
Multiple Hipster JavaScript Hacks
- Transfer
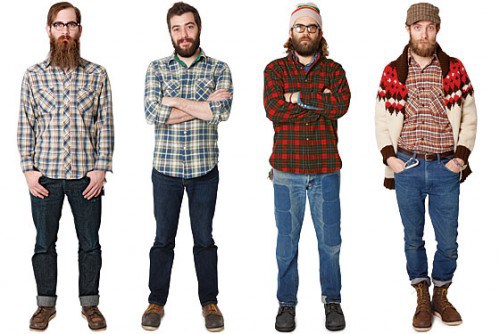
JavaScript is an entertaining programming language.
Constant fears of making mistakes in the code make you think all the time. Programming skills are improved due to the fact that you have to constantly execute code in your mind, there is no other way.
That's why it is important that the code is neat, compact, and sleek. One that you can fall in love with. Otherwise, JavaScript might scare you off.
I have selected for you some interesting snippets that please me, and which I myself use instead of a boring code that takes up a lot of space. Some of them make the code shorter, others sharper and clearer. A couple more are debugging hacks.
I learned all this by studying projects with source code, but here I write as if it was I who invented them.
Hipster Hack # 1 - Function Calling
I really hate the if / else block, getting rid of it will help us with a useful trick that allows you to make function calls based on a Boolean value.
// Скучно
if (success) {
obj.start();
} else {
obj.stop();
}
// По хипстерски
var method = (success ? 'start' : 'stop');
obj[method]();
Hipster Hack # 2 - String Join
Known fact - strings are friends with other strings. Sooner or later you will need to concatenate several of them. I don’t really like using "+", so join comes to the rescue:
['first', 'name'].join(' '); // = 'first name';
['milk', 'coffee', 'suger'].join(', '); // = 'milk, coffee, suger'
Hipster hack # 3 - default operator ||
In JavaScript, you never know exactly what is in an object. Sometimes you get it as an argument to a function, sometimes it is passed to you over the network or you read it in the config. In any case, you can use the convenient operator || which returns the second value if the first is false.
// возвращает 'No name' когда myName пусто (null или undefined)
var name = myName || 'No name';
// либо options нам передано, либо инициализируем его пустым объектом
var doStuff = function(options) {
options = options || {};
// ...
};
Hipster Hack # 4 - Security Operator &&
Like the default operator, it is extremely useful. It allows you to get rid of almost all IF and makes the code much more pleasant.
// Скучно
if (isThisAwesome) {
alert('yes'); // it's not
}
// Прикольно
isThisAwesome && alert('yes');
// Так же полезно для подстраховки
var aCoolFunction = undefined;
aCoolFunction && aCoolFunction(); // не запустится, но и не вылетит с ошибкой
Hipster Hack # 5 - Operator XXX
This operator is fully covered by copyright law. When I write code and for some reason I need to go online or edit another piece of code, I add a line with the xxx operator . The code in this place will be interrupted by the beloved one and it will be possible to finish what was started later. The string xxx is perfectly looked for, since it is not found in regular code, and generally there is no need to bother with a comment in TODO.
var z = 15;
doSomeMath(z, 10);
xxx // Отличный оператор. Я единственный, кто его использует вместо TODO
doSomeMoreMath(z, 15);
Hipster Hack # 6 - Time Measurement
You are interested in knowing which is faster: a loop with i ++ or with i--. I personally do not. Those who are interested can use the output of time measurements to the console. This is useful if you need to know the speed of operations that block the event loop.
var a = [1,2,3,4,5,6,7,8,9,10];
console.time('testing_forward');
for (var i = 0; i < a.length; i++);
console.timeEnd('testing_forward');
// вывод: testing_forward: 0.041ms
console.time('testing_backwards');
for (var i = a.length - 1; i >= 0; i--);
console.timeEnd('testing_backwards');
// вывод: testing_backwards: 0.030ms
Hipster Hack # 7 - Debugging
I learned this trick from one Java developer. I have absolutely no idea why he knew about him, but I do not. But be that as it may, since then I use it constantly. Just enter debugger into the code and the debugger stops at this line.
var x = 1;
debugger; // Здесь мы остановимся
x++;
var x = Math.random(2);
if (x > 0.5) {
debugger; // Условная точка останова...
}
x--;
Hipster Hack # 8 - Old School Debugging
I always liked to display the values of variables in some places of the code, instead of line-by-line debugging. If you are like me, you may need to put some private variables in the global scope. The main thing is to remember to clean up the code before passing it to the production.
var deeplyNestedFunction = function() {
var private_object = {
year: '2013'
};
// Делаем глобальной для отладки:
pub = private_object;
};
// Теперь в консоли вбиваем (Chrome dev tools, firefox tools, и т.д.)
pub.year;
Hipster Hack # 9 - Ultra-Light Template
Are you still using + to concatenate strings? There is a better way to join rows with data. It's called templating, and here's a great 2.5-line code framework.
var firstName = 'Tal';
var screenName = 'ketacode'
// Уродский способ
'Hi, my name is ' + firstName + ' and my twitter screen name is @' + screenName;
// Пацанский
var template = 'Hi, my name is {first-name} and my twitter screen name is @{screen-name}';
var txt = template.replace('{first-name}', firstName)
.replace('{screen-name}', screenName);
Did you already know all this?
And even the operator XXX invented by me personally? Yes, you are just a real hipster hacker!