
Door lock. Rfid
Introduction
Good day! In parallel to my previous article, I worked on another “project”. Actually, I had a couple of Chinese RFID readers lying around. Here are these:
photo RC522
Before that, sorting through the tool box, I found a brand new, but useless lock.
Device operation video
There is another Arduino on the video - it is only needed to power the Arduino Pro Mini. Of course, it is not in the final circuit, I just have not purchased a 5V power supply yet. Under the cut a lot of photos!
Read more
How it works?
The whole system consists of 5 elements:
- RFID Module RC522 13.56 Mhz;
- Microcontroller Arduino Pro Mini;
- Transistor;
- Voltage stabilizer 3.3V;
- Servo TowerPro SG-90.
Until the label is brought up to the RC522, the microcontroller listens to the state of the leg on which the button hangs. If the input is high voltage - the program inverts the position of the system: the lock is open - close, and vice versa. Depending on the open / closed, the LEDs light up: red - closed, green - open. Finally, put the mark on the RC522. Arduino reads 16 bytes of data and checks against the existing two-dimensional array of users. If there is a coincidence, the system is inverted, otherwise nothing happens.
For the lock we need:
Microcontroller Arduino.
Photo Arduino Pro Mini
RFID Tag Reader RC522. Photo module at the beginning of the article.
Servo TowerPro SG-90. Just because I was at hand. In fact, buying a more powerful and reliable would not be superfluous.
Photo TowerPro SG-90
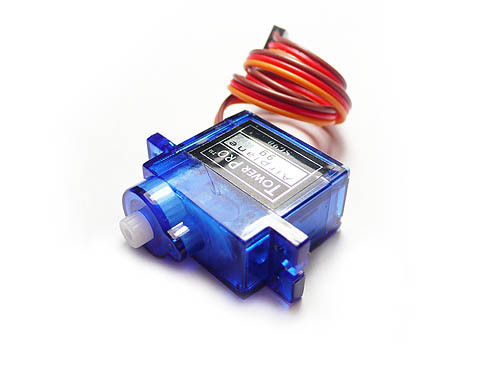
Any suitable transistor. I had 2N2222.
Photo 2N2222
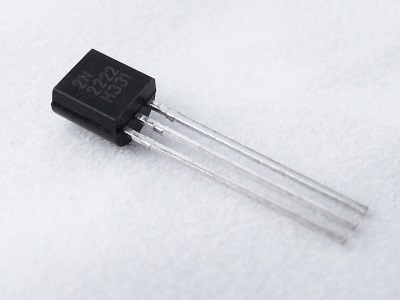
Any voltage regulator 3.3V. In stock was LF33CV.
Photo LF33CV
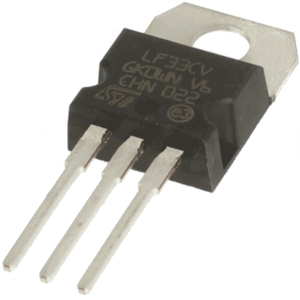
And of course the keys. While I was in Kiev, I bought this silicone RFID bracelet:
RFID Wristband Photo
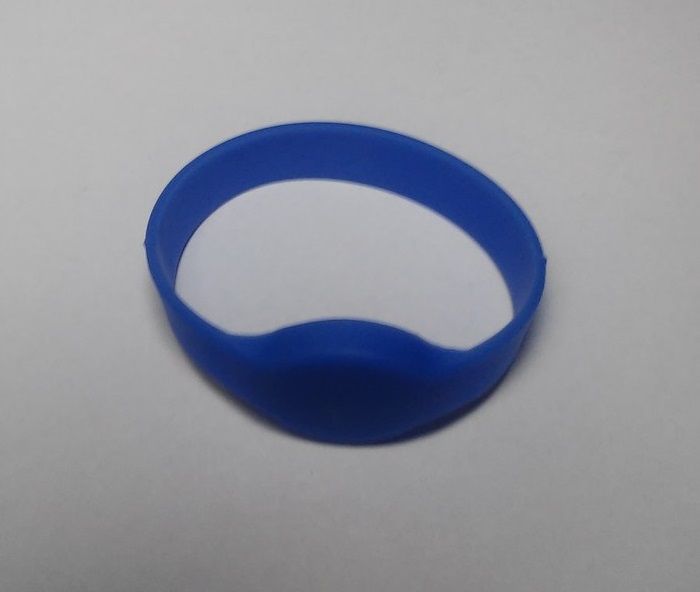
Device assembly
Lock
Step by step to remove the process of remaking the castle did not work. But I think it will be so clear.
The servo hole was neatly cut out and the bolt holes were drilled. The drive lever is made of two parts that came with it, and a regular clip. A screw is screwed at the end.
The locking part of the lock was reinforced at the base of the pins, and also thickened so as not to dangle.
Scheme
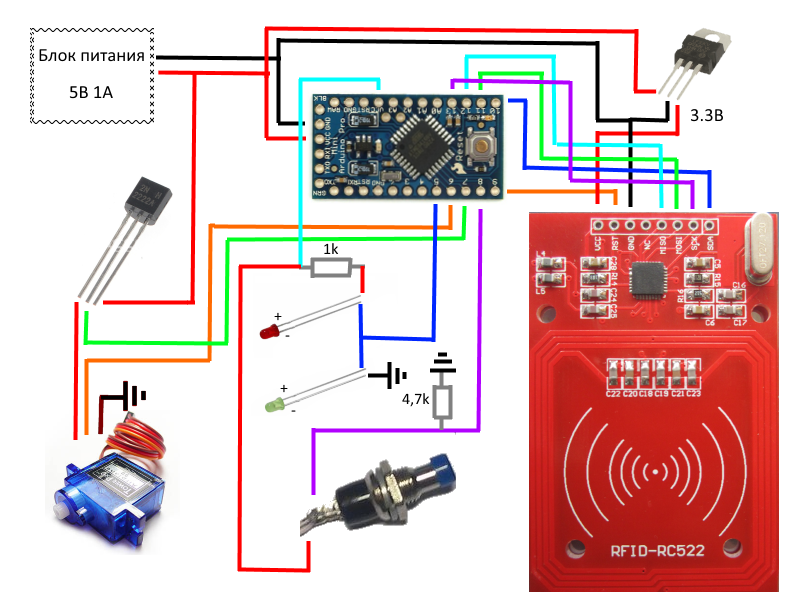
On the printed circuit board itself, there is an IR receiver connector, but did not implement it.
Device


In the final device, I completely forgot about the resistor for the button, but in the circuit I added it.
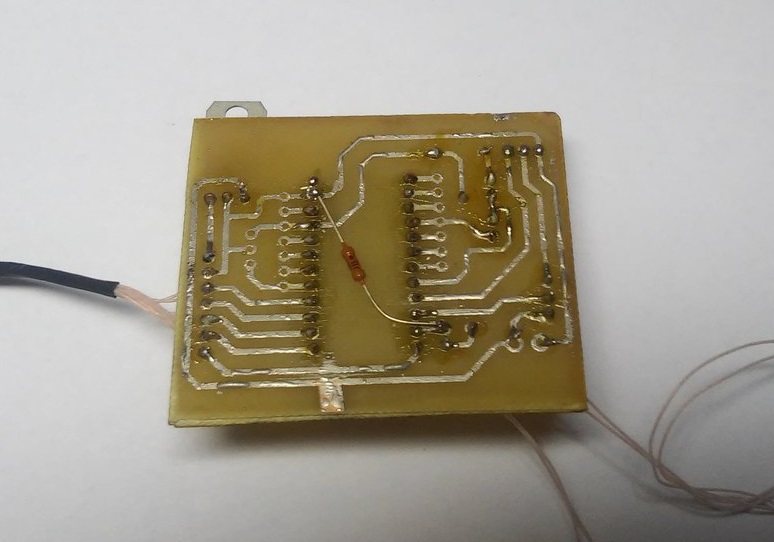
To power the Arduino Pro Mini, a plug was taken from an old PC.

Software part
Lock
/*
-----------------------------------------------------------------------------
* Pin layout should be as follows:
* Signal Pin Pin Pin
* Arduino Uno Arduino Mega MFRC522 board
* ------------------------------------------------------------
* Reset 9 5 RST
* SPI SS 10 53 SDA
* SPI MOSI 11 51 MOSI
* SPI MISO 12 50 MISO
* SPI SCK 13 52 SCK
*
*
*/
#include
#include
#include
#define SS_PIN 10
#define RST_PIN 9
MFRC522 mfrc522(SS_PIN, RST_PIN); // Create MFRC522 instance.
Servo myservo;
boolean doorOpen;
/*
Users
*/
int countUsers = 2;
byte Users[2][16] = {{1,2,3,4, 5,6,7,8, 9,10,255,12, 13,14,15,16},
{0,0,0,0, 0,0,0,0, 0,0,0,0, 0,0,0,1}};
void setup() {
Serial.begin(9600);
pinMode(8, INPUT); //button open/close door
pinMode(7, OUTPUT); //load transistor
digitalWrite(7, LOW);
pinMode(5, OUTPUT); //LED open/close door
myservo.attach(6);
SPI.begin();
mfrc522.PCD_Init();
}
void loop() {
MFRC522::MIFARE_Key key;
for (byte i = 0; i < 6; i++) {
key.keyByte[i] = 0xFF;
}
if ( ! mfrc522.PICC_IsNewCardPresent()) {
if (digitalRead(8) == HIGH) {
digitalWrite(7, HIGH);
delay(500);
if (doorOpen)
{
myservo.write(80);
doorOpen = false;
Serial.println("CLOSED!");
digitalWrite(5, HIGH);
}
else
{
myservo.write(2);
doorOpen = true;
Serial.println("OPENED!");
digitalWrite(5, LOW);
}
delay(500);
digitalWrite(7, LOW);
}
return;
}
if ( ! mfrc522.PICC_ReadCardSerial()) {
return;
}
Serial.print("!");
Serial.print("\r\n");
for (byte i = 0; i < mfrc522.uid.size; i++) {
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " ");
Serial.print(mfrc522.uid.uidByte[i], HEX);
}
Serial.print("\r\n");
byte piccType = mfrc522.PICC_GetType(mfrc522.uid.sak);
//Serial.print(mfrc522.PICC_GetTypeName(piccType));
//Serial.print("\r\n");
if ( piccType != MFRC522::PICC_TYPE_MIFARE_MINI
&& piccType != MFRC522::PICC_TYPE_MIFARE_1K
&& piccType != MFRC522::PICC_TYPE_MIFARE_4K) {
return;
}
byte sector = 1;
byte valueBlockA = 4;
byte valueBlockB = 5;
byte valueBlockC = 6;
byte trailerBlock = 7;
MFRC522::StatusCode status;
status = mfrc522.PCD_Authenticate(MFRC522::PICC_CMD_MF_AUTH_KEY_A, trailerBlock, &key, &(mfrc522.uid));
if (status != MFRC522::STATUS_OK) {
Serial.print("PCD_Authenticate() failed: ");
Serial.println(mfrc522.GetStatusCodeName(status));
return;
}
status = mfrc522.PCD_Authenticate(MFRC522::PICC_CMD_MF_AUTH_KEY_B, trailerBlock, &key, &(mfrc522.uid));
if (status != MFRC522::STATUS_OK) {
Serial.print("PCD_Authenticate() failed: ");
Serial.println(mfrc522.GetStatusCodeName(status));
return;
}
byte buffer[18];
byte size = sizeof(buffer);
status = mfrc522.MIFARE_Read(valueBlockA, buffer, &size);
Serial.print(buffer[0]);
Serial.print("\r\n");
Serial.print(buffer[1]);
Serial.print("\r\n");
Serial.print(buffer[2]);
Serial.print("\r\n");
Serial.print(buffer[3]);
Serial.print("\r\n");
Serial.print(buffer[4]);
Serial.print("\r\n");
Serial.print(buffer[5]);
Serial.print("\r\n");
Serial.print(buffer[6]);
Serial.print("\r\n");
Serial.print(buffer[7]);
Serial.print("\r\n");
Serial.print(buffer[8]);
Serial.print("\r\n");
Serial.print(buffer[9]);
Serial.print("\r\n");
Serial.print(buffer[10]);
Serial.print("\r\n");
Serial.print(buffer[11]);
Serial.print("\r\n");
Serial.print(buffer[12]);
Serial.print("\r\n");
Serial.print(buffer[13]);
Serial.print("\r\n");
Serial.print(buffer[14]);
Serial.print("\r\n");
Serial.print(buffer[15]);
Serial.print("\r\n");
byte trueBytes = 0;
boolean acceptUser = false;
for (int i = 0; i < countUsers; i++) {
if (!acceptUser) {
for (int j = 0; j < 16; j++) {
if (buffer[j] == Users[i][j]) {
trueBytes++;
}
}
}
if (trueBytes == 16) {
digitalWrite(7, HIGH);
delay(500);
if (doorOpen)
{
myservo.write(80);
doorOpen = false;
Serial.println("CLOSED!");
digitalWrite(5, HIGH);
}
else
{
myservo.write(2);
doorOpen = true;
Serial.println("OPENED!");
digitalWrite(5, LOW);
}
delay(500);
digitalWrite(7, LOW);
acceptUser = true;
trueBytes = 0;
} else trueBytes = 0;
}
mfrc522.PICC_HaltA();
mfrc522.PCD_StopCrypto1();
}
Output
“Boolean yes = false;” will be gone , but everything can be :)
Used libraries: MFRC522.h and Servo.h . An example was taken from the RFID library and added to it.
The examples also have a function to write the first block (after the second, the first read-only block). I was enough 16 bytes of data. Of course, it would be better to use another UID then it would be more reliable, but so far I have not intended to put it somewhere.