Silverlight cookbook: drag & drop recipe
In this article I will analyze the work with drag & drop using an example of such an application:
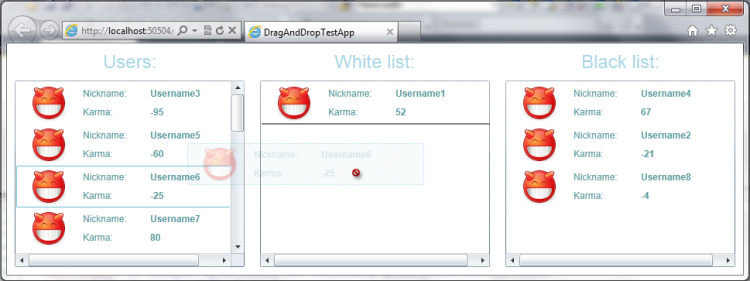
The idea is that from the list of all users we can personally (using drag & drop) sort users into two groups. Moreover, users with negative karma cannot be added to the white list.
But closer to the point. I ask for cat.
Out of the box, we cannot immediately take and start using the drag & drop functionality (we have to implement everything ourselves), but to make our life easier, the specially trained guys from Silverlight Team at Microsoft have already implemented everything in the Silverlight Toolkit .
This functionality is presented in the form of wrappers for some basic controls (User Controls) with the name of the control + DragDropTarget (for a ListBox, this is ListBoxDragDropTarget ). It is on the example of a ListBox, or rather several ListBoxes, that drag & drop will be demonstrated.
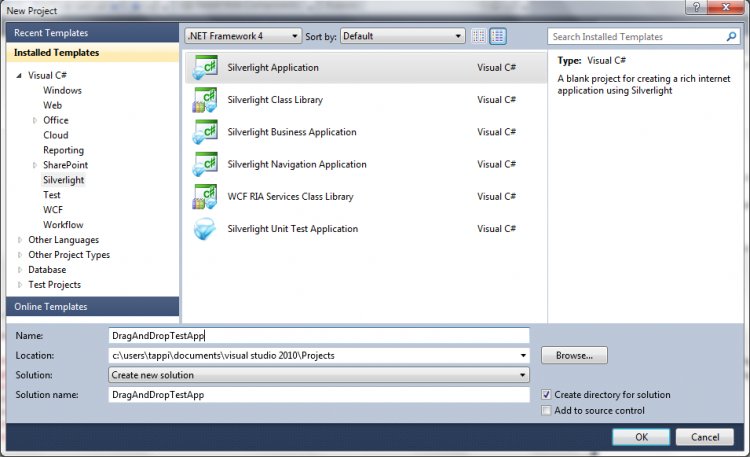
I called it DragAndDropTestApp, which, in fact, does not matter at all.
In the next dialog box, leave everything as is and click OK.
Immediately add a link to the Toolkit:
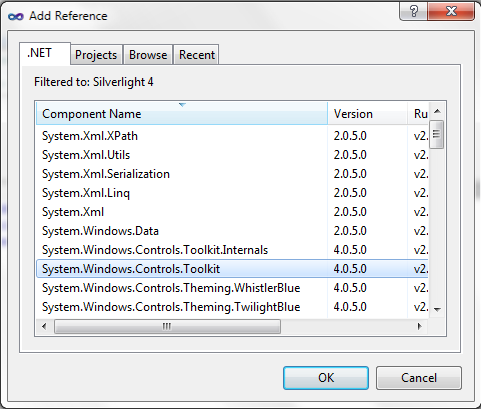
It is assumed that it has already been downloaded and installed.
Add the following namespaces to MainPage.xaml:
On the Toolkit. And to the namespace in which our application lies.
Sketch xaml.
Each of the three lists is represented by the following markup:
TextBox with a title for a list of elements (with all the styles I dabbled in App.xaml). And, actually, the ListBox itself, wrapped in a ListBoxDragDropTarget. ItemTemplate is redefined to display users. UCUser is my own control. There is nothing supernatural inside, see for yourself.
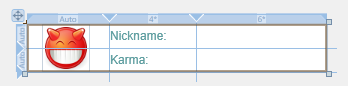
The Source is set for the picture and the data binding (Binding) is registered.
By the way, there is a separate class for the user. Here is his view:
Now create a collection for each list and simulate user loading:
As the DataContext for MainPage, install MainPage itself . I did this so that I could easily attach to the data. It looks like this:
The last touch is missing for Drag & Drop to work:
By setting this property to true, we allow the element to accept data using drag & drop. Moreover, you can install it both in ListBox and ListBoxDragDropTarget.
Here's what happened:

Now you can drag a user with negative karma to any of the lists.
According to the plan, you must prohibit dragging one to the White List.
First, let's look at the events that are generated when Drag-n-Drop'e:

DragEnter occurs when the dragged container crosses the border of the destination element (fires before the DragOver event).
DragLeave - the dragged container was pulled from the source element.
DragOver - fires when the dragged container moves over the destination element.
Drop - the dragged container was “dropped” to the destination element.
Add a DragEnter event handler for the whitelist.
And give ListBox a name, it will come in handy.
The handler itself:
DragEventArgs.Data returns an IDataObject that contains the data associated with the corresponding drag event. But the data cannot be accessed directly, they are marked as Non-Public:
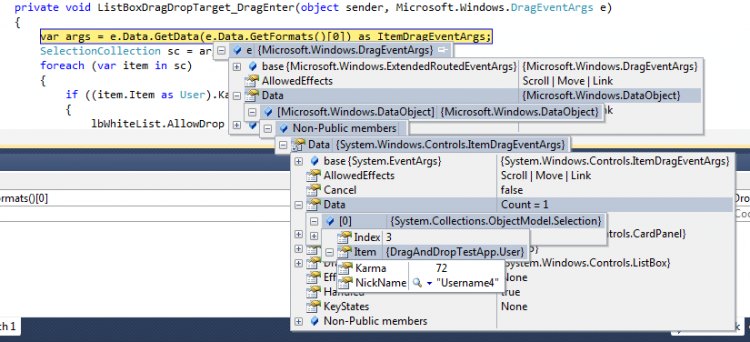
To get this data, the GetData () method is used , which returns the data in the format passed as a parameter (the format can be specified as a string or type). And you can find out the format using the GetFormats () method .
The result is an instance of the ItemDragEventArgs class . This class contains information describing a drag event on a UIElement. It has the Data property , which is already directly related to the draggable container. We bring this object toSelectionCollection .
The collection is used here because we can drag several elements at once
(this can be checked very simply, for the ListBox, set the SelectionMode property to Multiple ).
It remains to run through the entire collection and check whether there are guys with negative karma among the dragged users. If there is, then simply prohibit them "drop" in the white list.
You can return AllowDrop to its previous value (in True) in the MouseLeave event handler .
And lastly, to realize the ability to sort items within a single list using drag-n-drop, simply redefine the template for ItemsPanel:
You can download the project here .
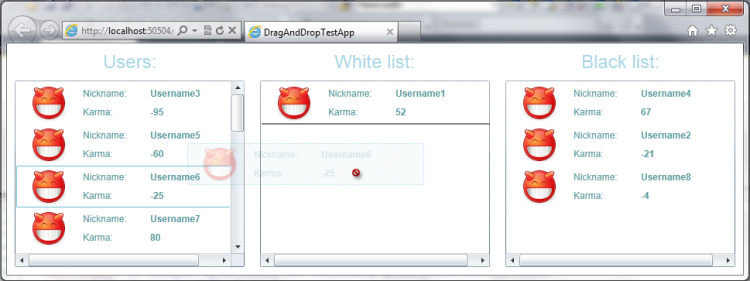
The idea is that from the list of all users we can personally (using drag & drop) sort users into two groups. Moreover, users with negative karma cannot be added to the white list.
But closer to the point. I ask for cat.
So, the introduction.
Out of the box, we cannot immediately take and start using the drag & drop functionality (we have to implement everything ourselves), but to make our life easier, the specially trained guys from Silverlight Team at Microsoft have already implemented everything in the Silverlight Toolkit .
This functionality is presented in the form of wrappers for some basic controls (User Controls) with the name of the control + DragDropTarget (for a ListBox, this is ListBoxDragDropTarget ). It is on the example of a ListBox, or rather several ListBoxes, that drag & drop will be demonstrated.
Let's create a new Silverlight application.
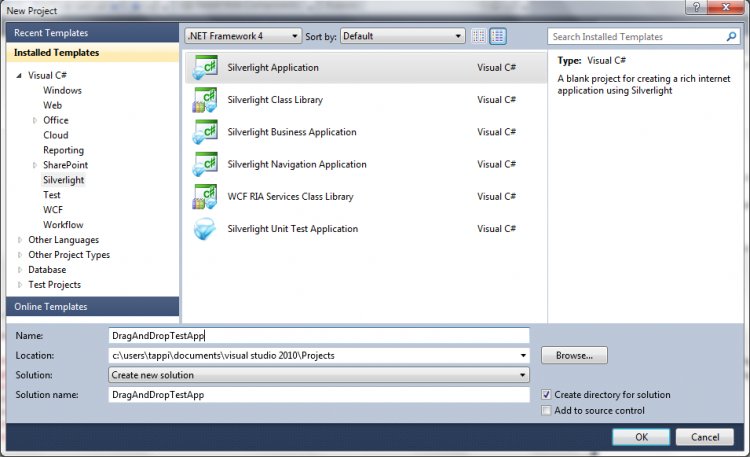
I called it DragAndDropTestApp, which, in fact, does not matter at all.
In the next dialog box, leave everything as is and click OK.
Immediately add a link to the Toolkit:
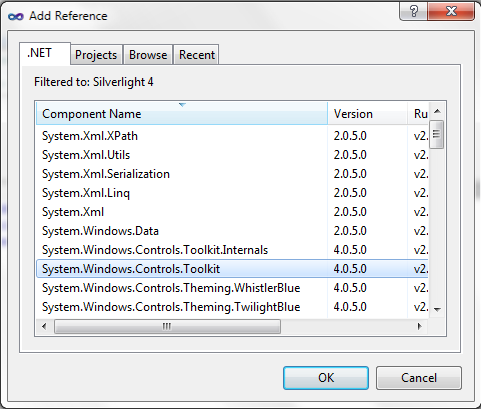
It is assumed that it has already been downloaded and installed.
Add the following namespaces to MainPage.xaml:
xmlns:toolkit="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Toolkit"
xmlns:my="clr-namespace:DragAndDropTestApp"
* All source code (under and below) was highlighted with Source Code Highlighter.
On the Toolkit. And to the namespace in which our application lies.
Sketch xaml.
Each of the three lists is represented by the following markup:
Text="Users:"
Style="{StaticResource Header}"/>
Grid.Row="1"
HorizontalContentAlignment="Stretch"
VerticalContentAlignment="Stretch">
TextBox with a title for a list of elements (with all the styles I dabbled in App.xaml). And, actually, the ListBox itself, wrapped in a ListBoxDragDropTarget. ItemTemplate is redefined to display users. UCUser is my own control. There is nothing supernatural inside, see for yourself.
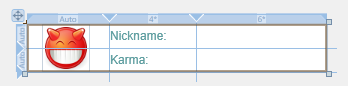
- x:Name="LayoutRoot"
- Background="Transparent">
-
-
-
-
-
-
-
-
-
-
- Source="../Images/gnome_face_devilish.png"
- Width="48"
- Height="48"
- Margin="15, 0, 20, 0"
- Grid.RowSpan="2"/>
-
- Text="Nickname:"
- Grid.Column="1"/>
-
- Text="{Binding Path=NickName, Mode=OneWay}"
- Grid.Column="2"
- FontWeight="Bold"/>
-
- Text="Karma:"
- Grid.Row="1"
- Grid.Column="1" />
-
- Text="{Binding Path=Karma, Mode=OneWay}"
- Grid.Row="1"
- Grid.Column="2"
- FontWeight="Bold" />
-
The Source is set for the picture and the data binding (Binding) is registered.
By the way, there is a separate class for the user. Here is his view:
public class User
{
public string NickName { get; set; }
public int Karma { get; set; }
}
Now create a collection for each list and simulate user loading:
- public partial class MainPage : UserControl
- {
- public MainPage()
- {
- InitializeComponent();
- this.DataContext = this;
- InitializeCollections();
- LoadUsers();
- }
-
- //Объявление коллекций
- public ObservableCollection
Users { get; set; } - public ObservableCollection
WhiteList { get; set; } - public ObservableCollection
BlackList { get; set; } -
- //Инициализация коллекций
- private void InitializeCollections()
- {
- Users = new ObservableCollection
(); - WhiteList = new ObservableCollection
(); - BlackList = new ObservableCollection
(); - }
-
- //Метод, имитирующий загрузку данных в коллекцию Users
- private void LoadUsers()
- {
- var r = new Random();
-
- //Добавление 20 пользователей со случайным значением кармы.
- for (int i = 1; i < 21; i++)
- {
- var newUser = new User
- {
- NickName = "Username" + i,
- Karma = r.Next(200) - 100
- };
-
- Users.Add(newUser);
- }
- }
- }
As the DataContext for MainPage, install MainPage itself . I did this so that I could easily attach to the data. It looks like this:
The last touch is missing for Drag & Drop to work:
AllowDrop="True"
By setting this property to true, we allow the element to accept data using drag & drop. Moreover, you can install it both in ListBox and ListBoxDragDropTarget.
Here's what happened:

Now you can drag a user with negative karma to any of the lists.
According to the plan, you must prohibit dragging one to the White List.
First, let's look at the events that are generated when Drag-n-Drop'e:

DragEnter occurs when the dragged container crosses the border of the destination element (fires before the DragOver event).
DragLeave - the dragged container was pulled from the source element.
DragOver - fires when the dragged container moves over the destination element.
Drop - the dragged container was “dropped” to the destination element.
Add a DragEnter event handler for the whitelist.
-
- AllowDrop="True"
- Grid.Row="1"
- Grid.Column="1"
- HorizontalContentAlignment="Stretch"
- VerticalContentAlignment="Stretch"
- DragEnter="ListBoxDragDropTarget_DragEnter">
-
- ItemsSource="{Binding Path=WhiteList, Mode=TwoWay}"
- x:Name="lbWhiteList">
-
-
-
-
-
-
-
And give ListBox a name, it will come in handy.
The handler itself:
- private void ListBoxDragDropTarget_DragEnter(object sender, Microsoft.Windows.DragEventArgs e)
- {
- //Узнали формат
- var dataFormat = e.Data.GetFormats()[0];
-
- //Получили объект в формате ItemDragEventArgs
- var dragEventArgs = e.Data.GetData(dataFormat) as ItemDragEventArgs;
-
- //Получили коллекцию перетаскиваемых элементов
- SelectionCollection sc = dragEventArgs.Data as SelectionCollection;
-
- //Проверка каждого элемента коллекции
- foreach (var item in sc)
- {
- //Если хоть один из перетаскиваемых элементов (пользователей) имеет отрицательную карму
- //запрещаем добавлять эти элементы в белый список.
- if ((item.Item as User).Karma < 0)
- {
- lbWhiteList.AllowDrop = false;
- break;
- }
- }
- }
DragEventArgs.Data returns an IDataObject that contains the data associated with the corresponding drag event. But the data cannot be accessed directly, they are marked as Non-Public:
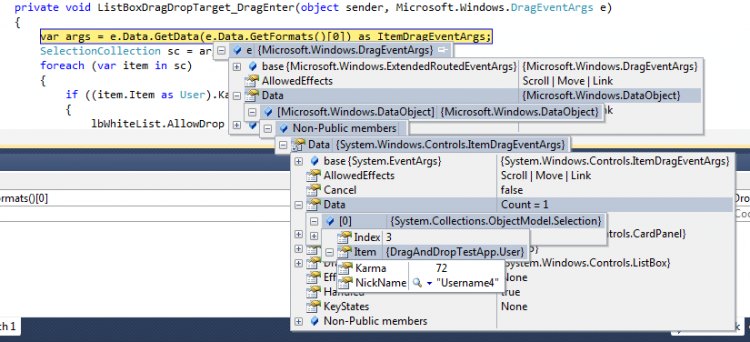
To get this data, the GetData () method is used , which returns the data in the format passed as a parameter (the format can be specified as a string or type). And you can find out the format using the GetFormats () method .
The result is an instance of the ItemDragEventArgs class . This class contains information describing a drag event on a UIElement. It has the Data property , which is already directly related to the draggable container. We bring this object toSelectionCollection .
The collection is used here because we can drag several elements at once
(this can be checked very simply, for the ListBox, set the SelectionMode property to Multiple ).
It remains to run through the entire collection and check whether there are guys with negative karma among the dragged users. If there is, then simply prohibit them "drop" in the white list.
You can return AllowDrop to its previous value (in True) in the MouseLeave event handler .
And lastly, to realize the ability to sort items within a single list using drag-n-drop, simply redefine the template for ItemsPanel:
You can download the project here .