 or 8. The cycle, rather total will not work as expected.
Another variant:
void something( char encryptionKey[9000] )
{
// WHATEVER, PROFIT
// this call is broken
SecureZeroMemory( encryptionKey, sizeof(encryptionKey)); // erase the key
}
here, the developers wanted to overwrite some data with zeros , but only the first few bytes would be overwritten due to an error. This error is harder to find by testing than the first.
To find such a code was easier, in gcc 5.1 and later, a warning is issued for such code and it is enabled by default.
Some readers are already in a hurry to comment on the curvature of the hands of the authors of the code from the examples above. However, the problem is so common that in C ++ code it is recommended to use the following focus ( from here ) with the template function:
template
char ( &ArrayElementsCountHelper(StoredType( &Array )[Size]) )[Size];
#define countOfElements(Array) sizeof(ArrayElementsCountHelper (Array))
Using countOfElements () in the code above will result in a compilation error, but this code:
char arr[100]
for( size_t index = 0; index < countOfElements(arr); index++ ) {
//WHATEVER
}
compiles and will work correctly.
In addition to explicitly specifying sizeof (smth) / sizeof (smth [0]), they also use a macro:
// in a header far, far away...
#define errorProneCountOfElements( arr ) (sizeof(arr)/sizeof((arr)[0]))
for( size_t index = 0; index < errorProneCountOfElements (arr); index++ ) {
//WHATEVER
}
Let's see how the new warning works in the above cases. We will try on gcc.godbolt.org
First, we will choose gcc 4.9.2 as the compiler - with default parameters there will be no warnings about incorrect size calculation in any of the examples. Then we change to gcc 5.1.0 - in the examples with the loop we get a line with the title of the loop
warning: 'sizeof' on array function parameter 'arr' will return size of 'char *' [-Wsizeof-array-argument]
In this code:
void somethingExplicitCount( char arr[] )
{
for( size_t index = 0; index < sizeof(arr)/sizeof(arr[0]); index++ ) {
//WHATEVER
}
}
the same warning is issued. Similarly in the macro code:
void somethingMacroCount( char arr[9000] )
{
for( size_t index = 0; index < errorProneCountOfElements(arr); index++ ) {
//WHATEVER, PROFIT
}
}
The rewrite code also gives a warning (use portable memset () for demonstration purposes only):
void somethingMemset( char key[9000] )
{
//WHATEVER, PROFIT
memset(key, 0, sizeof(key)); // don't use memset for sensitive data
}
PROFIT.
Of particular note is the fact that clang version 3.0 already knew how to issue the same warning. This was once said in the LLVM blog that this is a clang-specific warning and that gcc does not know how. NO MOAR.
A warning is enabled by default, developers will only have to correct the problem code correctly.
Dmitry Meshcheryakov,
product department for developers