InlineKeyboard in Telegram Bots
InlineKeyboard - a keyboard tied to a message, using a callback (CallbackQuery), instead of sending a message from an ordinary keyboard.
First, create a project on Maven and add the Telegram Bots repository :
Using BotFather, register the bot and get the token:
Next, we create a class Bot, inherit from TelegramLongPollingBot and Ovveraidaim methods:
Create final variables with the bot name and token, add the botUserName method to the getBotUsername () method, and get the to getBotToken () method. In the main method we register the bot:
The bot frame is ready! Now we will write a method with InlineKeyboard.
Create a keyboard layout object:
Now we build the position of the buttons.
Create an InlineKeyboardButton object that has 2 parameters: Text (What will be written on the button itself) and CallBackData (What will be sent to the server when the button is pressed).
Add it to the list, thus creating a series.
If you want to create another row, just make another list and add new buttons to it.
After that we need to integrate the rows, so we create a list of rows.
Feature
Developer took care of us and we can immediately write buttons to the list without creating a variable.
Now we can set the buttons in the keyboard layout object.
If a little is not clear description of the work with the creation of the keyboard, here's a diagram for you:

That's it! Now add the markup to the message:
Now we can send, here's your ready method:
We make a variant when the method is called in the onUpdateReceived request handler:
We try!

Now we need to handle making a new branch in if and processing CallbackQuery:
Check!

All source code:
Example



Creating a bot frame
First, create a project on Maven and add the Telegram Bots repository :
<dependency>
<groupId>org.telegram</groupId>
<artifactId>telegrambots</artifactId>
<version>4.0.0</version>
</dependency>
Using BotFather, register the bot and get the token:
Screen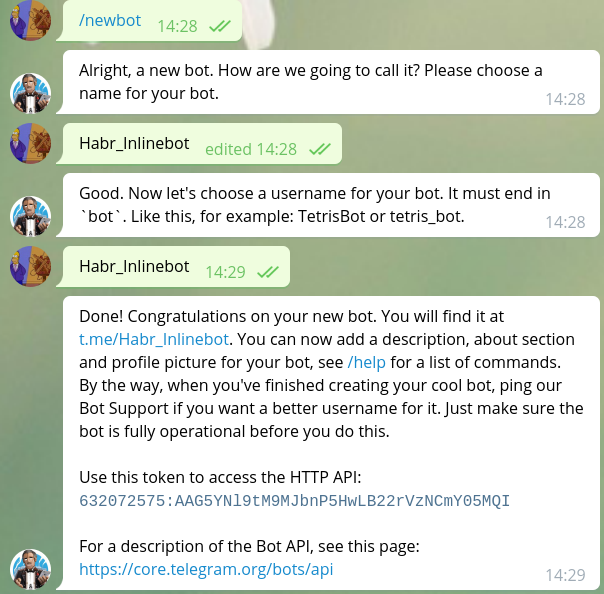
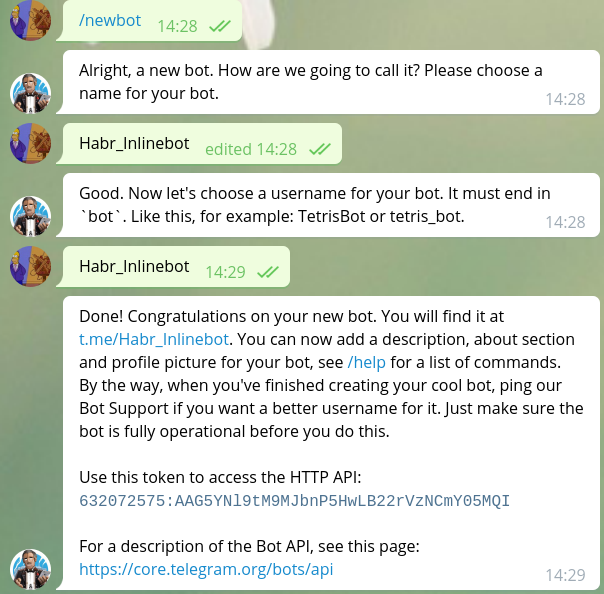
Next, we create a class Bot, inherit from TelegramLongPollingBot and Ovveraidaim methods:
Source
import org.telegram.telegrambots.bots.TelegramLongPollingBot;
import org.telegram.telegrambots.meta.api.objects.Update;
publicclassBotextendsTelegramLongPollingBot{
@OverridepublicvoidonUpdateReceived(Update update){
}
@Overridepublic String getBotUsername(){
returnnull;
}
@Overridepublic String getBotToken(){
returnnull;
}
}
Create final variables with the bot name and token, add the botUserName method to the getBotUsername () method, and get the to getBotToken () method. In the main method we register the bot:
Source
import org.telegram.telegrambots.bots.TelegramLongPollingBot;
import org.telegram.telegrambots.meta.TelegramBotsApi;
import org.telegram.telegrambots.meta.api.objects.Update;
import org.telegram.telegrambots.meta.exceptions.TelegramApiRequestException;
publicclassBotextendsTelegramLongPollingBot{
privatestaticfinal String botUserName = "Habr_Inlinebot";
privatestaticfinal String token = "632072575:AAG5YNl9tM9MJbnP5HwLB22rVzNCmY05MQI";
publicstaticvoidmain(String[] args){
ApiContextInitializer.init();
TelegramBotsApi telegramBotsApi = new TelegramBotsApi();
try {
telegramBotsApi.registerBot(new Bot());
} catch (TelegramApiRequestException e) {
e.printStackTrace();
}
}
@OverridepublicvoidonUpdateReceived(Update update){
}
@Overridepublic String getBotUsername(){
return botUserName;
}
@Overridepublic String getBotToken(){
return token;
}
}
The bot frame is ready! Now we will write a method with InlineKeyboard.
Work with InlineKeyboard
Create a keyboard layout object:
InlineKeyboardMarkup inlineKeyboardMarkup =new InlineKeyboardMarkup();
Now we build the position of the buttons.
Create an InlineKeyboardButton object that has 2 parameters: Text (What will be written on the button itself) and CallBackData (What will be sent to the server when the button is pressed).
InlineKeyboardButton inlineKeyboardButton = new InlineKeyboardButton();
inlineKeyboardButton.setText("Тык");
inlineKeyboardButton.setCallbackData("Button \"Тык\" has been pressed");
Add it to the list, thus creating a series.
List<InlineKeyboardButton> keyboardButtonsRow1 = new ArrayList<>();
keyboardButtons.add(inlineKeyboardButton);
If you want to create another row, just make another list and add new buttons to it.
List<InlineKeyboardButton> keyboardButtonsRow2 = new ArrayList<>();
keyboardButtons.add(inlineKeyboardButton2);
After that we need to integrate the rows, so we create a list of rows.
List<List<InlineKeyboardButton>> rowList= new ArrayList<>();
rowList.add(keyboardButtonsRow1);
rowList.add(keyboardButtonsRow2);
Feature
Developer took care of us and we can immediately write buttons to the list without creating a variable.
keyboardButtonsRow1.add(new InlineKeyboardButton().setText("Fi4a")
.setCallbackData("CallFi4a"));
Now we can set the buttons in the keyboard layout object.
inlineKeyboardMarkup.setKeyboard(rowList);
If a little is not clear description of the work with the creation of the keyboard, here's a diagram for you:

That's it! Now add the markup to the message:
SendMessage message = new SendMessage().setChatId(chatId).setText("Пример") .setReplyMarkup(inlineKeyboardMarkup);
Now we can send, here's your ready method:
Source
publicstatic SendMessage sendInlineKeyBoardMessage(long chatId){
InlineKeyboardMarkup inlineKeyboardMarkup = new InlineKeyboardMarkup();
InlineKeyboardButton inlineKeyboardButton1 = new InlineKeyboardButton();
InlineKeyboardButton inlineKeyboardButton2 = new InlineKeyboardButton();
inlineKeyboardButton1.setText("Тык");
inlineKeyboardButton1.setCallbackData("Button \"Тык\" has been pressed");
inlineKeyboardButton2.setText("Тык2");
inlineKeyboardButton2.setCallbackData("Button \"Тык2\" has been pressed");
List<InlineKeyboardButton> keyboardButtonsRow1 = new ArrayList<>();
List<InlineKeyboardButton> keyboardButtonsRow2 = new ArrayList<>();
keyboardButtonsRow1.add(inlineKeyboardButton1);
keyboardButtonsRow1.add(new InlineKeyboardButton().setText("Fi4a").setCallbackData("CallFi4a"));
keyboardButtonsRow2.add(inlineKeyboardButton2);
List<List<InlineKeyboardButton>> rowList = new ArrayList<>();
rowList.add(keyboardButtonsRow1);
rowList.add(keyboardButtonsRow2);
inlineKeyboardMarkup.setKeyboard(rowList);
returnnew SendMessage().setChatId(chatId).setText("Пример").setReplyMarkup(inlineKeyboardMarkup);
}
We make a variant when the method is called in the onUpdateReceived request handler:
Source
@OverridepublicvoidonUpdateReceived(Update update){
if(update.hasMessage()){
if(update.getMessage().hasText()){
if(update.getMessage().getText().equals("Hello")){
try {
execute(sendInlineKeyBoardMessage(update.getMessage().getChatId()));
} catch (TelegramApiException e) {
e.printStackTrace();
}
}
}
}
}
We try!

Now we need to handle making a new branch in if and processing CallbackQuery:
Source
@OverridepublicvoidonUpdateReceived(Update update){
if(update.hasMessage()){
if(update.getMessage().hasText()){
if(update.getMessage().getText().equals("Hello")){
try {
execute(sendInlineKeyBoardMessage(update.getMessage().getChatId()));
} catch (TelegramApiException e) {
e.printStackTrace();
}
}
}
}elseif(update.hasCallbackQuery()){
try {
execute(new SendMessage().setText(
update.getCallbackQuery().getData())
.setChatId(update.getCallbackQuery().getMessage().getChatId()));
} catch (TelegramApiException e) {
e.printStackTrace();
}
}
}
Check!

On this, perhaps all, thank you for your attention!
All source code:
All code
import org.telegram.telegrambots.ApiContextInitializer;
import org.telegram.telegrambots.bots.TelegramLongPollingBot;
import org.telegram.telegrambots.meta.ApiContext;
import org.telegram.telegrambots.meta.TelegramBotsApi;
import org.telegram.telegrambots.meta.api.methods.send.SendMessage;
import org.telegram.telegrambots.meta.api.objects.Update;
import org.telegram.telegrambots.meta.api.objects.replykeyboard.InlineKeyboardMarkup;
import org.telegram.telegrambots.meta.api.objects.replykeyboard.buttons.InlineKeyboardButton;
import org.telegram.telegrambots.meta.api.objects.replykeyboard.buttons.KeyboardButton;
import org.telegram.telegrambots.meta.exceptions.TelegramApiException;
import org.telegram.telegrambots.meta.exceptions.TelegramApiRequestException;
import java.util.ArrayList;
import java.util.List;
publicclassBotextendsTelegramLongPollingBot{
privatestaticfinal String botUserName = "Habr_Inlinebot";
privatestaticfinal String token = "632072575:AAG5YNl9tM9MJbnP5HwLB22rVzNCmY05MQI";
publicstaticvoidmain(String[] args){
ApiContextInitializer.init();
TelegramBotsApi telegramBotsApi = new TelegramBotsApi();
try {
telegramBotsApi.registerBot(new Bot());
} catch (TelegramApiRequestException e) {
e.printStackTrace();
}
}
@OverridepublicvoidonUpdateReceived(Update update){
if(update.hasMessage()){
if(update.getMessage().hasText()){
if(update.getMessage().getText().equals("Hello")){
try {
execute(sendInlineKeyBoardMessage(update.getMessage().getChatId()));
} catch (TelegramApiException e) {
e.printStackTrace();
}
}
}
}elseif(update.hasCallbackQuery()){
try {
execute(new SendMessage().setText(
update.getCallbackQuery().getData())
.setChatId(update.getCallbackQuery().getMessage().getChatId()));
} catch (TelegramApiException e) {
e.printStackTrace();
}
}
}
@Overridepublic String getBotUsername(){
return botUserName;
}
@Overridepublic String getBotToken(){
return token;
}
publicstatic SendMessage sendInlineKeyBoardMessage(long chatId){
InlineKeyboardMarkup inlineKeyboardMarkup = new InlineKeyboardMarkup();
InlineKeyboardButton inlineKeyboardButton1 = new InlineKeyboardButton();
InlineKeyboardButton inlineKeyboardButton2 = new InlineKeyboardButton();
inlineKeyboardButton1.setText("Тык");
inlineKeyboardButton1.setCallbackData("Button \"Тык\" has been pressed");
inlineKeyboardButton2.setText("Тык2");
inlineKeyboardButton2.setCallbackData("Button \"Тык2\" has been pressed");
List<InlineKeyboardButton> keyboardButtonsRow1 = new ArrayList<>();
List<InlineKeyboardButton> keyboardButtonsRow2 = new ArrayList<>();
keyboardButtonsRow1.add(inlineKeyboardButton1);
keyboardButtonsRow1.add(new InlineKeyboardButton().setText("Fi4a").setCallbackData("CallFi4a"));
keyboardButtonsRow2.add(inlineKeyboardButton2);
List<List<InlineKeyboardButton>> rowList = new ArrayList<>();
rowList.add(keyboardButtonsRow1);
rowList.add(keyboardButtonsRow2);
inlineKeyboardMarkup.setKeyboard(rowList);
returnnew SendMessage().setChatId(chatId).setText("Пример").setReplyMarkup(inlineKeyboardMarkup);
}
}