
Making authorization on Codeigniter 2.0 using ajax and HMVC
Good afternoon, dear Habrachitateli.
Not so long ago, I faced the task of implementing an authorization widget on a site. The idea is not new, I would even say a banal one, but either I googled badly, or the solution is so obvious that it makes no sense to write about it. In any case, even for beginners, this can cause problems, which is why I decided to write this article.
So, for starters, to understand how this is all implemented, you need to get acquainted (for those who are not familiar) with the HMVC extension for Codeigniter. You can do it here .
I will try to do everything very clearly, so I'll start with the file structure of the project:
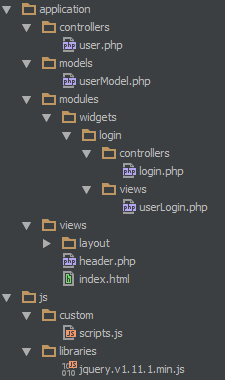
As you can see, I did not specify the entire file system, otherwise the structure that we need.
We submit the output of our authorization form to Modules / widgets, thereby using HMVC, but I implemented all the logic in application / controllers and application / models. Why did I do this? Honestly, I don’t know. You can of course do all this in Modules / widgets, but I decided to do just that. Nobody bothers you to do your own thing.
So, let's take a look at the file system
application / controllers / user - the controller in which ajax processes the authorization and exit events.
application / model / userModel - a model that checks for the existence of the user, and produces the desired array with user data.
application / modules / widgets / login / controllers / login - widget controller where session data will be transferred to.
application / modules / widgets / login / controllers / userLogin - view of the authorization widget.
application / views / layout / head, application / views / layout / footer - a header containing the beginning of the document, and footer
application / views / header - the header of our site, into which the authorization widget is displayed. We do not have controllers that display all this, but I think this is for granted.
js / custom / scripts - our script file, where we will call ajax methods.
and of course the jquery library.
Well, it's time to start already. We start by creating our widget in application / modules / widgets / login / controllers / login
load->view('userLogin');
}
}
Create a view of our widget:
Now pass it to our header view:
On the example of my project, after styling, of course, something like this should turn out:
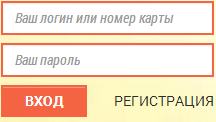
So, it's time to write our authorization. Provided of course that before that you yourself implemented it.
Let's first go to the user controller and create a prefab there with two methods:
class User extends MX_Controller {
public function __construct() {
parent::__construct();
$this->load->model('userModel');
}
public function ajax_login()
{
}
public function ajax_logout()
{
}
}
In advance, we will make a blank in our userModel model:
load->database();
}
// Авторизация
public function check_user($log) {
}
public function user_login($login) {
}
}
Now we are ready by ajax to submit form data. So let’s do it!
$(document).ready(function(){
// Ajax login
$('#account').on('click', '#signIn', function(){
var params = $('#login-form').serialize();
console.log(params); // для дебага, дабы узнать что мы передаем все то, что нужно. Потом можно убрать.
$.post('user/ajax_login', params, function(data){
if(data == 'denied') { // если не поняли, зачем здесь это условие, то поймете позже
alert('Неправильно введены логин или пароль');
} else {
$('#login-form').remove();
$('#account').append(data);
}
});
});
});
We transmitted the data, let's process it in the ajax_login method:
public function ajax_login()
{
$log['login'] = trim(strip_tags($_POST['login']));
$log['pass'] = sha1(md5($_POST['pass']));
$check = $this->TemplateModel->check_user($log);
if($check) {
$data['user_info'] = $this->TemplateModel->user_login($log['login']);
$ses_data = array(
'login' => $data['user_info']['login'],
);
$this->session->set_userdata($ses_data);
} else {
echo 'denied';
}
}
Dark spots remain at the moment, but we will disperse them now. Let's go into our model and create the check_user method, where we pass our $ log array
public function check_user($log) {
$this->db->where('login', $log['login']);
$this->db->where('password', $log['pass']);
$query = $this->db->get('user');
if($query->num_rows > 0) {
return TRUE;
} else {
return FALSE;
}
}
In this method, we check whether such fields exist, and if their number is greater than 0, then return TRUE.
Next, we return the operation of the user_login method to the $ data ['user_info'] array, into which we transfer the $ log ['login'] array data
public function user_login($login) {
$this->db->where('login', $login);
$query = $this->db->get('energy_user');
return $query->row_array();
}
And finally, we write the values we need into the session. And after the line:
$this->session->set_userdata($ses_data);
insert the magic line, which gives the result we need:
redirect(base_url().'widgets/login/login/userLogin');
Here, in fact, almost everything, it remains only to transfer the session values to our widget controller:
session->userdata('login');
$this->load->view('userLogin',$data);
}
}
Now everything works for us! Almost a profit. It remains to do a similar LogOut. Next, I will give 2 sections of code. I think you yourself will figure out where to insert them.
But before that you need to slightly modify the appearance of our widget:
And here are 2 promised sections of code:
public function ajax_logout()
{
$logout = $_POST['logout'];
if($logout) {
$this->session->unset_userdata('login');
redirect(base_url().'widgets/login/login/userLogin');
}
}
$('#account').on('click', '#logout', function(){
$.post('user/logout', {logout : true}, function(data){
$('#userWidget').remove();
$('#account').append(data);
});
});
In general, this is all I wanted to talk about. Of course, you can finish a lot, for example, if, being in your personal account, accidentally or even specially clicked the exit button in the widget, well, and the like.
I will be glad to hear the criticism of professionals.