
SteelToe - object, do not shoot me in the leg!
- Tutorial
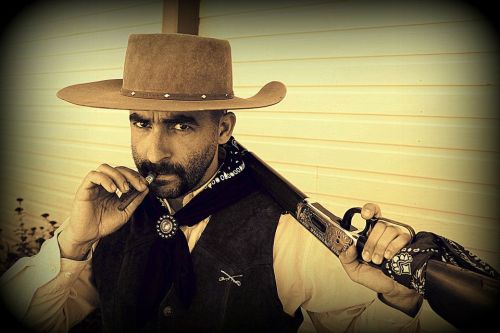
Getting Values
First way
var object = { info: { name: { first: 'Jonathan', last: 'Clem' } } }
steelToe(object)('info')('name')('last')(); // 'Clem'
steelToe(object)('info')('features')('hairColor')(); // undefined
Second way
var object = { info: { name: { first: 'Jonathan', last: 'Clem' } } }
steelToe(object).walk('info.name.last'); // 'Clem'
steelToe(object).walk('info.features.hairColor'); // undefined
Definition of Values
var jonathan = { info: { name: { first: 'Jonathan', last: 'Clem' } } },
steelToe(jonathan).set('info.name.middle', 'Tyler');
steelToe(jonathan).set('info.favorites.movie', 'Harold & Maude');
jonathan.info.name.middle; // Tyler
jonathan.info.favorites.movie; // Harold & Maude
What is going on here
Imagine - you have a complex Javascript object, with a large nesting of properties, which, for example, you got from JSON. And, for example, you need to do something like this:
var fatherFirstNames = [];
for (var i = 0; i < families.length; i ++) {
var first = families[i].father.info.name.first;
if (first) {
fatherFirstNames.push(first);
}
}
// TypeError: 'undefined' is not an object (evaluating 'family.father.info.name.first')
- Bliiin !, you say - a stupid shot in the leg! You can easily fall out an error
TypeError
, because nothing guarantees that a property is father
defined or that it has the properties you require. Usually, to prevent errors of this kind, we write wretchedness, such as thisvar farherFirstNames = [];
for (var i = 0; i < families.length; i++) {
var father = families[i].father;
if (father && father.info && father.info.name && father.info.name.first) {
fatherFirstNames.push(father.info.name.first);
}
}
Bueee ... See how elegantly SteelToe solves this problem!
var fatherFirstNames = [];
for (var i = 0; i < families.length; i++) {
var name = steelToe(families[i]).walk('father.info.name.first');
if (name) {
fatherFirstNames.push(name);
}
}
fatherFirstNames; // ["Hank", "Dale", "Bill"]
No more
TypeError
! Happiness! The library is presented on the site , and on github .