
Programming for the PlayStation 2: Controller Library - Part 2
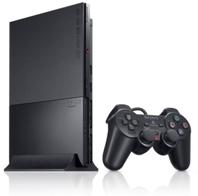
In the last article, I talked about the module exclusively for EE mode. In this chapter I will talk about a rather complicated, difficult to understand, but giving many opportunities module.
This module is recommended to be used for games where only one joystick is used.
There is multitap support, but I will not talk about it for this module.
There is a special libpad2 library. The library uses the Emotion Engine coprocessor (short for EE) and Input Output Processor (IOP).
At the moment, you can use it only for ordinary digital controllers, DualShock and DualShock 2.
Libraries for connecting EE: “libdbc.a” and “libpad2.a”. Header files: "libdbc.h" and "libpad2.h".
Modules for connecting IOP: “sio2man.irx” (version 2.4 or higher), “sio2d.irx”, “dbcman.irx”, controller driver modules (***. Irx).
IOP modules are loaded using the sceSifLoadModule () function after loading the EE application.
Modules use virtual sockets.
The following modules are used to connect controller drivers:
Module name | Digital controller | Dualshock | Dualshock 2 |
ds1u.irx | Supports | Supports | DualShock emulation |
ds2u.irx | Supports | Supports | Supports |
dgco.irx | Supports | Digital controller emulation | Digital controller emulation |
ds1o.irx | Not compatible | Supports | DualShock emulation |
ds2o.irx | Not compatible | Not compatible | Supports |
Drivers switch automatically when certain controllers are connected.
Also, the number of drivers loaded should match the number of controllers used.
How to do it?
Each controller has its own driver.
For controller 1 correspond: ds1u_s1.irx, ds2u_s1.irx, dgco_s1.irx, ds1o_s1.irx, ds2o_s1.irx.
For controller 2: ds1u_d.irx, ds2u_d.irx, dgco_d.irx, ds1o_d.irx, ds2o_d.irx.
Using a second controller is not advisable.
Module Procedures
sceSifLoadModule () - used to load sio2man.irx, sio2d.irx and dbcman.irx in the same sequence.
sceDbcInit () and scePad2Init () - used to initialize libdbc and libpad2.
scePad2CreateSocket () - used to create a socket for working with the controller. The first parameter is the port number (or NULL for the first controller) and the second is a 64-byte profile through which data will be transmitted.
scePad2ButtonProfile () - reads data from a socket and passes it to the buffer. The parameters are socket and buffer.
scePad2Read () - reads button data. The parameters are the same.
scePad2GetState () - checks the status of the controller. The parameters are the same.
scePad2DeleteSocket () - removes a socket. The parameter is the socket number.
scePad2End () and sceDbcEnd () - terminate the libpad2 and libdbc libraries, respectively.
The simplest program code would look like this:
main(){
int socket_number;
unsigned char rdata[32];
unsigned char profile[10];
u_long128 pad_buff[SCE_PAD2_DMA_BUFFER_MAX]__attribute__((aligned(64)));
sceDbcInit();
scePad2Init();
socket_number = scePad2CreateSocket( NULL, pad_buff );
while(1) {
scePad2ButtonProfile( socket_number, profile );
scePad2Read( socket_number, rdata );
//ваш код
}
scePad2DeleteSocket( socket_number );
scePad2End();
sceDbcEnd();
}
* This source code was highlighted with Source Code Highlighter.
Controller Profiles
The profile shows the features of the controllers, i.e. all information about the connected controller, which buttons are present and the number.
Each bit of the profile describes an individual feature of the controller. If the controller has the required capability, then the bit will be set to 1 and 0 - for lack of support.
The profile is 4 bytes of information that Sony wanted to expand in the future.
Byte | Bit | Button | Number of bits allocated |
0 | 0 | Select | 1 |
1 | L3 (clicking on the left stick) | 1 | |
2 | R3 (clicking on the right stick) | 1 | |
3 | Start | 1 | |
4 | Up arrow | 1 | |
5 | Right arrow | 1 | |
6 | Arrow to down | 1 | |
7 | Left arrow | 1 | |
1 | 8 | L2 | 1 |
9 | R2 | 1 | |
10 | L1 | 1 | |
eleven | R1 | 1 | |
12 | Triangle | 1 | |
thirteen | A circle | 1 | |
14 | X | 1 | |
fifteen | Square | 1 | |
2 | 16 | Analog mode: Right stick translated by X | 8 |
17 | Analog mode: Right stick translated by Y | 8 | |
18 | Analog mode: Left stick translated by X | 8 | |
19 | Analog mode: Left stick translated by Y | 8 | |
20 | Analog Mode: Right Arrow | 8 | |
21 | Analog Mode: Left Arrow | 8 | |
22 | Analog Mode: Up Arrow | 8 | |
23 | Analog Mode: Down Arrow | 8 | |
3 | 24 | Analog Mode: Triangle | 8 |
25 | Analog Mode: Circle | 8 | |
26 | Analog Mode: Cross | 8 | |
27 | Analog Mode: Square | 8 | |
28 | Analog Mode: L1 | 8 | |
29th | Analog Mode: R1 | 8 | |
thirty | Analog Mode: L2 | 8 | |
31 | Analog Mode: R2 | 8 |
Analog mode implies sensitivity.
For a digital controller, all analogs, R3 and L3 will be zero. Therefore, the profile for him will look like this:
Byte | Bit | Button | Number of bits allocated |
0 | 0 | Select | 1 |
1 | Start | 1 | |
2 | Up arrow | 1 | |
3 | Right arrow | 1 | |
4 | Arrow to down | 1 | |
5 | Left arrow | 1 | |
6 | L2 | 1 | |
7 | R2 | 1 | |
1 | 8 | L1 | 1 |
9 | R1 | 1 | |
10 | Triangle | 1 | |
eleven | A circle | 1 | |
12 | X | 1 | |
thirteen | Square | 1 | |
14 | -Void- | 1 | |
fifteen | -Void- | 1 |
Cases
It may happen that the user turns off the digital controller and connects an analog one.
The driver will switch automatically. And you may not process the data correctly.
In case the controller is disabled, the scePad2GetState () function will return scePad2StateNoLink. If after that the controller is connected back, the scePad2GetState () function will return scePad2StateStable. It is advisable to check the shutdown by calling the scePad2GetState () function before each frame.
At the end.
This type of information on the controller buttons is suitable for both modes.
It is necessary to monitor the controllers constantly.
On this, only the vibration library remained about the controllers, but I will tell it later, i.e. in the end.
PS : For today, I think that's enough.