Migration option from jQuery to pure javascript
The jQuery library was created in 2006 to fill in the missing Javascript functionality. Since then, the latter has progressed sufficiently in its development, so that developers can do without jQuery, the main problem of which is performance.
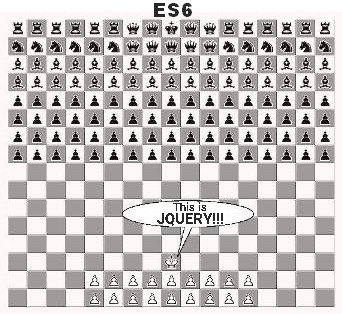
On Habré there were several articles with objective measurements of the "inhibition" of JQuery on selector queries.
(run in a cycle 10,000 times)
There are a lot of good descriptions of analogs of jQuery functions on pure Javascript-e — for example, here .
But the power of jQuery is in the brevity and beauty of its expressions. It is even psychologically difficult to rewrite the existing code, changing the elegant $ () into multi-line constructions.
Let's try, as far as possible, to leave the jQuery language, [partially] replacing it with itself. To do this, we only need to either override the $ () function, or replace (which is better) with our own - let it be $ jqr (). It will also return an object, but already “native” and not burdened with unnecessary jQuery functions.
Code example:
Jquery code:
Changes to:
In the class constructor it is desirable to parse sel in order to more efficiently use querySelectorAll (), getElementsByClassName () and getElementById ().
Thus, we can implement only the functions we need in the JQR class, without going beyond the standard Javascript, and not really touching the existing code.
It is not necessary to even completely get rid of jQuery - partial optimization will already give results.
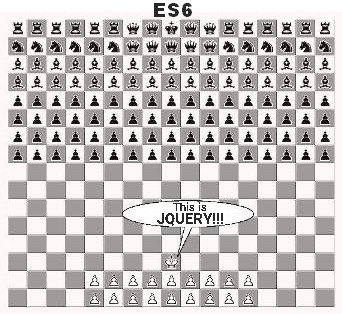
On Habré there were several articles with objective measurements of the "inhibition" of JQuery on selector queries.
| 4,649 ms |
| 3,437 ms |
| 1,362 ms |
| 1,168 ms |
| 107 ms |
| 75 ms |
There are a lot of good descriptions of analogs of jQuery functions on pure Javascript-e — for example, here .
But the power of jQuery is in the brevity and beauty of its expressions. It is even psychologically difficult to rewrite the existing code, changing the elegant $ () into multi-line constructions.
Let's try, as far as possible, to leave the jQuery language, [partially] replacing it with itself. To do this, we only need to either override the $ () function, or replace (which is better) with our own - let it be $ jqr (). It will also return an object, but already “native” and not burdened with unnecessary jQuery functions.
Code example:
<html><body><pid="message"></p></body></html>
Jquery code:
$("#message").html("Hello world!");
Changes to:
$jqr("#message").html("Hello world!");
// JQuery Replacementfunction$jqr(sel) {
returnnew JQR(sel);
}
classJQR{
constructor(sel) {
this.sel = sel;
this.elements = document.querySelectorAll(sel);
}
html(str) {
for (var i = 0; i < this.elements.length; i++) {
this.elements[i].innerHTML = str;
}
}
}
In the class constructor it is desirable to parse sel in order to more efficiently use querySelectorAll (), getElementsByClassName () and getElementById ().
Thus, we can implement only the functions we need in the JQR class, without going beyond the standard Javascript, and not really touching the existing code.
It is not necessary to even completely get rid of jQuery - partial optimization will already give results.